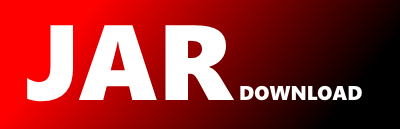
com.googlecode.mycontainer.util.ReflectionUtil Maven / Gradle / Ivy
package com.googlecode.mycontainer.util;
import java.lang.annotation.Annotation;
import java.lang.reflect.Constructor;
import java.lang.reflect.Field;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
public class ReflectionUtil {
public static void setBeanField(Object entidade, String key, Object value) {
try {
String methodName = toMethodName("set", key);
Method method = null;
if (value != null) {
method = ReflectionUtil.getMethod(entidade, methodName, value.getClass());
}
if (method == null) {
Class> type = ReflectionUtil.getBeanFieldType(entidade, key);
method = ReflectionUtil.getMethod(entidade, methodName, type);
}
if (method == null && value == null) {
throw new RuntimeException("impossible to guess type for field: " + key);
}
Class> paramType = method.getParameterTypes()[0];
if (value != null && !paramType.isAssignableFrom(value.getClass())) {
throw new RuntimeException("this method " + method + " can not be invoked with: " + value.getClass().getName());
}
method.invoke(entidade, new Object[] { value });
} catch (SecurityException e) {
throw new RuntimeException(e);
} catch (IllegalAccessException e) {
throw new RuntimeException(e);
} catch (IllegalArgumentException e) {
throw new RuntimeException(e);
} catch (InvocationTargetException e) {
throw new RuntimeException(e);
}
}
public static String toMethodName(String pre, String key) {
char c = Character.toUpperCase(key.charAt(0));
return "" + pre + c + key.substring(1);
}
public static List getBeanFields(Object obj) {
Class> clazz = obj.getClass();
return getBeanFieldsOfClass(clazz);
}
public static List getBeanFieldsOfClass(Class> clazz) {
List ret = new ArrayList();
Method[] methods = clazz.getMethods();
for (Method method : methods) {
if (method.getParameterTypes().length != 0) {
continue;
}
String name = method.getName();
if (!name.startsWith("get") || name.equals("getClass")) {
continue;
}
Class> type = method.getReturnType();
String fieldName = toBeanFieldName(name);
if (getMethodOfClass(clazz, toMethodName("set", fieldName), new Class[] { type }) != null) {
ret.add(fieldName);
}
}
return ret;
}
private static String toBeanFieldName(String name) {
char c = Character.toLowerCase(name.charAt(3));
return "" + c + name.substring(4);
}
public static Object getBeanField(Object obj, String fieldName) {
try {
String methodName = toMethodName("get", fieldName);
Method method = getMethod(obj, methodName);
return method.invoke(obj);
} catch (SecurityException e) {
throw new RuntimeException(e);
} catch (IllegalAccessException e) {
throw new RuntimeException(e);
} catch (IllegalArgumentException e) {
throw new RuntimeException(e);
} catch (InvocationTargetException e) {
throw new RuntimeException(e);
}
}
public static Method getMethod(Object obj, String methodName, Class>... types) {
Class> clazz = obj.getClass();
return getMethodOfClass(clazz, methodName, types);
}
public static Method getMethodOfClass(Class> clazz, String methodName, Class>... types) {
try {
return clazz.getMethod(methodName, types);
} catch (NoSuchMethodException e) {
return null;
} catch (SecurityException e) {
throw new RuntimeException(e);
}
}
public static boolean isBeanFieldAnnotated(Object obj, String fieldName, Class extends Annotation> annotation) {
Class> clazz = obj.getClass();
return isBeanFieldAnnotated(clazz, fieldName, annotation);
}
public static boolean isBeanFieldAnnotated(Class> clazz, String fieldName, Class extends Annotation> annotation) {
Method method = getMethodOfClass(clazz, toMethodName("get", fieldName));
if (method == null) {
return false;
}
return method.isAnnotationPresent(annotation);
}
public static Class> getBeanFieldType(Object obj, String fieldName) {
return getBeanFieldTypeOfClass(obj.getClass(), fieldName);
}
public static Class> getBeanFieldTypeOfClass(Class> clazz, String fieldName) {
Method method = getMethodOfClass(clazz, toMethodName("get", fieldName));
if (method == null) {
throw new RuntimeException("Bean field '" + fieldName + "' not found in " + clazz);
}
return method.getReturnType();
}
public static void checkBeanFieldAnnotated(Class> clazz, String fieldName, Class extends Annotation> annotation) {
if (!ReflectionUtil.isBeanFieldAnnotated(clazz, fieldName, annotation)) {
throw new IllegalStateException("Requires " + annotation + " on " + clazz.getName() + "." + fieldName);
}
}
public static List getMethods(Class> clazz, int modifier) {
Method[] methods = clazz.getMethods();
List ret = new ArrayList();
for (Method method : methods) {
int result = method.getModifiers() & modifier;
if (result > 0) {
ret.add(method);
}
}
return ret;
}
public static List getMethods(Class> clazz) {
return getMethods(clazz, 0xFFFFFFFF);
}
public static List getAnnotation(Method method) {
Annotation[] annotations = method.getAnnotations();
if (annotations == null || annotations.length == 0) {
return Collections.emptyList();
}
return Arrays.asList(annotations);
}
public static List getAnnotationField(Field field) {
Annotation[] annotations = field.getAnnotations();
if (annotations == null || annotations.length == 0) {
return Collections.emptyList();
}
return Arrays.asList(annotations);
}
public static List getAnnotationClass(Class> clazz) {
Annotation[] annotations = clazz.getAnnotations();
if (annotations == null || annotations.length == 0) {
return Collections.emptyList();
}
return Arrays.asList(annotations);
}
public static List getFields(Class> clazz) {
Field[] fields = clazz.getDeclaredFields();
List ret = new ArrayList(Arrays.asList(fields));
Class> superclass = clazz.getSuperclass();
if (superclass != null) {
ret.addAll(getFields(superclass));
}
return ret;
}
public static List getDeclaringMethods(Class> clazz) {
return getDeclaringMethods(clazz, 0xFFFFFFFF);
}
public static List getDeclaringMethods(Class> clazz, int modifier) {
Method[] methods = clazz.getDeclaredMethods();
List ret = new ArrayList();
for (Method method : methods) {
int result = method.getModifiers() & modifier;
if (result > 0) {
ret.add(method);
}
}
return ret;
}
public static Object invoke(Object o, Method m, Object... args) {
try {
return m.invoke(o, args);
} catch (IllegalAccessException e) {
throw new RuntimeException(e);
} catch (IllegalArgumentException e) {
throw new RuntimeException(e);
} catch (InvocationTargetException e) {
throw new RuntimeException(e);
}
}
@SuppressWarnings("unchecked")
public static Method getMethodByName(Class> clazz, String methodName) {
Method[] methods = clazz.getDeclaredMethods();
for (Method method : methods) {
boolean equals = method.getName().equals(methodName);
if (equals) {
return method;
}
}
Class
© 2015 - 2025 Weber Informatics LLC | Privacy Policy