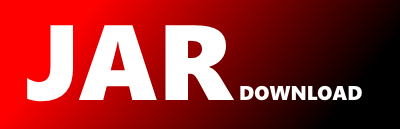
java.fedora.client.bmech.xml.BObjMETSSerializer Maven / Gradle / Ivy
Show all versions of fcrepo-client Show documentation
/*
* -----------------------------------------------------------------------------
*
* License and Copyright: The contents of this file are subject to the
* Apache License, Version 2.0 (the "License"); you may not use
* this file except in compliance with the License. You may obtain a copy of
* the License at
* http://www.fedora-commons.org/licenses.
*
* Software distributed under the License is distributed on an "AS IS" basis,
* WITHOUT WARRANTY OF ANY KIND, either express or implied. See the License for
* the specific language governing rights and limitations under the License.
*
* The entire file consists of original code.
* Copyright © 2008 Fedora Commons, Inc.
*
Copyright © 2002-2007 The Rector and Visitors of the University of
* Virginia and Cornell University
* All rights reserved.
*
* -----------------------------------------------------------------------------
*/
package fedora.client.bmech.xml;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.Attr;
import org.w3c.dom.Node;
import javax.xml.transform.dom.DOMResult;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.ParserConfigurationException;
import java.util.Vector;
import java.util.Date;
import java.io.PrintWriter;
import java.io.File;
import java.io.InputStream;
import fedora.client.bmech.data.*;
import fedora.client.bmech.BMechBuilderException;
import fedora.server.utilities.DateUtility;
/**
*
* Title: BObjMETSSerializer.java
* Description:
*
* @author [email protected]
* @version $Id: BObjMETSSerializer.java 5166 2006-10-25 11:05:45Z eddie $
*/
public abstract class BObjMETSSerializer
{
protected static final String METS = "http://www.loc.gov/METS/";
protected static final String AUDIT = "http://www.fedora.info/definitions/audit";
protected static final String DESC = "http://dl.lib.virginia.edu/bin/dtd/descmeta/descmeta.dtd";
protected static final String ADMIN = "http://dl.lib.virginia.edu/bin/dtd/admin/admin.dtd";
protected static final String XLINK = "http://www.w3.org/TR/xlink";
protected static final String SCHEMALOC =
"http://www.loc.gov/standards/METS/ http://www.fedora.info/definitions/1/0/mets-fedora-ext.xsd";
protected static final String XSI = "http://www.w3.org/2001/XMLSchema-instance";
protected static final String XMLNS = "http://www.w3.org/2000/xmlns/";
protected PrintWriter out;
protected Document document;
protected Element root;
//protected Element header;
protected Element bObjFileSec;
protected Vector docDSIDs = new Vector();
protected Element bObjStructMap;
protected Element bObjBehaviorSec;
protected BObjTemplate bObjData;
protected String now;
public BObjMETSSerializer(BObjTemplate bObjData)
throws BMechBuilderException
{
this.bObjData = bObjData;
this.now = DateUtility.convertDateToString(new Date());
}
// The BDefMETSSerializer and BMechMETSSerializer will implement this to
// deal with the specific metadata formats required in each.
protected abstract Element[] getInlineMD()
throws BMechBuilderException;
// The BDefMETSSerializer and BMechMETSSerializer will implement this to
// deal with the TYPE and PROFILE attributes.
protected abstract Attr[] getVariableRootAttrs()
throws BMechBuilderException;
// The BDefMETSSerializer and BMechMETSSerializer will implement this to
// deal with the specific datastream bindings required in each.
protected abstract Element[] getVariableStructMapDivs()
throws BMechBuilderException;
protected void serialize() throws BMechBuilderException
{
initializeTree();
genBaseMETS(bObjData);
finalizeTree();
}
protected void initializeTree() throws BMechBuilderException
{
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
factory.setValidating(false);
try
{
DocumentBuilder builder = factory.newDocumentBuilder();
document = builder.newDocument();
}
catch (ParserConfigurationException pce)
{
// Parser with specified options can't be built
pce.printStackTrace();
throw new BMechBuilderException("BObjMETSSerializer: " +
" Parser configuration exception initializing document builder.");
}
root = (Element)document.createElementNS(METS, "METS:mets");
//header = (Element)document.createElementNS(METS, "METS:metsHdr");
bObjFileSec = (Element)document.createElementNS(METS, "METS:fileSec");
bObjStructMap = (Element)document.createElementNS(METS, "METS:structMap");
bObjStructMap.setAttribute("ID", "S1");
bObjStructMap.setAttribute("TYPE", "fedora:dsBindingMap");
bObjBehaviorSec = (Element)document.createElementNS(METS, "METS:behaviorSec");
bObjBehaviorSec.setAttribute("ID", "DISS1");
bObjBehaviorSec.setAttribute("STATUS", "A");
}
protected void finalizeTree() throws BMechBuilderException
{
// put the tree together
document.appendChild(root);
//root.appendChild(header);
Element[] mdSecs = getInlineMD();
for (int i=0; i