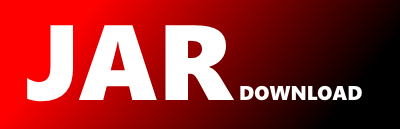
json.java.phase.LoadClassPhase Maven / Gradle / Ivy
package json.java.phase;
import java.io.IOException;
import java.lang.reflect.Method;
import java.util.regex.Matcher;
import json.java.application.AjaxApplication;
import json.java.application.AjaxContext;
import json.java.exception.LoadClassException;
import json.java.http.MutableRequest;
import json.java.http.MutableResponse;
public class LoadClassPhase extends PhaseContainer implements Phase {
public LoadClassPhase(AjaxContext context) {
super(context);
}
@Override
public void start() throws LoadClassException {
MutableRequest request = context.getRequest();
MutableResponse response = context.getResponse();
String contextPath = request.getContextPath();
String beanName = null;
Class> beanClass = null;
try {
StringBuilder script = new StringBuilder();
Matcher matcher = PATTERN_CLASS.matcher(request.getRequestURI());
if(matcher.find()) {
beanName = matcher.group(2).trim();
beanClass = AjaxApplication.ANNOTATED_CLASSES.get(beanName);
if(beanClass == null) {
throw new ClassNotFoundException();
} else {
Object obj = Class.forName(beanClass.getName(), true, this.getClass().getClassLoader()).newInstance();
Class> klass = obj.getClass();
StringBuilder json = new StringBuilder();
json.append("var " + beanName + " = {");
Method[] classMethods = klass.getDeclaredMethods();
for(int m = 0; m < classMethods.length; m++) {
Method method = classMethods[m];
if(!method.getDeclaringClass().equals(Object.class)) {
String methodName = method.getName();
Class>[] parameterTypes = method.getParameterTypes();
StringBuilder parametersName = new StringBuilder();
StringBuilder parametersType = new StringBuilder();
for(int i = 0; i < parameterTypes.length; i++) {
parametersName.append("param" + i + ((i == parameterTypes.length-1) ? "" : ", "));
parametersType.append("'" + parameterTypes[i].getCanonicalName() + "'" + ((i == parameterTypes.length-1) ? "" : ", "));
}
json.append(methodName + ": function("+ parametersName + ((parameterTypes.length > 0) ? ",": "") + " options) {");
json.append("return Loader.execute('" + beanName + "', '" + methodName + "', [" + parametersType + "], [" + parametersName + "], \""+ contextPath +"\", options);");
json.append("}");
json.append((m == classMethods.length-1) ? "" : ",");
}
}
json.append("};");
script.append(json);
response.setContentType("text/javascript");
response.getOutputStream().write(script.toString().getBytes());
}
} else {
throw new ClassNotFoundException();
}
} catch (IOException e) {
throw new LoadClassException(e);
} catch (InstantiationException e) {
throw new LoadClassException("Could not instantiate the class \""+ beanClass +"\".", e);
} catch (IllegalAccessException e) {
throw new LoadClassException("Could not instantiate the class \""+ beanClass +"\".", e);
} catch (ClassNotFoundException e) {
throw new LoadClassException("Could not find a class for the name \""+ beanName +"\".", e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy