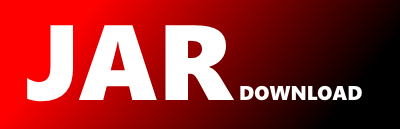
mockit.internal.expectations.UnorderedVerificationPhase Maven / Gradle / Ivy
/*
* Copyright (c) 2006-2011 Rogério Liesenfeld
* This file is subject to the terms of the MIT license (see LICENSE.txt).
*/
package mockit.internal.expectations;
import java.util.*;
import mockit.internal.expectations.invocation.*;
final class UnorderedVerificationPhase extends BaseVerificationPhase
{
final List verifiedExpectations;
private Expectation aggregate;
UnorderedVerificationPhase(
RecordAndReplayExecution recordAndReplay,
List expectationsInReplayOrder, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy