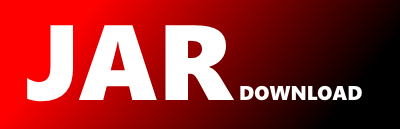
com.googlecode.protobuf.pro.stream.wire.StreamProtocol Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of protobuf-streamer-pro Show documentation
Show all versions of protobuf-streamer-pro Show documentation
Protobuf Streamer Pro is a java protocol buffers is a google protocol buffers transport for large streamed messages featuring multiplexed sending over reused TCP connections.
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: protobuf-stream.proto
package com.googlecode.protobuf.pro.stream.wire;
public final class StreamProtocol {
private StreamProtocol() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
}
public enum ChunkTypeCode
implements com.google.protobuf.ProtocolMessageEnum {
START(0, 0),
MIDDLE(1, 1),
END(2, 2),
;
public static final int START_VALUE = 0;
public static final int MIDDLE_VALUE = 1;
public static final int END_VALUE = 2;
public final int getNumber() { return value; }
public static ChunkTypeCode valueOf(int value) {
switch (value) {
case 0: return START;
case 1: return MIDDLE;
case 2: return END;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public ChunkTypeCode findValueByNumber(int number) {
return ChunkTypeCode.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.googlecode.protobuf.pro.stream.wire.StreamProtocol.getDescriptor().getEnumTypes().get(0);
}
private static final ChunkTypeCode[] VALUES = {
START, MIDDLE, END,
};
public static ChunkTypeCode valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private ChunkTypeCode(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:ChunkTypeCode)
}
public interface PullRequestOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required int32 correlationId = 1;
boolean hasCorrelationId();
int getCorrelationId();
// required bytes requestProto = 2;
boolean hasRequestProto();
com.google.protobuf.ByteString getRequestProto();
}
public static final class PullRequest extends
com.google.protobuf.GeneratedMessage
implements PullRequestOrBuilder {
// Use PullRequest.newBuilder() to construct.
private PullRequest(Builder builder) {
super(builder);
}
private PullRequest(boolean noInit) {}
private static final PullRequest defaultInstance;
public static PullRequest getDefaultInstance() {
return defaultInstance;
}
public PullRequest getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.stream.wire.StreamProtocol.internal_static_PullRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.stream.wire.StreamProtocol.internal_static_PullRequest_fieldAccessorTable;
}
private int bitField0_;
// required int32 correlationId = 1;
public static final int CORRELATIONID_FIELD_NUMBER = 1;
private int correlationId_;
public boolean hasCorrelationId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public int getCorrelationId() {
return correlationId_;
}
// required bytes requestProto = 2;
public static final int REQUESTPROTO_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString requestProto_;
public boolean hasRequestProto() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
public com.google.protobuf.ByteString getRequestProto() {
return requestProto_;
}
private void initFields() {
correlationId_ = 0;
requestProto_ = com.google.protobuf.ByteString.EMPTY;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasCorrelationId()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasRequestProto()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt32(1, correlationId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, requestProto_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, correlationId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, requestProto_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.stream.wire.StreamProtocol.internal_static_PullRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.stream.wire.StreamProtocol.internal_static_PullRequest_fieldAccessorTable;
}
// Construct using com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
correlationId_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
requestProto_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest.getDescriptor();
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest getDefaultInstanceForType() {
return com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest.getDefaultInstance();
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest build() {
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
private com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return result;
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest buildPartial() {
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest result = new com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.correlationId_ = correlationId_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.requestProto_ = requestProto_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest) {
return mergeFrom((com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest other) {
if (other == com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest.getDefaultInstance()) return this;
if (other.hasCorrelationId()) {
setCorrelationId(other.getCorrelationId());
}
if (other.hasRequestProto()) {
setRequestProto(other.getRequestProto());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasCorrelationId()) {
return false;
}
if (!hasRequestProto()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
correlationId_ = input.readInt32();
break;
}
case 18: {
bitField0_ |= 0x00000002;
requestProto_ = input.readBytes();
break;
}
}
}
}
private int bitField0_;
// required int32 correlationId = 1;
private int correlationId_ ;
public boolean hasCorrelationId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public int getCorrelationId() {
return correlationId_;
}
public Builder setCorrelationId(int value) {
bitField0_ |= 0x00000001;
correlationId_ = value;
onChanged();
return this;
}
public Builder clearCorrelationId() {
bitField0_ = (bitField0_ & ~0x00000001);
correlationId_ = 0;
onChanged();
return this;
}
// required bytes requestProto = 2;
private com.google.protobuf.ByteString requestProto_ = com.google.protobuf.ByteString.EMPTY;
public boolean hasRequestProto() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
public com.google.protobuf.ByteString getRequestProto() {
return requestProto_;
}
public Builder setRequestProto(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
requestProto_ = value;
onChanged();
return this;
}
public Builder clearRequestProto() {
bitField0_ = (bitField0_ & ~0x00000002);
requestProto_ = getDefaultInstance().getRequestProto();
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:PullRequest)
}
static {
defaultInstance = new PullRequest(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:PullRequest)
}
public interface PushRequestOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required int32 correlationId = 1;
boolean hasCorrelationId();
int getCorrelationId();
// required bytes requestProto = 2;
boolean hasRequestProto();
com.google.protobuf.ByteString getRequestProto();
}
public static final class PushRequest extends
com.google.protobuf.GeneratedMessage
implements PushRequestOrBuilder {
// Use PushRequest.newBuilder() to construct.
private PushRequest(Builder builder) {
super(builder);
}
private PushRequest(boolean noInit) {}
private static final PushRequest defaultInstance;
public static PushRequest getDefaultInstance() {
return defaultInstance;
}
public PushRequest getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.stream.wire.StreamProtocol.internal_static_PushRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.stream.wire.StreamProtocol.internal_static_PushRequest_fieldAccessorTable;
}
private int bitField0_;
// required int32 correlationId = 1;
public static final int CORRELATIONID_FIELD_NUMBER = 1;
private int correlationId_;
public boolean hasCorrelationId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public int getCorrelationId() {
return correlationId_;
}
// required bytes requestProto = 2;
public static final int REQUESTPROTO_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString requestProto_;
public boolean hasRequestProto() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
public com.google.protobuf.ByteString getRequestProto() {
return requestProto_;
}
private void initFields() {
correlationId_ = 0;
requestProto_ = com.google.protobuf.ByteString.EMPTY;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasCorrelationId()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasRequestProto()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt32(1, correlationId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, requestProto_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, correlationId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, requestProto_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.stream.wire.StreamProtocol.internal_static_PushRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.stream.wire.StreamProtocol.internal_static_PushRequest_fieldAccessorTable;
}
// Construct using com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
correlationId_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
requestProto_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest.getDescriptor();
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest getDefaultInstanceForType() {
return com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest.getDefaultInstance();
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest build() {
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
private com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return result;
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest buildPartial() {
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest result = new com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.correlationId_ = correlationId_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.requestProto_ = requestProto_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest) {
return mergeFrom((com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest other) {
if (other == com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest.getDefaultInstance()) return this;
if (other.hasCorrelationId()) {
setCorrelationId(other.getCorrelationId());
}
if (other.hasRequestProto()) {
setRequestProto(other.getRequestProto());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasCorrelationId()) {
return false;
}
if (!hasRequestProto()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
correlationId_ = input.readInt32();
break;
}
case 18: {
bitField0_ |= 0x00000002;
requestProto_ = input.readBytes();
break;
}
}
}
}
private int bitField0_;
// required int32 correlationId = 1;
private int correlationId_ ;
public boolean hasCorrelationId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public int getCorrelationId() {
return correlationId_;
}
public Builder setCorrelationId(int value) {
bitField0_ |= 0x00000001;
correlationId_ = value;
onChanged();
return this;
}
public Builder clearCorrelationId() {
bitField0_ = (bitField0_ & ~0x00000001);
correlationId_ = 0;
onChanged();
return this;
}
// required bytes requestProto = 2;
private com.google.protobuf.ByteString requestProto_ = com.google.protobuf.ByteString.EMPTY;
public boolean hasRequestProto() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
public com.google.protobuf.ByteString getRequestProto() {
return requestProto_;
}
public Builder setRequestProto(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
requestProto_ = value;
onChanged();
return this;
}
public Builder clearRequestProto() {
bitField0_ = (bitField0_ & ~0x00000002);
requestProto_ = getDefaultInstance().getRequestProto();
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:PushRequest)
}
static {
defaultInstance = new PushRequest(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:PushRequest)
}
public interface CloseNotificationOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required int32 correlationId = 1;
boolean hasCorrelationId();
int getCorrelationId();
}
public static final class CloseNotification extends
com.google.protobuf.GeneratedMessage
implements CloseNotificationOrBuilder {
// Use CloseNotification.newBuilder() to construct.
private CloseNotification(Builder builder) {
super(builder);
}
private CloseNotification(boolean noInit) {}
private static final CloseNotification defaultInstance;
public static CloseNotification getDefaultInstance() {
return defaultInstance;
}
public CloseNotification getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.stream.wire.StreamProtocol.internal_static_CloseNotification_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.stream.wire.StreamProtocol.internal_static_CloseNotification_fieldAccessorTable;
}
private int bitField0_;
// required int32 correlationId = 1;
public static final int CORRELATIONID_FIELD_NUMBER = 1;
private int correlationId_;
public boolean hasCorrelationId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public int getCorrelationId() {
return correlationId_;
}
private void initFields() {
correlationId_ = 0;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasCorrelationId()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt32(1, correlationId_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, correlationId_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotificationOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.stream.wire.StreamProtocol.internal_static_CloseNotification_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.stream.wire.StreamProtocol.internal_static_CloseNotification_fieldAccessorTable;
}
// Construct using com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
correlationId_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification.getDescriptor();
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification getDefaultInstanceForType() {
return com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification.getDefaultInstance();
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification build() {
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
private com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return result;
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification buildPartial() {
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification result = new com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.correlationId_ = correlationId_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification) {
return mergeFrom((com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification other) {
if (other == com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification.getDefaultInstance()) return this;
if (other.hasCorrelationId()) {
setCorrelationId(other.getCorrelationId());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasCorrelationId()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
correlationId_ = input.readInt32();
break;
}
}
}
}
private int bitField0_;
// required int32 correlationId = 1;
private int correlationId_ ;
public boolean hasCorrelationId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public int getCorrelationId() {
return correlationId_;
}
public Builder setCorrelationId(int value) {
bitField0_ |= 0x00000001;
correlationId_ = value;
onChanged();
return this;
}
public Builder clearCorrelationId() {
bitField0_ = (bitField0_ & ~0x00000001);
correlationId_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:CloseNotification)
}
static {
defaultInstance = new CloseNotification(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:CloseNotification)
}
public interface ParameterOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required string name = 1;
boolean hasName();
String getName();
// required string value = 2;
boolean hasValue();
String getValue();
}
public static final class Parameter extends
com.google.protobuf.GeneratedMessage
implements ParameterOrBuilder {
// Use Parameter.newBuilder() to construct.
private Parameter(Builder builder) {
super(builder);
}
private Parameter(boolean noInit) {}
private static final Parameter defaultInstance;
public static Parameter getDefaultInstance() {
return defaultInstance;
}
public Parameter getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.stream.wire.StreamProtocol.internal_static_Parameter_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.stream.wire.StreamProtocol.internal_static_Parameter_fieldAccessorTable;
}
private int bitField0_;
// required string name = 1;
public static final int NAME_FIELD_NUMBER = 1;
private java.lang.Object name_;
public boolean hasName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public String getName() {
java.lang.Object ref = name_;
if (ref instanceof String) {
return (String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
if (com.google.protobuf.Internal.isValidUtf8(bs)) {
name_ = s;
}
return s;
}
}
private com.google.protobuf.ByteString getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// required string value = 2;
public static final int VALUE_FIELD_NUMBER = 2;
private java.lang.Object value_;
public boolean hasValue() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
public String getValue() {
java.lang.Object ref = value_;
if (ref instanceof String) {
return (String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
if (com.google.protobuf.Internal.isValidUtf8(bs)) {
value_ = s;
}
return s;
}
}
private com.google.protobuf.ByteString getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private void initFields() {
name_ = "";
value_ = "";
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasName()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasValue()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getNameBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getValueBytes());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getNameBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getValueBytes());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.googlecode.protobuf.pro.stream.wire.StreamProtocol.ParameterOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.stream.wire.StreamProtocol.internal_static_Parameter_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.stream.wire.StreamProtocol.internal_static_Parameter_fieldAccessorTable;
}
// Construct using com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
name_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
value_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter.getDescriptor();
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter getDefaultInstanceForType() {
return com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter.getDefaultInstance();
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter build() {
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
private com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return result;
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter buildPartial() {
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter result = new com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.name_ = name_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.value_ = value_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter) {
return mergeFrom((com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter other) {
if (other == com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter.getDefaultInstance()) return this;
if (other.hasName()) {
setName(other.getName());
}
if (other.hasValue()) {
setValue(other.getValue());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasName()) {
return false;
}
if (!hasValue()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
name_ = input.readBytes();
break;
}
case 18: {
bitField0_ |= 0x00000002;
value_ = input.readBytes();
break;
}
}
}
}
private int bitField0_;
// required string name = 1;
private java.lang.Object name_ = "";
public boolean hasName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof String)) {
String s = ((com.google.protobuf.ByteString) ref).toStringUtf8();
name_ = s;
return s;
} else {
return (String) ref;
}
}
public Builder setName(String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000001);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
void setName(com.google.protobuf.ByteString value) {
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
}
// required string value = 2;
private java.lang.Object value_ = "";
public boolean hasValue() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
public String getValue() {
java.lang.Object ref = value_;
if (!(ref instanceof String)) {
String s = ((com.google.protobuf.ByteString) ref).toStringUtf8();
value_ = s;
return s;
} else {
return (String) ref;
}
}
public Builder setValue(String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
value_ = value;
onChanged();
return this;
}
public Builder clearValue() {
bitField0_ = (bitField0_ & ~0x00000002);
value_ = getDefaultInstance().getValue();
onChanged();
return this;
}
void setValue(com.google.protobuf.ByteString value) {
bitField0_ |= 0x00000002;
value_ = value;
onChanged();
}
// @@protoc_insertion_point(builder_scope:Parameter)
}
static {
defaultInstance = new Parameter(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:Parameter)
}
public interface ChunkOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required int32 correlationId = 1;
boolean hasCorrelationId();
int getCorrelationId();
// required .ChunkTypeCode chunkType = 2;
boolean hasChunkType();
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.ChunkTypeCode getChunkType();
// required int32 seqNo = 3;
boolean hasSeqNo();
int getSeqNo();
// repeated .Parameter parameter = 4;
java.util.List
getParameterList();
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter getParameter(int index);
int getParameterCount();
java.util.List extends com.googlecode.protobuf.pro.stream.wire.StreamProtocol.ParameterOrBuilder>
getParameterOrBuilderList();
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.ParameterOrBuilder getParameterOrBuilder(
int index);
// optional bytes payload = 5;
boolean hasPayload();
com.google.protobuf.ByteString getPayload();
}
public static final class Chunk extends
com.google.protobuf.GeneratedMessage
implements ChunkOrBuilder {
// Use Chunk.newBuilder() to construct.
private Chunk(Builder builder) {
super(builder);
}
private Chunk(boolean noInit) {}
private static final Chunk defaultInstance;
public static Chunk getDefaultInstance() {
return defaultInstance;
}
public Chunk getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.stream.wire.StreamProtocol.internal_static_Chunk_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.stream.wire.StreamProtocol.internal_static_Chunk_fieldAccessorTable;
}
private int bitField0_;
// required int32 correlationId = 1;
public static final int CORRELATIONID_FIELD_NUMBER = 1;
private int correlationId_;
public boolean hasCorrelationId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public int getCorrelationId() {
return correlationId_;
}
// required .ChunkTypeCode chunkType = 2;
public static final int CHUNKTYPE_FIELD_NUMBER = 2;
private com.googlecode.protobuf.pro.stream.wire.StreamProtocol.ChunkTypeCode chunkType_;
public boolean hasChunkType() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.ChunkTypeCode getChunkType() {
return chunkType_;
}
// required int32 seqNo = 3;
public static final int SEQNO_FIELD_NUMBER = 3;
private int seqNo_;
public boolean hasSeqNo() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
public int getSeqNo() {
return seqNo_;
}
// repeated .Parameter parameter = 4;
public static final int PARAMETER_FIELD_NUMBER = 4;
private java.util.List parameter_;
public java.util.List getParameterList() {
return parameter_;
}
public java.util.List extends com.googlecode.protobuf.pro.stream.wire.StreamProtocol.ParameterOrBuilder>
getParameterOrBuilderList() {
return parameter_;
}
public int getParameterCount() {
return parameter_.size();
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter getParameter(int index) {
return parameter_.get(index);
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.ParameterOrBuilder getParameterOrBuilder(
int index) {
return parameter_.get(index);
}
// optional bytes payload = 5;
public static final int PAYLOAD_FIELD_NUMBER = 5;
private com.google.protobuf.ByteString payload_;
public boolean hasPayload() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
public com.google.protobuf.ByteString getPayload() {
return payload_;
}
private void initFields() {
correlationId_ = 0;
chunkType_ = com.googlecode.protobuf.pro.stream.wire.StreamProtocol.ChunkTypeCode.START;
seqNo_ = 0;
parameter_ = java.util.Collections.emptyList();
payload_ = com.google.protobuf.ByteString.EMPTY;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasCorrelationId()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasChunkType()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasSeqNo()) {
memoizedIsInitialized = 0;
return false;
}
for (int i = 0; i < getParameterCount(); i++) {
if (!getParameter(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt32(1, correlationId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeEnum(2, chunkType_.getNumber());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeInt32(3, seqNo_);
}
for (int i = 0; i < parameter_.size(); i++) {
output.writeMessage(4, parameter_.get(i));
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBytes(5, payload_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, correlationId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, chunkType_.getNumber());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, seqNo_);
}
for (int i = 0; i < parameter_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, parameter_.get(i));
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(5, payload_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.googlecode.protobuf.pro.stream.wire.StreamProtocol.ChunkOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.stream.wire.StreamProtocol.internal_static_Chunk_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.stream.wire.StreamProtocol.internal_static_Chunk_fieldAccessorTable;
}
// Construct using com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getParameterFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
correlationId_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
chunkType_ = com.googlecode.protobuf.pro.stream.wire.StreamProtocol.ChunkTypeCode.START;
bitField0_ = (bitField0_ & ~0x00000002);
seqNo_ = 0;
bitField0_ = (bitField0_ & ~0x00000004);
if (parameterBuilder_ == null) {
parameter_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
} else {
parameterBuilder_.clear();
}
payload_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk.getDescriptor();
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk getDefaultInstanceForType() {
return com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk.getDefaultInstance();
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk build() {
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
private com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return result;
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk buildPartial() {
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk result = new com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.correlationId_ = correlationId_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.chunkType_ = chunkType_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.seqNo_ = seqNo_;
if (parameterBuilder_ == null) {
if (((bitField0_ & 0x00000008) == 0x00000008)) {
parameter_ = java.util.Collections.unmodifiableList(parameter_);
bitField0_ = (bitField0_ & ~0x00000008);
}
result.parameter_ = parameter_;
} else {
result.parameter_ = parameterBuilder_.build();
}
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000008;
}
result.payload_ = payload_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk) {
return mergeFrom((com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk other) {
if (other == com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk.getDefaultInstance()) return this;
if (other.hasCorrelationId()) {
setCorrelationId(other.getCorrelationId());
}
if (other.hasChunkType()) {
setChunkType(other.getChunkType());
}
if (other.hasSeqNo()) {
setSeqNo(other.getSeqNo());
}
if (parameterBuilder_ == null) {
if (!other.parameter_.isEmpty()) {
if (parameter_.isEmpty()) {
parameter_ = other.parameter_;
bitField0_ = (bitField0_ & ~0x00000008);
} else {
ensureParameterIsMutable();
parameter_.addAll(other.parameter_);
}
onChanged();
}
} else {
if (!other.parameter_.isEmpty()) {
if (parameterBuilder_.isEmpty()) {
parameterBuilder_.dispose();
parameterBuilder_ = null;
parameter_ = other.parameter_;
bitField0_ = (bitField0_ & ~0x00000008);
parameterBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getParameterFieldBuilder() : null;
} else {
parameterBuilder_.addAllMessages(other.parameter_);
}
}
}
if (other.hasPayload()) {
setPayload(other.getPayload());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasCorrelationId()) {
return false;
}
if (!hasChunkType()) {
return false;
}
if (!hasSeqNo()) {
return false;
}
for (int i = 0; i < getParameterCount(); i++) {
if (!getParameter(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
correlationId_ = input.readInt32();
break;
}
case 16: {
int rawValue = input.readEnum();
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.ChunkTypeCode value = com.googlecode.protobuf.pro.stream.wire.StreamProtocol.ChunkTypeCode.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(2, rawValue);
} else {
bitField0_ |= 0x00000002;
chunkType_ = value;
}
break;
}
case 24: {
bitField0_ |= 0x00000004;
seqNo_ = input.readInt32();
break;
}
case 34: {
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter.Builder subBuilder = com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter.newBuilder();
input.readMessage(subBuilder, extensionRegistry);
addParameter(subBuilder.buildPartial());
break;
}
case 42: {
bitField0_ |= 0x00000010;
payload_ = input.readBytes();
break;
}
}
}
}
private int bitField0_;
// required int32 correlationId = 1;
private int correlationId_ ;
public boolean hasCorrelationId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public int getCorrelationId() {
return correlationId_;
}
public Builder setCorrelationId(int value) {
bitField0_ |= 0x00000001;
correlationId_ = value;
onChanged();
return this;
}
public Builder clearCorrelationId() {
bitField0_ = (bitField0_ & ~0x00000001);
correlationId_ = 0;
onChanged();
return this;
}
// required .ChunkTypeCode chunkType = 2;
private com.googlecode.protobuf.pro.stream.wire.StreamProtocol.ChunkTypeCode chunkType_ = com.googlecode.protobuf.pro.stream.wire.StreamProtocol.ChunkTypeCode.START;
public boolean hasChunkType() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.ChunkTypeCode getChunkType() {
return chunkType_;
}
public Builder setChunkType(com.googlecode.protobuf.pro.stream.wire.StreamProtocol.ChunkTypeCode value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
chunkType_ = value;
onChanged();
return this;
}
public Builder clearChunkType() {
bitField0_ = (bitField0_ & ~0x00000002);
chunkType_ = com.googlecode.protobuf.pro.stream.wire.StreamProtocol.ChunkTypeCode.START;
onChanged();
return this;
}
// required int32 seqNo = 3;
private int seqNo_ ;
public boolean hasSeqNo() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
public int getSeqNo() {
return seqNo_;
}
public Builder setSeqNo(int value) {
bitField0_ |= 0x00000004;
seqNo_ = value;
onChanged();
return this;
}
public Builder clearSeqNo() {
bitField0_ = (bitField0_ & ~0x00000004);
seqNo_ = 0;
onChanged();
return this;
}
// repeated .Parameter parameter = 4;
private java.util.List parameter_ =
java.util.Collections.emptyList();
private void ensureParameterIsMutable() {
if (!((bitField0_ & 0x00000008) == 0x00000008)) {
parameter_ = new java.util.ArrayList(parameter_);
bitField0_ |= 0x00000008;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter, com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter.Builder, com.googlecode.protobuf.pro.stream.wire.StreamProtocol.ParameterOrBuilder> parameterBuilder_;
public java.util.List getParameterList() {
if (parameterBuilder_ == null) {
return java.util.Collections.unmodifiableList(parameter_);
} else {
return parameterBuilder_.getMessageList();
}
}
public int getParameterCount() {
if (parameterBuilder_ == null) {
return parameter_.size();
} else {
return parameterBuilder_.getCount();
}
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter getParameter(int index) {
if (parameterBuilder_ == null) {
return parameter_.get(index);
} else {
return parameterBuilder_.getMessage(index);
}
}
public Builder setParameter(
int index, com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter value) {
if (parameterBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureParameterIsMutable();
parameter_.set(index, value);
onChanged();
} else {
parameterBuilder_.setMessage(index, value);
}
return this;
}
public Builder setParameter(
int index, com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter.Builder builderForValue) {
if (parameterBuilder_ == null) {
ensureParameterIsMutable();
parameter_.set(index, builderForValue.build());
onChanged();
} else {
parameterBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
public Builder addParameter(com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter value) {
if (parameterBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureParameterIsMutable();
parameter_.add(value);
onChanged();
} else {
parameterBuilder_.addMessage(value);
}
return this;
}
public Builder addParameter(
int index, com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter value) {
if (parameterBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureParameterIsMutable();
parameter_.add(index, value);
onChanged();
} else {
parameterBuilder_.addMessage(index, value);
}
return this;
}
public Builder addParameter(
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter.Builder builderForValue) {
if (parameterBuilder_ == null) {
ensureParameterIsMutable();
parameter_.add(builderForValue.build());
onChanged();
} else {
parameterBuilder_.addMessage(builderForValue.build());
}
return this;
}
public Builder addParameter(
int index, com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter.Builder builderForValue) {
if (parameterBuilder_ == null) {
ensureParameterIsMutable();
parameter_.add(index, builderForValue.build());
onChanged();
} else {
parameterBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
public Builder addAllParameter(
java.lang.Iterable extends com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter> values) {
if (parameterBuilder_ == null) {
ensureParameterIsMutable();
super.addAll(values, parameter_);
onChanged();
} else {
parameterBuilder_.addAllMessages(values);
}
return this;
}
public Builder clearParameter() {
if (parameterBuilder_ == null) {
parameter_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
} else {
parameterBuilder_.clear();
}
return this;
}
public Builder removeParameter(int index) {
if (parameterBuilder_ == null) {
ensureParameterIsMutable();
parameter_.remove(index);
onChanged();
} else {
parameterBuilder_.remove(index);
}
return this;
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter.Builder getParameterBuilder(
int index) {
return getParameterFieldBuilder().getBuilder(index);
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.ParameterOrBuilder getParameterOrBuilder(
int index) {
if (parameterBuilder_ == null) {
return parameter_.get(index); } else {
return parameterBuilder_.getMessageOrBuilder(index);
}
}
public java.util.List extends com.googlecode.protobuf.pro.stream.wire.StreamProtocol.ParameterOrBuilder>
getParameterOrBuilderList() {
if (parameterBuilder_ != null) {
return parameterBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(parameter_);
}
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter.Builder addParameterBuilder() {
return getParameterFieldBuilder().addBuilder(
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter.getDefaultInstance());
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter.Builder addParameterBuilder(
int index) {
return getParameterFieldBuilder().addBuilder(
index, com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter.getDefaultInstance());
}
public java.util.List
getParameterBuilderList() {
return getParameterFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter, com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter.Builder, com.googlecode.protobuf.pro.stream.wire.StreamProtocol.ParameterOrBuilder>
getParameterFieldBuilder() {
if (parameterBuilder_ == null) {
parameterBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter, com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter.Builder, com.googlecode.protobuf.pro.stream.wire.StreamProtocol.ParameterOrBuilder>(
parameter_,
((bitField0_ & 0x00000008) == 0x00000008),
getParentForChildren(),
isClean());
parameter_ = null;
}
return parameterBuilder_;
}
// optional bytes payload = 5;
private com.google.protobuf.ByteString payload_ = com.google.protobuf.ByteString.EMPTY;
public boolean hasPayload() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
public com.google.protobuf.ByteString getPayload() {
return payload_;
}
public Builder setPayload(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
payload_ = value;
onChanged();
return this;
}
public Builder clearPayload() {
bitField0_ = (bitField0_ & ~0x00000010);
payload_ = getDefaultInstance().getPayload();
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:Chunk)
}
static {
defaultInstance = new Chunk(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:Chunk)
}
public interface WirePayloadOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional .Chunk chunk = 1;
boolean hasChunk();
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk getChunk();
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.ChunkOrBuilder getChunkOrBuilder();
// optional .PullRequest pull = 2;
boolean hasPull();
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest getPull();
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequestOrBuilder getPullOrBuilder();
// optional .PushRequest push = 3;
boolean hasPush();
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest getPush();
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequestOrBuilder getPushOrBuilder();
// optional .CloseNotification close = 4;
boolean hasClose();
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification getClose();
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotificationOrBuilder getCloseOrBuilder();
}
public static final class WirePayload extends
com.google.protobuf.GeneratedMessage
implements WirePayloadOrBuilder {
// Use WirePayload.newBuilder() to construct.
private WirePayload(Builder builder) {
super(builder);
}
private WirePayload(boolean noInit) {}
private static final WirePayload defaultInstance;
public static WirePayload getDefaultInstance() {
return defaultInstance;
}
public WirePayload getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.stream.wire.StreamProtocol.internal_static_WirePayload_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.stream.wire.StreamProtocol.internal_static_WirePayload_fieldAccessorTable;
}
private int bitField0_;
// optional .Chunk chunk = 1;
public static final int CHUNK_FIELD_NUMBER = 1;
private com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk chunk_;
public boolean hasChunk() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk getChunk() {
return chunk_;
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.ChunkOrBuilder getChunkOrBuilder() {
return chunk_;
}
// optional .PullRequest pull = 2;
public static final int PULL_FIELD_NUMBER = 2;
private com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest pull_;
public boolean hasPull() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest getPull() {
return pull_;
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequestOrBuilder getPullOrBuilder() {
return pull_;
}
// optional .PushRequest push = 3;
public static final int PUSH_FIELD_NUMBER = 3;
private com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest push_;
public boolean hasPush() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest getPush() {
return push_;
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequestOrBuilder getPushOrBuilder() {
return push_;
}
// optional .CloseNotification close = 4;
public static final int CLOSE_FIELD_NUMBER = 4;
private com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification close_;
public boolean hasClose() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification getClose() {
return close_;
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotificationOrBuilder getCloseOrBuilder() {
return close_;
}
private void initFields() {
chunk_ = com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk.getDefaultInstance();
pull_ = com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest.getDefaultInstance();
push_ = com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest.getDefaultInstance();
close_ = com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (hasChunk()) {
if (!getChunk().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasPull()) {
if (!getPull().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasPush()) {
if (!getPush().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasClose()) {
if (!getClose().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, chunk_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeMessage(2, pull_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeMessage(3, push_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeMessage(4, close_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, chunk_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, pull_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, push_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, close_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.WirePayload parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.WirePayload parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.WirePayload parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.WirePayload parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.WirePayload parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.WirePayload parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.WirePayload parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.WirePayload parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.WirePayload parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static com.googlecode.protobuf.pro.stream.wire.StreamProtocol.WirePayload parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.googlecode.protobuf.pro.stream.wire.StreamProtocol.WirePayload prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.googlecode.protobuf.pro.stream.wire.StreamProtocol.WirePayloadOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.googlecode.protobuf.pro.stream.wire.StreamProtocol.internal_static_WirePayload_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.googlecode.protobuf.pro.stream.wire.StreamProtocol.internal_static_WirePayload_fieldAccessorTable;
}
// Construct using com.googlecode.protobuf.pro.stream.wire.StreamProtocol.WirePayload.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getChunkFieldBuilder();
getPullFieldBuilder();
getPushFieldBuilder();
getCloseFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (chunkBuilder_ == null) {
chunk_ = com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk.getDefaultInstance();
} else {
chunkBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
if (pullBuilder_ == null) {
pull_ = com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest.getDefaultInstance();
} else {
pullBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
if (pushBuilder_ == null) {
push_ = com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest.getDefaultInstance();
} else {
pushBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
if (closeBuilder_ == null) {
close_ = com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification.getDefaultInstance();
} else {
closeBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.googlecode.protobuf.pro.stream.wire.StreamProtocol.WirePayload.getDescriptor();
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.WirePayload getDefaultInstanceForType() {
return com.googlecode.protobuf.pro.stream.wire.StreamProtocol.WirePayload.getDefaultInstance();
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.WirePayload build() {
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.WirePayload result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
private com.googlecode.protobuf.pro.stream.wire.StreamProtocol.WirePayload buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.WirePayload result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return result;
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.WirePayload buildPartial() {
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.WirePayload result = new com.googlecode.protobuf.pro.stream.wire.StreamProtocol.WirePayload(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
if (chunkBuilder_ == null) {
result.chunk_ = chunk_;
} else {
result.chunk_ = chunkBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
if (pullBuilder_ == null) {
result.pull_ = pull_;
} else {
result.pull_ = pullBuilder_.build();
}
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
if (pushBuilder_ == null) {
result.push_ = push_;
} else {
result.push_ = pushBuilder_.build();
}
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
if (closeBuilder_ == null) {
result.close_ = close_;
} else {
result.close_ = closeBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.googlecode.protobuf.pro.stream.wire.StreamProtocol.WirePayload) {
return mergeFrom((com.googlecode.protobuf.pro.stream.wire.StreamProtocol.WirePayload)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.googlecode.protobuf.pro.stream.wire.StreamProtocol.WirePayload other) {
if (other == com.googlecode.protobuf.pro.stream.wire.StreamProtocol.WirePayload.getDefaultInstance()) return this;
if (other.hasChunk()) {
mergeChunk(other.getChunk());
}
if (other.hasPull()) {
mergePull(other.getPull());
}
if (other.hasPush()) {
mergePush(other.getPush());
}
if (other.hasClose()) {
mergeClose(other.getClose());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (hasChunk()) {
if (!getChunk().isInitialized()) {
return false;
}
}
if (hasPull()) {
if (!getPull().isInitialized()) {
return false;
}
}
if (hasPush()) {
if (!getPush().isInitialized()) {
return false;
}
}
if (hasClose()) {
if (!getClose().isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
}
break;
}
case 10: {
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk.Builder subBuilder = com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk.newBuilder();
if (hasChunk()) {
subBuilder.mergeFrom(getChunk());
}
input.readMessage(subBuilder, extensionRegistry);
setChunk(subBuilder.buildPartial());
break;
}
case 18: {
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest.Builder subBuilder = com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest.newBuilder();
if (hasPull()) {
subBuilder.mergeFrom(getPull());
}
input.readMessage(subBuilder, extensionRegistry);
setPull(subBuilder.buildPartial());
break;
}
case 26: {
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest.Builder subBuilder = com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest.newBuilder();
if (hasPush()) {
subBuilder.mergeFrom(getPush());
}
input.readMessage(subBuilder, extensionRegistry);
setPush(subBuilder.buildPartial());
break;
}
case 34: {
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification.Builder subBuilder = com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification.newBuilder();
if (hasClose()) {
subBuilder.mergeFrom(getClose());
}
input.readMessage(subBuilder, extensionRegistry);
setClose(subBuilder.buildPartial());
break;
}
}
}
}
private int bitField0_;
// optional .Chunk chunk = 1;
private com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk chunk_ = com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk, com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk.Builder, com.googlecode.protobuf.pro.stream.wire.StreamProtocol.ChunkOrBuilder> chunkBuilder_;
public boolean hasChunk() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk getChunk() {
if (chunkBuilder_ == null) {
return chunk_;
} else {
return chunkBuilder_.getMessage();
}
}
public Builder setChunk(com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk value) {
if (chunkBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
chunk_ = value;
onChanged();
} else {
chunkBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
return this;
}
public Builder setChunk(
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk.Builder builderForValue) {
if (chunkBuilder_ == null) {
chunk_ = builderForValue.build();
onChanged();
} else {
chunkBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
return this;
}
public Builder mergeChunk(com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk value) {
if (chunkBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
chunk_ != com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk.getDefaultInstance()) {
chunk_ =
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk.newBuilder(chunk_).mergeFrom(value).buildPartial();
} else {
chunk_ = value;
}
onChanged();
} else {
chunkBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
return this;
}
public Builder clearChunk() {
if (chunkBuilder_ == null) {
chunk_ = com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk.getDefaultInstance();
onChanged();
} else {
chunkBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk.Builder getChunkBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getChunkFieldBuilder().getBuilder();
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.ChunkOrBuilder getChunkOrBuilder() {
if (chunkBuilder_ != null) {
return chunkBuilder_.getMessageOrBuilder();
} else {
return chunk_;
}
}
private com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk, com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk.Builder, com.googlecode.protobuf.pro.stream.wire.StreamProtocol.ChunkOrBuilder>
getChunkFieldBuilder() {
if (chunkBuilder_ == null) {
chunkBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk, com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk.Builder, com.googlecode.protobuf.pro.stream.wire.StreamProtocol.ChunkOrBuilder>(
chunk_,
getParentForChildren(),
isClean());
chunk_ = null;
}
return chunkBuilder_;
}
// optional .PullRequest pull = 2;
private com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest pull_ = com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest, com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest.Builder, com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequestOrBuilder> pullBuilder_;
public boolean hasPull() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest getPull() {
if (pullBuilder_ == null) {
return pull_;
} else {
return pullBuilder_.getMessage();
}
}
public Builder setPull(com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest value) {
if (pullBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
pull_ = value;
onChanged();
} else {
pullBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
return this;
}
public Builder setPull(
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest.Builder builderForValue) {
if (pullBuilder_ == null) {
pull_ = builderForValue.build();
onChanged();
} else {
pullBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
return this;
}
public Builder mergePull(com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest value) {
if (pullBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002) &&
pull_ != com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest.getDefaultInstance()) {
pull_ =
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest.newBuilder(pull_).mergeFrom(value).buildPartial();
} else {
pull_ = value;
}
onChanged();
} else {
pullBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
return this;
}
public Builder clearPull() {
if (pullBuilder_ == null) {
pull_ = com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest.getDefaultInstance();
onChanged();
} else {
pullBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest.Builder getPullBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getPullFieldBuilder().getBuilder();
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequestOrBuilder getPullOrBuilder() {
if (pullBuilder_ != null) {
return pullBuilder_.getMessageOrBuilder();
} else {
return pull_;
}
}
private com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest, com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest.Builder, com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequestOrBuilder>
getPullFieldBuilder() {
if (pullBuilder_ == null) {
pullBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest, com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest.Builder, com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequestOrBuilder>(
pull_,
getParentForChildren(),
isClean());
pull_ = null;
}
return pullBuilder_;
}
// optional .PushRequest push = 3;
private com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest push_ = com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest, com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest.Builder, com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequestOrBuilder> pushBuilder_;
public boolean hasPush() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest getPush() {
if (pushBuilder_ == null) {
return push_;
} else {
return pushBuilder_.getMessage();
}
}
public Builder setPush(com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest value) {
if (pushBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
push_ = value;
onChanged();
} else {
pushBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
return this;
}
public Builder setPush(
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest.Builder builderForValue) {
if (pushBuilder_ == null) {
push_ = builderForValue.build();
onChanged();
} else {
pushBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
return this;
}
public Builder mergePush(com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest value) {
if (pushBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004) &&
push_ != com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest.getDefaultInstance()) {
push_ =
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest.newBuilder(push_).mergeFrom(value).buildPartial();
} else {
push_ = value;
}
onChanged();
} else {
pushBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000004;
return this;
}
public Builder clearPush() {
if (pushBuilder_ == null) {
push_ = com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest.getDefaultInstance();
onChanged();
} else {
pushBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest.Builder getPushBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getPushFieldBuilder().getBuilder();
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequestOrBuilder getPushOrBuilder() {
if (pushBuilder_ != null) {
return pushBuilder_.getMessageOrBuilder();
} else {
return push_;
}
}
private com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest, com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest.Builder, com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequestOrBuilder>
getPushFieldBuilder() {
if (pushBuilder_ == null) {
pushBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest, com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest.Builder, com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequestOrBuilder>(
push_,
getParentForChildren(),
isClean());
push_ = null;
}
return pushBuilder_;
}
// optional .CloseNotification close = 4;
private com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification close_ = com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification, com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification.Builder, com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotificationOrBuilder> closeBuilder_;
public boolean hasClose() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification getClose() {
if (closeBuilder_ == null) {
return close_;
} else {
return closeBuilder_.getMessage();
}
}
public Builder setClose(com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification value) {
if (closeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
close_ = value;
onChanged();
} else {
closeBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
return this;
}
public Builder setClose(
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification.Builder builderForValue) {
if (closeBuilder_ == null) {
close_ = builderForValue.build();
onChanged();
} else {
closeBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
return this;
}
public Builder mergeClose(com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification value) {
if (closeBuilder_ == null) {
if (((bitField0_ & 0x00000008) == 0x00000008) &&
close_ != com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification.getDefaultInstance()) {
close_ =
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification.newBuilder(close_).mergeFrom(value).buildPartial();
} else {
close_ = value;
}
onChanged();
} else {
closeBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000008;
return this;
}
public Builder clearClose() {
if (closeBuilder_ == null) {
close_ = com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification.getDefaultInstance();
onChanged();
} else {
closeBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification.Builder getCloseBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getCloseFieldBuilder().getBuilder();
}
public com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotificationOrBuilder getCloseOrBuilder() {
if (closeBuilder_ != null) {
return closeBuilder_.getMessageOrBuilder();
} else {
return close_;
}
}
private com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification, com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification.Builder, com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotificationOrBuilder>
getCloseFieldBuilder() {
if (closeBuilder_ == null) {
closeBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification, com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification.Builder, com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotificationOrBuilder>(
close_,
getParentForChildren(),
isClean());
close_ = null;
}
return closeBuilder_;
}
// @@protoc_insertion_point(builder_scope:WirePayload)
}
static {
defaultInstance = new WirePayload(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:WirePayload)
}
private static com.google.protobuf.Descriptors.Descriptor
internal_static_PullRequest_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_PullRequest_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_PushRequest_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_PushRequest_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_CloseNotification_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_CloseNotification_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_Parameter_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_Parameter_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_Chunk_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_Chunk_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_WirePayload_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_WirePayload_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\025protobuf-stream.proto\":\n\013PullRequest\022\025" +
"\n\rcorrelationId\030\001 \002(\005\022\024\n\014requestProto\030\002 " +
"\002(\014\":\n\013PushRequest\022\025\n\rcorrelationId\030\001 \002(" +
"\005\022\024\n\014requestProto\030\002 \002(\014\"*\n\021CloseNotifica" +
"tion\022\025\n\rcorrelationId\030\001 \002(\005\"(\n\tParameter" +
"\022\014\n\004name\030\001 \002(\t\022\r\n\005value\030\002 \002(\t\"\200\001\n\005Chunk\022" +
"\025\n\rcorrelationId\030\001 \002(\005\022!\n\tchunkType\030\002 \002(" +
"\0162\016.ChunkTypeCode\022\r\n\005seqNo\030\003 \002(\005\022\035\n\tpara" +
"meter\030\004 \003(\0132\n.Parameter\022\017\n\007payload\030\005 \001(\014" +
"\"\177\n\013WirePayload\022\025\n\005chunk\030\001 \001(\0132\006.Chunk\022\032",
"\n\004pull\030\002 \001(\0132\014.PullRequest\022\032\n\004push\030\003 \001(\013" +
"2\014.PushRequest\022!\n\005close\030\004 \001(\0132\022.CloseNot" +
"ification*/\n\rChunkTypeCode\022\t\n\005START\020\000\022\n\n" +
"\006MIDDLE\020\001\022\007\n\003END\020\002B;\n\'com.googlecode.pro" +
"tobuf.pro.stream.wireB\016StreamProtocolH\001"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
internal_static_PullRequest_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_PullRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_PullRequest_descriptor,
new java.lang.String[] { "CorrelationId", "RequestProto", },
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest.class,
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PullRequest.Builder.class);
internal_static_PushRequest_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_PushRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_PushRequest_descriptor,
new java.lang.String[] { "CorrelationId", "RequestProto", },
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest.class,
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.PushRequest.Builder.class);
internal_static_CloseNotification_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_CloseNotification_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_CloseNotification_descriptor,
new java.lang.String[] { "CorrelationId", },
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification.class,
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.CloseNotification.Builder.class);
internal_static_Parameter_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_Parameter_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_Parameter_descriptor,
new java.lang.String[] { "Name", "Value", },
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter.class,
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Parameter.Builder.class);
internal_static_Chunk_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_Chunk_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_Chunk_descriptor,
new java.lang.String[] { "CorrelationId", "ChunkType", "SeqNo", "Parameter", "Payload", },
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk.class,
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.Chunk.Builder.class);
internal_static_WirePayload_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_WirePayload_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_WirePayload_descriptor,
new java.lang.String[] { "Chunk", "Pull", "Push", "Close", },
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.WirePayload.class,
com.googlecode.protobuf.pro.stream.wire.StreamProtocol.WirePayload.Builder.class);
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
}, assigner);
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy