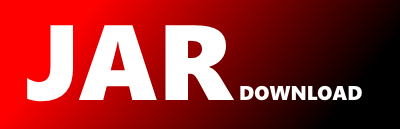
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of protobuf-rpc-pro-duplex Show documentation
Show all versions of protobuf-rpc-pro-duplex Show documentation
Protobuf RPC Pro is a java protocol buffers RPC implementation featuring bidirectional RPC calls and TCP socket connection re-use and the option of secure communications.
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: protobuf-rpc-duplex.proto
package com.googlecode.protobuf.pro.duplex.wire;
public final class DuplexProtocol {
private DuplexProtocol() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
}
public enum ConnectErrorCode
implements com.google.protobuf.ProtocolMessageEnum {
ALREADY_CONNECTED(0, 0),
;
public static final int ALREADY_CONNECTED_VALUE = 0;
public final int getNumber() { return value; }
public static ConnectErrorCode valueOf(int value) {
switch (value) {
case 0: return ALREADY_CONNECTED;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public ConnectErrorCode findValueByNumber(int number) {
return ConnectErrorCode.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.getDescriptor().getEnumTypes().get(0);
}
private static final ConnectErrorCode[] VALUES = {
ALREADY_CONNECTED,
};
public static ConnectErrorCode valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private ConnectErrorCode(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:ConnectErrorCode)
}
public interface ConnectRequestOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required int32 correlationId = 1;
boolean hasCorrelationId();
int getCorrelationId();
// required string clientHostName = 2;
boolean hasClientHostName();
String getClientHostName();
// required int32 clientPort = 3;
boolean hasClientPort();
int getClientPort();
// required string clientPID = 4;
boolean hasClientPID();
String getClientPID();
}
public static final class ConnectRequest extends
com.google.protobuf.GeneratedMessage
implements ConnectRequestOrBuilder {
// Use ConnectRequest.newBuilder() to construct.
private ConnectRequest(Builder builder) {
super(builder);
}
private ConnectRequest(boolean noInit) {}
private static final ConnectRequest defaultInstance;
public static ConnectRequest getDefaultInstance() {
return defaultInstance;
}
public ConnectRequest getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_ConnectRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_ConnectRequest_fieldAccessorTable;
}
private int bitField0_;
// required int32 correlationId = 1;
public static final int CORRELATIONID_FIELD_NUMBER = 1;
private int correlationId_;
public boolean hasCorrelationId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public int getCorrelationId() {
return correlationId_;
}
// required string clientHostName = 2;
public static final int CLIENTHOSTNAME_FIELD_NUMBER = 2;
private java.lang.Object clientHostName_;
public boolean hasClientHostName() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
public String getClientHostName() {
java.lang.Object ref = clientHostName_;
if (ref instanceof String) {
return (String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
if (com.google.protobuf.Internal.isValidUtf8(bs)) {
clientHostName_ = s;
}
return s;
}
}
private com.google.protobuf.ByteString getClientHostNameBytes() {
java.lang.Object ref = clientHostName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((String) ref);
clientHostName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// required int32 clientPort = 3;
public static final int CLIENTPORT_FIELD_NUMBER = 3;
private int clientPort_;
public boolean hasClientPort() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
public int getClientPort() {
return clientPort_;
}
// required string clientPID = 4;
public static final int CLIENTPID_FIELD_NUMBER = 4;
private java.lang.Object clientPID_;
public boolean hasClientPID() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
public String getClientPID() {
java.lang.Object ref = clientPID_;
if (ref instanceof String) {
return (String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
if (com.google.protobuf.Internal.isValidUtf8(bs)) {
clientPID_ = s;
}
return s;
}
}
private com.google.protobuf.ByteString getClientPIDBytes() {
java.lang.Object ref = clientPID_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((String) ref);
clientPID_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private void initFields() {
correlationId_ = 0;
clientHostName_ = "";
clientPort_ = 0;
clientPID_ = "";
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasCorrelationId()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasClientHostName()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasClientPort()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasClientPID()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt32(1, correlationId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getClientHostNameBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeInt32(3, clientPort_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBytes(4, getClientPIDBytes());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, correlationId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getClientHostNameBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, clientPort_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, getClientPIDBytes());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_ConnectRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_ConnectRequest_fieldAccessorTable;
}
// Construct using com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
correlationId_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
clientHostName_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
clientPort_ = 0;
bitField0_ = (bitField0_ & ~0x00000004);
clientPID_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest.getDescriptor();
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest getDefaultInstanceForType() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest.getDefaultInstance();
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest build() {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
private com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return result;
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest buildPartial() {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest result = new com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.correlationId_ = correlationId_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.clientHostName_ = clientHostName_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.clientPort_ = clientPort_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.clientPID_ = clientPID_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest) {
return mergeFrom((com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest other) {
if (other == com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest.getDefaultInstance()) return this;
if (other.hasCorrelationId()) {
setCorrelationId(other.getCorrelationId());
}
if (other.hasClientHostName()) {
setClientHostName(other.getClientHostName());
}
if (other.hasClientPort()) {
setClientPort(other.getClientPort());
}
if (other.hasClientPID()) {
setClientPID(other.getClientPID());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasCorrelationId()) {
return false;
}
if (!hasClientHostName()) {
return false;
}
if (!hasClientPort()) {
return false;
}
if (!hasClientPID()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
correlationId_ = input.readInt32();
break;
}
case 18: {
bitField0_ |= 0x00000002;
clientHostName_ = input.readBytes();
break;
}
case 24: {
bitField0_ |= 0x00000004;
clientPort_ = input.readInt32();
break;
}
case 34: {
bitField0_ |= 0x00000008;
clientPID_ = input.readBytes();
break;
}
}
}
}
private int bitField0_;
// required int32 correlationId = 1;
private int correlationId_ ;
public boolean hasCorrelationId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public int getCorrelationId() {
return correlationId_;
}
public Builder setCorrelationId(int value) {
bitField0_ |= 0x00000001;
correlationId_ = value;
onChanged();
return this;
}
public Builder clearCorrelationId() {
bitField0_ = (bitField0_ & ~0x00000001);
correlationId_ = 0;
onChanged();
return this;
}
// required string clientHostName = 2;
private java.lang.Object clientHostName_ = "";
public boolean hasClientHostName() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
public String getClientHostName() {
java.lang.Object ref = clientHostName_;
if (!(ref instanceof String)) {
String s = ((com.google.protobuf.ByteString) ref).toStringUtf8();
clientHostName_ = s;
return s;
} else {
return (String) ref;
}
}
public Builder setClientHostName(String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
clientHostName_ = value;
onChanged();
return this;
}
public Builder clearClientHostName() {
bitField0_ = (bitField0_ & ~0x00000002);
clientHostName_ = getDefaultInstance().getClientHostName();
onChanged();
return this;
}
void setClientHostName(com.google.protobuf.ByteString value) {
bitField0_ |= 0x00000002;
clientHostName_ = value;
onChanged();
}
// required int32 clientPort = 3;
private int clientPort_ ;
public boolean hasClientPort() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
public int getClientPort() {
return clientPort_;
}
public Builder setClientPort(int value) {
bitField0_ |= 0x00000004;
clientPort_ = value;
onChanged();
return this;
}
public Builder clearClientPort() {
bitField0_ = (bitField0_ & ~0x00000004);
clientPort_ = 0;
onChanged();
return this;
}
// required string clientPID = 4;
private java.lang.Object clientPID_ = "";
public boolean hasClientPID() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
public String getClientPID() {
java.lang.Object ref = clientPID_;
if (!(ref instanceof String)) {
String s = ((com.google.protobuf.ByteString) ref).toStringUtf8();
clientPID_ = s;
return s;
} else {
return (String) ref;
}
}
public Builder setClientPID(String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
clientPID_ = value;
onChanged();
return this;
}
public Builder clearClientPID() {
bitField0_ = (bitField0_ & ~0x00000008);
clientPID_ = getDefaultInstance().getClientPID();
onChanged();
return this;
}
void setClientPID(com.google.protobuf.ByteString value) {
bitField0_ |= 0x00000008;
clientPID_ = value;
onChanged();
}
// @@protoc_insertion_point(builder_scope:ConnectRequest)
}
static {
defaultInstance = new ConnectRequest(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:ConnectRequest)
}
public interface ConnectResponseOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required int32 correlationId = 1;
boolean hasCorrelationId();
int getCorrelationId();
// optional string serverPID = 2;
boolean hasServerPID();
String getServerPID();
// optional .ConnectErrorCode errorCode = 3;
boolean hasErrorCode();
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectErrorCode getErrorCode();
}
public static final class ConnectResponse extends
com.google.protobuf.GeneratedMessage
implements ConnectResponseOrBuilder {
// Use ConnectResponse.newBuilder() to construct.
private ConnectResponse(Builder builder) {
super(builder);
}
private ConnectResponse(boolean noInit) {}
private static final ConnectResponse defaultInstance;
public static ConnectResponse getDefaultInstance() {
return defaultInstance;
}
public ConnectResponse getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_ConnectResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_ConnectResponse_fieldAccessorTable;
}
private int bitField0_;
// required int32 correlationId = 1;
public static final int CORRELATIONID_FIELD_NUMBER = 1;
private int correlationId_;
public boolean hasCorrelationId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public int getCorrelationId() {
return correlationId_;
}
// optional string serverPID = 2;
public static final int SERVERPID_FIELD_NUMBER = 2;
private java.lang.Object serverPID_;
public boolean hasServerPID() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
public String getServerPID() {
java.lang.Object ref = serverPID_;
if (ref instanceof String) {
return (String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
if (com.google.protobuf.Internal.isValidUtf8(bs)) {
serverPID_ = s;
}
return s;
}
}
private com.google.protobuf.ByteString getServerPIDBytes() {
java.lang.Object ref = serverPID_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((String) ref);
serverPID_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional .ConnectErrorCode errorCode = 3;
public static final int ERRORCODE_FIELD_NUMBER = 3;
private com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectErrorCode errorCode_;
public boolean hasErrorCode() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectErrorCode getErrorCode() {
return errorCode_;
}
private void initFields() {
correlationId_ = 0;
serverPID_ = "";
errorCode_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectErrorCode.ALREADY_CONNECTED;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasCorrelationId()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt32(1, correlationId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getServerPIDBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeEnum(3, errorCode_.getNumber());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, correlationId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getServerPIDBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(3, errorCode_.getNumber());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_ConnectResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_ConnectResponse_fieldAccessorTable;
}
// Construct using com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
correlationId_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
serverPID_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
errorCode_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectErrorCode.ALREADY_CONNECTED;
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse.getDescriptor();
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse getDefaultInstanceForType() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse.getDefaultInstance();
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse build() {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
private com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return result;
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse buildPartial() {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse result = new com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.correlationId_ = correlationId_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.serverPID_ = serverPID_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.errorCode_ = errorCode_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse) {
return mergeFrom((com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse other) {
if (other == com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse.getDefaultInstance()) return this;
if (other.hasCorrelationId()) {
setCorrelationId(other.getCorrelationId());
}
if (other.hasServerPID()) {
setServerPID(other.getServerPID());
}
if (other.hasErrorCode()) {
setErrorCode(other.getErrorCode());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasCorrelationId()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
correlationId_ = input.readInt32();
break;
}
case 18: {
bitField0_ |= 0x00000002;
serverPID_ = input.readBytes();
break;
}
case 24: {
int rawValue = input.readEnum();
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectErrorCode value = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectErrorCode.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(3, rawValue);
} else {
bitField0_ |= 0x00000004;
errorCode_ = value;
}
break;
}
}
}
}
private int bitField0_;
// required int32 correlationId = 1;
private int correlationId_ ;
public boolean hasCorrelationId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public int getCorrelationId() {
return correlationId_;
}
public Builder setCorrelationId(int value) {
bitField0_ |= 0x00000001;
correlationId_ = value;
onChanged();
return this;
}
public Builder clearCorrelationId() {
bitField0_ = (bitField0_ & ~0x00000001);
correlationId_ = 0;
onChanged();
return this;
}
// optional string serverPID = 2;
private java.lang.Object serverPID_ = "";
public boolean hasServerPID() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
public String getServerPID() {
java.lang.Object ref = serverPID_;
if (!(ref instanceof String)) {
String s = ((com.google.protobuf.ByteString) ref).toStringUtf8();
serverPID_ = s;
return s;
} else {
return (String) ref;
}
}
public Builder setServerPID(String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
serverPID_ = value;
onChanged();
return this;
}
public Builder clearServerPID() {
bitField0_ = (bitField0_ & ~0x00000002);
serverPID_ = getDefaultInstance().getServerPID();
onChanged();
return this;
}
void setServerPID(com.google.protobuf.ByteString value) {
bitField0_ |= 0x00000002;
serverPID_ = value;
onChanged();
}
// optional .ConnectErrorCode errorCode = 3;
private com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectErrorCode errorCode_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectErrorCode.ALREADY_CONNECTED;
public boolean hasErrorCode() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectErrorCode getErrorCode() {
return errorCode_;
}
public Builder setErrorCode(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectErrorCode value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
errorCode_ = value;
onChanged();
return this;
}
public Builder clearErrorCode() {
bitField0_ = (bitField0_ & ~0x00000004);
errorCode_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectErrorCode.ALREADY_CONNECTED;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:ConnectResponse)
}
static {
defaultInstance = new ConnectResponse(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:ConnectResponse)
}
public interface RpcRequestOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required int32 correlationId = 1;
boolean hasCorrelationId();
int getCorrelationId();
// required string serviceIdentifier = 2;
boolean hasServiceIdentifier();
String getServiceIdentifier();
// required string methodIdentifier = 3;
boolean hasMethodIdentifier();
String getMethodIdentifier();
// required bytes requestBytes = 4;
boolean hasRequestBytes();
com.google.protobuf.ByteString getRequestBytes();
}
public static final class RpcRequest extends
com.google.protobuf.GeneratedMessage
implements RpcRequestOrBuilder {
// Use RpcRequest.newBuilder() to construct.
private RpcRequest(Builder builder) {
super(builder);
}
private RpcRequest(boolean noInit) {}
private static final RpcRequest defaultInstance;
public static RpcRequest getDefaultInstance() {
return defaultInstance;
}
public RpcRequest getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_RpcRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_RpcRequest_fieldAccessorTable;
}
private int bitField0_;
// required int32 correlationId = 1;
public static final int CORRELATIONID_FIELD_NUMBER = 1;
private int correlationId_;
public boolean hasCorrelationId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public int getCorrelationId() {
return correlationId_;
}
// required string serviceIdentifier = 2;
public static final int SERVICEIDENTIFIER_FIELD_NUMBER = 2;
private java.lang.Object serviceIdentifier_;
public boolean hasServiceIdentifier() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
public String getServiceIdentifier() {
java.lang.Object ref = serviceIdentifier_;
if (ref instanceof String) {
return (String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
if (com.google.protobuf.Internal.isValidUtf8(bs)) {
serviceIdentifier_ = s;
}
return s;
}
}
private com.google.protobuf.ByteString getServiceIdentifierBytes() {
java.lang.Object ref = serviceIdentifier_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((String) ref);
serviceIdentifier_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// required string methodIdentifier = 3;
public static final int METHODIDENTIFIER_FIELD_NUMBER = 3;
private java.lang.Object methodIdentifier_;
public boolean hasMethodIdentifier() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
public String getMethodIdentifier() {
java.lang.Object ref = methodIdentifier_;
if (ref instanceof String) {
return (String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
if (com.google.protobuf.Internal.isValidUtf8(bs)) {
methodIdentifier_ = s;
}
return s;
}
}
private com.google.protobuf.ByteString getMethodIdentifierBytes() {
java.lang.Object ref = methodIdentifier_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((String) ref);
methodIdentifier_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// required bytes requestBytes = 4;
public static final int REQUESTBYTES_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString requestBytes_;
public boolean hasRequestBytes() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
public com.google.protobuf.ByteString getRequestBytes() {
return requestBytes_;
}
private void initFields() {
correlationId_ = 0;
serviceIdentifier_ = "";
methodIdentifier_ = "";
requestBytes_ = com.google.protobuf.ByteString.EMPTY;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasCorrelationId()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasServiceIdentifier()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasMethodIdentifier()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasRequestBytes()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt32(1, correlationId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getServiceIdentifierBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBytes(3, getMethodIdentifierBytes());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBytes(4, requestBytes_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, correlationId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getServiceIdentifierBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, getMethodIdentifierBytes());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, requestBytes_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_RpcRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_RpcRequest_fieldAccessorTable;
}
// Construct using com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
correlationId_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
serviceIdentifier_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
methodIdentifier_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
requestBytes_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest.getDescriptor();
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest getDefaultInstanceForType() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest.getDefaultInstance();
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest build() {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
private com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return result;
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest buildPartial() {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest result = new com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.correlationId_ = correlationId_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.serviceIdentifier_ = serviceIdentifier_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.methodIdentifier_ = methodIdentifier_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.requestBytes_ = requestBytes_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest) {
return mergeFrom((com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest other) {
if (other == com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest.getDefaultInstance()) return this;
if (other.hasCorrelationId()) {
setCorrelationId(other.getCorrelationId());
}
if (other.hasServiceIdentifier()) {
setServiceIdentifier(other.getServiceIdentifier());
}
if (other.hasMethodIdentifier()) {
setMethodIdentifier(other.getMethodIdentifier());
}
if (other.hasRequestBytes()) {
setRequestBytes(other.getRequestBytes());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasCorrelationId()) {
return false;
}
if (!hasServiceIdentifier()) {
return false;
}
if (!hasMethodIdentifier()) {
return false;
}
if (!hasRequestBytes()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
correlationId_ = input.readInt32();
break;
}
case 18: {
bitField0_ |= 0x00000002;
serviceIdentifier_ = input.readBytes();
break;
}
case 26: {
bitField0_ |= 0x00000004;
methodIdentifier_ = input.readBytes();
break;
}
case 34: {
bitField0_ |= 0x00000008;
requestBytes_ = input.readBytes();
break;
}
}
}
}
private int bitField0_;
// required int32 correlationId = 1;
private int correlationId_ ;
public boolean hasCorrelationId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public int getCorrelationId() {
return correlationId_;
}
public Builder setCorrelationId(int value) {
bitField0_ |= 0x00000001;
correlationId_ = value;
onChanged();
return this;
}
public Builder clearCorrelationId() {
bitField0_ = (bitField0_ & ~0x00000001);
correlationId_ = 0;
onChanged();
return this;
}
// required string serviceIdentifier = 2;
private java.lang.Object serviceIdentifier_ = "";
public boolean hasServiceIdentifier() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
public String getServiceIdentifier() {
java.lang.Object ref = serviceIdentifier_;
if (!(ref instanceof String)) {
String s = ((com.google.protobuf.ByteString) ref).toStringUtf8();
serviceIdentifier_ = s;
return s;
} else {
return (String) ref;
}
}
public Builder setServiceIdentifier(String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
serviceIdentifier_ = value;
onChanged();
return this;
}
public Builder clearServiceIdentifier() {
bitField0_ = (bitField0_ & ~0x00000002);
serviceIdentifier_ = getDefaultInstance().getServiceIdentifier();
onChanged();
return this;
}
void setServiceIdentifier(com.google.protobuf.ByteString value) {
bitField0_ |= 0x00000002;
serviceIdentifier_ = value;
onChanged();
}
// required string methodIdentifier = 3;
private java.lang.Object methodIdentifier_ = "";
public boolean hasMethodIdentifier() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
public String getMethodIdentifier() {
java.lang.Object ref = methodIdentifier_;
if (!(ref instanceof String)) {
String s = ((com.google.protobuf.ByteString) ref).toStringUtf8();
methodIdentifier_ = s;
return s;
} else {
return (String) ref;
}
}
public Builder setMethodIdentifier(String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
methodIdentifier_ = value;
onChanged();
return this;
}
public Builder clearMethodIdentifier() {
bitField0_ = (bitField0_ & ~0x00000004);
methodIdentifier_ = getDefaultInstance().getMethodIdentifier();
onChanged();
return this;
}
void setMethodIdentifier(com.google.protobuf.ByteString value) {
bitField0_ |= 0x00000004;
methodIdentifier_ = value;
onChanged();
}
// required bytes requestBytes = 4;
private com.google.protobuf.ByteString requestBytes_ = com.google.protobuf.ByteString.EMPTY;
public boolean hasRequestBytes() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
public com.google.protobuf.ByteString getRequestBytes() {
return requestBytes_;
}
public Builder setRequestBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
requestBytes_ = value;
onChanged();
return this;
}
public Builder clearRequestBytes() {
bitField0_ = (bitField0_ & ~0x00000008);
requestBytes_ = getDefaultInstance().getRequestBytes();
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:RpcRequest)
}
static {
defaultInstance = new RpcRequest(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:RpcRequest)
}
public interface RpcResponseOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required int32 correlationId = 1;
boolean hasCorrelationId();
int getCorrelationId();
// required bytes responseBytes = 2;
boolean hasResponseBytes();
com.google.protobuf.ByteString getResponseBytes();
}
public static final class RpcResponse extends
com.google.protobuf.GeneratedMessage
implements RpcResponseOrBuilder {
// Use RpcResponse.newBuilder() to construct.
private RpcResponse(Builder builder) {
super(builder);
}
private RpcResponse(boolean noInit) {}
private static final RpcResponse defaultInstance;
public static RpcResponse getDefaultInstance() {
return defaultInstance;
}
public RpcResponse getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_RpcResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_RpcResponse_fieldAccessorTable;
}
private int bitField0_;
// required int32 correlationId = 1;
public static final int CORRELATIONID_FIELD_NUMBER = 1;
private int correlationId_;
public boolean hasCorrelationId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public int getCorrelationId() {
return correlationId_;
}
// required bytes responseBytes = 2;
public static final int RESPONSEBYTES_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString responseBytes_;
public boolean hasResponseBytes() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
public com.google.protobuf.ByteString getResponseBytes() {
return responseBytes_;
}
private void initFields() {
correlationId_ = 0;
responseBytes_ = com.google.protobuf.ByteString.EMPTY;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasCorrelationId()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasResponseBytes()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt32(1, correlationId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, responseBytes_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, correlationId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, responseBytes_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_RpcResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_RpcResponse_fieldAccessorTable;
}
// Construct using com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
correlationId_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
responseBytes_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse.getDescriptor();
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse getDefaultInstanceForType() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse.getDefaultInstance();
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse build() {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
private com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return result;
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse buildPartial() {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse result = new com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.correlationId_ = correlationId_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.responseBytes_ = responseBytes_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse) {
return mergeFrom((com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse other) {
if (other == com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse.getDefaultInstance()) return this;
if (other.hasCorrelationId()) {
setCorrelationId(other.getCorrelationId());
}
if (other.hasResponseBytes()) {
setResponseBytes(other.getResponseBytes());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasCorrelationId()) {
return false;
}
if (!hasResponseBytes()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
correlationId_ = input.readInt32();
break;
}
case 18: {
bitField0_ |= 0x00000002;
responseBytes_ = input.readBytes();
break;
}
}
}
}
private int bitField0_;
// required int32 correlationId = 1;
private int correlationId_ ;
public boolean hasCorrelationId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public int getCorrelationId() {
return correlationId_;
}
public Builder setCorrelationId(int value) {
bitField0_ |= 0x00000001;
correlationId_ = value;
onChanged();
return this;
}
public Builder clearCorrelationId() {
bitField0_ = (bitField0_ & ~0x00000001);
correlationId_ = 0;
onChanged();
return this;
}
// required bytes responseBytes = 2;
private com.google.protobuf.ByteString responseBytes_ = com.google.protobuf.ByteString.EMPTY;
public boolean hasResponseBytes() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
public com.google.protobuf.ByteString getResponseBytes() {
return responseBytes_;
}
public Builder setResponseBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
responseBytes_ = value;
onChanged();
return this;
}
public Builder clearResponseBytes() {
bitField0_ = (bitField0_ & ~0x00000002);
responseBytes_ = getDefaultInstance().getResponseBytes();
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:RpcResponse)
}
static {
defaultInstance = new RpcResponse(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:RpcResponse)
}
public interface RpcErrorOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required int32 correlationId = 1;
boolean hasCorrelationId();
int getCorrelationId();
// required string errorMessage = 2;
boolean hasErrorMessage();
String getErrorMessage();
}
public static final class RpcError extends
com.google.protobuf.GeneratedMessage
implements RpcErrorOrBuilder {
// Use RpcError.newBuilder() to construct.
private RpcError(Builder builder) {
super(builder);
}
private RpcError(boolean noInit) {}
private static final RpcError defaultInstance;
public static RpcError getDefaultInstance() {
return defaultInstance;
}
public RpcError getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_RpcError_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_RpcError_fieldAccessorTable;
}
private int bitField0_;
// required int32 correlationId = 1;
public static final int CORRELATIONID_FIELD_NUMBER = 1;
private int correlationId_;
public boolean hasCorrelationId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public int getCorrelationId() {
return correlationId_;
}
// required string errorMessage = 2;
public static final int ERRORMESSAGE_FIELD_NUMBER = 2;
private java.lang.Object errorMessage_;
public boolean hasErrorMessage() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
public String getErrorMessage() {
java.lang.Object ref = errorMessage_;
if (ref instanceof String) {
return (String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
if (com.google.protobuf.Internal.isValidUtf8(bs)) {
errorMessage_ = s;
}
return s;
}
}
private com.google.protobuf.ByteString getErrorMessageBytes() {
java.lang.Object ref = errorMessage_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((String) ref);
errorMessage_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private void initFields() {
correlationId_ = 0;
errorMessage_ = "";
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasCorrelationId()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasErrorMessage()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt32(1, correlationId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getErrorMessageBytes());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, correlationId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getErrorMessageBytes());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcErrorOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_RpcError_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_RpcError_fieldAccessorTable;
}
// Construct using com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
correlationId_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
errorMessage_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError.getDescriptor();
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError getDefaultInstanceForType() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError.getDefaultInstance();
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError build() {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
private com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return result;
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError buildPartial() {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError result = new com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.correlationId_ = correlationId_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.errorMessage_ = errorMessage_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError) {
return mergeFrom((com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError other) {
if (other == com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError.getDefaultInstance()) return this;
if (other.hasCorrelationId()) {
setCorrelationId(other.getCorrelationId());
}
if (other.hasErrorMessage()) {
setErrorMessage(other.getErrorMessage());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasCorrelationId()) {
return false;
}
if (!hasErrorMessage()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
correlationId_ = input.readInt32();
break;
}
case 18: {
bitField0_ |= 0x00000002;
errorMessage_ = input.readBytes();
break;
}
}
}
}
private int bitField0_;
// required int32 correlationId = 1;
private int correlationId_ ;
public boolean hasCorrelationId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public int getCorrelationId() {
return correlationId_;
}
public Builder setCorrelationId(int value) {
bitField0_ |= 0x00000001;
correlationId_ = value;
onChanged();
return this;
}
public Builder clearCorrelationId() {
bitField0_ = (bitField0_ & ~0x00000001);
correlationId_ = 0;
onChanged();
return this;
}
// required string errorMessage = 2;
private java.lang.Object errorMessage_ = "";
public boolean hasErrorMessage() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
public String getErrorMessage() {
java.lang.Object ref = errorMessage_;
if (!(ref instanceof String)) {
String s = ((com.google.protobuf.ByteString) ref).toStringUtf8();
errorMessage_ = s;
return s;
} else {
return (String) ref;
}
}
public Builder setErrorMessage(String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
errorMessage_ = value;
onChanged();
return this;
}
public Builder clearErrorMessage() {
bitField0_ = (bitField0_ & ~0x00000002);
errorMessage_ = getDefaultInstance().getErrorMessage();
onChanged();
return this;
}
void setErrorMessage(com.google.protobuf.ByteString value) {
bitField0_ |= 0x00000002;
errorMessage_ = value;
onChanged();
}
// @@protoc_insertion_point(builder_scope:RpcError)
}
static {
defaultInstance = new RpcError(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:RpcError)
}
public interface RpcCancelOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required int32 correlationId = 1;
boolean hasCorrelationId();
int getCorrelationId();
}
public static final class RpcCancel extends
com.google.protobuf.GeneratedMessage
implements RpcCancelOrBuilder {
// Use RpcCancel.newBuilder() to construct.
private RpcCancel(Builder builder) {
super(builder);
}
private RpcCancel(boolean noInit) {}
private static final RpcCancel defaultInstance;
public static RpcCancel getDefaultInstance() {
return defaultInstance;
}
public RpcCancel getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_RpcCancel_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_RpcCancel_fieldAccessorTable;
}
private int bitField0_;
// required int32 correlationId = 1;
public static final int CORRELATIONID_FIELD_NUMBER = 1;
private int correlationId_;
public boolean hasCorrelationId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public int getCorrelationId() {
return correlationId_;
}
private void initFields() {
correlationId_ = 0;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasCorrelationId()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt32(1, correlationId_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, correlationId_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancelOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_RpcCancel_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_RpcCancel_fieldAccessorTable;
}
// Construct using com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
correlationId_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel.getDescriptor();
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel getDefaultInstanceForType() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel.getDefaultInstance();
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel build() {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
private com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return result;
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel buildPartial() {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel result = new com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.correlationId_ = correlationId_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel) {
return mergeFrom((com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel other) {
if (other == com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel.getDefaultInstance()) return this;
if (other.hasCorrelationId()) {
setCorrelationId(other.getCorrelationId());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasCorrelationId()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
correlationId_ = input.readInt32();
break;
}
}
}
}
private int bitField0_;
// required int32 correlationId = 1;
private int correlationId_ ;
public boolean hasCorrelationId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public int getCorrelationId() {
return correlationId_;
}
public Builder setCorrelationId(int value) {
bitField0_ |= 0x00000001;
correlationId_ = value;
onChanged();
return this;
}
public Builder clearCorrelationId() {
bitField0_ = (bitField0_ & ~0x00000001);
correlationId_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:RpcCancel)
}
static {
defaultInstance = new RpcCancel(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:RpcCancel)
}
public interface WirePayloadOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional .ConnectRequest connectRequest = 1;
boolean hasConnectRequest();
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest getConnectRequest();
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequestOrBuilder getConnectRequestOrBuilder();
// optional .ConnectResponse connectResponse = 2;
boolean hasConnectResponse();
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse getConnectResponse();
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponseOrBuilder getConnectResponseOrBuilder();
// optional .RpcRequest rpcRequest = 3;
boolean hasRpcRequest();
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest getRpcRequest();
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequestOrBuilder getRpcRequestOrBuilder();
// optional .RpcResponse rpcResponse = 4;
boolean hasRpcResponse();
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse getRpcResponse();
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponseOrBuilder getRpcResponseOrBuilder();
// optional .RpcError rpcError = 5;
boolean hasRpcError();
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError getRpcError();
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcErrorOrBuilder getRpcErrorOrBuilder();
// optional .RpcCancel rpcCancel = 6;
boolean hasRpcCancel();
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel getRpcCancel();
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancelOrBuilder getRpcCancelOrBuilder();
}
public static final class WirePayload extends
com.google.protobuf.GeneratedMessage
implements WirePayloadOrBuilder {
// Use WirePayload.newBuilder() to construct.
private WirePayload(Builder builder) {
super(builder);
}
private WirePayload(boolean noInit) {}
private static final WirePayload defaultInstance;
public static WirePayload getDefaultInstance() {
return defaultInstance;
}
public WirePayload getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_WirePayload_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_WirePayload_fieldAccessorTable;
}
private int bitField0_;
// optional .ConnectRequest connectRequest = 1;
public static final int CONNECTREQUEST_FIELD_NUMBER = 1;
private com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest connectRequest_;
public boolean hasConnectRequest() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest getConnectRequest() {
return connectRequest_;
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequestOrBuilder getConnectRequestOrBuilder() {
return connectRequest_;
}
// optional .ConnectResponse connectResponse = 2;
public static final int CONNECTRESPONSE_FIELD_NUMBER = 2;
private com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse connectResponse_;
public boolean hasConnectResponse() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse getConnectResponse() {
return connectResponse_;
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponseOrBuilder getConnectResponseOrBuilder() {
return connectResponse_;
}
// optional .RpcRequest rpcRequest = 3;
public static final int RPCREQUEST_FIELD_NUMBER = 3;
private com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest rpcRequest_;
public boolean hasRpcRequest() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest getRpcRequest() {
return rpcRequest_;
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequestOrBuilder getRpcRequestOrBuilder() {
return rpcRequest_;
}
// optional .RpcResponse rpcResponse = 4;
public static final int RPCRESPONSE_FIELD_NUMBER = 4;
private com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse rpcResponse_;
public boolean hasRpcResponse() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse getRpcResponse() {
return rpcResponse_;
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponseOrBuilder getRpcResponseOrBuilder() {
return rpcResponse_;
}
// optional .RpcError rpcError = 5;
public static final int RPCERROR_FIELD_NUMBER = 5;
private com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError rpcError_;
public boolean hasRpcError() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError getRpcError() {
return rpcError_;
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcErrorOrBuilder getRpcErrorOrBuilder() {
return rpcError_;
}
// optional .RpcCancel rpcCancel = 6;
public static final int RPCCANCEL_FIELD_NUMBER = 6;
private com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel rpcCancel_;
public boolean hasRpcCancel() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel getRpcCancel() {
return rpcCancel_;
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancelOrBuilder getRpcCancelOrBuilder() {
return rpcCancel_;
}
private void initFields() {
connectRequest_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest.getDefaultInstance();
connectResponse_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse.getDefaultInstance();
rpcRequest_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest.getDefaultInstance();
rpcResponse_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse.getDefaultInstance();
rpcError_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError.getDefaultInstance();
rpcCancel_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (hasConnectRequest()) {
if (!getConnectRequest().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasConnectResponse()) {
if (!getConnectResponse().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasRpcRequest()) {
if (!getRpcRequest().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasRpcResponse()) {
if (!getRpcResponse().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasRpcError()) {
if (!getRpcError().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasRpcCancel()) {
if (!getRpcCancel().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, connectRequest_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeMessage(2, connectResponse_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeMessage(3, rpcRequest_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeMessage(4, rpcResponse_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeMessage(5, rpcError_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeMessage(6, rpcCancel_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, connectRequest_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, connectResponse_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, rpcRequest_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, rpcResponse_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, rpcError_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, rpcCancel_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayloadOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_WirePayload_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_WirePayload_fieldAccessorTable;
}
// Construct using com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getConnectRequestFieldBuilder();
getConnectResponseFieldBuilder();
getRpcRequestFieldBuilder();
getRpcResponseFieldBuilder();
getRpcErrorFieldBuilder();
getRpcCancelFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (connectRequestBuilder_ == null) {
connectRequest_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest.getDefaultInstance();
} else {
connectRequestBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
if (connectResponseBuilder_ == null) {
connectResponse_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse.getDefaultInstance();
} else {
connectResponseBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
if (rpcRequestBuilder_ == null) {
rpcRequest_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest.getDefaultInstance();
} else {
rpcRequestBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
if (rpcResponseBuilder_ == null) {
rpcResponse_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse.getDefaultInstance();
} else {
rpcResponseBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
if (rpcErrorBuilder_ == null) {
rpcError_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError.getDefaultInstance();
} else {
rpcErrorBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000010);
if (rpcCancelBuilder_ == null) {
rpcCancel_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel.getDefaultInstance();
} else {
rpcCancelBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000020);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload.getDescriptor();
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload getDefaultInstanceForType() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload.getDefaultInstance();
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload build() {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
private com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return result;
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload buildPartial() {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload result = new com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
if (connectRequestBuilder_ == null) {
result.connectRequest_ = connectRequest_;
} else {
result.connectRequest_ = connectRequestBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
if (connectResponseBuilder_ == null) {
result.connectResponse_ = connectResponse_;
} else {
result.connectResponse_ = connectResponseBuilder_.build();
}
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
if (rpcRequestBuilder_ == null) {
result.rpcRequest_ = rpcRequest_;
} else {
result.rpcRequest_ = rpcRequestBuilder_.build();
}
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
if (rpcResponseBuilder_ == null) {
result.rpcResponse_ = rpcResponse_;
} else {
result.rpcResponse_ = rpcResponseBuilder_.build();
}
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
if (rpcErrorBuilder_ == null) {
result.rpcError_ = rpcError_;
} else {
result.rpcError_ = rpcErrorBuilder_.build();
}
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
if (rpcCancelBuilder_ == null) {
result.rpcCancel_ = rpcCancel_;
} else {
result.rpcCancel_ = rpcCancelBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload) {
return mergeFrom((com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload other) {
if (other == com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload.getDefaultInstance()) return this;
if (other.hasConnectRequest()) {
mergeConnectRequest(other.getConnectRequest());
}
if (other.hasConnectResponse()) {
mergeConnectResponse(other.getConnectResponse());
}
if (other.hasRpcRequest()) {
mergeRpcRequest(other.getRpcRequest());
}
if (other.hasRpcResponse()) {
mergeRpcResponse(other.getRpcResponse());
}
if (other.hasRpcError()) {
mergeRpcError(other.getRpcError());
}
if (other.hasRpcCancel()) {
mergeRpcCancel(other.getRpcCancel());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (hasConnectRequest()) {
if (!getConnectRequest().isInitialized()) {
return false;
}
}
if (hasConnectResponse()) {
if (!getConnectResponse().isInitialized()) {
return false;
}
}
if (hasRpcRequest()) {
if (!getRpcRequest().isInitialized()) {
return false;
}
}
if (hasRpcResponse()) {
if (!getRpcResponse().isInitialized()) {
return false;
}
}
if (hasRpcError()) {
if (!getRpcError().isInitialized()) {
return false;
}
}
if (hasRpcCancel()) {
if (!getRpcCancel().isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
}
break;
}
case 10: {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest.Builder subBuilder = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest.newBuilder();
if (hasConnectRequest()) {
subBuilder.mergeFrom(getConnectRequest());
}
input.readMessage(subBuilder, extensionRegistry);
setConnectRequest(subBuilder.buildPartial());
break;
}
case 18: {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse.Builder subBuilder = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse.newBuilder();
if (hasConnectResponse()) {
subBuilder.mergeFrom(getConnectResponse());
}
input.readMessage(subBuilder, extensionRegistry);
setConnectResponse(subBuilder.buildPartial());
break;
}
case 26: {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest.Builder subBuilder = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest.newBuilder();
if (hasRpcRequest()) {
subBuilder.mergeFrom(getRpcRequest());
}
input.readMessage(subBuilder, extensionRegistry);
setRpcRequest(subBuilder.buildPartial());
break;
}
case 34: {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse.Builder subBuilder = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse.newBuilder();
if (hasRpcResponse()) {
subBuilder.mergeFrom(getRpcResponse());
}
input.readMessage(subBuilder, extensionRegistry);
setRpcResponse(subBuilder.buildPartial());
break;
}
case 42: {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError.Builder subBuilder = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError.newBuilder();
if (hasRpcError()) {
subBuilder.mergeFrom(getRpcError());
}
input.readMessage(subBuilder, extensionRegistry);
setRpcError(subBuilder.buildPartial());
break;
}
case 50: {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel.Builder subBuilder = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel.newBuilder();
if (hasRpcCancel()) {
subBuilder.mergeFrom(getRpcCancel());
}
input.readMessage(subBuilder, extensionRegistry);
setRpcCancel(subBuilder.buildPartial());
break;
}
}
}
}
private int bitField0_;
// optional .ConnectRequest connectRequest = 1;
private com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest connectRequest_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequestOrBuilder> connectRequestBuilder_;
public boolean hasConnectRequest() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest getConnectRequest() {
if (connectRequestBuilder_ == null) {
return connectRequest_;
} else {
return connectRequestBuilder_.getMessage();
}
}
public Builder setConnectRequest(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest value) {
if (connectRequestBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
connectRequest_ = value;
onChanged();
} else {
connectRequestBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
return this;
}
public Builder setConnectRequest(
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest.Builder builderForValue) {
if (connectRequestBuilder_ == null) {
connectRequest_ = builderForValue.build();
onChanged();
} else {
connectRequestBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
return this;
}
public Builder mergeConnectRequest(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest value) {
if (connectRequestBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
connectRequest_ != com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest.getDefaultInstance()) {
connectRequest_ =
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest.newBuilder(connectRequest_).mergeFrom(value).buildPartial();
} else {
connectRequest_ = value;
}
onChanged();
} else {
connectRequestBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
return this;
}
public Builder clearConnectRequest() {
if (connectRequestBuilder_ == null) {
connectRequest_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest.getDefaultInstance();
onChanged();
} else {
connectRequestBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest.Builder getConnectRequestBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getConnectRequestFieldBuilder().getBuilder();
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequestOrBuilder getConnectRequestOrBuilder() {
if (connectRequestBuilder_ != null) {
return connectRequestBuilder_.getMessageOrBuilder();
} else {
return connectRequest_;
}
}
private com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequestOrBuilder>
getConnectRequestFieldBuilder() {
if (connectRequestBuilder_ == null) {
connectRequestBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequestOrBuilder>(
connectRequest_,
getParentForChildren(),
isClean());
connectRequest_ = null;
}
return connectRequestBuilder_;
}
// optional .ConnectResponse connectResponse = 2;
private com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse connectResponse_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponseOrBuilder> connectResponseBuilder_;
public boolean hasConnectResponse() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse getConnectResponse() {
if (connectResponseBuilder_ == null) {
return connectResponse_;
} else {
return connectResponseBuilder_.getMessage();
}
}
public Builder setConnectResponse(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse value) {
if (connectResponseBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
connectResponse_ = value;
onChanged();
} else {
connectResponseBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
return this;
}
public Builder setConnectResponse(
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse.Builder builderForValue) {
if (connectResponseBuilder_ == null) {
connectResponse_ = builderForValue.build();
onChanged();
} else {
connectResponseBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
return this;
}
public Builder mergeConnectResponse(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse value) {
if (connectResponseBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002) &&
connectResponse_ != com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse.getDefaultInstance()) {
connectResponse_ =
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse.newBuilder(connectResponse_).mergeFrom(value).buildPartial();
} else {
connectResponse_ = value;
}
onChanged();
} else {
connectResponseBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
return this;
}
public Builder clearConnectResponse() {
if (connectResponseBuilder_ == null) {
connectResponse_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse.getDefaultInstance();
onChanged();
} else {
connectResponseBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse.Builder getConnectResponseBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getConnectResponseFieldBuilder().getBuilder();
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponseOrBuilder getConnectResponseOrBuilder() {
if (connectResponseBuilder_ != null) {
return connectResponseBuilder_.getMessageOrBuilder();
} else {
return connectResponse_;
}
}
private com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponseOrBuilder>
getConnectResponseFieldBuilder() {
if (connectResponseBuilder_ == null) {
connectResponseBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponseOrBuilder>(
connectResponse_,
getParentForChildren(),
isClean());
connectResponse_ = null;
}
return connectResponseBuilder_;
}
// optional .RpcRequest rpcRequest = 3;
private com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest rpcRequest_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequestOrBuilder> rpcRequestBuilder_;
public boolean hasRpcRequest() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest getRpcRequest() {
if (rpcRequestBuilder_ == null) {
return rpcRequest_;
} else {
return rpcRequestBuilder_.getMessage();
}
}
public Builder setRpcRequest(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest value) {
if (rpcRequestBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
rpcRequest_ = value;
onChanged();
} else {
rpcRequestBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
return this;
}
public Builder setRpcRequest(
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest.Builder builderForValue) {
if (rpcRequestBuilder_ == null) {
rpcRequest_ = builderForValue.build();
onChanged();
} else {
rpcRequestBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
return this;
}
public Builder mergeRpcRequest(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest value) {
if (rpcRequestBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004) &&
rpcRequest_ != com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest.getDefaultInstance()) {
rpcRequest_ =
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest.newBuilder(rpcRequest_).mergeFrom(value).buildPartial();
} else {
rpcRequest_ = value;
}
onChanged();
} else {
rpcRequestBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000004;
return this;
}
public Builder clearRpcRequest() {
if (rpcRequestBuilder_ == null) {
rpcRequest_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest.getDefaultInstance();
onChanged();
} else {
rpcRequestBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest.Builder getRpcRequestBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getRpcRequestFieldBuilder().getBuilder();
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequestOrBuilder getRpcRequestOrBuilder() {
if (rpcRequestBuilder_ != null) {
return rpcRequestBuilder_.getMessageOrBuilder();
} else {
return rpcRequest_;
}
}
private com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequestOrBuilder>
getRpcRequestFieldBuilder() {
if (rpcRequestBuilder_ == null) {
rpcRequestBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequestOrBuilder>(
rpcRequest_,
getParentForChildren(),
isClean());
rpcRequest_ = null;
}
return rpcRequestBuilder_;
}
// optional .RpcResponse rpcResponse = 4;
private com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse rpcResponse_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponseOrBuilder> rpcResponseBuilder_;
public boolean hasRpcResponse() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse getRpcResponse() {
if (rpcResponseBuilder_ == null) {
return rpcResponse_;
} else {
return rpcResponseBuilder_.getMessage();
}
}
public Builder setRpcResponse(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse value) {
if (rpcResponseBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
rpcResponse_ = value;
onChanged();
} else {
rpcResponseBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
return this;
}
public Builder setRpcResponse(
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse.Builder builderForValue) {
if (rpcResponseBuilder_ == null) {
rpcResponse_ = builderForValue.build();
onChanged();
} else {
rpcResponseBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
return this;
}
public Builder mergeRpcResponse(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse value) {
if (rpcResponseBuilder_ == null) {
if (((bitField0_ & 0x00000008) == 0x00000008) &&
rpcResponse_ != com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse.getDefaultInstance()) {
rpcResponse_ =
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse.newBuilder(rpcResponse_).mergeFrom(value).buildPartial();
} else {
rpcResponse_ = value;
}
onChanged();
} else {
rpcResponseBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000008;
return this;
}
public Builder clearRpcResponse() {
if (rpcResponseBuilder_ == null) {
rpcResponse_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse.getDefaultInstance();
onChanged();
} else {
rpcResponseBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse.Builder getRpcResponseBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getRpcResponseFieldBuilder().getBuilder();
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponseOrBuilder getRpcResponseOrBuilder() {
if (rpcResponseBuilder_ != null) {
return rpcResponseBuilder_.getMessageOrBuilder();
} else {
return rpcResponse_;
}
}
private com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponseOrBuilder>
getRpcResponseFieldBuilder() {
if (rpcResponseBuilder_ == null) {
rpcResponseBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponseOrBuilder>(
rpcResponse_,
getParentForChildren(),
isClean());
rpcResponse_ = null;
}
return rpcResponseBuilder_;
}
// optional .RpcError rpcError = 5;
private com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError rpcError_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcErrorOrBuilder> rpcErrorBuilder_;
public boolean hasRpcError() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError getRpcError() {
if (rpcErrorBuilder_ == null) {
return rpcError_;
} else {
return rpcErrorBuilder_.getMessage();
}
}
public Builder setRpcError(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError value) {
if (rpcErrorBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
rpcError_ = value;
onChanged();
} else {
rpcErrorBuilder_.setMessage(value);
}
bitField0_ |= 0x00000010;
return this;
}
public Builder setRpcError(
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError.Builder builderForValue) {
if (rpcErrorBuilder_ == null) {
rpcError_ = builderForValue.build();
onChanged();
} else {
rpcErrorBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000010;
return this;
}
public Builder mergeRpcError(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError value) {
if (rpcErrorBuilder_ == null) {
if (((bitField0_ & 0x00000010) == 0x00000010) &&
rpcError_ != com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError.getDefaultInstance()) {
rpcError_ =
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError.newBuilder(rpcError_).mergeFrom(value).buildPartial();
} else {
rpcError_ = value;
}
onChanged();
} else {
rpcErrorBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000010;
return this;
}
public Builder clearRpcError() {
if (rpcErrorBuilder_ == null) {
rpcError_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError.getDefaultInstance();
onChanged();
} else {
rpcErrorBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError.Builder getRpcErrorBuilder() {
bitField0_ |= 0x00000010;
onChanged();
return getRpcErrorFieldBuilder().getBuilder();
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcErrorOrBuilder getRpcErrorOrBuilder() {
if (rpcErrorBuilder_ != null) {
return rpcErrorBuilder_.getMessageOrBuilder();
} else {
return rpcError_;
}
}
private com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcErrorOrBuilder>
getRpcErrorFieldBuilder() {
if (rpcErrorBuilder_ == null) {
rpcErrorBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcErrorOrBuilder>(
rpcError_,
getParentForChildren(),
isClean());
rpcError_ = null;
}
return rpcErrorBuilder_;
}
// optional .RpcCancel rpcCancel = 6;
private com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel rpcCancel_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancelOrBuilder> rpcCancelBuilder_;
public boolean hasRpcCancel() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel getRpcCancel() {
if (rpcCancelBuilder_ == null) {
return rpcCancel_;
} else {
return rpcCancelBuilder_.getMessage();
}
}
public Builder setRpcCancel(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel value) {
if (rpcCancelBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
rpcCancel_ = value;
onChanged();
} else {
rpcCancelBuilder_.setMessage(value);
}
bitField0_ |= 0x00000020;
return this;
}
public Builder setRpcCancel(
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel.Builder builderForValue) {
if (rpcCancelBuilder_ == null) {
rpcCancel_ = builderForValue.build();
onChanged();
} else {
rpcCancelBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000020;
return this;
}
public Builder mergeRpcCancel(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel value) {
if (rpcCancelBuilder_ == null) {
if (((bitField0_ & 0x00000020) == 0x00000020) &&
rpcCancel_ != com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel.getDefaultInstance()) {
rpcCancel_ =
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel.newBuilder(rpcCancel_).mergeFrom(value).buildPartial();
} else {
rpcCancel_ = value;
}
onChanged();
} else {
rpcCancelBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000020;
return this;
}
public Builder clearRpcCancel() {
if (rpcCancelBuilder_ == null) {
rpcCancel_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel.getDefaultInstance();
onChanged();
} else {
rpcCancelBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000020);
return this;
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel.Builder getRpcCancelBuilder() {
bitField0_ |= 0x00000020;
onChanged();
return getRpcCancelFieldBuilder().getBuilder();
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancelOrBuilder getRpcCancelOrBuilder() {
if (rpcCancelBuilder_ != null) {
return rpcCancelBuilder_.getMessageOrBuilder();
} else {
return rpcCancel_;
}
}
private com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancelOrBuilder>
getRpcCancelFieldBuilder() {
if (rpcCancelBuilder_ == null) {
rpcCancelBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancelOrBuilder>(
rpcCancel_,
getParentForChildren(),
isClean());
rpcCancel_ = null;
}
return rpcCancelBuilder_;
}
// @@protoc_insertion_point(builder_scope:WirePayload)
}
static {
defaultInstance = new WirePayload(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:WirePayload)
}
private static com.google.protobuf.Descriptors.Descriptor
internal_static_ConnectRequest_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_ConnectRequest_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_ConnectResponse_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_ConnectResponse_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_RpcRequest_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_RpcRequest_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_RpcResponse_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_RpcResponse_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_RpcError_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_RpcError_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_RpcCancel_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_RpcCancel_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_WirePayload_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_WirePayload_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\031protobuf-rpc-duplex.proto\"f\n\016ConnectRe" +
"quest\022\025\n\rcorrelationId\030\001 \002(\005\022\026\n\016clientHo" +
"stName\030\002 \002(\t\022\022\n\nclientPort\030\003 \002(\005\022\021\n\tclie" +
"ntPID\030\004 \002(\t\"a\n\017ConnectResponse\022\025\n\rcorrel" +
"ationId\030\001 \002(\005\022\021\n\tserverPID\030\002 \001(\t\022$\n\terro" +
"rCode\030\003 \001(\0162\021.ConnectErrorCode\"n\n\nRpcReq" +
"uest\022\025\n\rcorrelationId\030\001 \002(\005\022\031\n\021serviceId" +
"entifier\030\002 \002(\t\022\030\n\020methodIdentifier\030\003 \002(\t" +
"\022\024\n\014requestBytes\030\004 \002(\014\";\n\013RpcResponse\022\025\n" +
"\rcorrelationId\030\001 \002(\005\022\025\n\rresponseBytes\030\002 ",
"\002(\014\"7\n\010RpcError\022\025\n\rcorrelationId\030\001 \002(\005\022\024" +
"\n\014errorMessage\030\002 \002(\t\"\"\n\tRpcCancel\022\025\n\rcor" +
"relationId\030\001 \002(\005\"\341\001\n\013WirePayload\022\'\n\016conn" +
"ectRequest\030\001 \001(\0132\017.ConnectRequest\022)\n\017con" +
"nectResponse\030\002 \001(\0132\020.ConnectResponse\022\037\n\n" +
"rpcRequest\030\003 \001(\0132\013.RpcRequest\022!\n\013rpcResp" +
"onse\030\004 \001(\0132\014.RpcResponse\022\033\n\010rpcError\030\005 \001" +
"(\0132\t.RpcError\022\035\n\trpcCancel\030\006 \001(\0132\n.RpcCa" +
"ncel*)\n\020ConnectErrorCode\022\025\n\021ALREADY_CONN" +
"ECTED\020\000B;\n\'com.googlecode.protobuf.pro.d",
"uplex.wireB\016DuplexProtocolH\001"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
internal_static_ConnectRequest_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_ConnectRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_ConnectRequest_descriptor,
new java.lang.String[] { "CorrelationId", "ClientHostName", "ClientPort", "ClientPID", },
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest.class,
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest.Builder.class);
internal_static_ConnectResponse_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_ConnectResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_ConnectResponse_descriptor,
new java.lang.String[] { "CorrelationId", "ServerPID", "ErrorCode", },
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse.class,
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse.Builder.class);
internal_static_RpcRequest_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_RpcRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_RpcRequest_descriptor,
new java.lang.String[] { "CorrelationId", "ServiceIdentifier", "MethodIdentifier", "RequestBytes", },
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest.class,
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest.Builder.class);
internal_static_RpcResponse_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_RpcResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_RpcResponse_descriptor,
new java.lang.String[] { "CorrelationId", "ResponseBytes", },
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse.class,
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse.Builder.class);
internal_static_RpcError_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_RpcError_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_RpcError_descriptor,
new java.lang.String[] { "CorrelationId", "ErrorMessage", },
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError.class,
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError.Builder.class);
internal_static_RpcCancel_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_RpcCancel_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_RpcCancel_descriptor,
new java.lang.String[] { "CorrelationId", },
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel.class,
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel.Builder.class);
internal_static_WirePayload_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_WirePayload_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_WirePayload_descriptor,
new java.lang.String[] { "ConnectRequest", "ConnectResponse", "RpcRequest", "RpcResponse", "RpcError", "RpcCancel", },
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload.class,
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload.Builder.class);
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
}, assigner);
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy