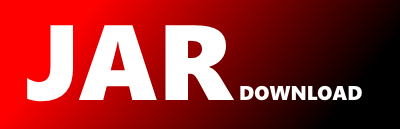
json.java.phase.PhaseContainer Maven / Gradle / Ivy
package json.java.phase;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import json.java.application.AjaxContext;
import json.java.exception.AjaxException;
import json.java.exception.PhaseException;
public class PhaseContainer {
protected static final Pattern PATTERN_ENGINE = Pattern.compile("(.*?)parser/engine/(.*?).js");
protected static final Pattern PATTERN_CLASS = Pattern.compile("(.*?)parser/script/(.*?).js");
private static final String LOAD_RESOURCE = "LOAD_RESOURCE";
private static final String LOAD_CLASS = "LOAD_CLASS";
private static final String EXECUTE = "EXECUTE";
protected AjaxContext context;
public PhaseContainer(AjaxContext context) {
this.context = context;
}
public void selectPhase() throws PhaseException {
Phase phase = null;
String typePhase = this.getPhaseName();
if(typePhase == null || typePhase.isEmpty()) {
throw new AjaxException("A type phase cannot be empty.");
}
if(typePhase == LOAD_RESOURCE) {
phase = new LoadResourcePhase(context);
}
if(typePhase == LOAD_CLASS) {
phase = new LoadClassPhase(context);
}
if(typePhase == EXECUTE) {
phase = new ExecutePhase(context);
}
if(phase == null) {
throw new AjaxException("A type phase must be declared.");
}
phase.start();
}
private String getPhaseName() {
Matcher matcherEngine = PATTERN_ENGINE.matcher(context.getRequest().getRequestURI());
Matcher matcherClass = PATTERN_CLASS.matcher(context.getRequest().getRequestURI());
if(matcherEngine.find()) {
return LOAD_RESOURCE;
} else if(matcherClass.find()) {
return LOAD_CLASS;
} else {
return EXECUTE;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy