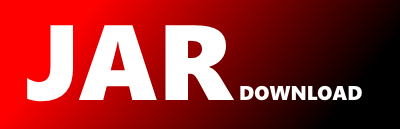
com.googlecode.mycontainer.kernel.Kernel Maven / Gradle / Ivy
/*
* Copyright 2008 Whohoo Licensed under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0 Unless required by applicable
* law or agreed to in writing, software distributed under the License is
* distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the specific
* language governing permissions and limitations under the License.
*/
package com.googlecode.mycontainer.kernel;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Hashtable;
import java.util.List;
import java.util.Map;
import javax.naming.NamingException;
import com.googlecode.mycontainer.kernel.naming.MyContainerContext;
import com.googlecode.mycontainer.kernel.naming.MyContainerContextFactory;
import com.googlecode.mycontainer.kernel.naming.ThreadLocalObjectProvider;
public class Kernel implements Serializable {
private static final long serialVersionUID = -9088221853026134701L;
private static final org.slf4j.Logger LOG = org.slf4j.LoggerFactory.getLogger(Kernel.class);
private MyContainerContext context;
private Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy