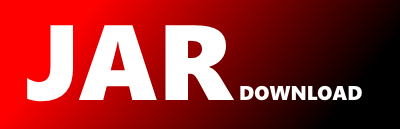
com.googlecode.jpattern.rest.RestServletResourceMap Maven / Gradle / Ivy
package com.googlecode.jpattern.rest;
import java.util.HashMap;
import java.util.Map;
import com.googlecode.jpattern.rest.action.ActionWrapper;
import com.googlecode.jpattern.rest.action.IAction;
import com.googlecode.jpattern.rest.action.IActionWrapper;
import com.googlecode.jpattern.rest.action.IDeleteAction;
import com.googlecode.jpattern.rest.action.IGetAction;
import com.googlecode.jpattern.rest.action.IPostAction;
import com.googlecode.jpattern.rest.action.IPutAction;
import com.googlecode.jpattern.rest.action.NullAction;
import com.googlecode.jpattern.logger.ILoggerFactory;
/**
*
* @author Francesco Cina'
*
* 11/mag/2011
*/
public class RestServletResourceMap implements IRestServletResourceMap {
private static final long serialVersionUID = 1L;
Map postMap = new HashMap();
Map getMap = new HashMap();
Map putMap = new HashMap();
Map deleteMap = new HashMap();
private final ILoggerFactory loggerFactory;
public RestServletResourceMap(ILoggerFactory loggerFactory) {
this.loggerFactory = loggerFactory;
}
@Override
public void addAction(String path, IAction action) {
addPut(path, action);
addPost(path, action);
addGet(path, action);
addDelete(path, action);
}
@Override
public IActionWrapper getAction(String pathInfo) {
String path = getBestKey(pathInfo, getMap);
if (getMap.containsKey(path)) {
return new ActionWrapper(getMap.get(path), getRelativePath(pathInfo, path));
}
return new ActionWrapper(new NullAction(), (path));
}
@Override
public IActionWrapper deleteAction(String pathInfo) {
String path = getBestKey(pathInfo, deleteMap);
if (deleteMap.containsKey(path)) {
return new ActionWrapper(deleteMap.get(path), getRelativePath(pathInfo, path));
}
return new ActionWrapper(new NullAction(), (path));
}
@Override
public IActionWrapper postAction(String pathInfo) {
String path = getBestKey(pathInfo, postMap);
if (postMap.containsKey(path)) {
return new ActionWrapper(postMap.get(path), getRelativePath(pathInfo, path));
}
return new ActionWrapper(new NullAction(), (path));
}
@Override
public IActionWrapper putAction(String pathInfo) {
String path = getBestKey(pathInfo, putMap);
if (putMap.containsKey(path)) {
return new ActionWrapper(putMap.get(path), getRelativePath(pathInfo, path));
}
return new ActionWrapper(new NullAction(), (path));
}
private void addPost(String path, IAction action) {
if (action instanceof IPostAction) {
postMap.put(path, (IPostAction) action);
}
}
private void addPut(String path, IAction action) {
if (action instanceof IPutAction) {
putMap.put(path, (IPutAction) action);
}
}
private void addGet(String path, IAction action) {
if (action instanceof IGetAction) {
getMap.put(path, (IGetAction) action);
}
}
private void addDelete(String path, IAction action) {
if (action instanceof IDeleteAction) {
deleteMap.put(path, (IDeleteAction) action);
}
}
protected String getBestKey(String url, Map map) {
String temp = url;
while (!temp.isEmpty()) {
if ( map.containsKey(temp) ) {
return temp;
}
int index = temp.lastIndexOf("/");
if (index>=0) {
temp = temp.substring(0, index);
} else {
temp="";
}
}
return temp;
}
protected String getRelativePath( String fullpath, String subPath ) {
if (fullpath.length()>=subPath.length()) {
return fullpath.substring(subPath.length(), fullpath.length());
}
return fullpath;
}
@Override
public ILoggerFactory loggerFactory() {
return loggerFactory;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy