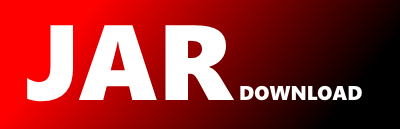
com.googlecode.placesapiclient.client.argument.ArgumentMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of places-api-client Show documentation
Show all versions of places-api-client Show documentation
Java client for Google Places service
The newest version!
package com.googlecode.placesapiclient.client.argument;
import com.googlecode.placesapiclient.client.argument.annotation.ArgumentMapping;
import com.googlecode.placesapiclient.client.argument.annotation.ArgumentMappings;
import com.googlecode.placesapiclient.client.argument.annotation.ArgumentScope;
import com.googlecode.placesapiclient.client.argument.type.RankBy;
import com.googlecode.placesapiclient.client.service.ServiceName;
/**
* Container that holds request arguments and mapping configuration.
*
* Date: 21.06.13
* Time: 08:44
*
* @author Łukasz Opaluch
*/
public class ArgumentMap {
public ArgumentMap(String apiKey) {
this.apiKey = apiKey;
}
@ArgumentMappings(mappings = {
// Search services
@ArgumentMapping(scope = ArgumentScope.REQUIRED, serviceName = ServiceName.PLACE_NEARBY_SEARCH_REQUEST, mapping = "key"),
@ArgumentMapping(scope = ArgumentScope.REQUIRED, serviceName = ServiceName.PLACE_TEXT_SEARCH_REQUEST, mapping = "key"),
@ArgumentMapping(scope = ArgumentScope.REQUIRED, serviceName = ServiceName.PLACE_RADAR_SEARCH_REQUEST, mapping = "key"),
// Details services
@ArgumentMapping(scope = ArgumentScope.REQUIRED, serviceName = ServiceName.PLACE_DETAILS_REQUEST, mapping = "key"),
// Autocomplete services
@ArgumentMapping(scope = ArgumentScope.REQUIRED, serviceName = ServiceName.PLACE_AUTOCOMPLETE_REQUEST, mapping = "key"),
@ArgumentMapping(scope = ArgumentScope.REQUIRED, serviceName = ServiceName.PLACE_QUERY_AUTOCOMPLETE_REQUEST, mapping = "key")
})
private String apiKey;
/**
* Indicates whether or not the Place request came from a device using a location sensor (e.g. a GPS) to determine
* the location sent in this request. This value must be either true or false.
*/
@ArgumentMappings(mappings = {
// Search services
@ArgumentMapping(scope = ArgumentScope.REQUIRED, serviceName = ServiceName.PLACE_NEARBY_SEARCH_REQUEST, mapping = "sensor"),
@ArgumentMapping(scope = ArgumentScope.REQUIRED, serviceName = ServiceName.PLACE_TEXT_SEARCH_REQUEST, mapping = "sensor"),
@ArgumentMapping(scope = ArgumentScope.REQUIRED, serviceName = ServiceName.PLACE_RADAR_SEARCH_REQUEST, mapping = "sensor"),
// Details services
@ArgumentMapping(scope = ArgumentScope.REQUIRED, serviceName = ServiceName.PLACE_DETAILS_REQUEST, mapping = "sensor"),
// Autocomplete services
@ArgumentMapping(scope = ArgumentScope.REQUIRED, serviceName = ServiceName.PLACE_AUTOCOMPLETE_REQUEST, mapping = "sensor"),
@ArgumentMapping(scope = ArgumentScope.REQUIRED, serviceName = ServiceName.PLACE_QUERY_AUTOCOMPLETE_REQUEST, mapping = "sensor")
})
private Boolean sensor;
/**
* The point around which you wish to retrieve Place information.
*
*/
@ArgumentMappings(mappings = {
// Search services
@ArgumentMapping(scope = ArgumentScope.REQUIRED, serviceName = ServiceName.PLACE_NEARBY_SEARCH_REQUEST, mapping = "location"),
@ArgumentMapping(scope = ArgumentScope.OPTIONAL, serviceName = ServiceName.PLACE_TEXT_SEARCH_REQUEST, mapping = "location"),
@ArgumentMapping(scope = ArgumentScope.REQUIRED, serviceName = ServiceName.PLACE_RADAR_SEARCH_REQUEST, mapping = "location"),
// Details services
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_DETAILS_REQUEST),
// Autocomplete services
@ArgumentMapping(scope = ArgumentScope.OPTIONAL, serviceName = ServiceName.PLACE_AUTOCOMPLETE_REQUEST, mapping = "location"),
@ArgumentMapping(scope = ArgumentScope.OPTIONAL, serviceName = ServiceName.PLACE_QUERY_AUTOCOMPLETE_REQUEST, mapping = "location")
})
private String location;
/**
* A term to be matched against the names of Places. Results will be restricted to those containing the passed name value.
* Note that a Place may have additional names associated with it, beyond its listed name.
* The API will try to match the passed name value against all of these names; as a result,
* Places may be returned in the results whose listed names do not match the search term, but whose associated names do.
*/
@ArgumentMappings(mappings = {
// Search services
@ArgumentMapping(scope = ArgumentScope.OPTIONAL, serviceName = ServiceName.PLACE_NEARBY_SEARCH_REQUEST, mapping = "name"),
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_TEXT_SEARCH_REQUEST),
@ArgumentMapping(scope = ArgumentScope.OPTIONAL, serviceName = ServiceName.PLACE_RADAR_SEARCH_REQUEST, mapping = "name"),
// Details services
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_DETAILS_REQUEST),
// Autocomplete services
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_AUTOCOMPLETE_REQUEST),
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_QUERY_AUTOCOMPLETE_REQUEST)
})
private String name;
/**
*
* The language in which to return results. See https://developers.google.com/maps/faq#languagesupport for the supported list of domain languages.
* If language is not supplied, the Place service will attempt to use the native language of the domain from which the request is sent.
*/
@ArgumentMappings(mappings = {
// Search services
@ArgumentMapping(scope = ArgumentScope.OPTIONAL, serviceName = ServiceName.PLACE_NEARBY_SEARCH_REQUEST, mapping = "language"),
@ArgumentMapping(scope = ArgumentScope.OPTIONAL, serviceName = ServiceName.PLACE_TEXT_SEARCH_REQUEST, mapping = "language"),
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_RADAR_SEARCH_REQUEST),
// Details services
@ArgumentMapping(scope = ArgumentScope.OPTIONAL, serviceName = ServiceName.PLACE_DETAILS_REQUEST, mapping = "language"),
// Autocomplete services
@ArgumentMapping(scope = ArgumentScope.OPTIONAL, serviceName = ServiceName.PLACE_AUTOCOMPLETE_REQUEST, mapping = "language"),
@ArgumentMapping(scope = ArgumentScope.OPTIONAL, serviceName = ServiceName.PLACE_QUERY_AUTOCOMPLETE_REQUEST, mapping = "language")
})
private String language;
/**
* Restricts results to only those places within the specified range. Valid values range between 0 (most affordable) to 4 (most expensive), inclusive.
* The exact amount indicated by a specific value will vary from region to region.
*/
@ArgumentMappings(mappings = {
// Search services
@ArgumentMapping(scope = ArgumentScope.OPTIONAL, serviceName = ServiceName.PLACE_NEARBY_SEARCH_REQUEST, mapping = "minprice"),
@ArgumentMapping(scope = ArgumentScope.OPTIONAL, serviceName = ServiceName.PLACE_TEXT_SEARCH_REQUEST, mapping = "minprice"),
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_RADAR_SEARCH_REQUEST),
// Details services
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_DETAILS_REQUEST),
// Autocomplete services
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_AUTOCOMPLETE_REQUEST),
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_QUERY_AUTOCOMPLETE_REQUEST)
})
private Double minPrice;
/**
* Restricts results to only those places within the specified range. Valid values range between 0 (most affordable) to 4 (most expensive), inclusive.
