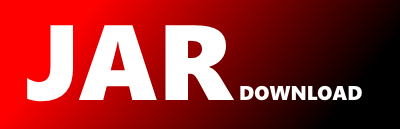
com.googlecode.paradox.ParadoxStatement Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of paradoxdriver Show documentation
Show all versions of paradoxdriver Show documentation
A Paradox Java Driver (using JDBC 4)
/*
* ParadoxStatement.java 03/14/2009 Copyright (C) 2009 Leonardo Alves da Costa This program is free software: you can
* redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software
* Foundation, either version 3 of the License, or (at your option) any later version. This program is distributed in
* the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details. You should have received a
* copy of the GNU General Public License along with this program. If not, see .
*/
package com.googlecode.paradox;
import com.googlecode.paradox.data.table.value.FieldValue;
import com.googlecode.paradox.parser.SQLParser;
import com.googlecode.paradox.parser.nodes.SelectNode;
import com.googlecode.paradox.parser.nodes.StatementNode;
import com.googlecode.paradox.planner.Planner;
import com.googlecode.paradox.planner.plan.SelectPlan;
import com.googlecode.paradox.results.Column;
import com.googlecode.paradox.utils.SQLStates;
import com.googlecode.paradox.utils.Utils;
import java.sql.Connection;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.SQLFeatureNotSupportedException;
import java.sql.SQLWarning;
import java.sql.Statement;
import java.util.ArrayList;
import java.util.List;
/**
* JDBC statement implementation.
*
* @author Leonardo Alves da Costa
* @version 1.0
* @since 1.0
*/
final class ParadoxStatement implements Statement {
/**
* The Paradox connection.
*/
private final ParadoxConnection conn;
/**
* If this statement is closed.
*/
private boolean closed;
/**
* The cursor name.
*/
private String cursorName = "NO_NAME";
/**
* The fetch direction.
*/
private int fetchDirection = ResultSet.FETCH_FORWARD;
/**
* The fetch size.
*/
private int fetchSize = 10;
/**
* The max field size.
*/
private int maxFieldSize = 255;
/**
* The max rows.
*/
private int maxRows;
/**
* If this statement is poolable.
*/
private boolean poolable;
/**
* The query timeout.
*/
private int queryTimeout = 20;
/**
* The result set associated with this statement.
*/
private ParadoxResultSet rs;
/**
* Creates a statement.
*
* @param conn the paradox connection.
*/
ParadoxStatement(final ParadoxConnection conn) {
this.conn = conn;
}
/**
* {@inheritDoc}.
*/
@Override
public void addBatch(final String sql) throws SQLException {
throw new SQLFeatureNotSupportedException();
}
/**
* {@inheritDoc}.
*/
@Override
public void cancel() {
throw new UnsupportedOperationException();
}
/**
* {@inheritDoc}.
*/
@Override
public void clearBatch() throws SQLException {
throw new SQLFeatureNotSupportedException();
}
/**
* {@inheritDoc}.
*/
@Override
public void clearWarnings() {
// Not used.
}
/**
* {@inheritDoc}.
*/
@Override
public void close() throws SQLException {
if ((this.rs != null) && !this.rs.isClosed()) {
this.rs.close();
}
this.closed = true;
}
/**
* {@inheritDoc}.
*/
@Override
public void closeOnCompletion() {
throw new UnsupportedOperationException("Not supported yet.");
}
/**
* {@inheritDoc}.
*/
@Override
public boolean execute(final String sql) throws SQLException {
if ((this.rs != null) && !this.rs.isClosed()) {
this.rs.close();
}
boolean select = false;
final SQLParser parser = new SQLParser(sql);
final List statements = parser.parse();
for (final StatementNode statement : statements) {
if (statement instanceof SelectNode) {
this.executeSelect((SelectNode) statement);
select = true;
}
}
return select;
}
/**
* {@inheritDoc}.
*/
@Override
public boolean execute(final String sql, final int autoGeneratedKeys) throws SQLException {
return this.execute(sql);
}
/**
* {@inheritDoc}.
*/
@Override
public boolean execute(final String sql, final int[] columnIndexes) throws SQLException {
return this.execute(sql);
}
/**
* {@inheritDoc}.
*/
@Override
public boolean execute(final String sql, final String[] columnNames) throws SQLException {
return this.execute(sql);
}
/**
* {@inheritDoc}.
*/
@Override
public int[] executeBatch() throws SQLException {
throw new SQLFeatureNotSupportedException();
}
/**
* {@inheritDoc}.
*/
@Override
public ResultSet executeQuery(final String sql) throws SQLException {
if ((this.rs != null) && !this.rs.isClosed()) {
this.rs.close();
}
final SQLParser parser = new SQLParser(sql);
final List statementList = parser.parse();
if (statementList.size() > 1) {
throw new SQLFeatureNotSupportedException("Unsupported operation.", SQLStates.INVALID_SQL.getValue());
}
final StatementNode node = statementList.get(0);
if (!(node instanceof SelectNode)) {
throw new SQLFeatureNotSupportedException("Not a SELECT statement.", SQLStates.INVALID_SQL.getValue());
}
this.executeSelect((SelectNode) node);
return this.rs;
}
/**
* {@inheritDoc}.
*/
@Override
public int executeUpdate(final String sql) throws SQLException {
throw new SQLFeatureNotSupportedException();
}
/**
* {@inheritDoc}.
*/
@Override
public int executeUpdate(final String sql, final int autoGeneratedKeys) {
return 0;
}
/**
* {@inheritDoc}.
*/
@Override
public int executeUpdate(final String sql, final int[] columnIndexes) {
return 0;
}
/**
* {@inheritDoc}.
*/
@Override
public int executeUpdate(final String sql, final String[] columnNames) {
return 0;
}
/**
* {@inheritDoc}.
*/
@Override
public Connection getConnection() {
return this.conn;
}
/**
* {@inheritDoc}.
*/
@Override
public int getFetchDirection() {
return this.fetchDirection;
}
/**
* {@inheritDoc}.
*/
@Override
public void setFetchDirection(final int direction) throws SQLException {
if (direction != ResultSet.FETCH_FORWARD) {
throw new SQLException("O result set somente pode ser ResultSet.FETCH_FORWARD",
SQLStates.INVALID_PARAMETER.getValue());
}
this.fetchDirection = direction;
}
/**
* {@inheritDoc}.
*/
@Override
public int getFetchSize() {
return this.fetchSize;
}
/**
* {@inheritDoc}.
*/
@Override
public void setFetchSize(final int rows) {
this.fetchSize = rows;
}
/**
* {@inheritDoc}.
*/
@Override
public ResultSet getGeneratedKeys() {
return new ParadoxResultSet(this.conn, this, new ArrayList>(), new ArrayList());
}
/**
* {@inheritDoc}.
*/
@Override
public int getMaxFieldSize() {
return this.maxFieldSize;
}
/**
* {@inheritDoc}.
*/
@Override
public void setMaxFieldSize(final int max) throws SQLException {
if (max > 255) {
throw new SQLException("Value bigger than 255.", SQLStates.INVALID_PARAMETER.getValue());
}
this.maxFieldSize = max;
}
/**
* {@inheritDoc}.
*/
@Override
public int getMaxRows() {
return this.maxRows;
}
/**
* {@inheritDoc}.
*/
@Override
public void setMaxRows(final int max) {
this.maxRows = max;
}
/**
* {@inheritDoc}.
*/
@Override
public boolean getMoreResults() {
return false;
}
/**
* {@inheritDoc}.
*/
@Override
public boolean getMoreResults(final int current) {
return false;
}
/**
* {@inheritDoc}.
*/
@Override
public int getQueryTimeout() {
return this.queryTimeout;
}
/**
* {@inheritDoc}.
*/
@Override
public void setQueryTimeout(final int seconds) {
this.queryTimeout = seconds;
}
/**
* {@inheritDoc}.
*/
@Override
public ResultSet getResultSet() {
return this.rs;
}
/**
* {@inheritDoc}.
*/
@Override
public int getResultSetConcurrency() {
return ResultSet.CONCUR_READ_ONLY;
}
/**
* {@inheritDoc}.
*/
@Override
public int getResultSetHoldability() {
return this.conn.getHoldability();
}
/**
* {@inheritDoc}.
*/
@Override
public int getResultSetType() {
return ResultSet.TYPE_FORWARD_ONLY;
}
/**
* {@inheritDoc}.
*/
@Override
public int getUpdateCount() {
return -1;
}
/**
* {@inheritDoc}.
*/
@Override
public SQLWarning getWarnings() {
return null;
}
/**
* {@inheritDoc}.
*/
@Override
public boolean isClosed() {
return this.closed;
}
/**
* {@inheritDoc}.
*/
@Override
public boolean isCloseOnCompletion() {
return true;
}
/**
* {@inheritDoc}.
*/
@Override
public boolean isPoolable() {
return this.poolable;
}
/**
* {@inheritDoc}.
*/
@Override
public void setPoolable(final boolean poolable) {
this.poolable = poolable;
}
/**
* {@inheritDoc}.
*/
@Override
public boolean isWrapperFor(final Class> iFace) {
return Utils.isWrapperFor(this, iFace);
}
/**
* {@inheritDoc}.
*/
@Override
public void setEscapeProcessing(final boolean enable) {
throw new UnsupportedOperationException();
}
/**
* {@inheritDoc}.
*/
@Override
public T unwrap(final Class iFace) throws SQLException {
return Utils.unwrap(this, iFace);
}
private void executeSelect(final SelectNode node) throws SQLException {
final Planner planner = new Planner(this.conn);
final SelectPlan plan = (SelectPlan) planner.create(node, this.conn.getCurrentSchema());
plan.execute();
this.rs = new ParadoxResultSet(this.conn, this, plan.getValues(), plan.getColumns());
}
/**
* Gets the cursor name.
*
* @return the cursor name.
*/
String getCursorName() {
return this.cursorName;
}
/**
* {@inheritDoc}.
*/
@Override
public void setCursorName(final String name) {
this.cursorName = name;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy