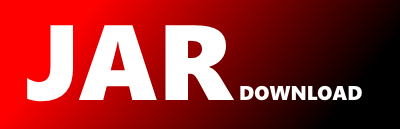
metridoc.workflows.validation.impl.HashSqlValidator.groovy Maven / Gradle / Ivy
/*
* Copyright 2010 Trustees of the University of Pennsylvania Licensed under the
* Educational Community License, Version 2.0 (the "License"); you may
* not use this file except in compliance with the License. You may
* obtain a copy of the License at
*
* http://www.osedu.org/licenses/ECL-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an "AS IS"
* BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package metridoc.workflows.validation.impl
import groovy.util.logging.Slf4j
import metridoc.utils.SystemUtils
import metridoc.workflows.validation.Validator
/**
* Created by IntelliJ IDEA.
* User: tbarker
* Date: 2/20/12
* Time: 4:40 PM
*/
@Slf4j
class HashSqlValidator implements Validator
© 2015 - 2025 Weber Informatics LLC | Privacy Policy