* The exact amount indicated by a specific value will vary from region to region.
*/
@ArgumentMappings(mappings = {
// Search services
@ArgumentMapping(scope = ArgumentScope.OPTIONAL, serviceName = ServiceName.PLACE_NEARBY_SEARCH_REQUEST, mapping = "maxprice"),
@ArgumentMapping(scope = ArgumentScope.OPTIONAL, serviceName = ServiceName.PLACE_TEXT_SEARCH_REQUEST, mapping = "maxprice"),
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_RADAR_SEARCH_REQUEST),
// Details services
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_DETAILS_REQUEST),
// Autocomplete services
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_AUTOCOMPLETE_REQUEST),
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_QUERY_AUTOCOMPLETE_REQUEST)
})
private Double maxPrice;
/**
* The distance (in meters) within which to return Place results. Note that setting a radius biases results to the indicated area,
* but may not fully restrict results to the specified area.
*/
@ArgumentMappings(mappings = {
// Search services
@ArgumentMapping(scope = ArgumentScope.REQUIRED, serviceName = ServiceName.PLACE_NEARBY_SEARCH_REQUEST, mapping = "radius"),
@ArgumentMapping(scope = ArgumentScope.OPTIONAL, serviceName = ServiceName.PLACE_TEXT_SEARCH_REQUEST, mapping = "radius"),
@ArgumentMapping(scope = ArgumentScope.REQUIRED, serviceName = ServiceName.PLACE_RADAR_SEARCH_REQUEST, mapping = "radius"),
// Details services
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_DETAILS_REQUEST),
// Autocomplete services
@ArgumentMapping(scope = ArgumentScope.OPTIONAL, serviceName = ServiceName.PLACE_AUTOCOMPLETE_REQUEST, mapping = "radius"),
@ArgumentMapping(scope = ArgumentScope.OPTIONAL, serviceName = ServiceName.PLACE_QUERY_AUTOCOMPLETE_REQUEST, mapping = "radius")
})
private Integer radius;
/**
*
*/
@ArgumentMappings(mappings = {
// Search services
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_NEARBY_SEARCH_REQUEST),
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_TEXT_SEARCH_REQUEST),
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_RADAR_SEARCH_REQUEST),
// Details services
@ArgumentMapping(scope = ArgumentScope.REQUIRED, serviceName = ServiceName.PLACE_DETAILS_REQUEST, mapping = "reference"),
// Autocomplete services
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_AUTOCOMPLETE_REQUEST),
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_QUERY_AUTOCOMPLETE_REQUEST)
})
private String reference;
/**
* Returns the next 20 results from a previously run search. Setting a pagetoken parameter will execute a search
* with the same parameters used previously — all parameters other than pagetoken will be ignored.
*/
@ArgumentMappings(mappings = {
// Search services
@ArgumentMapping(scope = ArgumentScope.OPTIONAL, serviceName = ServiceName.PLACE_NEARBY_SEARCH_REQUEST, mapping = "pagetoken"),
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_TEXT_SEARCH_REQUEST),
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_RADAR_SEARCH_REQUEST),
// Details services
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_DETAILS_REQUEST),
// Autocomplete services
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_AUTOCOMPLETE_REQUEST),
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_QUERY_AUTOCOMPLETE_REQUEST)
})
private String pageToken;
/**
* Returns only those Places that are open for business at the time the query is sent. Places that do not specify
* opening hours in the Google Places database will not be returned if you include this parameter in your query.
*/
@ArgumentMappings(mappings = {
// Search services
@ArgumentMapping(scope = ArgumentScope.OPTIONAL, serviceName = ServiceName.PLACE_NEARBY_SEARCH_REQUEST, mapping = "opennow"),
@ArgumentMapping(scope = ArgumentScope.OPTIONAL, serviceName = ServiceName.PLACE_TEXT_SEARCH_REQUEST, mapping = "opennow"),
@ArgumentMapping(scope = ArgumentScope.OPTIONAL, serviceName = ServiceName.PLACE_RADAR_SEARCH_REQUEST, mapping = "opennow"),
// Details services
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_DETAILS_REQUEST),
// Autocomplete services
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_AUTOCOMPLETE_REQUEST),
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_QUERY_AUTOCOMPLETE_REQUEST)
})
private Boolean openNow;
/**
* Restricts the results to Places matching at least one of the specified types.
* Types should be separated with a pipe symbol (type1|type2|etc).
* See the https://developers.google.com/places/documentation/supported_types for list of supported types.
*
*/
@ArgumentMappings(mappings = {
// Search services
@ArgumentMapping(scope = ArgumentScope.OPTIONAL, serviceName = ServiceName.PLACE_NEARBY_SEARCH_REQUEST, mapping = "types"),
@ArgumentMapping(scope = ArgumentScope.OPTIONAL, serviceName = ServiceName.PLACE_TEXT_SEARCH_REQUEST, mapping = "types"),
@ArgumentMapping(scope = ArgumentScope.OPTIONAL, serviceName = ServiceName.PLACE_RADAR_SEARCH_REQUEST, mapping = "types"),
// Details services
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_DETAILS_REQUEST),
// Autocomplete services
@ArgumentMapping(scope = ArgumentScope.OPTIONAL, serviceName = ServiceName.PLACE_AUTOCOMPLETE_REQUEST, mapping = "types"),
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_QUERY_AUTOCOMPLETE_REQUEST)
})
private String placeTypes;
/**
*
*/
@ArgumentMappings(mappings = {
// Search services
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_NEARBY_SEARCH_REQUEST),
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_TEXT_SEARCH_REQUEST),
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_RADAR_SEARCH_REQUEST),
// Details services
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_DETAILS_REQUEST),
// Autocomplete services
@ArgumentMapping(scope = ArgumentScope.REQUIRED, serviceName = ServiceName.PLACE_AUTOCOMPLETE_REQUEST, mapping = "input"),
@ArgumentMapping(scope = ArgumentScope.REQUIRED, serviceName = ServiceName.PLACE_QUERY_AUTOCOMPLETE_REQUEST, mapping = "input")
})
private String input;
/**
* The character position in the input term at which the service uses text for predictions.
* For example, if the input is 'Googl' and the completion point is 3, the service will match on 'Goo'.
* The offset should generally be set to the position of the text caret. If no offset is supplied, the service will use the entire term.
*
*/
@ArgumentMappings(mappings = {
// Search services
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_NEARBY_SEARCH_REQUEST),
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_TEXT_SEARCH_REQUEST),
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_RADAR_SEARCH_REQUEST),
// Details services
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_DETAILS_REQUEST),
// Autocomplete services
@ArgumentMapping(scope = ArgumentScope.OPTIONAL, serviceName = ServiceName.PLACE_AUTOCOMPLETE_REQUEST, mapping = "offset"),
@ArgumentMapping(scope = ArgumentScope.OPTIONAL, serviceName = ServiceName.PLACE_QUERY_AUTOCOMPLETE_REQUEST, mapping = "offset")
})
private String offset;
/**
* Specifies the order in which results are listed. Possible values are:
* - prominence (default). This option sorts results based on their importance. Ranking will favor prominent places within the specified area.
* Prominence can be affected by a Place's ranking in Google's index, the number of check-ins from your application, global popularity, and other factors.
* - distance. This option sorts results in ascending order by their distance from the specified location. Ranking results by distance will set a
* fixed search radius of 50km. One or more of keyword, name, or types is required.
*/
@ArgumentMappings(mappings = {
// Search services
@ArgumentMapping(scope = ArgumentScope.OPTIONAL, serviceName = ServiceName.PLACE_NEARBY_SEARCH_REQUEST, mapping = "rankby"),
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_TEXT_SEARCH_REQUEST),
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_RADAR_SEARCH_REQUEST),
// Details services
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_DETAILS_REQUEST),
// Autocomplete services
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_AUTOCOMPLETE_REQUEST),
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_QUERY_AUTOCOMPLETE_REQUEST)
})
private RankBy rankBy;
/**
*
*/
@ArgumentMappings(mappings = {
// Search services
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_NEARBY_SEARCH_REQUEST),
@ArgumentMapping(scope = ArgumentScope.REQUIRED, serviceName = ServiceName.PLACE_TEXT_SEARCH_REQUEST, mapping = "query"),
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_RADAR_SEARCH_REQUEST),
// Details services
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_DETAILS_REQUEST),
// Autocomplete services
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_AUTOCOMPLETE_REQUEST),
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_QUERY_AUTOCOMPLETE_REQUEST)
})
private String query;
/**
* A term to be matched against all content that Google has indexed for this Place, including but not limited to name, type, and address, as well as customer reviews and other third-party content.
*/
@ArgumentMappings(mappings = {
// Search services
@ArgumentMapping(scope = ArgumentScope.OPTIONAL, serviceName = ServiceName.PLACE_NEARBY_SEARCH_REQUEST, mapping = "keyword"),
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_TEXT_SEARCH_REQUEST),
@ArgumentMapping(scope = ArgumentScope.OPTIONAL, serviceName = ServiceName.PLACE_RADAR_SEARCH_REQUEST, mapping = "keyword"),
// Details services
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_DETAILS_REQUEST),
// Autocomplete services
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_AUTOCOMPLETE_REQUEST),
@ArgumentMapping(scope = ArgumentScope.NOT_SUPPORTED, serviceName = ServiceName.PLACE_QUERY_AUTOCOMPLETE_REQUEST)
})
private String keyword;
public String getName() {
return name;
}
public void putName(String name) {
this.name = name;
}
public Boolean getSensor() {
return sensor;
}
public String getApiKey() {
return apiKey;
}
public void putApiKey(String apiKey) {
this.apiKey = apiKey;
}
public void putSensor(Boolean sensor) {
this.sensor = sensor;
}
public String getLanguage() {
return language;
}
public void putLanguage(String language) {
this.language = language;
}
public String getLocation() {
return location;
}
public void putLocation(Double latitude, Double longitude) {
this.location = Double.toString(latitude) + "," + Double.toString(longitude);
}
public String getInput() {
return input;
}
public void putInput(String input) {
this.input = input;
}
public String getPlaceTypes() {
return placeTypes;
}
public void putPlaceTypes(String placeTypes) {
this.placeTypes = placeTypes;
}
public Boolean getOpenNow() {
return openNow;
}
public void putOpenNow(Boolean openNow) {
this.openNow = openNow;
}
public String getPageToken() {
return pageToken;
}
public void putPageToken(String pageToken) {
this.pageToken = pageToken;
}
public String getReference() {
return reference;
}
public void putReference(String reference) {
this.reference = reference;
}
public Integer getRadius() {
return radius;
}
public void putRadius(Integer radius) {
this.radius = radius;
}
public RankBy getRankBy() {
return rankBy;
}
public void putRankBy(RankBy rankBy) {
this.rankBy = rankBy;
}
public String getKeyword() {
return keyword;
}
public void putKeyword(String keyword) {
this.keyword = keyword;
}
public String getOffset() {
return offset;
}
public void putOffset(String offset) {
this.offset = offset;
}
public Double getMinPrice() {
return minPrice;
}
public void putMinPrice(Double minPrice) {
this.minPrice = minPrice;
}
public Double getMaxPrice() {
return maxPrice;
}
public void putMaxPrice(Double maxPrice) {
this.maxPrice = maxPrice;
}
public String getQuery() {
return query;
}
public void putQuery(String query) {
this.query = query;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy