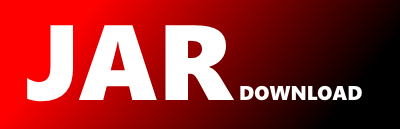
org.ocap.hardware.Host.html Maven / Gradle / Ivy
Host
Overview
Package
Class
Tree
Deprecated
Index
Help
PREV CLASS
NEXT CLASS
FRAMES
NO FRAMES
SUMMARY: NESTED | FIELD | CONSTR | METHOD
DETAIL: FIELD | CONSTR | METHOD
org.ocap.hardware
Class Host
java.lang.Object
org.ocap.hardware.Host
public class Host
- extends java.lang.Object
This class represents the host terminal device and provides access to the Host ID, raw image data, the power state of the host and a java.util.Enumeration of references to VideoOutputPort instances. See also org.ocap.OcapSystem to get the singleton instance.
Field Summary | |
---|---|
static int |
FULL_POWER
Power mode constant for normal "on" mode. |
static int |
LOW_POWER
Power mode constant for "standby" mode. |
Constructor Summary | |
---|---|
protected |
Host()
A constructor of this class. |
Method Summary | |
---|---|
void |
addPowerModeChangeListener(PowerModeChangeListener l)
Adds the PowerModeChangeListener to be called ( PowerModeChangeListener.powerModeChanged(int)
when the power mode of the box changes (for example when the user presses the Power button). |
void |
codeDownload()
This method initiates a download of the operating software in the Host as specified by [CCIF2.0]. |
boolean |
getACOutlet()
Query whether power to the AC Outlet, if present, is currently On (true) or Off (false) NOTE: AC Outlet refers to an external power plug on the STB. |
java.lang.String |
getID()
Get a human-readable string representing the ID of this Host. |
static Host |
getInstance()
This method returns a singleton system-wide instance of the Host class. |
int |
getPowerMode()
|
java.lang.String |
getReverseChannelMAC()
Gets the MAC address used by the Host for reverse channel unicast communications. |
boolean |
getRFBypass()
Queries whether RF Bypass is currently enabled. |
boolean |
getRFBypassCapability()
Returns capability of RF bypass control on the host. |
java.util.Enumeration |
getVideoOutputPorts()
This method returns a java.util.Enumeration of references to VideoOutputPort instances. |
boolean |
isACOutletPresent()
Query whether there is an AC Outlet on the STB. |
void |
reboot()
This method initiates a reboot of the Host device. |
void |
removePowerModeChangeListener(PowerModeChangeListener l)
Removes the previously-added PowerModeChangeListener. |
void |
removeXAIT()
Removes the XAIT saved to persistent storage. |
void |
setACOutlet(boolean enable)
Switch power to AC Outlet, if present, On (true) or Off (false) NOTE: AC Outlet refers to an external power plug on the STB. |
void |
setPowerMode(int mode)
Transition the power mode of the system to the given mode. |
void |
setRFBypass(boolean enable)
Enables or disables RF Bypass. |
Methods inherited from class java.lang.Object |
---|
clone, equals, finalize, getClass, hashCode, notify, notifyAll, toString, wait, wait, wait |
Field Detail |
---|
FULL_POWER
public static final int FULL_POWER
- Power mode constant for normal "on" mode.
- See Also:
- Constant Field Values
LOW_POWER
public static final int LOW_POWER
- Power mode constant for "standby" mode.
- See Also:
- Constant Field Values
Constructor Detail |
---|
Host
protected Host()
- A constructor of this class. An application must use the
getInstance()
method to create an instance.
Method Detail |
---|
getInstance
public static Host getInstance()
- This method returns a singleton system-wide instance of the Host class.
- Returns:
- a singleton Host instance.
getID
public java.lang.String getID()
- Get a human-readable string representing the ID of this Host. This
should be a string that could be read over the phone to an MSO that
uniquely identifies the Host.
- Returns:
- id String host id
getPowerMode
public int getPowerMode()
- Returns:
- the current power mode of the box (for example LOW_POWER).
- See Also:
FULL_POWER
,LOW_POWER
getReverseChannelMAC
public java.lang.String getReverseChannelMAC()
- Gets the MAC address used by the Host for reverse channel unicast
communications. This value SHALL match the value the Host would
use in DSG mode for a DHCP request. The format of the String returned
SHALL be six pairs of characters where each pair represents a
hexadecimal byte value of the address and where each pair is separated
by a colon. For example "0D:0E:0F:10:11:12". The first byte
representation in the String starting at location 0 SHALL be the most
significant byte in the address.
- Returns:
- MAC address of the Host.
addPowerModeChangeListener
public void addPowerModeChangeListener(PowerModeChangeListener l)
- Adds the PowerModeChangeListener to be called (
PowerModeChangeListener.powerModeChanged(int)
when the power mode of the box changes (for example when the user presses the Power button).- Parameters:
l
- is an instance implementing PowerModeChangeListener whose powerModeChanged method will be called when the power mode of the Host Device changes.
removePowerModeChangeListener
public void removePowerModeChangeListener(PowerModeChangeListener l)
- Removes the previously-added PowerModeChangeListener.
- Parameters:
l
- is the PowerModeChangeListener to disable. Does nothing if l was never added, has been removed, or is null.
getVideoOutputPorts
public java.util.Enumeration getVideoOutputPorts()
- This method returns a java.util.Enumeration of references to
VideoOutputPort instances.
- Returns:
- the java.util.Enumeration of references to VideoOutputPort instances.
reboot
public void reboot()
This method initiates a reboot of the Host device. The method caller shall have the MonitorAppPermission("reboot").
Note that the
SystemEventListener.notifyEvent(org.ocap.system.event.SystemEvent)
method SHALL be called before the initiated reboot is performed by the Host device. The monitor application MAY clean up resources in the SystemEventListener.notifyEvent method call. After the SystemEventListener.notifyEvent method call returns, the Host device SHALL continue the reboot following the boot process described in the Boot Process Section of this specification.- Throws:
java.lang.SecurityException
- if the caller does not have the MonitorAppPermission("reboot").
codeDownload
public void codeDownload()
This method initiates a download of the operating software in the Host as specified by [CCIF2.0].
- Throws:
java.lang.SecurityException
- if the caller does not have MonitorAppPermission("codeDownload").
isACOutletPresent
public boolean isACOutletPresent()
- Query whether there is an AC Outlet on the STB.
NOTE: AC Outlet refers to an external power plug on the STB. That is, a device such as a VCR can plug
into the STB for power.
- Returns:
- true if there is an AC Outlet, else false.
getACOutlet
public boolean getACOutlet()
- Query whether power to the AC Outlet, if present, is currently On (true) or Off (false)
NOTE: AC Outlet refers to an external power plug on the STB. That is, a device such as a VCR can plug
into the STB for power.
- Returns:
- The current AC Outlet status (false = Off, true = On).
- Throws:
java.lang.IllegalStateException
- if this method is called when there is no AC Outlet.
setACOutlet
public void setACOutlet(boolean enable)
- Switch power to AC Outlet, if present, On (true) or Off (false)
NOTE: AC Outlet refers to an external power plug on the STB. That is, a device such as a VCR can plug
into the STB for power.
- Parameters:
enable
- The power setting for the AC Outlet.- Throws:
java.lang.IllegalStateException
- if this method is called when there is no AC Outlet.
getRFBypassCapability
public boolean getRFBypassCapability()
- Returns capability of RF bypass control on the host.
- Returns:
- true if the host can control RF bypass on/off, else false.
getRFBypass
public boolean getRFBypass()
- Queries whether RF Bypass is currently enabled. If RF Bypass is enabled,
the incoming RF signal is directly routed to the RF output port when the
host is in a stand by mode, thereby totally bypassing the host.
- Returns:
- true if RF Bypass is currently enabled, else false. If the host doesn?t support RF bypass, false returns.
setRFBypass
public void setRFBypass(boolean enable)
- Enables or disables RF Bypass. If RF Bypass is enabled, the incoming RF
signal is directly routed to the RF output port when the host is in a
stand by mode, thereby totally bypassing the host.
- Parameters:
enable
- If true, RF Bypass will be enabled. Otherwise it will be disabled.- Throws:
java.lang.IllegalStateException
- if the host doesn?t support RF bypass.
removeXAIT
public void removeXAIT()
- Removes the XAIT saved to persistent storage. If no XAIT is present
in persistent storage this method does nothing successfully. This
method SHALL NOT affect a cached XAIT and any running applications.
- Throws:
java.lang.SecurityException
- if the calling application is not granted MonitorAppPermission("storage").
setPowerMode
public void setPowerMode(int mode)
- Transition the power mode of the system to the given mode.
If the power mode is already in the target mode, this method SHALL do nothing. Setting host power mode to low-power SHALL NOT disrupt any ongoing recording. In devices where a separate power mode is maintained for standby recordings, setting the power mode to low-power SHALL transition to standby-recording power mode when a recording is in progress.
A change of power mode SHALL be communicated to installed
PowerModeChangeListener
s.- Parameters:
mode
- The new power mode for the system.- Throws:
java.lang.IllegalArgumentException
- if mode is not one ofFULL_POWER
orLOW_POWER
java.lang.SecurityException
- if the caller does not haveMonitorAppPermission("powerMode")
|
|||||||||
PREV CLASS NEXT CLASS | FRAMES NO FRAMES | ||||||||
SUMMARY: NESTED | FIELD | CONSTR | METHOD | DETAIL: FIELD | CONSTR | METHOD |