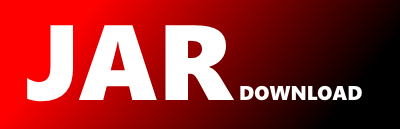
com.googlecode.kevinarpe.papaya.filesystem.TraversePathIterable Maven / Gradle / Ivy
package com.googlecode.kevinarpe.papaya.filesystem;
/*
* #%L
* This file is part of Papaya.
* %%
* Copyright (C) 2013 - 2014 Kevin Connor ARPE ([email protected])
* %%
* Papaya is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* GPL Classpath Exception:
* This project is subject to the "Classpath" exception as provided in
* the LICENSE file that accompanied this code.
*
* Papaya is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with Papaya. If not, see .
* #L%
*/
import com.googlecode.kevinarpe.papaya.compare.ComparatorUtils;
import java.io.File;
import java.util.Collection;
import java.util.Comparator;
/**
* @author Kevin Connor ARPE ([email protected])
*/
public interface TraversePathIterable
extends TraversePathIterSettings, Iterable {
/**
* Constructs a new iterable from the current, replacing the root directory to traverse.
* If the depth policy is {@link TraversePathDepthPolicy#DEPTH_FIRST}, this directory will be
* the last path considered for iteration (depending upon the filter). The vice versa
* also holds true for {@link TraversePathDepthPolicy#DEPTH_LAST}: this directory will be the
* first path considered for iteration (depending upon the filter).
*
* @param rootDirPath
* root directory to traverse. Must not be {@code null}, but need not exist. Directory
* trees may be highly ephemeral, so the existance of this directory at the time of
* construction is not required.
*
* @return new iterable
*
* @throws NullPointerException
* if {@code rootDirPath} is {@code null}
*
* @see #withRootDirPath()
*/
TraversePathIterable withRootDirPath(File rootDirPath);
/**
* Constructs a new iterable from the current, replacing the depth policy.
*
* @param depthPolicy
* when to descend directories: first or last. Must not be {@code null}.
*
* @return new iterable
*
* @throws NullPointerException
* if {@code depthPolicy} is {@code null}
*
* @see #withDepthPolicy()
*/
TraversePathIterable withDepthPolicy(TraversePathDepthPolicy depthPolicy);
/**
* Constructs a new iterable from the current, replacing the exception policy. The
* default value is {@link #DEFAULT_EXCEPTION_POLICY}.
*
* @param exceptionPolicy
* how to handle exceptions thrown during directory listings. Must not be {@code null}.
*
* @return new iterable
*
* @throws NullPointerException
* if {@code exceptionPolicy} is {@code null}
*
* @see #DEFAULT_EXCEPTION_POLICY
* @see #withExceptionPolicy()
*/
TraversePathIterable withExceptionPolicy(TraversePathExceptionPolicy exceptionPolicy);
/**
* Constructs a new iterable from the current, replacing the optional descend directory
* path filter. This attribute filters directories before traversal, including the root
* directory: {@link #withRootDirPath()}. Use the second parameter, {@code depth}, in method
* {@link PathFilter#accept(File, int)} to easily control this case.
*
* Example:
*
* new PathFilter() {
* @Override
* public boolean accept(File path, int depth) {
* if (0 == depth) {
* return true; // always accept the root directory
* }
* // else, apply different logic
* }
* }
*
*
* If the path filter is {@code null}, then all directories are traversed. To combine
* more than one path filter, consider using {@link PathFilterUtils#anyOf(Collection)} or
* {@link PathFilterUtils#allOf(Collection)}. To control which paths are iterated, see
* {@link #withOptionalIteratePathFilter(PathFilter)}.
*
* @param optDescendDirPathFilter
* path filter for descend directories. May be {@code null}.
*
* @return new iterable
*
* @see #withOptionalDescendDirPathFilter()
*/
TraversePathIterable withOptionalDescendDirPathFilter(PathFilter optDescendDirPathFilter);
/**
* Constructs a new iterable from the current, replacing the optional descend directory
* comparator. This attribute sorts directories before traversal. If {@code null}, directories
* are not sorted before traversal. To combine more than one comparator, consider using
* {@link ComparatorUtils#chain(Collection)}.
*
* To control the order paths are iterated, see
* {@link #withOptionalIteratePathComparator(Comparator)}.
*
* @param optDescendDirPathComparator
* comparator to sort descend directories. May be {@code null}.
*
* @return new iterable
*
* @see #withOptionalDescendDirPathComparator()
*/
TraversePathIterable withOptionalDescendDirPathComparator(
Comparator optDescendDirPathComparator);
/**
* Constructs a new iterable from the current, replacing the optional iterated paths
* filter. This attribute filters paths before iteration, including the root directory:
* {@link #withRootDirPath()}. Use the second parameter, {@code depth}, in method
* {@link PathFilter#accept(File, int)} to easily control this case.
*
* Example:
*
* new PathFilter() {
* @Override
* public boolean accept(File path, int depth) {
* if (0 == depth) {
* return true; // always accept the root directory
* }
* // else, apply different logic
* }
* }
*
*
* If the path filter is {@code null}, then all paths are iterated, including
* directories. To combine more than one path filter, consider using
* {@link PathFilterUtils#anyOf(Collection)} or {@link PathFilterUtils#allOf(Collection)}.
*
* To control which directories are traversed, see
* {@link #withOptionalIteratePathFilter(PathFilter)}.
* To control how directories are paths are traversed, see
* {@link #withOptionalDescendDirPathFilter(PathFilter)}.
*
* @param optIteratePathFilter
* path filter for iterated paths. May be {@code null}.
*
* @return new iterable
*
* @see #withOptionalIteratePathFilter()
*/
TraversePathIterable withOptionalIteratePathFilter(PathFilter optIteratePathFilter);
/**
* Constructs a new iterable from the current, replacing the optional iterated paths
* comparator. This attribute sorts paths before iteration. If {@code null}, paths are
* not sorted before iteration. To combine more than one comparator, consider using
* {@link ComparatorUtils#chain(Collection)}.
*
* To control the order directories are traversed, see
* {@link #withOptionalDescendDirPathComparator(Comparator)}.
*
* @param optIteratePathComparator
* comparator to sort iterated paths. May be {@code null}.
*
* @return new iterable
*
* @see #withOptionalIteratePathComparator()
*/
TraversePathIterable withOptionalIteratePathComparator(
Comparator optIteratePathComparator);
/**
* Depending on the depth policy, returns a new instance of
* {@link TraversePathDepthFirstIterator} or {@link TraversePathDepthLastIterator}.
*
* @return new path iterator
*
* @see #withDepthPolicy()
*/
TraversePathIterator iterator();
/**
* Returns hash code of all attributes. These are:
*
* - {@link #withRootDirPath()}
* - {@link #withDepthPolicy()}
* - {@link #withExceptionPolicy()}
* - {@link #withOptionalDescendDirPathFilter()}
* - {@link #withOptionalDescendDirPathComparator()}
* - {@link #withOptionalIteratePathFilter()}
* - {@link #withOptionalIteratePathComparator()}
*
*
* {@inheritDoc}
*/
@Override
int hashCode();
/**
* Equates by all attributes. These are:
*
* - {@link #withRootDirPath()}
* - {@link #withDepthPolicy()}
* - {@link #withExceptionPolicy()}
* - {@link #withOptionalDescendDirPathFilter()}
* - {@link #withOptionalDescendDirPathComparator()}
* - {@link #withOptionalIteratePathFilter()}
* - {@link #withOptionalIteratePathComparator()}
*
*
* {@inheritDoc}
*/
@Override
boolean equals(Object obj);
}