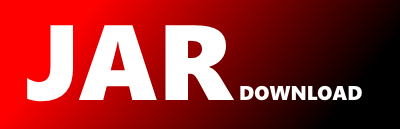
com.googlecode.mycontainer.kernel.deploy.AsyncDeployer Maven / Gradle / Ivy
package com.googlecode.mycontainer.kernel.deploy;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.Future;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.TimeoutException;
public class AsyncDeployer {
private static final org.slf4j.Logger LOGGER = org.slf4j.LoggerFactory.getLogger(AsyncDeployer.class);
private static final long TIMEOUT = 60;
private ExecutorService executorService;
public AsyncDeployer() {
this(Executors.newCachedThreadPool());
}
public AsyncDeployer(ExecutorService executorService) {
super();
this.executorService = executorService;
}
public Future deployAll(SimpleDeployer... deployers) {
final List> futures = new ArrayList>();
for (SimpleDeployer deployer : deployers) {
Future future = deploy(deployer);
futures.add(future);
}
return new CollectionFuture(futures);
}
public Future deployAll(Collection deployers) {
final List> futures = new ArrayList>();
for (SimpleDeployer deployer : deployers) {
Future future = deploy(deployer);
futures.add(future);
}
return new CollectionFuture(futures);
}
public Future getAll(Collection> futures) {
return new CollectionFuture(futures);
}
public Future getAll(Future... futures) {
return new CollectionFuture(Arrays.asList(futures));
}
@SuppressWarnings("unchecked")
public Future deploy(final SimpleDeployer deployer) {
return (Future) executorService.submit(new DeployTask(deployer));
}
public void shutdown() {
try {
this.executorService.awaitTermination(TIMEOUT, TimeUnit.SECONDS);
this.executorService.shutdown();
} catch (InterruptedException e) {
throw new RuntimeException(e);
}
}
public static class CollectionFuture implements Future {
private final Collection> futures;
private CollectionFuture(Collection> futures) {
this.futures = futures;
}
public boolean cancel(boolean mayInterruptIfRunning) {
for (Future future : futures) {
if (!future.cancel(true)) {
return false;
}
}
return true;
}
public boolean isCancelled() {
for (Future future : futures) {
if (!future.isCancelled()) {
return false;
}
}
return true;
}
public boolean isDone() {
for (Future future : futures) {
if (!future.isDone()) {
return false;
}
}
return true;
}
public Void get() throws InterruptedException, ExecutionException {
for (Future future : futures) {
future.get();
}
return null;
}
public Void get(long timeout, TimeUnit unit) throws InterruptedException, ExecutionException, TimeoutException {
for (Future future : futures) {
future.get(timeout, unit);
}
return null;
}
}
private final class DeployTask implements Runnable {
private SimpleDeployer deployer;
public DeployTask(SimpleDeployer deployer) {
super();
this.deployer = deployer;
}
public void run() {
LOGGER.info("Executing deployer " + deployer);
deployer.deploy();
LOGGER.info("Executed deployer " + deployer);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy