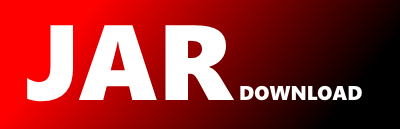
com.googlecode.protobuf.pro.stream.logging.StreamLogEntry Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of protobuf-streamer-pro Show documentation
Show all versions of protobuf-streamer-pro Show documentation
Protobuf Streamer Pro is a java protocol buffers is a google protocol buffers transport for large streamed messages featuring multiplexed sending over reused TCP connections.
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: protobuf-stream-log.proto
package com.googlecode.protobuf.pro.stream.logging;
public final class StreamLogEntry {
private StreamLogEntry() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
}
public enum TransferType
implements com.google.protobuf.ProtocolMessageEnum {
PUSH(0, 1),
PULL(1, 2),
;
public static final int PUSH_VALUE = 1;
public static final int PULL_VALUE = 2;
public final int getNumber() { return value; }
public static TransferType valueOf(int value) {
switch (value) {
case 1: return PUSH;
case 2: return PULL;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public TransferType findValueByNumber(int number) {
return TransferType.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.getDescriptor().getEnumTypes().get(0);
}
private static final TransferType[] VALUES = {
PUSH, PULL,
};
public static TransferType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private TransferType(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:TransferType)
}
public interface ParameterOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required string name = 1;
boolean hasName();
String getName();
// required string value = 2;
boolean hasValue();
String getValue();
}
public static final class Parameter extends
com.google.protobuf.GeneratedMessage
implements ParameterOrBuilder {
// Use Parameter.newBuilder() to construct.
private Parameter(Builder builder) {
super(builder);
}
private Parameter(boolean noInit) {}
private static final Parameter defaultInstance;
public static Parameter getDefaultInstance() {
return defaultInstance;
}
public Parameter getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.internal_static_Parameter_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.internal_static_Parameter_fieldAccessorTable;
}
private int bitField0_;
// required string name = 1;
public static final int NAME_FIELD_NUMBER = 1;
private java.lang.Object name_;
public boolean hasName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public String getName() {
java.lang.Object ref = name_;
if (ref instanceof String) {
return (String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
if (com.google.protobuf.Internal.isValidUtf8(bs)) {
name_ = s;
}
return s;
}
}
private com.google.protobuf.ByteString getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// required string value = 2;
public static final int VALUE_FIELD_NUMBER = 2;
private java.lang.Object value_;
public boolean hasValue() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
public String getValue() {
java.lang.Object ref = value_;
if (ref instanceof String) {
return (String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
if (com.google.protobuf.Internal.isValidUtf8(bs)) {
value_ = s;
}
return s;
}
}
private com.google.protobuf.ByteString getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private void initFields() {
name_ = "";
value_ = "";
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasName()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasValue()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getNameBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getValueBytes());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getNameBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getValueBytes());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.ParameterOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.internal_static_Parameter_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.internal_static_Parameter_fieldAccessorTable;
}
// Construct using com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
name_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
value_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter.getDescriptor();
}
public com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter getDefaultInstanceForType() {
return com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter.getDefaultInstance();
}
public com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter build() {
com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
private com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return result;
}
public com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter buildPartial() {
com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter result = new com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.name_ = name_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.value_ = value_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter) {
return mergeFrom((com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter other) {
if (other == com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter.getDefaultInstance()) return this;
if (other.hasName()) {
setName(other.getName());
}
if (other.hasValue()) {
setValue(other.getValue());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasName()) {
return false;
}
if (!hasValue()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
name_ = input.readBytes();
break;
}
case 18: {
bitField0_ |= 0x00000002;
value_ = input.readBytes();
break;
}
}
}
}
private int bitField0_;
// required string name = 1;
private java.lang.Object name_ = "";
public boolean hasName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof String)) {
String s = ((com.google.protobuf.ByteString) ref).toStringUtf8();
name_ = s;
return s;
} else {
return (String) ref;
}
}
public Builder setName(String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000001);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
void setName(com.google.protobuf.ByteString value) {
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
}
// required string value = 2;
private java.lang.Object value_ = "";
public boolean hasValue() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
public String getValue() {
java.lang.Object ref = value_;
if (!(ref instanceof String)) {
String s = ((com.google.protobuf.ByteString) ref).toStringUtf8();
value_ = s;
return s;
} else {
return (String) ref;
}
}
public Builder setValue(String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
value_ = value;
onChanged();
return this;
}
public Builder clearValue() {
bitField0_ = (bitField0_ & ~0x00000002);
value_ = getDefaultInstance().getValue();
onChanged();
return this;
}
void setValue(com.google.protobuf.ByteString value) {
bitField0_ |= 0x00000002;
value_ = value;
onChanged();
}
// @@protoc_insertion_point(builder_scope:Parameter)
}
static {
defaultInstance = new Parameter(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:Parameter)
}
public interface StreamTransferOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required .TransferType transferType = 1;
boolean hasTransferType();
com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.TransferType getTransferType();
// required string requestType = 2;
boolean hasRequestType();
String getRequestType();
// required int32 duration = 3;
boolean hasDuration();
int getDuration();
// required string server = 4;
boolean hasServer();
String getServer();
// required string client = 5;
boolean hasClient();
String getClient();
// required int32 corId = 6;
boolean hasCorId();
int getCorId();
// optional string error = 7;
boolean hasError();
String getError();
// required int64 startTS = 8;
boolean hasStartTS();
long getStartTS();
// optional int32 sizeBytes = 9;
boolean hasSizeBytes();
int getSizeBytes();
// repeated .Parameter parameter = 10;
java.util.List
getParameterList();
com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter getParameter(int index);
int getParameterCount();
java.util.List extends com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.ParameterOrBuilder>
getParameterOrBuilderList();
com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.ParameterOrBuilder getParameterOrBuilder(
int index);
}
public static final class StreamTransfer extends
com.google.protobuf.GeneratedMessage
implements StreamTransferOrBuilder {
// Use StreamTransfer.newBuilder() to construct.
private StreamTransfer(Builder builder) {
super(builder);
}
private StreamTransfer(boolean noInit) {}
private static final StreamTransfer defaultInstance;
public static StreamTransfer getDefaultInstance() {
return defaultInstance;
}
public StreamTransfer getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.internal_static_StreamTransfer_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.internal_static_StreamTransfer_fieldAccessorTable;
}
private int bitField0_;
// required .TransferType transferType = 1;
public static final int TRANSFERTYPE_FIELD_NUMBER = 1;
private com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.TransferType transferType_;
public boolean hasTransferType() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.TransferType getTransferType() {
return transferType_;
}
// required string requestType = 2;
public static final int REQUESTTYPE_FIELD_NUMBER = 2;
private java.lang.Object requestType_;
public boolean hasRequestType() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
public String getRequestType() {
java.lang.Object ref = requestType_;
if (ref instanceof String) {
return (String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
if (com.google.protobuf.Internal.isValidUtf8(bs)) {
requestType_ = s;
}
return s;
}
}
private com.google.protobuf.ByteString getRequestTypeBytes() {
java.lang.Object ref = requestType_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((String) ref);
requestType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// required int32 duration = 3;
public static final int DURATION_FIELD_NUMBER = 3;
private int duration_;
public boolean hasDuration() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
public int getDuration() {
return duration_;
}
// required string server = 4;
public static final int SERVER_FIELD_NUMBER = 4;
private java.lang.Object server_;
public boolean hasServer() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
public String getServer() {
java.lang.Object ref = server_;
if (ref instanceof String) {
return (String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
if (com.google.protobuf.Internal.isValidUtf8(bs)) {
server_ = s;
}
return s;
}
}
private com.google.protobuf.ByteString getServerBytes() {
java.lang.Object ref = server_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((String) ref);
server_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// required string client = 5;
public static final int CLIENT_FIELD_NUMBER = 5;
private java.lang.Object client_;
public boolean hasClient() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
public String getClient() {
java.lang.Object ref = client_;
if (ref instanceof String) {
return (String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
if (com.google.protobuf.Internal.isValidUtf8(bs)) {
client_ = s;
}
return s;
}
}
private com.google.protobuf.ByteString getClientBytes() {
java.lang.Object ref = client_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((String) ref);
client_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// required int32 corId = 6;
public static final int CORID_FIELD_NUMBER = 6;
private int corId_;
public boolean hasCorId() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
public int getCorId() {
return corId_;
}
// optional string error = 7;
public static final int ERROR_FIELD_NUMBER = 7;
private java.lang.Object error_;
public boolean hasError() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
public String getError() {
java.lang.Object ref = error_;
if (ref instanceof String) {
return (String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
if (com.google.protobuf.Internal.isValidUtf8(bs)) {
error_ = s;
}
return s;
}
}
private com.google.protobuf.ByteString getErrorBytes() {
java.lang.Object ref = error_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((String) ref);
error_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// required int64 startTS = 8;
public static final int STARTTS_FIELD_NUMBER = 8;
private long startTS_;
public boolean hasStartTS() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
public long getStartTS() {
return startTS_;
}
// optional int32 sizeBytes = 9;
public static final int SIZEBYTES_FIELD_NUMBER = 9;
private int sizeBytes_;
public boolean hasSizeBytes() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
public int getSizeBytes() {
return sizeBytes_;
}
// repeated .Parameter parameter = 10;
public static final int PARAMETER_FIELD_NUMBER = 10;
private java.util.List parameter_;
public java.util.List getParameterList() {
return parameter_;
}
public java.util.List extends com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.ParameterOrBuilder>
getParameterOrBuilderList() {
return parameter_;
}
public int getParameterCount() {
return parameter_.size();
}
public com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter getParameter(int index) {
return parameter_.get(index);
}
public com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.ParameterOrBuilder getParameterOrBuilder(
int index) {
return parameter_.get(index);
}
private void initFields() {
transferType_ = com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.TransferType.PUSH;
requestType_ = "";
duration_ = 0;
server_ = "";
client_ = "";
corId_ = 0;
error_ = "";
startTS_ = 0L;
sizeBytes_ = 0;
parameter_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasTransferType()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasRequestType()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasDuration()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasServer()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasClient()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasCorId()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasStartTS()) {
memoizedIsInitialized = 0;
return false;
}
for (int i = 0; i < getParameterCount(); i++) {
if (!getParameter(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeEnum(1, transferType_.getNumber());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getRequestTypeBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeInt32(3, duration_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBytes(4, getServerBytes());
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeBytes(5, getClientBytes());
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeInt32(6, corId_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeBytes(7, getErrorBytes());
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
output.writeInt64(8, startTS_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
output.writeInt32(9, sizeBytes_);
}
for (int i = 0; i < parameter_.size(); i++) {
output.writeMessage(10, parameter_.get(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, transferType_.getNumber());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getRequestTypeBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, duration_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, getServerBytes());
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(5, getClientBytes());
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(6, corId_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(7, getErrorBytes());
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(8, startTS_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(9, sizeBytes_);
}
for (int i = 0; i < parameter_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, parameter_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.StreamTransfer parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.StreamTransfer parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.StreamTransfer parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.StreamTransfer parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.StreamTransfer parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.StreamTransfer parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.StreamTransfer parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.StreamTransfer parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.StreamTransfer parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.StreamTransfer parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.StreamTransfer prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.StreamTransferOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.internal_static_StreamTransfer_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.internal_static_StreamTransfer_fieldAccessorTable;
}
// Construct using com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.StreamTransfer.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getParameterFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
transferType_ = com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.TransferType.PUSH;
bitField0_ = (bitField0_ & ~0x00000001);
requestType_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
duration_ = 0;
bitField0_ = (bitField0_ & ~0x00000004);
server_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
client_ = "";
bitField0_ = (bitField0_ & ~0x00000010);
corId_ = 0;
bitField0_ = (bitField0_ & ~0x00000020);
error_ = "";
bitField0_ = (bitField0_ & ~0x00000040);
startTS_ = 0L;
bitField0_ = (bitField0_ & ~0x00000080);
sizeBytes_ = 0;
bitField0_ = (bitField0_ & ~0x00000100);
if (parameterBuilder_ == null) {
parameter_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000200);
} else {
parameterBuilder_.clear();
}
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.StreamTransfer.getDescriptor();
}
public com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.StreamTransfer getDefaultInstanceForType() {
return com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.StreamTransfer.getDefaultInstance();
}
public com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.StreamTransfer build() {
com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.StreamTransfer result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
private com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.StreamTransfer buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.StreamTransfer result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return result;
}
public com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.StreamTransfer buildPartial() {
com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.StreamTransfer result = new com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.StreamTransfer(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.transferType_ = transferType_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.requestType_ = requestType_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.duration_ = duration_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.server_ = server_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.client_ = client_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
result.corId_ = corId_;
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000040;
}
result.error_ = error_;
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000080;
}
result.startTS_ = startTS_;
if (((from_bitField0_ & 0x00000100) == 0x00000100)) {
to_bitField0_ |= 0x00000100;
}
result.sizeBytes_ = sizeBytes_;
if (parameterBuilder_ == null) {
if (((bitField0_ & 0x00000200) == 0x00000200)) {
parameter_ = java.util.Collections.unmodifiableList(parameter_);
bitField0_ = (bitField0_ & ~0x00000200);
}
result.parameter_ = parameter_;
} else {
result.parameter_ = parameterBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.StreamTransfer) {
return mergeFrom((com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.StreamTransfer)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.StreamTransfer other) {
if (other == com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.StreamTransfer.getDefaultInstance()) return this;
if (other.hasTransferType()) {
setTransferType(other.getTransferType());
}
if (other.hasRequestType()) {
setRequestType(other.getRequestType());
}
if (other.hasDuration()) {
setDuration(other.getDuration());
}
if (other.hasServer()) {
setServer(other.getServer());
}
if (other.hasClient()) {
setClient(other.getClient());
}
if (other.hasCorId()) {
setCorId(other.getCorId());
}
if (other.hasError()) {
setError(other.getError());
}
if (other.hasStartTS()) {
setStartTS(other.getStartTS());
}
if (other.hasSizeBytes()) {
setSizeBytes(other.getSizeBytes());
}
if (parameterBuilder_ == null) {
if (!other.parameter_.isEmpty()) {
if (parameter_.isEmpty()) {
parameter_ = other.parameter_;
bitField0_ = (bitField0_ & ~0x00000200);
} else {
ensureParameterIsMutable();
parameter_.addAll(other.parameter_);
}
onChanged();
}
} else {
if (!other.parameter_.isEmpty()) {
if (parameterBuilder_.isEmpty()) {
parameterBuilder_.dispose();
parameterBuilder_ = null;
parameter_ = other.parameter_;
bitField0_ = (bitField0_ & ~0x00000200);
parameterBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getParameterFieldBuilder() : null;
} else {
parameterBuilder_.addAllMessages(other.parameter_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasTransferType()) {
return false;
}
if (!hasRequestType()) {
return false;
}
if (!hasDuration()) {
return false;
}
if (!hasServer()) {
return false;
}
if (!hasClient()) {
return false;
}
if (!hasCorId()) {
return false;
}
if (!hasStartTS()) {
return false;
}
for (int i = 0; i < getParameterCount(); i++) {
if (!getParameter(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
}
break;
}
case 8: {
int rawValue = input.readEnum();
com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.TransferType value = com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.TransferType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(1, rawValue);
} else {
bitField0_ |= 0x00000001;
transferType_ = value;
}
break;
}
case 18: {
bitField0_ |= 0x00000002;
requestType_ = input.readBytes();
break;
}
case 24: {
bitField0_ |= 0x00000004;
duration_ = input.readInt32();
break;
}
case 34: {
bitField0_ |= 0x00000008;
server_ = input.readBytes();
break;
}
case 42: {
bitField0_ |= 0x00000010;
client_ = input.readBytes();
break;
}
case 48: {
bitField0_ |= 0x00000020;
corId_ = input.readInt32();
break;
}
case 58: {
bitField0_ |= 0x00000040;
error_ = input.readBytes();
break;
}
case 64: {
bitField0_ |= 0x00000080;
startTS_ = input.readInt64();
break;
}
case 72: {
bitField0_ |= 0x00000100;
sizeBytes_ = input.readInt32();
break;
}
case 82: {
com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter.Builder subBuilder = com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter.newBuilder();
input.readMessage(subBuilder, extensionRegistry);
addParameter(subBuilder.buildPartial());
break;
}
}
}
}
private int bitField0_;
// required .TransferType transferType = 1;
private com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.TransferType transferType_ = com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.TransferType.PUSH;
public boolean hasTransferType() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.TransferType getTransferType() {
return transferType_;
}
public Builder setTransferType(com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.TransferType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
transferType_ = value;
onChanged();
return this;
}
public Builder clearTransferType() {
bitField0_ = (bitField0_ & ~0x00000001);
transferType_ = com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.TransferType.PUSH;
onChanged();
return this;
}
// required string requestType = 2;
private java.lang.Object requestType_ = "";
public boolean hasRequestType() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
public String getRequestType() {
java.lang.Object ref = requestType_;
if (!(ref instanceof String)) {
String s = ((com.google.protobuf.ByteString) ref).toStringUtf8();
requestType_ = s;
return s;
} else {
return (String) ref;
}
}
public Builder setRequestType(String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
requestType_ = value;
onChanged();
return this;
}
public Builder clearRequestType() {
bitField0_ = (bitField0_ & ~0x00000002);
requestType_ = getDefaultInstance().getRequestType();
onChanged();
return this;
}
void setRequestType(com.google.protobuf.ByteString value) {
bitField0_ |= 0x00000002;
requestType_ = value;
onChanged();
}
// required int32 duration = 3;
private int duration_ ;
public boolean hasDuration() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
public int getDuration() {
return duration_;
}
public Builder setDuration(int value) {
bitField0_ |= 0x00000004;
duration_ = value;
onChanged();
return this;
}
public Builder clearDuration() {
bitField0_ = (bitField0_ & ~0x00000004);
duration_ = 0;
onChanged();
return this;
}
// required string server = 4;
private java.lang.Object server_ = "";
public boolean hasServer() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
public String getServer() {
java.lang.Object ref = server_;
if (!(ref instanceof String)) {
String s = ((com.google.protobuf.ByteString) ref).toStringUtf8();
server_ = s;
return s;
} else {
return (String) ref;
}
}
public Builder setServer(String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
server_ = value;
onChanged();
return this;
}
public Builder clearServer() {
bitField0_ = (bitField0_ & ~0x00000008);
server_ = getDefaultInstance().getServer();
onChanged();
return this;
}
void setServer(com.google.protobuf.ByteString value) {
bitField0_ |= 0x00000008;
server_ = value;
onChanged();
}
// required string client = 5;
private java.lang.Object client_ = "";
public boolean hasClient() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
public String getClient() {
java.lang.Object ref = client_;
if (!(ref instanceof String)) {
String s = ((com.google.protobuf.ByteString) ref).toStringUtf8();
client_ = s;
return s;
} else {
return (String) ref;
}
}
public Builder setClient(String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
client_ = value;
onChanged();
return this;
}
public Builder clearClient() {
bitField0_ = (bitField0_ & ~0x00000010);
client_ = getDefaultInstance().getClient();
onChanged();
return this;
}
void setClient(com.google.protobuf.ByteString value) {
bitField0_ |= 0x00000010;
client_ = value;
onChanged();
}
// required int32 corId = 6;
private int corId_ ;
public boolean hasCorId() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
public int getCorId() {
return corId_;
}
public Builder setCorId(int value) {
bitField0_ |= 0x00000020;
corId_ = value;
onChanged();
return this;
}
public Builder clearCorId() {
bitField0_ = (bitField0_ & ~0x00000020);
corId_ = 0;
onChanged();
return this;
}
// optional string error = 7;
private java.lang.Object error_ = "";
public boolean hasError() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
public String getError() {
java.lang.Object ref = error_;
if (!(ref instanceof String)) {
String s = ((com.google.protobuf.ByteString) ref).toStringUtf8();
error_ = s;
return s;
} else {
return (String) ref;
}
}
public Builder setError(String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
error_ = value;
onChanged();
return this;
}
public Builder clearError() {
bitField0_ = (bitField0_ & ~0x00000040);
error_ = getDefaultInstance().getError();
onChanged();
return this;
}
void setError(com.google.protobuf.ByteString value) {
bitField0_ |= 0x00000040;
error_ = value;
onChanged();
}
// required int64 startTS = 8;
private long startTS_ ;
public boolean hasStartTS() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
public long getStartTS() {
return startTS_;
}
public Builder setStartTS(long value) {
bitField0_ |= 0x00000080;
startTS_ = value;
onChanged();
return this;
}
public Builder clearStartTS() {
bitField0_ = (bitField0_ & ~0x00000080);
startTS_ = 0L;
onChanged();
return this;
}
// optional int32 sizeBytes = 9;
private int sizeBytes_ ;
public boolean hasSizeBytes() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
public int getSizeBytes() {
return sizeBytes_;
}
public Builder setSizeBytes(int value) {
bitField0_ |= 0x00000100;
sizeBytes_ = value;
onChanged();
return this;
}
public Builder clearSizeBytes() {
bitField0_ = (bitField0_ & ~0x00000100);
sizeBytes_ = 0;
onChanged();
return this;
}
// repeated .Parameter parameter = 10;
private java.util.List parameter_ =
java.util.Collections.emptyList();
private void ensureParameterIsMutable() {
if (!((bitField0_ & 0x00000200) == 0x00000200)) {
parameter_ = new java.util.ArrayList(parameter_);
bitField0_ |= 0x00000200;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter, com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter.Builder, com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.ParameterOrBuilder> parameterBuilder_;
public java.util.List getParameterList() {
if (parameterBuilder_ == null) {
return java.util.Collections.unmodifiableList(parameter_);
} else {
return parameterBuilder_.getMessageList();
}
}
public int getParameterCount() {
if (parameterBuilder_ == null) {
return parameter_.size();
} else {
return parameterBuilder_.getCount();
}
}
public com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter getParameter(int index) {
if (parameterBuilder_ == null) {
return parameter_.get(index);
} else {
return parameterBuilder_.getMessage(index);
}
}
public Builder setParameter(
int index, com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter value) {
if (parameterBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureParameterIsMutable();
parameter_.set(index, value);
onChanged();
} else {
parameterBuilder_.setMessage(index, value);
}
return this;
}
public Builder setParameter(
int index, com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter.Builder builderForValue) {
if (parameterBuilder_ == null) {
ensureParameterIsMutable();
parameter_.set(index, builderForValue.build());
onChanged();
} else {
parameterBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
public Builder addParameter(com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter value) {
if (parameterBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureParameterIsMutable();
parameter_.add(value);
onChanged();
} else {
parameterBuilder_.addMessage(value);
}
return this;
}
public Builder addParameter(
int index, com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter value) {
if (parameterBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureParameterIsMutable();
parameter_.add(index, value);
onChanged();
} else {
parameterBuilder_.addMessage(index, value);
}
return this;
}
public Builder addParameter(
com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter.Builder builderForValue) {
if (parameterBuilder_ == null) {
ensureParameterIsMutable();
parameter_.add(builderForValue.build());
onChanged();
} else {
parameterBuilder_.addMessage(builderForValue.build());
}
return this;
}
public Builder addParameter(
int index, com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter.Builder builderForValue) {
if (parameterBuilder_ == null) {
ensureParameterIsMutable();
parameter_.add(index, builderForValue.build());
onChanged();
} else {
parameterBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
public Builder addAllParameter(
java.lang.Iterable extends com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter> values) {
if (parameterBuilder_ == null) {
ensureParameterIsMutable();
super.addAll(values, parameter_);
onChanged();
} else {
parameterBuilder_.addAllMessages(values);
}
return this;
}
public Builder clearParameter() {
if (parameterBuilder_ == null) {
parameter_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000200);
onChanged();
} else {
parameterBuilder_.clear();
}
return this;
}
public Builder removeParameter(int index) {
if (parameterBuilder_ == null) {
ensureParameterIsMutable();
parameter_.remove(index);
onChanged();
} else {
parameterBuilder_.remove(index);
}
return this;
}
public com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter.Builder getParameterBuilder(
int index) {
return getParameterFieldBuilder().getBuilder(index);
}
public com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.ParameterOrBuilder getParameterOrBuilder(
int index) {
if (parameterBuilder_ == null) {
return parameter_.get(index); } else {
return parameterBuilder_.getMessageOrBuilder(index);
}
}
public java.util.List extends com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.ParameterOrBuilder>
getParameterOrBuilderList() {
if (parameterBuilder_ != null) {
return parameterBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(parameter_);
}
}
public com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter.Builder addParameterBuilder() {
return getParameterFieldBuilder().addBuilder(
com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter.getDefaultInstance());
}
public com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter.Builder addParameterBuilder(
int index) {
return getParameterFieldBuilder().addBuilder(
index, com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter.getDefaultInstance());
}
public java.util.List
getParameterBuilderList() {
return getParameterFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter, com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter.Builder, com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.ParameterOrBuilder>
getParameterFieldBuilder() {
if (parameterBuilder_ == null) {
parameterBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter, com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter.Builder, com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.ParameterOrBuilder>(
parameter_,
((bitField0_ & 0x00000200) == 0x00000200),
getParentForChildren(),
isClean());
parameter_ = null;
}
return parameterBuilder_;
}
// @@protoc_insertion_point(builder_scope:StreamTransfer)
}
static {
defaultInstance = new StreamTransfer(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:StreamTransfer)
}
private static com.google.protobuf.Descriptors.Descriptor
internal_static_Parameter_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_Parameter_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_StreamTransfer_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_StreamTransfer_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\031protobuf-stream-log.proto\"(\n\tParameter" +
"\022\014\n\004name\030\001 \002(\t\022\r\n\005value\030\002 \002(\t\"\335\001\n\016Stream" +
"Transfer\022#\n\014transferType\030\001 \002(\0162\r.Transfe" +
"rType\022\023\n\013requestType\030\002 \002(\t\022\020\n\010duration\030\003" +
" \002(\005\022\016\n\006server\030\004 \002(\t\022\016\n\006client\030\005 \002(\t\022\r\n\005" +
"corId\030\006 \002(\005\022\r\n\005error\030\007 \001(\t\022\017\n\007startTS\030\010 " +
"\002(\003\022\021\n\tsizeBytes\030\t \001(\005\022\035\n\tparameter\030\n \003(" +
"\0132\n.Parameter*\"\n\014TransferType\022\010\n\004PUSH\020\001\022" +
"\010\n\004PULL\020\002B>\n*com.googlecode.protobuf.pro" +
".stream.loggingB\016StreamLogEntryH\001"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
internal_static_Parameter_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_Parameter_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_Parameter_descriptor,
new java.lang.String[] { "Name", "Value", },
com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter.class,
com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.Parameter.Builder.class);
internal_static_StreamTransfer_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_StreamTransfer_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_StreamTransfer_descriptor,
new java.lang.String[] { "TransferType", "RequestType", "Duration", "Server", "Client", "CorId", "Error", "StartTS", "SizeBytes", "Parameter", },
com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.StreamTransfer.class,
com.googlecode.protobuf.pro.stream.logging.StreamLogEntry.StreamTransfer.Builder.class);
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
}, assigner);
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy