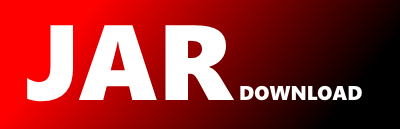
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of protobuf-rpc-pro-duplex Show documentation
Show all versions of protobuf-rpc-pro-duplex Show documentation
Protobuf RPC Pro is a java protocol buffers RPC implementation featuring bidirectional RPC calls and TCP socket connection re-use and the option of secure communications.
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: protobuf-rpc-duplex.proto
package com.googlecode.protobuf.pro.duplex.wire;
public final class DuplexProtocol {
private DuplexProtocol() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
}
/**
* Protobuf enum {@code ConnectErrorCode}
*/
public enum ConnectErrorCode
implements com.google.protobuf.ProtocolMessageEnum {
/**
* ALREADY_CONNECTED = 0;
*
*
* Client with same name already connected.
*
*/
ALREADY_CONNECTED(0, 0),
;
/**
* ALREADY_CONNECTED = 0;
*
*
* Client with same name already connected.
*
*/
public static final int ALREADY_CONNECTED_VALUE = 0;
public final int getNumber() { return value; }
public static ConnectErrorCode valueOf(int value) {
switch (value) {
case 0: return ALREADY_CONNECTED;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public ConnectErrorCode findValueByNumber(int number) {
return ConnectErrorCode.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.getDescriptor().getEnumTypes().get(0);
}
private static final ConnectErrorCode[] VALUES = values();
public static ConnectErrorCode valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private ConnectErrorCode(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:ConnectErrorCode)
}
public interface ConnectRequestOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required int32 correlationId = 1;
/**
* required int32 correlationId = 1;
*/
boolean hasCorrelationId();
/**
* required int32 correlationId = 1;
*/
int getCorrelationId();
// required string clientHostName = 2;
/**
* required string clientHostName = 2;
*/
boolean hasClientHostName();
/**
* required string clientHostName = 2;
*/
java.lang.String getClientHostName();
/**
* required string clientHostName = 2;
*/
com.google.protobuf.ByteString
getClientHostNameBytes();
// required int32 clientPort = 3;
/**
* required int32 clientPort = 3;
*/
boolean hasClientPort();
/**
* required int32 clientPort = 3;
*/
int getClientPort();
// required string clientPID = 4;
/**
* required string clientPID = 4;
*/
boolean hasClientPID();
/**
* required string clientPID = 4;
*/
java.lang.String getClientPID();
/**
* required string clientPID = 4;
*/
com.google.protobuf.ByteString
getClientPIDBytes();
// optional bool compress = 5;
/**
* optional bool compress = 5;
*/
boolean hasCompress();
/**
* optional bool compress = 5;
*/
boolean getCompress();
}
/**
* Protobuf type {@code ConnectRequest}
*/
public static final class ConnectRequest extends
com.google.protobuf.GeneratedMessage
implements ConnectRequestOrBuilder {
// Use ConnectRequest.newBuilder() to construct.
private ConnectRequest(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private ConnectRequest(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final ConnectRequest defaultInstance;
public static ConnectRequest getDefaultInstance() {
return defaultInstance;
}
public ConnectRequest getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ConnectRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
correlationId_ = input.readInt32();
break;
}
case 18: {
bitField0_ |= 0x00000002;
clientHostName_ = input.readBytes();
break;
}
case 24: {
bitField0_ |= 0x00000004;
clientPort_ = input.readInt32();
break;
}
case 34: {
bitField0_ |= 0x00000008;
clientPID_ = input.readBytes();
break;
}
case 40: {
bitField0_ |= 0x00000010;
compress_ = input.readBool();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_ConnectRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_ConnectRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest.class, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public ConnectRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ConnectRequest(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required int32 correlationId = 1;
public static final int CORRELATIONID_FIELD_NUMBER = 1;
private int correlationId_;
/**
* required int32 correlationId = 1;
*/
public boolean hasCorrelationId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int32 correlationId = 1;
*/
public int getCorrelationId() {
return correlationId_;
}
// required string clientHostName = 2;
public static final int CLIENTHOSTNAME_FIELD_NUMBER = 2;
private java.lang.Object clientHostName_;
/**
* required string clientHostName = 2;
*/
public boolean hasClientHostName() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required string clientHostName = 2;
*/
public java.lang.String getClientHostName() {
java.lang.Object ref = clientHostName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
clientHostName_ = s;
}
return s;
}
}
/**
* required string clientHostName = 2;
*/
public com.google.protobuf.ByteString
getClientHostNameBytes() {
java.lang.Object ref = clientHostName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
clientHostName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// required int32 clientPort = 3;
public static final int CLIENTPORT_FIELD_NUMBER = 3;
private int clientPort_;
/**
* required int32 clientPort = 3;
*/
public boolean hasClientPort() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required int32 clientPort = 3;
*/
public int getClientPort() {
return clientPort_;
}
// required string clientPID = 4;
public static final int CLIENTPID_FIELD_NUMBER = 4;
private java.lang.Object clientPID_;
/**
* required string clientPID = 4;
*/
public boolean hasClientPID() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* required string clientPID = 4;
*/
public java.lang.String getClientPID() {
java.lang.Object ref = clientPID_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
clientPID_ = s;
}
return s;
}
}
/**
* required string clientPID = 4;
*/
public com.google.protobuf.ByteString
getClientPIDBytes() {
java.lang.Object ref = clientPID_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
clientPID_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional bool compress = 5;
public static final int COMPRESS_FIELD_NUMBER = 5;
private boolean compress_;
/**
* optional bool compress = 5;
*/
public boolean hasCompress() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional bool compress = 5;
*/
public boolean getCompress() {
return compress_;
}
private void initFields() {
correlationId_ = 0;
clientHostName_ = "";
clientPort_ = 0;
clientPID_ = "";
compress_ = false;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasCorrelationId()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasClientHostName()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasClientPort()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasClientPID()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt32(1, correlationId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getClientHostNameBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeInt32(3, clientPort_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBytes(4, getClientPIDBytes());
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeBool(5, compress_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, correlationId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getClientHostNameBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, clientPort_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, getClientPIDBytes());
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, compress_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code ConnectRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_ConnectRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_ConnectRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest.class, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest.Builder.class);
}
// Construct using com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
correlationId_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
clientHostName_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
clientPort_ = 0;
bitField0_ = (bitField0_ & ~0x00000004);
clientPID_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
compress_ = false;
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_ConnectRequest_descriptor;
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest getDefaultInstanceForType() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest.getDefaultInstance();
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest build() {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest buildPartial() {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest result = new com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.correlationId_ = correlationId_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.clientHostName_ = clientHostName_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.clientPort_ = clientPort_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.clientPID_ = clientPID_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.compress_ = compress_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest) {
return mergeFrom((com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest other) {
if (other == com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest.getDefaultInstance()) return this;
if (other.hasCorrelationId()) {
setCorrelationId(other.getCorrelationId());
}
if (other.hasClientHostName()) {
bitField0_ |= 0x00000002;
clientHostName_ = other.clientHostName_;
onChanged();
}
if (other.hasClientPort()) {
setClientPort(other.getClientPort());
}
if (other.hasClientPID()) {
bitField0_ |= 0x00000008;
clientPID_ = other.clientPID_;
onChanged();
}
if (other.hasCompress()) {
setCompress(other.getCompress());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasCorrelationId()) {
return false;
}
if (!hasClientHostName()) {
return false;
}
if (!hasClientPort()) {
return false;
}
if (!hasClientPID()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required int32 correlationId = 1;
private int correlationId_ ;
/**
* required int32 correlationId = 1;
*/
public boolean hasCorrelationId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int32 correlationId = 1;
*/
public int getCorrelationId() {
return correlationId_;
}
/**
* required int32 correlationId = 1;
*/
public Builder setCorrelationId(int value) {
bitField0_ |= 0x00000001;
correlationId_ = value;
onChanged();
return this;
}
/**
* required int32 correlationId = 1;
*/
public Builder clearCorrelationId() {
bitField0_ = (bitField0_ & ~0x00000001);
correlationId_ = 0;
onChanged();
return this;
}
// required string clientHostName = 2;
private java.lang.Object clientHostName_ = "";
/**
* required string clientHostName = 2;
*/
public boolean hasClientHostName() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required string clientHostName = 2;
*/
public java.lang.String getClientHostName() {
java.lang.Object ref = clientHostName_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
clientHostName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string clientHostName = 2;
*/
public com.google.protobuf.ByteString
getClientHostNameBytes() {
java.lang.Object ref = clientHostName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
clientHostName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string clientHostName = 2;
*/
public Builder setClientHostName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
clientHostName_ = value;
onChanged();
return this;
}
/**
* required string clientHostName = 2;
*/
public Builder clearClientHostName() {
bitField0_ = (bitField0_ & ~0x00000002);
clientHostName_ = getDefaultInstance().getClientHostName();
onChanged();
return this;
}
/**
* required string clientHostName = 2;
*/
public Builder setClientHostNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
clientHostName_ = value;
onChanged();
return this;
}
// required int32 clientPort = 3;
private int clientPort_ ;
/**
* required int32 clientPort = 3;
*/
public boolean hasClientPort() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required int32 clientPort = 3;
*/
public int getClientPort() {
return clientPort_;
}
/**
* required int32 clientPort = 3;
*/
public Builder setClientPort(int value) {
bitField0_ |= 0x00000004;
clientPort_ = value;
onChanged();
return this;
}
/**
* required int32 clientPort = 3;
*/
public Builder clearClientPort() {
bitField0_ = (bitField0_ & ~0x00000004);
clientPort_ = 0;
onChanged();
return this;
}
// required string clientPID = 4;
private java.lang.Object clientPID_ = "";
/**
* required string clientPID = 4;
*/
public boolean hasClientPID() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* required string clientPID = 4;
*/
public java.lang.String getClientPID() {
java.lang.Object ref = clientPID_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
clientPID_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string clientPID = 4;
*/
public com.google.protobuf.ByteString
getClientPIDBytes() {
java.lang.Object ref = clientPID_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
clientPID_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string clientPID = 4;
*/
public Builder setClientPID(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
clientPID_ = value;
onChanged();
return this;
}
/**
* required string clientPID = 4;
*/
public Builder clearClientPID() {
bitField0_ = (bitField0_ & ~0x00000008);
clientPID_ = getDefaultInstance().getClientPID();
onChanged();
return this;
}
/**
* required string clientPID = 4;
*/
public Builder setClientPIDBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
clientPID_ = value;
onChanged();
return this;
}
// optional bool compress = 5;
private boolean compress_ ;
/**
* optional bool compress = 5;
*/
public boolean hasCompress() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional bool compress = 5;
*/
public boolean getCompress() {
return compress_;
}
/**
* optional bool compress = 5;
*/
public Builder setCompress(boolean value) {
bitField0_ |= 0x00000010;
compress_ = value;
onChanged();
return this;
}
/**
* optional bool compress = 5;
*/
public Builder clearCompress() {
bitField0_ = (bitField0_ & ~0x00000010);
compress_ = false;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:ConnectRequest)
}
static {
defaultInstance = new ConnectRequest(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:ConnectRequest)
}
public interface ConnectResponseOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required int32 correlationId = 1;
/**
* required int32 correlationId = 1;
*/
boolean hasCorrelationId();
/**
* required int32 correlationId = 1;
*/
int getCorrelationId();
// optional string serverPID = 2;
/**
* optional string serverPID = 2;
*/
boolean hasServerPID();
/**
* optional string serverPID = 2;
*/
java.lang.String getServerPID();
/**
* optional string serverPID = 2;
*/
com.google.protobuf.ByteString
getServerPIDBytes();
// optional .ConnectErrorCode errorCode = 3;
/**
* optional .ConnectErrorCode errorCode = 3;
*/
boolean hasErrorCode();
/**
* optional .ConnectErrorCode errorCode = 3;
*/
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectErrorCode getErrorCode();
// optional bool compress = 4;
/**
* optional bool compress = 4;
*/
boolean hasCompress();
/**
* optional bool compress = 4;
*/
boolean getCompress();
}
/**
* Protobuf type {@code ConnectResponse}
*/
public static final class ConnectResponse extends
com.google.protobuf.GeneratedMessage
implements ConnectResponseOrBuilder {
// Use ConnectResponse.newBuilder() to construct.
private ConnectResponse(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private ConnectResponse(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final ConnectResponse defaultInstance;
public static ConnectResponse getDefaultInstance() {
return defaultInstance;
}
public ConnectResponse getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ConnectResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
correlationId_ = input.readInt32();
break;
}
case 18: {
bitField0_ |= 0x00000002;
serverPID_ = input.readBytes();
break;
}
case 24: {
int rawValue = input.readEnum();
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectErrorCode value = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectErrorCode.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(3, rawValue);
} else {
bitField0_ |= 0x00000004;
errorCode_ = value;
}
break;
}
case 32: {
bitField0_ |= 0x00000008;
compress_ = input.readBool();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_ConnectResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_ConnectResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse.class, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public ConnectResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ConnectResponse(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required int32 correlationId = 1;
public static final int CORRELATIONID_FIELD_NUMBER = 1;
private int correlationId_;
/**
* required int32 correlationId = 1;
*/
public boolean hasCorrelationId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int32 correlationId = 1;
*/
public int getCorrelationId() {
return correlationId_;
}
// optional string serverPID = 2;
public static final int SERVERPID_FIELD_NUMBER = 2;
private java.lang.Object serverPID_;
/**
* optional string serverPID = 2;
*/
public boolean hasServerPID() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string serverPID = 2;
*/
public java.lang.String getServerPID() {
java.lang.Object ref = serverPID_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
serverPID_ = s;
}
return s;
}
}
/**
* optional string serverPID = 2;
*/
public com.google.protobuf.ByteString
getServerPIDBytes() {
java.lang.Object ref = serverPID_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
serverPID_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional .ConnectErrorCode errorCode = 3;
public static final int ERRORCODE_FIELD_NUMBER = 3;
private com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectErrorCode errorCode_;
/**
* optional .ConnectErrorCode errorCode = 3;
*/
public boolean hasErrorCode() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .ConnectErrorCode errorCode = 3;
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectErrorCode getErrorCode() {
return errorCode_;
}
// optional bool compress = 4;
public static final int COMPRESS_FIELD_NUMBER = 4;
private boolean compress_;
/**
* optional bool compress = 4;
*/
public boolean hasCompress() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional bool compress = 4;
*/
public boolean getCompress() {
return compress_;
}
private void initFields() {
correlationId_ = 0;
serverPID_ = "";
errorCode_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectErrorCode.ALREADY_CONNECTED;
compress_ = false;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasCorrelationId()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt32(1, correlationId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getServerPIDBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeEnum(3, errorCode_.getNumber());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBool(4, compress_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, correlationId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getServerPIDBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(3, errorCode_.getNumber());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(4, compress_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code ConnectResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_ConnectResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_ConnectResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse.class, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse.Builder.class);
}
// Construct using com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
correlationId_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
serverPID_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
errorCode_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectErrorCode.ALREADY_CONNECTED;
bitField0_ = (bitField0_ & ~0x00000004);
compress_ = false;
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_ConnectResponse_descriptor;
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse getDefaultInstanceForType() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse.getDefaultInstance();
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse build() {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse buildPartial() {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse result = new com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.correlationId_ = correlationId_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.serverPID_ = serverPID_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.errorCode_ = errorCode_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.compress_ = compress_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse) {
return mergeFrom((com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse other) {
if (other == com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse.getDefaultInstance()) return this;
if (other.hasCorrelationId()) {
setCorrelationId(other.getCorrelationId());
}
if (other.hasServerPID()) {
bitField0_ |= 0x00000002;
serverPID_ = other.serverPID_;
onChanged();
}
if (other.hasErrorCode()) {
setErrorCode(other.getErrorCode());
}
if (other.hasCompress()) {
setCompress(other.getCompress());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasCorrelationId()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required int32 correlationId = 1;
private int correlationId_ ;
/**
* required int32 correlationId = 1;
*/
public boolean hasCorrelationId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int32 correlationId = 1;
*/
public int getCorrelationId() {
return correlationId_;
}
/**
* required int32 correlationId = 1;
*/
public Builder setCorrelationId(int value) {
bitField0_ |= 0x00000001;
correlationId_ = value;
onChanged();
return this;
}
/**
* required int32 correlationId = 1;
*/
public Builder clearCorrelationId() {
bitField0_ = (bitField0_ & ~0x00000001);
correlationId_ = 0;
onChanged();
return this;
}
// optional string serverPID = 2;
private java.lang.Object serverPID_ = "";
/**
* optional string serverPID = 2;
*/
public boolean hasServerPID() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string serverPID = 2;
*/
public java.lang.String getServerPID() {
java.lang.Object ref = serverPID_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
serverPID_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string serverPID = 2;
*/
public com.google.protobuf.ByteString
getServerPIDBytes() {
java.lang.Object ref = serverPID_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
serverPID_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string serverPID = 2;
*/
public Builder setServerPID(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
serverPID_ = value;
onChanged();
return this;
}
/**
* optional string serverPID = 2;
*/
public Builder clearServerPID() {
bitField0_ = (bitField0_ & ~0x00000002);
serverPID_ = getDefaultInstance().getServerPID();
onChanged();
return this;
}
/**
* optional string serverPID = 2;
*/
public Builder setServerPIDBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
serverPID_ = value;
onChanged();
return this;
}
// optional .ConnectErrorCode errorCode = 3;
private com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectErrorCode errorCode_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectErrorCode.ALREADY_CONNECTED;
/**
* optional .ConnectErrorCode errorCode = 3;
*/
public boolean hasErrorCode() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .ConnectErrorCode errorCode = 3;
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectErrorCode getErrorCode() {
return errorCode_;
}
/**
* optional .ConnectErrorCode errorCode = 3;
*/
public Builder setErrorCode(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectErrorCode value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
errorCode_ = value;
onChanged();
return this;
}
/**
* optional .ConnectErrorCode errorCode = 3;
*/
public Builder clearErrorCode() {
bitField0_ = (bitField0_ & ~0x00000004);
errorCode_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectErrorCode.ALREADY_CONNECTED;
onChanged();
return this;
}
// optional bool compress = 4;
private boolean compress_ ;
/**
* optional bool compress = 4;
*/
public boolean hasCompress() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional bool compress = 4;
*/
public boolean getCompress() {
return compress_;
}
/**
* optional bool compress = 4;
*/
public Builder setCompress(boolean value) {
bitField0_ |= 0x00000008;
compress_ = value;
onChanged();
return this;
}
/**
* optional bool compress = 4;
*/
public Builder clearCompress() {
bitField0_ = (bitField0_ & ~0x00000008);
compress_ = false;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:ConnectResponse)
}
static {
defaultInstance = new ConnectResponse(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:ConnectResponse)
}
public interface RpcRequestOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required int32 correlationId = 1;
/**
* required int32 correlationId = 1;
*/
boolean hasCorrelationId();
/**
* required int32 correlationId = 1;
*/
int getCorrelationId();
// required string serviceIdentifier = 2;
/**
* required string serviceIdentifier = 2;
*/
boolean hasServiceIdentifier();
/**
* required string serviceIdentifier = 2;
*/
java.lang.String getServiceIdentifier();
/**
* required string serviceIdentifier = 2;
*/
com.google.protobuf.ByteString
getServiceIdentifierBytes();
// required string methodIdentifier = 3;
/**
* required string methodIdentifier = 3;
*/
boolean hasMethodIdentifier();
/**
* required string methodIdentifier = 3;
*/
java.lang.String getMethodIdentifier();
/**
* required string methodIdentifier = 3;
*/
com.google.protobuf.ByteString
getMethodIdentifierBytes();
// required bytes requestBytes = 4;
/**
* required bytes requestBytes = 4;
*/
boolean hasRequestBytes();
/**
* required bytes requestBytes = 4;
*/
com.google.protobuf.ByteString getRequestBytes();
// optional int32 timeoutMs = 5;
/**
* optional int32 timeoutMs = 5;
*/
boolean hasTimeoutMs();
/**
* optional int32 timeoutMs = 5;
*/
int getTimeoutMs();
}
/**
* Protobuf type {@code RpcRequest}
*/
public static final class RpcRequest extends
com.google.protobuf.GeneratedMessage
implements RpcRequestOrBuilder {
// Use RpcRequest.newBuilder() to construct.
private RpcRequest(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private RpcRequest(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final RpcRequest defaultInstance;
public static RpcRequest getDefaultInstance() {
return defaultInstance;
}
public RpcRequest getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RpcRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
correlationId_ = input.readInt32();
break;
}
case 18: {
bitField0_ |= 0x00000002;
serviceIdentifier_ = input.readBytes();
break;
}
case 26: {
bitField0_ |= 0x00000004;
methodIdentifier_ = input.readBytes();
break;
}
case 34: {
bitField0_ |= 0x00000008;
requestBytes_ = input.readBytes();
break;
}
case 40: {
bitField0_ |= 0x00000010;
timeoutMs_ = input.readInt32();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_RpcRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_RpcRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest.class, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public RpcRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RpcRequest(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required int32 correlationId = 1;
public static final int CORRELATIONID_FIELD_NUMBER = 1;
private int correlationId_;
/**
* required int32 correlationId = 1;
*/
public boolean hasCorrelationId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int32 correlationId = 1;
*/
public int getCorrelationId() {
return correlationId_;
}
// required string serviceIdentifier = 2;
public static final int SERVICEIDENTIFIER_FIELD_NUMBER = 2;
private java.lang.Object serviceIdentifier_;
/**
* required string serviceIdentifier = 2;
*/
public boolean hasServiceIdentifier() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required string serviceIdentifier = 2;
*/
public java.lang.String getServiceIdentifier() {
java.lang.Object ref = serviceIdentifier_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
serviceIdentifier_ = s;
}
return s;
}
}
/**
* required string serviceIdentifier = 2;
*/
public com.google.protobuf.ByteString
getServiceIdentifierBytes() {
java.lang.Object ref = serviceIdentifier_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
serviceIdentifier_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// required string methodIdentifier = 3;
public static final int METHODIDENTIFIER_FIELD_NUMBER = 3;
private java.lang.Object methodIdentifier_;
/**
* required string methodIdentifier = 3;
*/
public boolean hasMethodIdentifier() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required string methodIdentifier = 3;
*/
public java.lang.String getMethodIdentifier() {
java.lang.Object ref = methodIdentifier_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
methodIdentifier_ = s;
}
return s;
}
}
/**
* required string methodIdentifier = 3;
*/
public com.google.protobuf.ByteString
getMethodIdentifierBytes() {
java.lang.Object ref = methodIdentifier_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
methodIdentifier_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// required bytes requestBytes = 4;
public static final int REQUESTBYTES_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString requestBytes_;
/**
* required bytes requestBytes = 4;
*/
public boolean hasRequestBytes() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* required bytes requestBytes = 4;
*/
public com.google.protobuf.ByteString getRequestBytes() {
return requestBytes_;
}
// optional int32 timeoutMs = 5;
public static final int TIMEOUTMS_FIELD_NUMBER = 5;
private int timeoutMs_;
/**
* optional int32 timeoutMs = 5;
*/
public boolean hasTimeoutMs() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional int32 timeoutMs = 5;
*/
public int getTimeoutMs() {
return timeoutMs_;
}
private void initFields() {
correlationId_ = 0;
serviceIdentifier_ = "";
methodIdentifier_ = "";
requestBytes_ = com.google.protobuf.ByteString.EMPTY;
timeoutMs_ = 0;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasCorrelationId()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasServiceIdentifier()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasMethodIdentifier()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasRequestBytes()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt32(1, correlationId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getServiceIdentifierBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBytes(3, getMethodIdentifierBytes());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBytes(4, requestBytes_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeInt32(5, timeoutMs_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, correlationId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getServiceIdentifierBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, getMethodIdentifierBytes());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, requestBytes_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(5, timeoutMs_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code RpcRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_RpcRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_RpcRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest.class, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest.Builder.class);
}
// Construct using com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
correlationId_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
serviceIdentifier_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
methodIdentifier_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
requestBytes_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000008);
timeoutMs_ = 0;
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_RpcRequest_descriptor;
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest getDefaultInstanceForType() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest.getDefaultInstance();
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest build() {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest buildPartial() {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest result = new com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.correlationId_ = correlationId_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.serviceIdentifier_ = serviceIdentifier_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.methodIdentifier_ = methodIdentifier_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.requestBytes_ = requestBytes_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.timeoutMs_ = timeoutMs_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest) {
return mergeFrom((com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest other) {
if (other == com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest.getDefaultInstance()) return this;
if (other.hasCorrelationId()) {
setCorrelationId(other.getCorrelationId());
}
if (other.hasServiceIdentifier()) {
bitField0_ |= 0x00000002;
serviceIdentifier_ = other.serviceIdentifier_;
onChanged();
}
if (other.hasMethodIdentifier()) {
bitField0_ |= 0x00000004;
methodIdentifier_ = other.methodIdentifier_;
onChanged();
}
if (other.hasRequestBytes()) {
setRequestBytes(other.getRequestBytes());
}
if (other.hasTimeoutMs()) {
setTimeoutMs(other.getTimeoutMs());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasCorrelationId()) {
return false;
}
if (!hasServiceIdentifier()) {
return false;
}
if (!hasMethodIdentifier()) {
return false;
}
if (!hasRequestBytes()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required int32 correlationId = 1;
private int correlationId_ ;
/**
* required int32 correlationId = 1;
*/
public boolean hasCorrelationId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int32 correlationId = 1;
*/
public int getCorrelationId() {
return correlationId_;
}
/**
* required int32 correlationId = 1;
*/
public Builder setCorrelationId(int value) {
bitField0_ |= 0x00000001;
correlationId_ = value;
onChanged();
return this;
}
/**
* required int32 correlationId = 1;
*/
public Builder clearCorrelationId() {
bitField0_ = (bitField0_ & ~0x00000001);
correlationId_ = 0;
onChanged();
return this;
}
// required string serviceIdentifier = 2;
private java.lang.Object serviceIdentifier_ = "";
/**
* required string serviceIdentifier = 2;
*/
public boolean hasServiceIdentifier() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required string serviceIdentifier = 2;
*/
public java.lang.String getServiceIdentifier() {
java.lang.Object ref = serviceIdentifier_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
serviceIdentifier_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string serviceIdentifier = 2;
*/
public com.google.protobuf.ByteString
getServiceIdentifierBytes() {
java.lang.Object ref = serviceIdentifier_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
serviceIdentifier_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string serviceIdentifier = 2;
*/
public Builder setServiceIdentifier(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
serviceIdentifier_ = value;
onChanged();
return this;
}
/**
* required string serviceIdentifier = 2;
*/
public Builder clearServiceIdentifier() {
bitField0_ = (bitField0_ & ~0x00000002);
serviceIdentifier_ = getDefaultInstance().getServiceIdentifier();
onChanged();
return this;
}
/**
* required string serviceIdentifier = 2;
*/
public Builder setServiceIdentifierBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
serviceIdentifier_ = value;
onChanged();
return this;
}
// required string methodIdentifier = 3;
private java.lang.Object methodIdentifier_ = "";
/**
* required string methodIdentifier = 3;
*/
public boolean hasMethodIdentifier() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required string methodIdentifier = 3;
*/
public java.lang.String getMethodIdentifier() {
java.lang.Object ref = methodIdentifier_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
methodIdentifier_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string methodIdentifier = 3;
*/
public com.google.protobuf.ByteString
getMethodIdentifierBytes() {
java.lang.Object ref = methodIdentifier_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
methodIdentifier_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string methodIdentifier = 3;
*/
public Builder setMethodIdentifier(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
methodIdentifier_ = value;
onChanged();
return this;
}
/**
* required string methodIdentifier = 3;
*/
public Builder clearMethodIdentifier() {
bitField0_ = (bitField0_ & ~0x00000004);
methodIdentifier_ = getDefaultInstance().getMethodIdentifier();
onChanged();
return this;
}
/**
* required string methodIdentifier = 3;
*/
public Builder setMethodIdentifierBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
methodIdentifier_ = value;
onChanged();
return this;
}
// required bytes requestBytes = 4;
private com.google.protobuf.ByteString requestBytes_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes requestBytes = 4;
*/
public boolean hasRequestBytes() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* required bytes requestBytes = 4;
*/
public com.google.protobuf.ByteString getRequestBytes() {
return requestBytes_;
}
/**
* required bytes requestBytes = 4;
*/
public Builder setRequestBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
requestBytes_ = value;
onChanged();
return this;
}
/**
* required bytes requestBytes = 4;
*/
public Builder clearRequestBytes() {
bitField0_ = (bitField0_ & ~0x00000008);
requestBytes_ = getDefaultInstance().getRequestBytes();
onChanged();
return this;
}
// optional int32 timeoutMs = 5;
private int timeoutMs_ ;
/**
* optional int32 timeoutMs = 5;
*/
public boolean hasTimeoutMs() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional int32 timeoutMs = 5;
*/
public int getTimeoutMs() {
return timeoutMs_;
}
/**
* optional int32 timeoutMs = 5;
*/
public Builder setTimeoutMs(int value) {
bitField0_ |= 0x00000010;
timeoutMs_ = value;
onChanged();
return this;
}
/**
* optional int32 timeoutMs = 5;
*/
public Builder clearTimeoutMs() {
bitField0_ = (bitField0_ & ~0x00000010);
timeoutMs_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:RpcRequest)
}
static {
defaultInstance = new RpcRequest(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:RpcRequest)
}
public interface RpcResponseOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required int32 correlationId = 1;
/**
* required int32 correlationId = 1;
*/
boolean hasCorrelationId();
/**
* required int32 correlationId = 1;
*/
int getCorrelationId();
// required bytes responseBytes = 2;
/**
* required bytes responseBytes = 2;
*/
boolean hasResponseBytes();
/**
* required bytes responseBytes = 2;
*/
com.google.protobuf.ByteString getResponseBytes();
}
/**
* Protobuf type {@code RpcResponse}
*/
public static final class RpcResponse extends
com.google.protobuf.GeneratedMessage
implements RpcResponseOrBuilder {
// Use RpcResponse.newBuilder() to construct.
private RpcResponse(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private RpcResponse(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final RpcResponse defaultInstance;
public static RpcResponse getDefaultInstance() {
return defaultInstance;
}
public RpcResponse getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RpcResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
correlationId_ = input.readInt32();
break;
}
case 18: {
bitField0_ |= 0x00000002;
responseBytes_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_RpcResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_RpcResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse.class, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public RpcResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RpcResponse(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required int32 correlationId = 1;
public static final int CORRELATIONID_FIELD_NUMBER = 1;
private int correlationId_;
/**
* required int32 correlationId = 1;
*/
public boolean hasCorrelationId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int32 correlationId = 1;
*/
public int getCorrelationId() {
return correlationId_;
}
// required bytes responseBytes = 2;
public static final int RESPONSEBYTES_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString responseBytes_;
/**
* required bytes responseBytes = 2;
*/
public boolean hasResponseBytes() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required bytes responseBytes = 2;
*/
public com.google.protobuf.ByteString getResponseBytes() {
return responseBytes_;
}
private void initFields() {
correlationId_ = 0;
responseBytes_ = com.google.protobuf.ByteString.EMPTY;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasCorrelationId()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasResponseBytes()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt32(1, correlationId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, responseBytes_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, correlationId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, responseBytes_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code RpcResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_RpcResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_RpcResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse.class, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse.Builder.class);
}
// Construct using com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
correlationId_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
responseBytes_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_RpcResponse_descriptor;
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse getDefaultInstanceForType() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse.getDefaultInstance();
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse build() {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse buildPartial() {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse result = new com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.correlationId_ = correlationId_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.responseBytes_ = responseBytes_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse) {
return mergeFrom((com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse other) {
if (other == com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse.getDefaultInstance()) return this;
if (other.hasCorrelationId()) {
setCorrelationId(other.getCorrelationId());
}
if (other.hasResponseBytes()) {
setResponseBytes(other.getResponseBytes());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasCorrelationId()) {
return false;
}
if (!hasResponseBytes()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required int32 correlationId = 1;
private int correlationId_ ;
/**
* required int32 correlationId = 1;
*/
public boolean hasCorrelationId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int32 correlationId = 1;
*/
public int getCorrelationId() {
return correlationId_;
}
/**
* required int32 correlationId = 1;
*/
public Builder setCorrelationId(int value) {
bitField0_ |= 0x00000001;
correlationId_ = value;
onChanged();
return this;
}
/**
* required int32 correlationId = 1;
*/
public Builder clearCorrelationId() {
bitField0_ = (bitField0_ & ~0x00000001);
correlationId_ = 0;
onChanged();
return this;
}
// required bytes responseBytes = 2;
private com.google.protobuf.ByteString responseBytes_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes responseBytes = 2;
*/
public boolean hasResponseBytes() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required bytes responseBytes = 2;
*/
public com.google.protobuf.ByteString getResponseBytes() {
return responseBytes_;
}
/**
* required bytes responseBytes = 2;
*/
public Builder setResponseBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
responseBytes_ = value;
onChanged();
return this;
}
/**
* required bytes responseBytes = 2;
*/
public Builder clearResponseBytes() {
bitField0_ = (bitField0_ & ~0x00000002);
responseBytes_ = getDefaultInstance().getResponseBytes();
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:RpcResponse)
}
static {
defaultInstance = new RpcResponse(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:RpcResponse)
}
public interface RpcErrorOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required int32 correlationId = 1;
/**
* required int32 correlationId = 1;
*/
boolean hasCorrelationId();
/**
* required int32 correlationId = 1;
*/
int getCorrelationId();
// required string errorMessage = 2;
/**
* required string errorMessage = 2;
*/
boolean hasErrorMessage();
/**
* required string errorMessage = 2;
*/
java.lang.String getErrorMessage();
/**
* required string errorMessage = 2;
*/
com.google.protobuf.ByteString
getErrorMessageBytes();
}
/**
* Protobuf type {@code RpcError}
*/
public static final class RpcError extends
com.google.protobuf.GeneratedMessage
implements RpcErrorOrBuilder {
// Use RpcError.newBuilder() to construct.
private RpcError(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private RpcError(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final RpcError defaultInstance;
public static RpcError getDefaultInstance() {
return defaultInstance;
}
public RpcError getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RpcError(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
correlationId_ = input.readInt32();
break;
}
case 18: {
bitField0_ |= 0x00000002;
errorMessage_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_RpcError_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_RpcError_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError.class, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public RpcError parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RpcError(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required int32 correlationId = 1;
public static final int CORRELATIONID_FIELD_NUMBER = 1;
private int correlationId_;
/**
* required int32 correlationId = 1;
*/
public boolean hasCorrelationId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int32 correlationId = 1;
*/
public int getCorrelationId() {
return correlationId_;
}
// required string errorMessage = 2;
public static final int ERRORMESSAGE_FIELD_NUMBER = 2;
private java.lang.Object errorMessage_;
/**
* required string errorMessage = 2;
*/
public boolean hasErrorMessage() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required string errorMessage = 2;
*/
public java.lang.String getErrorMessage() {
java.lang.Object ref = errorMessage_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
errorMessage_ = s;
}
return s;
}
}
/**
* required string errorMessage = 2;
*/
public com.google.protobuf.ByteString
getErrorMessageBytes() {
java.lang.Object ref = errorMessage_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
errorMessage_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private void initFields() {
correlationId_ = 0;
errorMessage_ = "";
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasCorrelationId()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasErrorMessage()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt32(1, correlationId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getErrorMessageBytes());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, correlationId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getErrorMessageBytes());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code RpcError}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcErrorOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_RpcError_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_RpcError_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError.class, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError.Builder.class);
}
// Construct using com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
correlationId_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
errorMessage_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_RpcError_descriptor;
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError getDefaultInstanceForType() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError.getDefaultInstance();
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError build() {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError buildPartial() {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError result = new com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.correlationId_ = correlationId_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.errorMessage_ = errorMessage_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError) {
return mergeFrom((com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError other) {
if (other == com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError.getDefaultInstance()) return this;
if (other.hasCorrelationId()) {
setCorrelationId(other.getCorrelationId());
}
if (other.hasErrorMessage()) {
bitField0_ |= 0x00000002;
errorMessage_ = other.errorMessage_;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasCorrelationId()) {
return false;
}
if (!hasErrorMessage()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required int32 correlationId = 1;
private int correlationId_ ;
/**
* required int32 correlationId = 1;
*/
public boolean hasCorrelationId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int32 correlationId = 1;
*/
public int getCorrelationId() {
return correlationId_;
}
/**
* required int32 correlationId = 1;
*/
public Builder setCorrelationId(int value) {
bitField0_ |= 0x00000001;
correlationId_ = value;
onChanged();
return this;
}
/**
* required int32 correlationId = 1;
*/
public Builder clearCorrelationId() {
bitField0_ = (bitField0_ & ~0x00000001);
correlationId_ = 0;
onChanged();
return this;
}
// required string errorMessage = 2;
private java.lang.Object errorMessage_ = "";
/**
* required string errorMessage = 2;
*/
public boolean hasErrorMessage() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required string errorMessage = 2;
*/
public java.lang.String getErrorMessage() {
java.lang.Object ref = errorMessage_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
errorMessage_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string errorMessage = 2;
*/
public com.google.protobuf.ByteString
getErrorMessageBytes() {
java.lang.Object ref = errorMessage_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
errorMessage_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string errorMessage = 2;
*/
public Builder setErrorMessage(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
errorMessage_ = value;
onChanged();
return this;
}
/**
* required string errorMessage = 2;
*/
public Builder clearErrorMessage() {
bitField0_ = (bitField0_ & ~0x00000002);
errorMessage_ = getDefaultInstance().getErrorMessage();
onChanged();
return this;
}
/**
* required string errorMessage = 2;
*/
public Builder setErrorMessageBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
errorMessage_ = value;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:RpcError)
}
static {
defaultInstance = new RpcError(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:RpcError)
}
public interface RpcCancelOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required int32 correlationId = 1;
/**
* required int32 correlationId = 1;
*/
boolean hasCorrelationId();
/**
* required int32 correlationId = 1;
*/
int getCorrelationId();
}
/**
* Protobuf type {@code RpcCancel}
*/
public static final class RpcCancel extends
com.google.protobuf.GeneratedMessage
implements RpcCancelOrBuilder {
// Use RpcCancel.newBuilder() to construct.
private RpcCancel(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private RpcCancel(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final RpcCancel defaultInstance;
public static RpcCancel getDefaultInstance() {
return defaultInstance;
}
public RpcCancel getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RpcCancel(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
correlationId_ = input.readInt32();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_RpcCancel_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_RpcCancel_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel.class, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public RpcCancel parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RpcCancel(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required int32 correlationId = 1;
public static final int CORRELATIONID_FIELD_NUMBER = 1;
private int correlationId_;
/**
* required int32 correlationId = 1;
*/
public boolean hasCorrelationId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int32 correlationId = 1;
*/
public int getCorrelationId() {
return correlationId_;
}
private void initFields() {
correlationId_ = 0;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasCorrelationId()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt32(1, correlationId_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, correlationId_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code RpcCancel}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancelOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_RpcCancel_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_RpcCancel_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel.class, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel.Builder.class);
}
// Construct using com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
correlationId_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_RpcCancel_descriptor;
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel getDefaultInstanceForType() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel.getDefaultInstance();
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel build() {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel buildPartial() {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel result = new com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.correlationId_ = correlationId_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel) {
return mergeFrom((com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel other) {
if (other == com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel.getDefaultInstance()) return this;
if (other.hasCorrelationId()) {
setCorrelationId(other.getCorrelationId());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasCorrelationId()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required int32 correlationId = 1;
private int correlationId_ ;
/**
* required int32 correlationId = 1;
*/
public boolean hasCorrelationId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int32 correlationId = 1;
*/
public int getCorrelationId() {
return correlationId_;
}
/**
* required int32 correlationId = 1;
*/
public Builder setCorrelationId(int value) {
bitField0_ |= 0x00000001;
correlationId_ = value;
onChanged();
return this;
}
/**
* required int32 correlationId = 1;
*/
public Builder clearCorrelationId() {
bitField0_ = (bitField0_ & ~0x00000001);
correlationId_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:RpcCancel)
}
static {
defaultInstance = new RpcCancel(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:RpcCancel)
}
public interface OobResponseOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required int32 correlationId = 1;
/**
* required int32 correlationId = 1;
*/
boolean hasCorrelationId();
/**
* required int32 correlationId = 1;
*/
int getCorrelationId();
// required bytes messageBytes = 2;
/**
* required bytes messageBytes = 2;
*/
boolean hasMessageBytes();
/**
* required bytes messageBytes = 2;
*/
com.google.protobuf.ByteString getMessageBytes();
}
/**
* Protobuf type {@code OobResponse}
*/
public static final class OobResponse extends
com.google.protobuf.GeneratedMessage
implements OobResponseOrBuilder {
// Use OobResponse.newBuilder() to construct.
private OobResponse(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private OobResponse(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final OobResponse defaultInstance;
public static OobResponse getDefaultInstance() {
return defaultInstance;
}
public OobResponse getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private OobResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
correlationId_ = input.readInt32();
break;
}
case 18: {
bitField0_ |= 0x00000002;
messageBytes_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_OobResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_OobResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse.class, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public OobResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new OobResponse(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required int32 correlationId = 1;
public static final int CORRELATIONID_FIELD_NUMBER = 1;
private int correlationId_;
/**
* required int32 correlationId = 1;
*/
public boolean hasCorrelationId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int32 correlationId = 1;
*/
public int getCorrelationId() {
return correlationId_;
}
// required bytes messageBytes = 2;
public static final int MESSAGEBYTES_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString messageBytes_;
/**
* required bytes messageBytes = 2;
*/
public boolean hasMessageBytes() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required bytes messageBytes = 2;
*/
public com.google.protobuf.ByteString getMessageBytes() {
return messageBytes_;
}
private void initFields() {
correlationId_ = 0;
messageBytes_ = com.google.protobuf.ByteString.EMPTY;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasCorrelationId()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasMessageBytes()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt32(1, correlationId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, messageBytes_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, correlationId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, messageBytes_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code OobResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_OobResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_OobResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse.class, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse.Builder.class);
}
// Construct using com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
correlationId_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
messageBytes_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_OobResponse_descriptor;
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse getDefaultInstanceForType() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse.getDefaultInstance();
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse build() {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse buildPartial() {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse result = new com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.correlationId_ = correlationId_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.messageBytes_ = messageBytes_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse) {
return mergeFrom((com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse other) {
if (other == com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse.getDefaultInstance()) return this;
if (other.hasCorrelationId()) {
setCorrelationId(other.getCorrelationId());
}
if (other.hasMessageBytes()) {
setMessageBytes(other.getMessageBytes());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasCorrelationId()) {
return false;
}
if (!hasMessageBytes()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required int32 correlationId = 1;
private int correlationId_ ;
/**
* required int32 correlationId = 1;
*/
public boolean hasCorrelationId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int32 correlationId = 1;
*/
public int getCorrelationId() {
return correlationId_;
}
/**
* required int32 correlationId = 1;
*/
public Builder setCorrelationId(int value) {
bitField0_ |= 0x00000001;
correlationId_ = value;
onChanged();
return this;
}
/**
* required int32 correlationId = 1;
*/
public Builder clearCorrelationId() {
bitField0_ = (bitField0_ & ~0x00000001);
correlationId_ = 0;
onChanged();
return this;
}
// required bytes messageBytes = 2;
private com.google.protobuf.ByteString messageBytes_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes messageBytes = 2;
*/
public boolean hasMessageBytes() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required bytes messageBytes = 2;
*/
public com.google.protobuf.ByteString getMessageBytes() {
return messageBytes_;
}
/**
* required bytes messageBytes = 2;
*/
public Builder setMessageBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
messageBytes_ = value;
onChanged();
return this;
}
/**
* required bytes messageBytes = 2;
*/
public Builder clearMessageBytes() {
bitField0_ = (bitField0_ & ~0x00000002);
messageBytes_ = getDefaultInstance().getMessageBytes();
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:OobResponse)
}
static {
defaultInstance = new OobResponse(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:OobResponse)
}
public interface OobMessageOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required bytes messageBytes = 1;
/**
* required bytes messageBytes = 1;
*/
boolean hasMessageBytes();
/**
* required bytes messageBytes = 1;
*/
com.google.protobuf.ByteString getMessageBytes();
}
/**
* Protobuf type {@code OobMessage}
*/
public static final class OobMessage extends
com.google.protobuf.GeneratedMessage
implements OobMessageOrBuilder {
// Use OobMessage.newBuilder() to construct.
private OobMessage(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private OobMessage(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final OobMessage defaultInstance;
public static OobMessage getDefaultInstance() {
return defaultInstance;
}
public OobMessage getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private OobMessage(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
messageBytes_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_OobMessage_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_OobMessage_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage.class, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public OobMessage parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new OobMessage(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required bytes messageBytes = 1;
public static final int MESSAGEBYTES_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString messageBytes_;
/**
* required bytes messageBytes = 1;
*/
public boolean hasMessageBytes() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes messageBytes = 1;
*/
public com.google.protobuf.ByteString getMessageBytes() {
return messageBytes_;
}
private void initFields() {
messageBytes_ = com.google.protobuf.ByteString.EMPTY;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasMessageBytes()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, messageBytes_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, messageBytes_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code OobMessage}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessageOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_OobMessage_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_OobMessage_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage.class, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage.Builder.class);
}
// Construct using com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
messageBytes_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_OobMessage_descriptor;
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage getDefaultInstanceForType() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage.getDefaultInstance();
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage build() {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage buildPartial() {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage result = new com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.messageBytes_ = messageBytes_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage) {
return mergeFrom((com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage other) {
if (other == com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage.getDefaultInstance()) return this;
if (other.hasMessageBytes()) {
setMessageBytes(other.getMessageBytes());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasMessageBytes()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required bytes messageBytes = 1;
private com.google.protobuf.ByteString messageBytes_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes messageBytes = 1;
*/
public boolean hasMessageBytes() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes messageBytes = 1;
*/
public com.google.protobuf.ByteString getMessageBytes() {
return messageBytes_;
}
/**
* required bytes messageBytes = 1;
*/
public Builder setMessageBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
messageBytes_ = value;
onChanged();
return this;
}
/**
* required bytes messageBytes = 1;
*/
public Builder clearMessageBytes() {
bitField0_ = (bitField0_ & ~0x00000001);
messageBytes_ = getDefaultInstance().getMessageBytes();
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:OobMessage)
}
static {
defaultInstance = new OobMessage(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:OobMessage)
}
public interface WirePayloadOrBuilder extends
com.google.protobuf.GeneratedMessage.
ExtendableMessageOrBuilder {
// optional .ConnectRequest connectRequest = 1;
/**
* optional .ConnectRequest connectRequest = 1;
*/
boolean hasConnectRequest();
/**
* optional .ConnectRequest connectRequest = 1;
*/
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest getConnectRequest();
/**
* optional .ConnectRequest connectRequest = 1;
*/
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequestOrBuilder getConnectRequestOrBuilder();
// optional .ConnectResponse connectResponse = 2;
/**
* optional .ConnectResponse connectResponse = 2;
*/
boolean hasConnectResponse();
/**
* optional .ConnectResponse connectResponse = 2;
*/
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse getConnectResponse();
/**
* optional .ConnectResponse connectResponse = 2;
*/
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponseOrBuilder getConnectResponseOrBuilder();
// optional .RpcRequest rpcRequest = 3;
/**
* optional .RpcRequest rpcRequest = 3;
*/
boolean hasRpcRequest();
/**
* optional .RpcRequest rpcRequest = 3;
*/
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest getRpcRequest();
/**
* optional .RpcRequest rpcRequest = 3;
*/
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequestOrBuilder getRpcRequestOrBuilder();
// optional .RpcResponse rpcResponse = 4;
/**
* optional .RpcResponse rpcResponse = 4;
*/
boolean hasRpcResponse();
/**
* optional .RpcResponse rpcResponse = 4;
*/
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse getRpcResponse();
/**
* optional .RpcResponse rpcResponse = 4;
*/
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponseOrBuilder getRpcResponseOrBuilder();
// optional .RpcError rpcError = 5;
/**
* optional .RpcError rpcError = 5;
*/
boolean hasRpcError();
/**
* optional .RpcError rpcError = 5;
*/
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError getRpcError();
/**
* optional .RpcError rpcError = 5;
*/
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcErrorOrBuilder getRpcErrorOrBuilder();
// optional .RpcCancel rpcCancel = 6;
/**
* optional .RpcCancel rpcCancel = 6;
*/
boolean hasRpcCancel();
/**
* optional .RpcCancel rpcCancel = 6;
*/
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel getRpcCancel();
/**
* optional .RpcCancel rpcCancel = 6;
*/
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancelOrBuilder getRpcCancelOrBuilder();
// optional .OobResponse oobResponse = 7;
/**
* optional .OobResponse oobResponse = 7;
*
*
* out of band responses from RPC server to client during
* the processing of a RPC call
*
*/
boolean hasOobResponse();
/**
* optional .OobResponse oobResponse = 7;
*
*
* out of band responses from RPC server to client during
* the processing of a RPC call
*
*/
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse getOobResponse();
/**
* optional .OobResponse oobResponse = 7;
*
*
* out of band responses from RPC server to client during
* the processing of a RPC call
*
*/
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponseOrBuilder getOobResponseOrBuilder();
// optional .OobMessage oobMessage = 8;
/**
* optional .OobMessage oobMessage = 8;
*
*
* bidirectional message not related to any particular RPC call.
*
*/
boolean hasOobMessage();
/**
* optional .OobMessage oobMessage = 8;
*
*
* bidirectional message not related to any particular RPC call.
*
*/
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage getOobMessage();
/**
* optional .OobMessage oobMessage = 8;
*
*
* bidirectional message not related to any particular RPC call.
*
*/
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessageOrBuilder getOobMessageOrBuilder();
// optional .OobMessage transparentMessage = 100;
/**
* optional .OobMessage transparentMessage = 100;
*
*
*Message just passed through the Pipeline and not touched.
*Reduces need of extension handling for several of customization cases.
*
*/
boolean hasTransparentMessage();
/**
* optional .OobMessage transparentMessage = 100;
*
*
*Message just passed through the Pipeline and not touched.
*Reduces need of extension handling for several of customization cases.
*
*/
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage getTransparentMessage();
/**
* optional .OobMessage transparentMessage = 100;
*
*
*Message just passed through the Pipeline and not touched.
*Reduces need of extension handling for several of customization cases.
*
*/
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessageOrBuilder getTransparentMessageOrBuilder();
}
/**
* Protobuf type {@code WirePayload}
*/
public static final class WirePayload extends
com.google.protobuf.GeneratedMessage.ExtendableMessage<
WirePayload> implements WirePayloadOrBuilder {
// Use WirePayload.newBuilder() to construct.
private WirePayload(com.google.protobuf.GeneratedMessage.ExtendableBuilder builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private WirePayload(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final WirePayload defaultInstance;
public static WirePayload getDefaultInstance() {
return defaultInstance;
}
public WirePayload getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private WirePayload(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = connectRequest_.toBuilder();
}
connectRequest_ = input.readMessage(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(connectRequest_);
connectRequest_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 18: {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
subBuilder = connectResponse_.toBuilder();
}
connectResponse_ = input.readMessage(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(connectResponse_);
connectResponse_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
case 26: {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest.Builder subBuilder = null;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
subBuilder = rpcRequest_.toBuilder();
}
rpcRequest_ = input.readMessage(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(rpcRequest_);
rpcRequest_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000004;
break;
}
case 34: {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse.Builder subBuilder = null;
if (((bitField0_ & 0x00000008) == 0x00000008)) {
subBuilder = rpcResponse_.toBuilder();
}
rpcResponse_ = input.readMessage(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(rpcResponse_);
rpcResponse_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000008;
break;
}
case 42: {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError.Builder subBuilder = null;
if (((bitField0_ & 0x00000010) == 0x00000010)) {
subBuilder = rpcError_.toBuilder();
}
rpcError_ = input.readMessage(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(rpcError_);
rpcError_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000010;
break;
}
case 50: {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel.Builder subBuilder = null;
if (((bitField0_ & 0x00000020) == 0x00000020)) {
subBuilder = rpcCancel_.toBuilder();
}
rpcCancel_ = input.readMessage(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(rpcCancel_);
rpcCancel_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000020;
break;
}
case 58: {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse.Builder subBuilder = null;
if (((bitField0_ & 0x00000040) == 0x00000040)) {
subBuilder = oobResponse_.toBuilder();
}
oobResponse_ = input.readMessage(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(oobResponse_);
oobResponse_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000040;
break;
}
case 66: {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage.Builder subBuilder = null;
if (((bitField0_ & 0x00000080) == 0x00000080)) {
subBuilder = oobMessage_.toBuilder();
}
oobMessage_ = input.readMessage(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(oobMessage_);
oobMessage_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000080;
break;
}
case 802: {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage.Builder subBuilder = null;
if (((bitField0_ & 0x00000100) == 0x00000100)) {
subBuilder = transparentMessage_.toBuilder();
}
transparentMessage_ = input.readMessage(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(transparentMessage_);
transparentMessage_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000100;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_WirePayload_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_WirePayload_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload.class, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public WirePayload parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new WirePayload(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// optional .ConnectRequest connectRequest = 1;
public static final int CONNECTREQUEST_FIELD_NUMBER = 1;
private com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest connectRequest_;
/**
* optional .ConnectRequest connectRequest = 1;
*/
public boolean hasConnectRequest() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .ConnectRequest connectRequest = 1;
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest getConnectRequest() {
return connectRequest_;
}
/**
* optional .ConnectRequest connectRequest = 1;
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequestOrBuilder getConnectRequestOrBuilder() {
return connectRequest_;
}
// optional .ConnectResponse connectResponse = 2;
public static final int CONNECTRESPONSE_FIELD_NUMBER = 2;
private com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse connectResponse_;
/**
* optional .ConnectResponse connectResponse = 2;
*/
public boolean hasConnectResponse() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .ConnectResponse connectResponse = 2;
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse getConnectResponse() {
return connectResponse_;
}
/**
* optional .ConnectResponse connectResponse = 2;
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponseOrBuilder getConnectResponseOrBuilder() {
return connectResponse_;
}
// optional .RpcRequest rpcRequest = 3;
public static final int RPCREQUEST_FIELD_NUMBER = 3;
private com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest rpcRequest_;
/**
* optional .RpcRequest rpcRequest = 3;
*/
public boolean hasRpcRequest() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .RpcRequest rpcRequest = 3;
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest getRpcRequest() {
return rpcRequest_;
}
/**
* optional .RpcRequest rpcRequest = 3;
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequestOrBuilder getRpcRequestOrBuilder() {
return rpcRequest_;
}
// optional .RpcResponse rpcResponse = 4;
public static final int RPCRESPONSE_FIELD_NUMBER = 4;
private com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse rpcResponse_;
/**
* optional .RpcResponse rpcResponse = 4;
*/
public boolean hasRpcResponse() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional .RpcResponse rpcResponse = 4;
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse getRpcResponse() {
return rpcResponse_;
}
/**
* optional .RpcResponse rpcResponse = 4;
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponseOrBuilder getRpcResponseOrBuilder() {
return rpcResponse_;
}
// optional .RpcError rpcError = 5;
public static final int RPCERROR_FIELD_NUMBER = 5;
private com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError rpcError_;
/**
* optional .RpcError rpcError = 5;
*/
public boolean hasRpcError() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional .RpcError rpcError = 5;
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError getRpcError() {
return rpcError_;
}
/**
* optional .RpcError rpcError = 5;
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcErrorOrBuilder getRpcErrorOrBuilder() {
return rpcError_;
}
// optional .RpcCancel rpcCancel = 6;
public static final int RPCCANCEL_FIELD_NUMBER = 6;
private com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel rpcCancel_;
/**
* optional .RpcCancel rpcCancel = 6;
*/
public boolean hasRpcCancel() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional .RpcCancel rpcCancel = 6;
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel getRpcCancel() {
return rpcCancel_;
}
/**
* optional .RpcCancel rpcCancel = 6;
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancelOrBuilder getRpcCancelOrBuilder() {
return rpcCancel_;
}
// optional .OobResponse oobResponse = 7;
public static final int OOBRESPONSE_FIELD_NUMBER = 7;
private com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse oobResponse_;
/**
* optional .OobResponse oobResponse = 7;
*
*
* out of band responses from RPC server to client during
* the processing of a RPC call
*
*/
public boolean hasOobResponse() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional .OobResponse oobResponse = 7;
*
*
* out of band responses from RPC server to client during
* the processing of a RPC call
*
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse getOobResponse() {
return oobResponse_;
}
/**
* optional .OobResponse oobResponse = 7;
*
*
* out of band responses from RPC server to client during
* the processing of a RPC call
*
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponseOrBuilder getOobResponseOrBuilder() {
return oobResponse_;
}
// optional .OobMessage oobMessage = 8;
public static final int OOBMESSAGE_FIELD_NUMBER = 8;
private com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage oobMessage_;
/**
* optional .OobMessage oobMessage = 8;
*
*
* bidirectional message not related to any particular RPC call.
*
*/
public boolean hasOobMessage() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional .OobMessage oobMessage = 8;
*
*
* bidirectional message not related to any particular RPC call.
*
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage getOobMessage() {
return oobMessage_;
}
/**
* optional .OobMessage oobMessage = 8;
*
*
* bidirectional message not related to any particular RPC call.
*
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessageOrBuilder getOobMessageOrBuilder() {
return oobMessage_;
}
// optional .OobMessage transparentMessage = 100;
public static final int TRANSPARENTMESSAGE_FIELD_NUMBER = 100;
private com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage transparentMessage_;
/**
* optional .OobMessage transparentMessage = 100;
*
*
*Message just passed through the Pipeline and not touched.
*Reduces need of extension handling for several of customization cases.
*
*/
public boolean hasTransparentMessage() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional .OobMessage transparentMessage = 100;
*
*
*Message just passed through the Pipeline and not touched.
*Reduces need of extension handling for several of customization cases.
*
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage getTransparentMessage() {
return transparentMessage_;
}
/**
* optional .OobMessage transparentMessage = 100;
*
*
*Message just passed through the Pipeline and not touched.
*Reduces need of extension handling for several of customization cases.
*
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessageOrBuilder getTransparentMessageOrBuilder() {
return transparentMessage_;
}
private void initFields() {
connectRequest_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest.getDefaultInstance();
connectResponse_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse.getDefaultInstance();
rpcRequest_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest.getDefaultInstance();
rpcResponse_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse.getDefaultInstance();
rpcError_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError.getDefaultInstance();
rpcCancel_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel.getDefaultInstance();
oobResponse_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse.getDefaultInstance();
oobMessage_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage.getDefaultInstance();
transparentMessage_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (hasConnectRequest()) {
if (!getConnectRequest().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasConnectResponse()) {
if (!getConnectResponse().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasRpcRequest()) {
if (!getRpcRequest().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasRpcResponse()) {
if (!getRpcResponse().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasRpcError()) {
if (!getRpcError().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasRpcCancel()) {
if (!getRpcCancel().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasOobResponse()) {
if (!getOobResponse().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasOobMessage()) {
if (!getOobMessage().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasTransparentMessage()) {
if (!getTransparentMessage().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (!extensionsAreInitialized()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
com.google.protobuf.GeneratedMessage
.ExtendableMessage.ExtensionWriter extensionWriter =
newExtensionWriter();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, connectRequest_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeMessage(2, connectResponse_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeMessage(3, rpcRequest_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeMessage(4, rpcResponse_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeMessage(5, rpcError_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeMessage(6, rpcCancel_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeMessage(7, oobResponse_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
output.writeMessage(8, oobMessage_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
output.writeMessage(100, transparentMessage_);
}
extensionWriter.writeUntil(536870912, output);
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, connectRequest_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, connectResponse_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, rpcRequest_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, rpcResponse_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, rpcError_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, rpcCancel_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, oobResponse_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, oobMessage_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(100, transparentMessage_);
}
size += extensionsSerializedSize();
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code WirePayload}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.ExtendableBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload, Builder> implements com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayloadOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_WirePayload_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_WirePayload_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload.class, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload.Builder.class);
}
// Construct using com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getConnectRequestFieldBuilder();
getConnectResponseFieldBuilder();
getRpcRequestFieldBuilder();
getRpcResponseFieldBuilder();
getRpcErrorFieldBuilder();
getRpcCancelFieldBuilder();
getOobResponseFieldBuilder();
getOobMessageFieldBuilder();
getTransparentMessageFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (connectRequestBuilder_ == null) {
connectRequest_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest.getDefaultInstance();
} else {
connectRequestBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
if (connectResponseBuilder_ == null) {
connectResponse_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse.getDefaultInstance();
} else {
connectResponseBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
if (rpcRequestBuilder_ == null) {
rpcRequest_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest.getDefaultInstance();
} else {
rpcRequestBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
if (rpcResponseBuilder_ == null) {
rpcResponse_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse.getDefaultInstance();
} else {
rpcResponseBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
if (rpcErrorBuilder_ == null) {
rpcError_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError.getDefaultInstance();
} else {
rpcErrorBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000010);
if (rpcCancelBuilder_ == null) {
rpcCancel_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel.getDefaultInstance();
} else {
rpcCancelBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000020);
if (oobResponseBuilder_ == null) {
oobResponse_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse.getDefaultInstance();
} else {
oobResponseBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000040);
if (oobMessageBuilder_ == null) {
oobMessage_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage.getDefaultInstance();
} else {
oobMessageBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000080);
if (transparentMessageBuilder_ == null) {
transparentMessage_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage.getDefaultInstance();
} else {
transparentMessageBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000100);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.internal_static_WirePayload_descriptor;
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload getDefaultInstanceForType() {
return com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload.getDefaultInstance();
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload build() {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload buildPartial() {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload result = new com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
if (connectRequestBuilder_ == null) {
result.connectRequest_ = connectRequest_;
} else {
result.connectRequest_ = connectRequestBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
if (connectResponseBuilder_ == null) {
result.connectResponse_ = connectResponse_;
} else {
result.connectResponse_ = connectResponseBuilder_.build();
}
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
if (rpcRequestBuilder_ == null) {
result.rpcRequest_ = rpcRequest_;
} else {
result.rpcRequest_ = rpcRequestBuilder_.build();
}
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
if (rpcResponseBuilder_ == null) {
result.rpcResponse_ = rpcResponse_;
} else {
result.rpcResponse_ = rpcResponseBuilder_.build();
}
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
if (rpcErrorBuilder_ == null) {
result.rpcError_ = rpcError_;
} else {
result.rpcError_ = rpcErrorBuilder_.build();
}
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
if (rpcCancelBuilder_ == null) {
result.rpcCancel_ = rpcCancel_;
} else {
result.rpcCancel_ = rpcCancelBuilder_.build();
}
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000040;
}
if (oobResponseBuilder_ == null) {
result.oobResponse_ = oobResponse_;
} else {
result.oobResponse_ = oobResponseBuilder_.build();
}
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000080;
}
if (oobMessageBuilder_ == null) {
result.oobMessage_ = oobMessage_;
} else {
result.oobMessage_ = oobMessageBuilder_.build();
}
if (((from_bitField0_ & 0x00000100) == 0x00000100)) {
to_bitField0_ |= 0x00000100;
}
if (transparentMessageBuilder_ == null) {
result.transparentMessage_ = transparentMessage_;
} else {
result.transparentMessage_ = transparentMessageBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload) {
return mergeFrom((com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload other) {
if (other == com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload.getDefaultInstance()) return this;
if (other.hasConnectRequest()) {
mergeConnectRequest(other.getConnectRequest());
}
if (other.hasConnectResponse()) {
mergeConnectResponse(other.getConnectResponse());
}
if (other.hasRpcRequest()) {
mergeRpcRequest(other.getRpcRequest());
}
if (other.hasRpcResponse()) {
mergeRpcResponse(other.getRpcResponse());
}
if (other.hasRpcError()) {
mergeRpcError(other.getRpcError());
}
if (other.hasRpcCancel()) {
mergeRpcCancel(other.getRpcCancel());
}
if (other.hasOobResponse()) {
mergeOobResponse(other.getOobResponse());
}
if (other.hasOobMessage()) {
mergeOobMessage(other.getOobMessage());
}
if (other.hasTransparentMessage()) {
mergeTransparentMessage(other.getTransparentMessage());
}
this.mergeExtensionFields(other);
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (hasConnectRequest()) {
if (!getConnectRequest().isInitialized()) {
return false;
}
}
if (hasConnectResponse()) {
if (!getConnectResponse().isInitialized()) {
return false;
}
}
if (hasRpcRequest()) {
if (!getRpcRequest().isInitialized()) {
return false;
}
}
if (hasRpcResponse()) {
if (!getRpcResponse().isInitialized()) {
return false;
}
}
if (hasRpcError()) {
if (!getRpcError().isInitialized()) {
return false;
}
}
if (hasRpcCancel()) {
if (!getRpcCancel().isInitialized()) {
return false;
}
}
if (hasOobResponse()) {
if (!getOobResponse().isInitialized()) {
return false;
}
}
if (hasOobMessage()) {
if (!getOobMessage().isInitialized()) {
return false;
}
}
if (hasTransparentMessage()) {
if (!getTransparentMessage().isInitialized()) {
return false;
}
}
if (!extensionsAreInitialized()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.WirePayload) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional .ConnectRequest connectRequest = 1;
private com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest connectRequest_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequestOrBuilder> connectRequestBuilder_;
/**
* optional .ConnectRequest connectRequest = 1;
*/
public boolean hasConnectRequest() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .ConnectRequest connectRequest = 1;
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest getConnectRequest() {
if (connectRequestBuilder_ == null) {
return connectRequest_;
} else {
return connectRequestBuilder_.getMessage();
}
}
/**
* optional .ConnectRequest connectRequest = 1;
*/
public Builder setConnectRequest(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest value) {
if (connectRequestBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
connectRequest_ = value;
onChanged();
} else {
connectRequestBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .ConnectRequest connectRequest = 1;
*/
public Builder setConnectRequest(
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest.Builder builderForValue) {
if (connectRequestBuilder_ == null) {
connectRequest_ = builderForValue.build();
onChanged();
} else {
connectRequestBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .ConnectRequest connectRequest = 1;
*/
public Builder mergeConnectRequest(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest value) {
if (connectRequestBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
connectRequest_ != com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest.getDefaultInstance()) {
connectRequest_ =
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest.newBuilder(connectRequest_).mergeFrom(value).buildPartial();
} else {
connectRequest_ = value;
}
onChanged();
} else {
connectRequestBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .ConnectRequest connectRequest = 1;
*/
public Builder clearConnectRequest() {
if (connectRequestBuilder_ == null) {
connectRequest_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest.getDefaultInstance();
onChanged();
} else {
connectRequestBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
* optional .ConnectRequest connectRequest = 1;
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest.Builder getConnectRequestBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getConnectRequestFieldBuilder().getBuilder();
}
/**
* optional .ConnectRequest connectRequest = 1;
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequestOrBuilder getConnectRequestOrBuilder() {
if (connectRequestBuilder_ != null) {
return connectRequestBuilder_.getMessageOrBuilder();
} else {
return connectRequest_;
}
}
/**
* optional .ConnectRequest connectRequest = 1;
*/
private com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequestOrBuilder>
getConnectRequestFieldBuilder() {
if (connectRequestBuilder_ == null) {
connectRequestBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequest.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectRequestOrBuilder>(
connectRequest_,
getParentForChildren(),
isClean());
connectRequest_ = null;
}
return connectRequestBuilder_;
}
// optional .ConnectResponse connectResponse = 2;
private com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse connectResponse_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponseOrBuilder> connectResponseBuilder_;
/**
* optional .ConnectResponse connectResponse = 2;
*/
public boolean hasConnectResponse() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .ConnectResponse connectResponse = 2;
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse getConnectResponse() {
if (connectResponseBuilder_ == null) {
return connectResponse_;
} else {
return connectResponseBuilder_.getMessage();
}
}
/**
* optional .ConnectResponse connectResponse = 2;
*/
public Builder setConnectResponse(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse value) {
if (connectResponseBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
connectResponse_ = value;
onChanged();
} else {
connectResponseBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .ConnectResponse connectResponse = 2;
*/
public Builder setConnectResponse(
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse.Builder builderForValue) {
if (connectResponseBuilder_ == null) {
connectResponse_ = builderForValue.build();
onChanged();
} else {
connectResponseBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .ConnectResponse connectResponse = 2;
*/
public Builder mergeConnectResponse(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse value) {
if (connectResponseBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002) &&
connectResponse_ != com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse.getDefaultInstance()) {
connectResponse_ =
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse.newBuilder(connectResponse_).mergeFrom(value).buildPartial();
} else {
connectResponse_ = value;
}
onChanged();
} else {
connectResponseBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .ConnectResponse connectResponse = 2;
*/
public Builder clearConnectResponse() {
if (connectResponseBuilder_ == null) {
connectResponse_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse.getDefaultInstance();
onChanged();
} else {
connectResponseBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
* optional .ConnectResponse connectResponse = 2;
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse.Builder getConnectResponseBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getConnectResponseFieldBuilder().getBuilder();
}
/**
* optional .ConnectResponse connectResponse = 2;
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponseOrBuilder getConnectResponseOrBuilder() {
if (connectResponseBuilder_ != null) {
return connectResponseBuilder_.getMessageOrBuilder();
} else {
return connectResponse_;
}
}
/**
* optional .ConnectResponse connectResponse = 2;
*/
private com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponseOrBuilder>
getConnectResponseFieldBuilder() {
if (connectResponseBuilder_ == null) {
connectResponseBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponse.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.ConnectResponseOrBuilder>(
connectResponse_,
getParentForChildren(),
isClean());
connectResponse_ = null;
}
return connectResponseBuilder_;
}
// optional .RpcRequest rpcRequest = 3;
private com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest rpcRequest_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequestOrBuilder> rpcRequestBuilder_;
/**
* optional .RpcRequest rpcRequest = 3;
*/
public boolean hasRpcRequest() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .RpcRequest rpcRequest = 3;
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest getRpcRequest() {
if (rpcRequestBuilder_ == null) {
return rpcRequest_;
} else {
return rpcRequestBuilder_.getMessage();
}
}
/**
* optional .RpcRequest rpcRequest = 3;
*/
public Builder setRpcRequest(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest value) {
if (rpcRequestBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
rpcRequest_ = value;
onChanged();
} else {
rpcRequestBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .RpcRequest rpcRequest = 3;
*/
public Builder setRpcRequest(
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest.Builder builderForValue) {
if (rpcRequestBuilder_ == null) {
rpcRequest_ = builderForValue.build();
onChanged();
} else {
rpcRequestBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .RpcRequest rpcRequest = 3;
*/
public Builder mergeRpcRequest(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest value) {
if (rpcRequestBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004) &&
rpcRequest_ != com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest.getDefaultInstance()) {
rpcRequest_ =
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest.newBuilder(rpcRequest_).mergeFrom(value).buildPartial();
} else {
rpcRequest_ = value;
}
onChanged();
} else {
rpcRequestBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .RpcRequest rpcRequest = 3;
*/
public Builder clearRpcRequest() {
if (rpcRequestBuilder_ == null) {
rpcRequest_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest.getDefaultInstance();
onChanged();
} else {
rpcRequestBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
/**
* optional .RpcRequest rpcRequest = 3;
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest.Builder getRpcRequestBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getRpcRequestFieldBuilder().getBuilder();
}
/**
* optional .RpcRequest rpcRequest = 3;
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequestOrBuilder getRpcRequestOrBuilder() {
if (rpcRequestBuilder_ != null) {
return rpcRequestBuilder_.getMessageOrBuilder();
} else {
return rpcRequest_;
}
}
/**
* optional .RpcRequest rpcRequest = 3;
*/
private com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequestOrBuilder>
getRpcRequestFieldBuilder() {
if (rpcRequestBuilder_ == null) {
rpcRequestBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequest.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcRequestOrBuilder>(
rpcRequest_,
getParentForChildren(),
isClean());
rpcRequest_ = null;
}
return rpcRequestBuilder_;
}
// optional .RpcResponse rpcResponse = 4;
private com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse rpcResponse_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponseOrBuilder> rpcResponseBuilder_;
/**
* optional .RpcResponse rpcResponse = 4;
*/
public boolean hasRpcResponse() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional .RpcResponse rpcResponse = 4;
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse getRpcResponse() {
if (rpcResponseBuilder_ == null) {
return rpcResponse_;
} else {
return rpcResponseBuilder_.getMessage();
}
}
/**
* optional .RpcResponse rpcResponse = 4;
*/
public Builder setRpcResponse(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse value) {
if (rpcResponseBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
rpcResponse_ = value;
onChanged();
} else {
rpcResponseBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .RpcResponse rpcResponse = 4;
*/
public Builder setRpcResponse(
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse.Builder builderForValue) {
if (rpcResponseBuilder_ == null) {
rpcResponse_ = builderForValue.build();
onChanged();
} else {
rpcResponseBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .RpcResponse rpcResponse = 4;
*/
public Builder mergeRpcResponse(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse value) {
if (rpcResponseBuilder_ == null) {
if (((bitField0_ & 0x00000008) == 0x00000008) &&
rpcResponse_ != com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse.getDefaultInstance()) {
rpcResponse_ =
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse.newBuilder(rpcResponse_).mergeFrom(value).buildPartial();
} else {
rpcResponse_ = value;
}
onChanged();
} else {
rpcResponseBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .RpcResponse rpcResponse = 4;
*/
public Builder clearRpcResponse() {
if (rpcResponseBuilder_ == null) {
rpcResponse_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse.getDefaultInstance();
onChanged();
} else {
rpcResponseBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
/**
* optional .RpcResponse rpcResponse = 4;
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse.Builder getRpcResponseBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getRpcResponseFieldBuilder().getBuilder();
}
/**
* optional .RpcResponse rpcResponse = 4;
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponseOrBuilder getRpcResponseOrBuilder() {
if (rpcResponseBuilder_ != null) {
return rpcResponseBuilder_.getMessageOrBuilder();
} else {
return rpcResponse_;
}
}
/**
* optional .RpcResponse rpcResponse = 4;
*/
private com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponseOrBuilder>
getRpcResponseFieldBuilder() {
if (rpcResponseBuilder_ == null) {
rpcResponseBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponse.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcResponseOrBuilder>(
rpcResponse_,
getParentForChildren(),
isClean());
rpcResponse_ = null;
}
return rpcResponseBuilder_;
}
// optional .RpcError rpcError = 5;
private com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError rpcError_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcErrorOrBuilder> rpcErrorBuilder_;
/**
* optional .RpcError rpcError = 5;
*/
public boolean hasRpcError() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional .RpcError rpcError = 5;
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError getRpcError() {
if (rpcErrorBuilder_ == null) {
return rpcError_;
} else {
return rpcErrorBuilder_.getMessage();
}
}
/**
* optional .RpcError rpcError = 5;
*/
public Builder setRpcError(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError value) {
if (rpcErrorBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
rpcError_ = value;
onChanged();
} else {
rpcErrorBuilder_.setMessage(value);
}
bitField0_ |= 0x00000010;
return this;
}
/**
* optional .RpcError rpcError = 5;
*/
public Builder setRpcError(
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError.Builder builderForValue) {
if (rpcErrorBuilder_ == null) {
rpcError_ = builderForValue.build();
onChanged();
} else {
rpcErrorBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000010;
return this;
}
/**
* optional .RpcError rpcError = 5;
*/
public Builder mergeRpcError(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError value) {
if (rpcErrorBuilder_ == null) {
if (((bitField0_ & 0x00000010) == 0x00000010) &&
rpcError_ != com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError.getDefaultInstance()) {
rpcError_ =
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError.newBuilder(rpcError_).mergeFrom(value).buildPartial();
} else {
rpcError_ = value;
}
onChanged();
} else {
rpcErrorBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000010;
return this;
}
/**
* optional .RpcError rpcError = 5;
*/
public Builder clearRpcError() {
if (rpcErrorBuilder_ == null) {
rpcError_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError.getDefaultInstance();
onChanged();
} else {
rpcErrorBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
/**
* optional .RpcError rpcError = 5;
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError.Builder getRpcErrorBuilder() {
bitField0_ |= 0x00000010;
onChanged();
return getRpcErrorFieldBuilder().getBuilder();
}
/**
* optional .RpcError rpcError = 5;
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcErrorOrBuilder getRpcErrorOrBuilder() {
if (rpcErrorBuilder_ != null) {
return rpcErrorBuilder_.getMessageOrBuilder();
} else {
return rpcError_;
}
}
/**
* optional .RpcError rpcError = 5;
*/
private com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcErrorOrBuilder>
getRpcErrorFieldBuilder() {
if (rpcErrorBuilder_ == null) {
rpcErrorBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcError.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcErrorOrBuilder>(
rpcError_,
getParentForChildren(),
isClean());
rpcError_ = null;
}
return rpcErrorBuilder_;
}
// optional .RpcCancel rpcCancel = 6;
private com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel rpcCancel_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancelOrBuilder> rpcCancelBuilder_;
/**
* optional .RpcCancel rpcCancel = 6;
*/
public boolean hasRpcCancel() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional .RpcCancel rpcCancel = 6;
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel getRpcCancel() {
if (rpcCancelBuilder_ == null) {
return rpcCancel_;
} else {
return rpcCancelBuilder_.getMessage();
}
}
/**
* optional .RpcCancel rpcCancel = 6;
*/
public Builder setRpcCancel(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel value) {
if (rpcCancelBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
rpcCancel_ = value;
onChanged();
} else {
rpcCancelBuilder_.setMessage(value);
}
bitField0_ |= 0x00000020;
return this;
}
/**
* optional .RpcCancel rpcCancel = 6;
*/
public Builder setRpcCancel(
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel.Builder builderForValue) {
if (rpcCancelBuilder_ == null) {
rpcCancel_ = builderForValue.build();
onChanged();
} else {
rpcCancelBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000020;
return this;
}
/**
* optional .RpcCancel rpcCancel = 6;
*/
public Builder mergeRpcCancel(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel value) {
if (rpcCancelBuilder_ == null) {
if (((bitField0_ & 0x00000020) == 0x00000020) &&
rpcCancel_ != com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel.getDefaultInstance()) {
rpcCancel_ =
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel.newBuilder(rpcCancel_).mergeFrom(value).buildPartial();
} else {
rpcCancel_ = value;
}
onChanged();
} else {
rpcCancelBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000020;
return this;
}
/**
* optional .RpcCancel rpcCancel = 6;
*/
public Builder clearRpcCancel() {
if (rpcCancelBuilder_ == null) {
rpcCancel_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel.getDefaultInstance();
onChanged();
} else {
rpcCancelBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000020);
return this;
}
/**
* optional .RpcCancel rpcCancel = 6;
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel.Builder getRpcCancelBuilder() {
bitField0_ |= 0x00000020;
onChanged();
return getRpcCancelFieldBuilder().getBuilder();
}
/**
* optional .RpcCancel rpcCancel = 6;
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancelOrBuilder getRpcCancelOrBuilder() {
if (rpcCancelBuilder_ != null) {
return rpcCancelBuilder_.getMessageOrBuilder();
} else {
return rpcCancel_;
}
}
/**
* optional .RpcCancel rpcCancel = 6;
*/
private com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancelOrBuilder>
getRpcCancelFieldBuilder() {
if (rpcCancelBuilder_ == null) {
rpcCancelBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancel.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.RpcCancelOrBuilder>(
rpcCancel_,
getParentForChildren(),
isClean());
rpcCancel_ = null;
}
return rpcCancelBuilder_;
}
// optional .OobResponse oobResponse = 7;
private com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse oobResponse_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponseOrBuilder> oobResponseBuilder_;
/**
* optional .OobResponse oobResponse = 7;
*
*
* out of band responses from RPC server to client during
* the processing of a RPC call
*
*/
public boolean hasOobResponse() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional .OobResponse oobResponse = 7;
*
*
* out of band responses from RPC server to client during
* the processing of a RPC call
*
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse getOobResponse() {
if (oobResponseBuilder_ == null) {
return oobResponse_;
} else {
return oobResponseBuilder_.getMessage();
}
}
/**
* optional .OobResponse oobResponse = 7;
*
*
* out of band responses from RPC server to client during
* the processing of a RPC call
*
*/
public Builder setOobResponse(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse value) {
if (oobResponseBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
oobResponse_ = value;
onChanged();
} else {
oobResponseBuilder_.setMessage(value);
}
bitField0_ |= 0x00000040;
return this;
}
/**
* optional .OobResponse oobResponse = 7;
*
*
* out of band responses from RPC server to client during
* the processing of a RPC call
*
*/
public Builder setOobResponse(
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse.Builder builderForValue) {
if (oobResponseBuilder_ == null) {
oobResponse_ = builderForValue.build();
onChanged();
} else {
oobResponseBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000040;
return this;
}
/**
* optional .OobResponse oobResponse = 7;
*
*
* out of band responses from RPC server to client during
* the processing of a RPC call
*
*/
public Builder mergeOobResponse(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse value) {
if (oobResponseBuilder_ == null) {
if (((bitField0_ & 0x00000040) == 0x00000040) &&
oobResponse_ != com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse.getDefaultInstance()) {
oobResponse_ =
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse.newBuilder(oobResponse_).mergeFrom(value).buildPartial();
} else {
oobResponse_ = value;
}
onChanged();
} else {
oobResponseBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000040;
return this;
}
/**
* optional .OobResponse oobResponse = 7;
*
*
* out of band responses from RPC server to client during
* the processing of a RPC call
*
*/
public Builder clearOobResponse() {
if (oobResponseBuilder_ == null) {
oobResponse_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse.getDefaultInstance();
onChanged();
} else {
oobResponseBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000040);
return this;
}
/**
* optional .OobResponse oobResponse = 7;
*
*
* out of band responses from RPC server to client during
* the processing of a RPC call
*
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse.Builder getOobResponseBuilder() {
bitField0_ |= 0x00000040;
onChanged();
return getOobResponseFieldBuilder().getBuilder();
}
/**
* optional .OobResponse oobResponse = 7;
*
*
* out of band responses from RPC server to client during
* the processing of a RPC call
*
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponseOrBuilder getOobResponseOrBuilder() {
if (oobResponseBuilder_ != null) {
return oobResponseBuilder_.getMessageOrBuilder();
} else {
return oobResponse_;
}
}
/**
* optional .OobResponse oobResponse = 7;
*
*
* out of band responses from RPC server to client during
* the processing of a RPC call
*
*/
private com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponseOrBuilder>
getOobResponseFieldBuilder() {
if (oobResponseBuilder_ == null) {
oobResponseBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponse.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobResponseOrBuilder>(
oobResponse_,
getParentForChildren(),
isClean());
oobResponse_ = null;
}
return oobResponseBuilder_;
}
// optional .OobMessage oobMessage = 8;
private com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage oobMessage_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessageOrBuilder> oobMessageBuilder_;
/**
* optional .OobMessage oobMessage = 8;
*
*
* bidirectional message not related to any particular RPC call.
*
*/
public boolean hasOobMessage() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional .OobMessage oobMessage = 8;
*
*
* bidirectional message not related to any particular RPC call.
*
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage getOobMessage() {
if (oobMessageBuilder_ == null) {
return oobMessage_;
} else {
return oobMessageBuilder_.getMessage();
}
}
/**
* optional .OobMessage oobMessage = 8;
*
*
* bidirectional message not related to any particular RPC call.
*
*/
public Builder setOobMessage(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage value) {
if (oobMessageBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
oobMessage_ = value;
onChanged();
} else {
oobMessageBuilder_.setMessage(value);
}
bitField0_ |= 0x00000080;
return this;
}
/**
* optional .OobMessage oobMessage = 8;
*
*
* bidirectional message not related to any particular RPC call.
*
*/
public Builder setOobMessage(
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage.Builder builderForValue) {
if (oobMessageBuilder_ == null) {
oobMessage_ = builderForValue.build();
onChanged();
} else {
oobMessageBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000080;
return this;
}
/**
* optional .OobMessage oobMessage = 8;
*
*
* bidirectional message not related to any particular RPC call.
*
*/
public Builder mergeOobMessage(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage value) {
if (oobMessageBuilder_ == null) {
if (((bitField0_ & 0x00000080) == 0x00000080) &&
oobMessage_ != com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage.getDefaultInstance()) {
oobMessage_ =
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage.newBuilder(oobMessage_).mergeFrom(value).buildPartial();
} else {
oobMessage_ = value;
}
onChanged();
} else {
oobMessageBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000080;
return this;
}
/**
* optional .OobMessage oobMessage = 8;
*
*
* bidirectional message not related to any particular RPC call.
*
*/
public Builder clearOobMessage() {
if (oobMessageBuilder_ == null) {
oobMessage_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage.getDefaultInstance();
onChanged();
} else {
oobMessageBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000080);
return this;
}
/**
* optional .OobMessage oobMessage = 8;
*
*
* bidirectional message not related to any particular RPC call.
*
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage.Builder getOobMessageBuilder() {
bitField0_ |= 0x00000080;
onChanged();
return getOobMessageFieldBuilder().getBuilder();
}
/**
* optional .OobMessage oobMessage = 8;
*
*
* bidirectional message not related to any particular RPC call.
*
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessageOrBuilder getOobMessageOrBuilder() {
if (oobMessageBuilder_ != null) {
return oobMessageBuilder_.getMessageOrBuilder();
} else {
return oobMessage_;
}
}
/**
* optional .OobMessage oobMessage = 8;
*
*
* bidirectional message not related to any particular RPC call.
*
*/
private com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessageOrBuilder>
getOobMessageFieldBuilder() {
if (oobMessageBuilder_ == null) {
oobMessageBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessageOrBuilder>(
oobMessage_,
getParentForChildren(),
isClean());
oobMessage_ = null;
}
return oobMessageBuilder_;
}
// optional .OobMessage transparentMessage = 100;
private com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage transparentMessage_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessageOrBuilder> transparentMessageBuilder_;
/**
* optional .OobMessage transparentMessage = 100;
*
*
*Message just passed through the Pipeline and not touched.
*Reduces need of extension handling for several of customization cases.
*
*/
public boolean hasTransparentMessage() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional .OobMessage transparentMessage = 100;
*
*
*Message just passed through the Pipeline and not touched.
*Reduces need of extension handling for several of customization cases.
*
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage getTransparentMessage() {
if (transparentMessageBuilder_ == null) {
return transparentMessage_;
} else {
return transparentMessageBuilder_.getMessage();
}
}
/**
* optional .OobMessage transparentMessage = 100;
*
*
*Message just passed through the Pipeline and not touched.
*Reduces need of extension handling for several of customization cases.
*
*/
public Builder setTransparentMessage(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage value) {
if (transparentMessageBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
transparentMessage_ = value;
onChanged();
} else {
transparentMessageBuilder_.setMessage(value);
}
bitField0_ |= 0x00000100;
return this;
}
/**
* optional .OobMessage transparentMessage = 100;
*
*
*Message just passed through the Pipeline and not touched.
*Reduces need of extension handling for several of customization cases.
*
*/
public Builder setTransparentMessage(
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage.Builder builderForValue) {
if (transparentMessageBuilder_ == null) {
transparentMessage_ = builderForValue.build();
onChanged();
} else {
transparentMessageBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000100;
return this;
}
/**
* optional .OobMessage transparentMessage = 100;
*
*
*Message just passed through the Pipeline and not touched.
*Reduces need of extension handling for several of customization cases.
*
*/
public Builder mergeTransparentMessage(com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage value) {
if (transparentMessageBuilder_ == null) {
if (((bitField0_ & 0x00000100) == 0x00000100) &&
transparentMessage_ != com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage.getDefaultInstance()) {
transparentMessage_ =
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage.newBuilder(transparentMessage_).mergeFrom(value).buildPartial();
} else {
transparentMessage_ = value;
}
onChanged();
} else {
transparentMessageBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000100;
return this;
}
/**
* optional .OobMessage transparentMessage = 100;
*
*
*Message just passed through the Pipeline and not touched.
*Reduces need of extension handling for several of customization cases.
*
*/
public Builder clearTransparentMessage() {
if (transparentMessageBuilder_ == null) {
transparentMessage_ = com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage.getDefaultInstance();
onChanged();
} else {
transparentMessageBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000100);
return this;
}
/**
* optional .OobMessage transparentMessage = 100;
*
*
*Message just passed through the Pipeline and not touched.
*Reduces need of extension handling for several of customization cases.
*
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage.Builder getTransparentMessageBuilder() {
bitField0_ |= 0x00000100;
onChanged();
return getTransparentMessageFieldBuilder().getBuilder();
}
/**
* optional .OobMessage transparentMessage = 100;
*
*
*Message just passed through the Pipeline and not touched.
*Reduces need of extension handling for several of customization cases.
*
*/
public com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessageOrBuilder getTransparentMessageOrBuilder() {
if (transparentMessageBuilder_ != null) {
return transparentMessageBuilder_.getMessageOrBuilder();
} else {
return transparentMessage_;
}
}
/**
* optional .OobMessage transparentMessage = 100;
*
*
*Message just passed through the Pipeline and not touched.
*Reduces need of extension handling for several of customization cases.
*
*/
private com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessageOrBuilder>
getTransparentMessageFieldBuilder() {
if (transparentMessageBuilder_ == null) {
transparentMessageBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessage.Builder, com.googlecode.protobuf.pro.duplex.wire.DuplexProtocol.OobMessageOrBuilder>(
transparentMessage_,
getParentForChildren(),
isClean());
transparentMessage_ = null;
}
return transparentMessageBuilder_;
}
// @@protoc_insertion_point(builder_scope:WirePayload)
}
static {
defaultInstance = new WirePayload(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:WirePayload)
}
private static com.google.protobuf.Descriptors.Descriptor
internal_static_ConnectRequest_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_ConnectRequest_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_ConnectResponse_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_ConnectResponse_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_RpcRequest_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_RpcRequest_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_RpcResponse_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_RpcResponse_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_RpcError_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_RpcError_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_RpcCancel_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_RpcCancel_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_OobResponse_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_OobResponse_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_OobMessage_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_OobMessage_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_WirePayload_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_WirePayload_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\031protobuf-rpc-duplex.proto\"x\n\016ConnectRe" +
"quest\022\025\n\rcorrelationId\030\001 \002(\005\022\026\n\016clientHo" +
"stName\030\002 \002(\t\022\022\n\nclientPort\030\003 \002(\005\022\021\n\tclie" +
"ntPID\030\004 \002(\t\022\020\n\010compress\030\005 \001(\010\"s\n\017Connect" +
"Response\022\025\n\rcorrelationId\030\001 \002(\005\022\021\n\tserve" +
"rPID\030\002 \001(\t\022$\n\terrorCode\030\003 \001(\0162\021.ConnectE" +
"rrorCode\022\020\n\010compress\030\004 \001(\010\"\201\001\n\nRpcReques" +
"t\022\025\n\rcorrelationId\030\001 \002(\005\022\031\n\021serviceIdent" +
"ifier\030\002 \002(\t\022\030\n\020methodIdentifier\030\003 \002(\t\022\024\n" +
"\014requestBytes\030\004 \002(\014\022\021\n\ttimeoutMs\030\005 \001(\005\";",
"\n\013RpcResponse\022\025\n\rcorrelationId\030\001 \002(\005\022\025\n\r" +
"responseBytes\030\002 \002(\014\"7\n\010RpcError\022\025\n\rcorre" +
"lationId\030\001 \002(\005\022\024\n\014errorMessage\030\002 \002(\t\"\"\n\t" +
"RpcCancel\022\025\n\rcorrelationId\030\001 \002(\005\":\n\013OobR" +
"esponse\022\025\n\rcorrelationId\030\001 \002(\005\022\024\n\014messag" +
"eBytes\030\002 \002(\014\"\"\n\nOobMessage\022\024\n\014messageByt" +
"es\030\001 \002(\014\"\331\002\n\013WirePayload\022\'\n\016connectReque" +
"st\030\001 \001(\0132\017.ConnectRequest\022)\n\017connectResp" +
"onse\030\002 \001(\0132\020.ConnectResponse\022\037\n\nrpcReque" +
"st\030\003 \001(\0132\013.RpcRequest\022!\n\013rpcResponse\030\004 \001",
"(\0132\014.RpcResponse\022\033\n\010rpcError\030\005 \001(\0132\t.Rpc" +
"Error\022\035\n\trpcCancel\030\006 \001(\0132\n.RpcCancel\022!\n\013" +
"oobResponse\030\007 \001(\0132\014.OobResponse\022\037\n\noobMe" +
"ssage\030\010 \001(\0132\013.OobMessage\022\'\n\022transparentM" +
"essage\030d \001(\0132\013.OobMessage*\t\010\350\007\020\200\200\200\200\002*)\n\020" +
"ConnectErrorCode\022\025\n\021ALREADY_CONNECTED\020\000B" +
";\n\'com.googlecode.protobuf.pro.duplex.wi" +
"reB\016DuplexProtocolH\001"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
internal_static_ConnectRequest_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_ConnectRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_ConnectRequest_descriptor,
new java.lang.String[] { "CorrelationId", "ClientHostName", "ClientPort", "ClientPID", "Compress", });
internal_static_ConnectResponse_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_ConnectResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_ConnectResponse_descriptor,
new java.lang.String[] { "CorrelationId", "ServerPID", "ErrorCode", "Compress", });
internal_static_RpcRequest_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_RpcRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_RpcRequest_descriptor,
new java.lang.String[] { "CorrelationId", "ServiceIdentifier", "MethodIdentifier", "RequestBytes", "TimeoutMs", });
internal_static_RpcResponse_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_RpcResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_RpcResponse_descriptor,
new java.lang.String[] { "CorrelationId", "ResponseBytes", });
internal_static_RpcError_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_RpcError_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_RpcError_descriptor,
new java.lang.String[] { "CorrelationId", "ErrorMessage", });
internal_static_RpcCancel_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_RpcCancel_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_RpcCancel_descriptor,
new java.lang.String[] { "CorrelationId", });
internal_static_OobResponse_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_OobResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_OobResponse_descriptor,
new java.lang.String[] { "CorrelationId", "MessageBytes", });
internal_static_OobMessage_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_OobMessage_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_OobMessage_descriptor,
new java.lang.String[] { "MessageBytes", });
internal_static_WirePayload_descriptor =
getDescriptor().getMessageTypes().get(8);
internal_static_WirePayload_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_WirePayload_descriptor,
new java.lang.String[] { "ConnectRequest", "ConnectResponse", "RpcRequest", "RpcResponse", "RpcError", "RpcCancel", "OobResponse", "OobMessage", "TransparentMessage", });
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
}, assigner);
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy