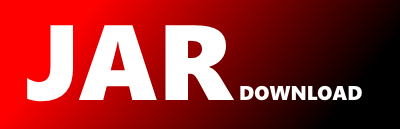
com.googlecode.placesapiclient.client.entity.Place Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of places-api-client Show documentation
Show all versions of places-api-client Show documentation
Java client for Google Places service
The newest version!
package com.googlecode.placesapiclient.client.entity;
import java.util.ArrayList;
import java.util.List;
/**
*/
public class Place {
/**
* Contains a unique stable identifier denoting this place. This identifier may not be used to retrieve information about this place,
* but can be used to consolidate data about this Place, and to verify the identity of a Place across separate searches.
* As ids can occasionally change, it's recommended that the stored id for a Place be compared with the id returned in later Details requests for the same Place, and updated if necessary.
*/
protected String id;
/**
* Contains the URL of a suggested icon which may be displayed to the user when indicating this result on a map
*/
protected String icon;
/**
* Contains the human-readable name for the returned result. For establishment results, this is usually the canonicalized business name.
*/
protected String name;
/**
* The Place's rating, from 0.0 to 5.0, based on user reviews
*/
protected Double rating;
/**
* Lists a simplified address for the Place, including the street name, street number, and locality, but not the province/state, postal code, or country.
* For example, Google's Sydney, Australia office has a vicinity value of 48 Pirrama Road, Pyrmont.
*/
protected String vicinity;
/**
* The price level of the Place, on a scale of 0 to 4. The exact amount indicated by a specific value will vary from region to region. Price levels are interpreted as follows:
* 0 — Free
* 1 — Inexpensive
* 2 — Moderate
* 3 — Expensive
* 4 — Very Expensive
*/
protected Integer priceLevel;
/**
* Contains the geocoded latitude value for this place
*/
protected Double latitude;
/**
* Contains the geocoded longitude value for this place
*/
protected Double longitude;
/**
* Contains info about opening hours for this place
*/
protected OpeningHours openingHours;
protected List typeList;
public Place() {
openingHours = new OpeningHours();
typeList = new ArrayList();
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getIcon() {
return icon;
}
public void setIcon(String icon) {
this.icon = icon;
}
public Double getLatitude() {
return latitude;
}
public void setLatitude(Double latitude) {
this.latitude = latitude;
}
public Double getLongitude() {
return longitude;
}
public void setLongitude(Double longitude) {
this.longitude = longitude;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getVicinity() {
return vicinity;
}
public void setVicinity(String vicinity) {
this.vicinity = vicinity;
}
public void setOpeningHours(OpeningHours openingHours) {
this.openingHours = openingHours;
}
public OpeningHours getOpeningHours() {
return openingHours;
}
@Override
public String toString() {
final StringBuilder sb = new StringBuilder("Place{");
sb.append("id='").append(id).append('\'');
sb.append(", icon='").append(icon).append('\'');
sb.append(", name='").append(name).append('\'');
sb.append(", rating=").append(rating);
sb.append(", vicinity='").append(vicinity).append('\'');
sb.append(", latitude=").append(latitude);
sb.append(", longitude=").append(longitude);
sb.append(", openingHours=").append(openingHours);
sb.append(", typeList=").append(typeList);
sb.append('}');
return sb.toString();
}
public Double getRating() {
return rating;
}
public void setRating(Double rating) {
this.rating = rating;
}
public List getTypeList() {
return typeList;
}
public void setTypeList(List typeList) {
this.typeList = typeList;
}
public Integer getPriceLevel() {
return priceLevel;
}
public void setPriceLevel(Integer priceLevel) {
this.priceLevel = priceLevel;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy