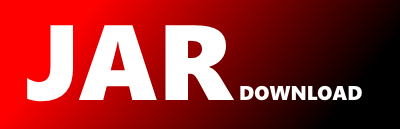
com.googlecode.protobuf.pro.stream.util.KeyStoreImportUtil Maven / Gradle / Ivy
Show all versions of protobuf-streamer-pro Show documentation
/**
* Copyright 2010 Peter Klauser
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.googlecode.protobuf.pro.stream.util;
import java.io.ByteArrayInputStream;
import java.io.DataInputStream;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.security.KeyFactory;
import java.security.KeyStore;
import java.security.PrivateKey;
import java.security.cert.Certificate;
import java.security.cert.CertificateFactory;
import java.security.spec.PKCS8EncodedKeySpec;
import java.util.Collection;
/**
* KeyStoreImportUtil.java
* - adapted from http://www.agentbob.info/agentbob/79-AB.html
*
* This class imports a (private) key and a (signed public) certificate
* into a keystore.
*
* Both the key and the certificate file must be in DER
-format.
* The private key must be encoded with PKCS#8
-format.
* The public certificate must be encoded in X.509
-format.
*
* Key format:
* openssl pkcs8 -topk8 -nocrypt -in YOUR.KEY -out YOUR.KEY.der -outform der
*
* Format of the certificate:
* openssl x509 -in YOUR.CERT -out YOUR.CERT.der -outform der
*
* Import key and certificate:
* java com.googlecode.protobuf.pro.stream.util.KeyStoreImportUtil YOUR.KEY.der YOUR.CERT.der YOUR.KEYSTORE.out keystorePass keyAlias
*
* Caution: the old YOUR.KEYSTORE.out
file is
* deleted and replaced with a keystore only containing YOUR.KEY
* and YOUR.CERT
.
*
* The keystore and the key has no password;
* to set key password use command: keytool -keypasswd
* to set keystore password use command: keytool -storepasswd
*
*/
public class KeyStoreImportUtil {
/**
* see class def
*/
public static void main ( String args[]) {
if (args.length != 5) {
System.out.println("Usage: YOUR.KEY.der YOUR.CERT.der YOUR.KEYSTORE.out keystorePass keyAlias");
System.exit(0);
}
String keyfile = args[0];
String certfile = args[1];
String keystorename = args[2];
String keypass = args[3];
String defaultalias = args[4];
try {
// initializing and clearing keystore
KeyStore ks = KeyStore.getInstance("JKS", "SUN");
ks.load( null , keypass.toCharArray());
System.out.println("Using keystore-file : "+keystorename);
ks.store(new FileOutputStream ( keystorename ),
keypass.toCharArray());
ks.load(new FileInputStream ( keystorename ),
keypass.toCharArray());
// loading Key
InputStream fl = fullStream (keyfile);
byte[] key = new byte[fl.available()];
KeyFactory kf = KeyFactory.getInstance("RSA");
fl.read ( key, 0, fl.available() );
fl.close();
PKCS8EncodedKeySpec keysp = new PKCS8EncodedKeySpec ( key );
PrivateKey ff = kf.generatePrivate (keysp);
// loading CertificateChain
CertificateFactory cf = CertificateFactory.getInstance("X.509");
InputStream certstream = fullStream (certfile);
Collection c = generateCertificates(cf, certstream);
Certificate[] certs = new Certificate[c.toArray().length];
if (c.size() == 1) {
certstream = fullStream (certfile);
System.out.println("One certificate, no chain.");
Certificate cert = cf.generateCertificate(certstream) ;
certs[0] = cert;
} else {
System.out.println("Certificate chain length: "+c.size());
certs = (Certificate[])c.toArray();
}
// storing keystore
ks.setKeyEntry(defaultalias, ff,
keypass.toCharArray(),
certs );
System.out.println ("Key and certificate stored.");
System.out.println ("Alias:"+defaultalias+" Password:"+keypass);
ks.store(new FileOutputStream ( keystorename ),
keypass.toCharArray());
} catch (Exception ex) {
ex.printStackTrace();
}
}
@SuppressWarnings("unchecked")
private static Collection generateCertificates(CertificateFactory cf, InputStream certstream)
throws Exception {
return (Collection) cf.generateCertificates(certstream) ;
}
/**
* Creates an InputStream from a file, and fills it with the complete
* file. Thus, available() on the returned InputStream will return the
* full number of bytes the file contains
* @param fname The filename
* @return The filled InputStream
* @exception IOException, if the Streams couldn't be created.
**/
private static InputStream fullStream ( String fname ) throws IOException {
FileInputStream fis = new FileInputStream(fname);
DataInputStream dis = new DataInputStream(fis);
byte[] bytes = new byte[dis.available()];
dis.readFully(bytes);
ByteArrayInputStream bais = new ByteArrayInputStream(bytes);
return bais;
}
}