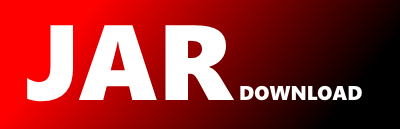
mockit.internal.expectations.mocking.TestedClassInstantiations Maven / Gradle / Ivy
/*
* Copyright (c) 2006-2011 Rogério Liesenfeld
* This file is subject to the terms of the MIT license (see LICENSE.txt).
*/
package mockit.internal.expectations.mocking;
import java.lang.reflect.*;
import java.util.*;
import static java.lang.reflect.Modifier.*;
import static mockit.internal.util.Utilities.*;
public final class TestedClassInstantiations
{
private final List testedFields;
private final List injectableFields;
TestedClassInstantiations(TestedClassRedefinitions redefinitions)
{
testedFields = redefinitions.testedFields;
injectableFields = redefinitions.injectableFields;
}
public void assignNewInstancesToTestedFields(Object objectWithFields)
{
for (Field testedField : testedFields) {
Object originalFieldValue = getFieldValue(testedField, objectWithFields);
if (originalFieldValue == null) {
new TestedObjectCreation(objectWithFields, testedField).create();
}
}
}
private final class TestedObjectCreation
{
final Object objectWithFields;
final Field testedField;
final Class> testedClass;
Constructor> constructor;
Type[] parameterTypes;
Type declaredType;
TestedObjectCreation(Object objectWithFields, Field testedField)
{
this.objectWithFields = objectWithFields;
this.testedField = testedField;
testedClass = testedField.getType();
}
void create()
{
findSingleConstructorAccordingToClassVisibility();
if (constructor != null) {
Object testedObject = instantiateUsingConstructor();
injectIntoFieldsThatAreStillNull(testedClass, testedObject);
setFieldValue(testedField, objectWithFields, testedObject);
}
}
private void findSingleConstructorAccordingToClassVisibility()
{
Constructor>[] constructors;
if (isPublic(testedClass.getModifiers())) {
constructors = testedClass.getConstructors();
if (constructors.length == 1) {
constructor = constructors[0];
return;
}
}
constructors = testedClass.getDeclaredConstructors();
for (Constructor> c : constructors) {
if (c.getModifiers() == 0) {
if (constructor == null) {
constructor = c;
}
else {
constructor = null;
return;
}
}
}
}
private Object instantiateUsingConstructor()
{
parameterTypes = constructor.getGenericParameterTypes();
Object[] arguments = obtainInjectedConstructorArguments();
return invoke(constructor, arguments);
}
private Object[] obtainInjectedConstructorArguments()
{
int n = parameterTypes.length;
Object[] parameterValues = new Object[n];
if (constructor.isVarArgs()) {
n--;
}
for (int i = 0; i < n; i++) {
declaredType = parameterTypes[i];
int position = findRelativePositionForInjectableField(i);
parameterValues[i] = getArgumentValueToInject(position);
}
if (constructor.isVarArgs()) {
parameterValues[n] = obtainInjectedValuesForVarargsParameter(n);
}
return parameterValues;
}
private Object obtainInjectedValuesForVarargsParameter(int varargsParameterIndex)
{
declaredType = ((Class>) parameterTypes[varargsParameterIndex]).getComponentType();
int position = findRelativePositionForInjectableField(varargsParameterIndex) + 1;
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy