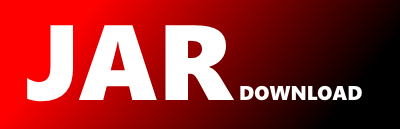
org.ocap.diagnostics.SNMPResponse.html Maven / Gradle / Ivy
SNMPResponse
Overview
Package
Class
Tree
Deprecated
Index
Help
PREV CLASS
NEXT CLASS
FRAMES
NO FRAMES
SUMMARY: NESTED | FIELD | CONSTR | METHOD
DETAIL: FIELD | CONSTR | METHOD
org.ocap.diagnostics
Class SNMPResponse
java.lang.Object
org.ocap.diagnostics.SNMPResponse
public class SNMPResponse
- extends java.lang.Object
This interface represents a response to an implementation request to an application that has registered control over a specific MIB.
Field Summary | |
---|---|
static int |
SNMP_REQUEST_AUTHORIZATION_ERROR
A problem occurred in authorization. |
static int |
SNMP_REQUEST_BAD_VALUE
A Check/Set value (or syntax) error occurred. |
static int |
SNMP_REQUEST_COMMIT_FAILED
An attempt to set a particular variable failed. |
static int |
SNMP_REQUEST_GENERIC_ERROR
Any error not covered by the other error types. |
static int |
SNMP_REQUEST_INCONSISTENT_NAME
The variable does not exist; the agent cannot create it because the named object instance is inconsistent with the values of other managed objects. |
static int |
SNMP_REQUEST_INCONSISTENT_VALUE
A variable binding specifies a value that could be held by the variable but cannot be assigned to it at this time. |
static int |
SNMP_REQUEST_NO_ACCESS
Access was denied to the object for security reasons. |
static int |
SNMP_REQUEST_NO_CREATION
A specified variable does not exist and cannot be created. |
static int |
SNMP_REQUEST_NO_SUCH_NAME
The OID could not be found or there is no OID to respond to in a get next request. |
static int |
SNMP_REQUEST_NOT_WRITABLE
The variable does not exist; the agent cannot create it because the named object instance is inconsistent with the values of other managed objects. |
static int |
SNMP_REQUEST_READ_ONLY
An attempt was made to set a variable that has an access value of Read-Only |
static int |
SNMP_REQUEST_RESOURCE_UNAVAILABLE
An attempt to set a variable required a resource that is not available. |
static int |
SNMP_REQUEST_SUCCESS
The request completed successfully and the MIBObject contains valid contents. |
static int |
SNMP_REQUEST_TOO_BIG
The size of the Response-PDU would be too large to transport. |
static int |
SNMP_REQUEST_UNDO_FAILED
An attempt to set a particular variable as part of a group of variables failed, and the attempt to then undo the setting of other variables was not successful. |
static int |
SNMP_REQUEST_WRONG_ENCODING
A variable binding specifies an encoding incorrect for the object. |
static int |
SNMP_REQUEST_WRONG_LENGTH
A variable binding specifies a length incorrect for the object. |
static int |
SNMP_REQUEST_WRONG_TYPE
The object type in the request is incorrect for the object. |
static int |
SNMP_REQUEST_WRONG_VALUE
The value given in a variable binding is not possible for the object |
Constructor Summary | |
---|---|
SNMPResponse(int status,
MIBObject object)
Constructs an SNMPResponse. |
Method Summary | |
---|---|
MIBObject |
getMIBObject()
Gets the encoding of the MIB object associated with the OID in the request that caused this response. |
int |
getStatus()
Get the status of the response. |
Methods inherited from class java.lang.Object |
---|
clone, equals, finalize, getClass, hashCode, notify, notifyAll, toString, wait, wait, wait |
Field Detail |
---|
SNMP_REQUEST_SUCCESS
public static final int SNMP_REQUEST_SUCCESS
- The request completed successfully and the MIBObject contains valid
contents.
- See Also:
- Constant Field Values
SNMP_REQUEST_TOO_BIG
public static final int SNMP_REQUEST_TOO_BIG
- The size of the Response-PDU would be too large to transport.
- See Also:
- Constant Field Values
SNMP_REQUEST_NO_SUCH_NAME
public static final int SNMP_REQUEST_NO_SUCH_NAME
- The OID could not be found or there is no OID to respond to in a
get next request.
- See Also:
- Constant Field Values
SNMP_REQUEST_BAD_VALUE
public static final int SNMP_REQUEST_BAD_VALUE
- A Check/Set value (or syntax) error occurred.
- See Also:
- Constant Field Values
SNMP_REQUEST_READ_ONLY
public static final int SNMP_REQUEST_READ_ONLY
- An attempt was made to set a variable that
has an access value of Read-Only
- See Also:
- Constant Field Values
SNMP_REQUEST_GENERIC_ERROR
public static final int SNMP_REQUEST_GENERIC_ERROR
- Any error not covered by the other error types.
- See Also:
- Constant Field Values
SNMP_REQUEST_NO_ACCESS
public static final int SNMP_REQUEST_NO_ACCESS
- Access was denied to the object for security reasons.
- See Also:
- Constant Field Values
SNMP_REQUEST_WRONG_TYPE
public static final int SNMP_REQUEST_WRONG_TYPE
- The object type in the request is incorrect for the object.
- See Also:
- Constant Field Values
SNMP_REQUEST_WRONG_LENGTH
public static final int SNMP_REQUEST_WRONG_LENGTH
- A variable binding specifies a length incorrect for the object.
- See Also:
- Constant Field Values
SNMP_REQUEST_WRONG_ENCODING
public static final int SNMP_REQUEST_WRONG_ENCODING
- A variable binding specifies an encoding incorrect for the object.
- See Also:
- Constant Field Values
SNMP_REQUEST_WRONG_VALUE
public static final int SNMP_REQUEST_WRONG_VALUE
- The value given in a variable binding is not possible for the object
- See Also:
- Constant Field Values
SNMP_REQUEST_NO_CREATION
public static final int SNMP_REQUEST_NO_CREATION
- A specified variable does not exist and cannot be created.
- See Also:
- Constant Field Values
SNMP_REQUEST_INCONSISTENT_VALUE
public static final int SNMP_REQUEST_INCONSISTENT_VALUE
- A variable binding specifies a value that could be held by
the variable but cannot be assigned to it at this time.
(For example, is not CURRENTLY valid to set because of the
value of another MIB object, e.g. one MIB value indicates
if a clock display is 12 or 24 hours, and is set to 12,
but then someone tries to set the time to 13:00)
- See Also:
- Constant Field Values
SNMP_REQUEST_RESOURCE_UNAVAILABLE
public static final int SNMP_REQUEST_RESOURCE_UNAVAILABLE
- An attempt to set a variable required a resource
that is not available.
- See Also:
- Constant Field Values
SNMP_REQUEST_COMMIT_FAILED
public static final int SNMP_REQUEST_COMMIT_FAILED
- An attempt to set a particular variable failed.
- See Also:
- Constant Field Values
SNMP_REQUEST_UNDO_FAILED
public static final int SNMP_REQUEST_UNDO_FAILED
- An attempt to set a particular variable as part of a group of
variables failed, and the attempt to then undo the setting of
other variables was not successful.
- See Also:
- Constant Field Values
SNMP_REQUEST_AUTHORIZATION_ERROR
public static final int SNMP_REQUEST_AUTHORIZATION_ERROR
- A problem occurred in authorization.
- See Also:
- Constant Field Values
SNMP_REQUEST_NOT_WRITABLE
public static final int SNMP_REQUEST_NOT_WRITABLE
- The variable does not exist; the agent cannot create
it because the named object instance is inconsistent
with the values of other managed objects.
- See Also:
- Constant Field Values
SNMP_REQUEST_INCONSISTENT_NAME
public static final int SNMP_REQUEST_INCONSISTENT_NAME
- The variable does not exist; the agent cannot create it
because the named object instance is inconsistent with
the values of other managed objects.
- See Also:
- Constant Field Values
Constructor Detail |
---|
SNMPResponse
public SNMPResponse(int status, MIBObject object)
- Constructs an SNMPResponse.
- Parameters:
status
- Status of the corresponding request. Possible values include any of the SNMP_REQUEST_* constants in this class.object
- MIBObject resulting from the corresponding request.
Method Detail |
---|
getStatus
public int getStatus()
- Get the status of the response.
- Returns:
- One of the request constants defined in this interface.
getMIBObject
public MIBObject getMIBObject()
- Gets the encoding of the MIB object associated with the OID in the
request that caused this response.
- Returns:
- If the getStatus method returns SNMP_REQUEST_SUCCESS this method SHALL return a populated MIB Object, otherwise the MIB object returned SHALL contain an empty data array with length 0.
|
|||||||||
PREV CLASS NEXT CLASS | FRAMES NO FRAMES | ||||||||
SUMMARY: NESTED | FIELD | CONSTR | METHOD | DETAIL: FIELD | CONSTR | METHOD |
© 2015 - 2025 Weber Informatics LLC | Privacy Policy