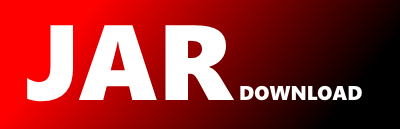
java.fedora.client.test.PerformanceTests Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fcrepo-client Show documentation
Show all versions of fcrepo-client Show documentation
The Fedora Client is a Java Library that allows API access to a Fedora Repository. The client is typically one part of a full Fedora installation.
The newest version!
/*
* -----------------------------------------------------------------------------
*
* License and Copyright: The contents of this file are subject to the
* Apache License, Version 2.0 (the "License"); you may not use
* this file except in compliance with the License. You may obtain a copy of
* the License at
* http://www.fedora-commons.org/licenses.
*
* Software distributed under the License is distributed on an "AS IS" basis,
* WITHOUT WARRANTY OF ANY KIND, either express or implied. See the License for
* the specific language governing rights and limitations under the License.
*
* The entire file consists of original code.
* Copyright © 2008 Fedora Commons, Inc.
*
Copyright © 2002-2007 The Rector and Visitors of the University of
* Virginia and Cornell University
* All rights reserved.
*
* -----------------------------------------------------------------------------
*/
package fedora.client.test;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
import java.io.InputStream;
import java.io.PrintStream;
import java.util.ArrayList;
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.math.BigDecimal;
import org.apache.axis.types.NonNegativeInteger;
import org.apache.commons.httpclient.HttpClient;
import org.apache.commons.httpclient.HttpMethod;
import org.apache.commons.httpclient.UsernamePasswordCredentials;
import org.apache.commons.httpclient.auth.AuthScope;
import org.apache.commons.httpclient.methods.GetMethod;
import fedora.client.FedoraClient;
import fedora.common.Constants;
import fedora.server.access.FedoraAPIA;
import fedora.server.management.FedoraAPIM;
/**
* Performs test to determine the time necessary to execute
* various repository activities.
*
* @author Bill Branan
*/
public class PerformanceTests
implements Constants {
private FedoraAPIM apim;
private FedoraAPIA apia;
private static int iterations = 10;
private static int threads = 10;
private static final String pid = "demo:performance";
private static String DEMO_FOXML_TEXT;
private static String datastream =
"http://localhost:8080/fedora-demo/simple-image-demo/coliseum-thumb.jpg";
private String host;
private String port;
private String username;
private String password;
private String[] PIDS;
private byte[][] FOXML;
static {
// Test FOXML object
StringBuilder sb = new StringBuilder();
sb.append("");
sb.append("");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ingest ");
sb.append(" ");
sb.append(" fedoraAdmin ");
sb.append(" 2008-07-09T19:28:04.890Z ");
sb.append(" Created New Object ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" Test Object ");
sb.append(" " + pid + " ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" Datastream Content ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" Datastream Content ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" PHhtbD5EYXRhc3RyZWFtIENvbnRlbnQ8L3htbD4=");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" PHhtbD5EYXRhc3RyZWFtIENvbnRlbnQ8L3htbD4=");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
DEMO_FOXML_TEXT = sb.toString();
}
public void init(String host, String port, String username, String password) throws Exception {
this.host = host;
this.port = port;
this.username = username;
this.password = password;
String baseURL = "http://" + host + ":" + port + "/fedora";
FedoraClient fedoraClient = new FedoraClient(baseURL, username, password);
apim = fedoraClient.getAPIM();
apia = fedoraClient.getAPIA();
PIDS = apim.getNextPID(new NonNegativeInteger(Integer.valueOf(iterations).toString()), "demo");
FOXML = new byte[iterations][];
for(int i=0; i 0) {
input = in.read();
}
}
/**
* @return average run time for a single operation
*/
public long runIngestTest() throws Exception {
long totalTime = 0;
long startTime = 0;
long stopTime = 0;
for(int i=0; i 0) {
input = in.read();
}
}
stopTime = System.currentTimeMillis();
totalTime = (stopTime - startTime);
runPurgeObject(PIDS[0]);
return totalTime;
}
private HttpMethod getHttpMethod(String pid) {
String url = "http://" + host + ":" + port + "/fedora/get/" + pid + "/" + "MDS1";
HttpMethod httpMethod = new GetMethod(url);
httpMethod.setDoAuthentication(true);
httpMethod.getParams().setParameter("Connection", "Keep-Alive");
return httpMethod;
}
private HttpClient getHttpClient() {
HttpClient client = new HttpClient();
client.getParams().setAuthenticationPreemptive(true);
client.getState().setCredentials(
new AuthScope(host, Integer.valueOf(port), "realm"),
new UsernamePasswordCredentials(username, password));
return client;
}
/**
* @return total time to run all iterations
* {ingest, addDatastream, modifyDatastreamByReference,
* modifyDatastreamByValue, purgeDatastream, purgeObject}
*/
public long[] runThroughputTests() throws Exception {
long ingestTime = 0;
long addDsTime = 0;
long modifyRefTime = 0;
long modifyValTime = 0;
long purgeDsTime = 0;
long purgeObjectTime = 0;
long startTime = 0;
long stopTime = 0;
// Ingest
startTime = System.currentTimeMillis();
for(int i=0; i> ingestRunnerList = new ArrayList>();
for(int i=0; i> addDatastreamRunnerList = new ArrayList>();
for(int i=0; i> modDatastreamRefRunnerList = new ArrayList>();
for(int i=0; i> modDatastreamValRunnerList = new ArrayList>();
for(int i=0; i> purgeDatastreamRunnerList = new ArrayList>();
for(int i=0; i> purgeObjectRunnerList = new ArrayList>();
for(int i=0; i> getDatastreamRunnerList = new ArrayList>();
for(int i=0; i> getDatastreamRestRunnerList = new ArrayList>();
for(int i=0; i {
MethodType methodType;
int index;
public MethodRunner(MethodType methodType, int index) {
this.methodType = methodType;
this.index = index;
}
public Boolean call() throws Exception {
if(methodType.equals(MethodType.INGEST)) {
runIngest(FOXML[index]);
}else if(methodType.equals(MethodType.ADD_DATASTREAM)) {
runAddDatastream(PIDS[index], "MDS3");
} else if(methodType.equals(MethodType.MODIFY_DATASTREAM_REF)) {
runModifyDatastreamByRef(PIDS[index]);
} else if(methodType.equals(MethodType.MODIFY_DATASTREAM_VAL)) {
runModifyDatastreamByValue(PIDS[index]);
} else if(methodType.equals(MethodType.PURGE_DATASTREAM)) {
runPurgeDatastream(PIDS[index], "MDS1");
} else if(methodType.equals(MethodType.PURGE_OBJECT)) {
runPurgeObject(PIDS[index]);
} else if(methodType.equals(MethodType.GET_DATASTREAM)) {
runGetDatastream(PIDS[index]);
} else if(methodType.equals(MethodType.GET_DATASTREAM_REST)) {
runGetDatastreamRest(PIDS[index]);
}
return new Boolean(true);
}
}
private static double round(double d) {
int decimalPlace = 5;
BigDecimal bd = new BigDecimal(Double.toString(d));
bd = bd.setScale(decimalPlace, BigDecimal.ROUND_HALF_UP);
return bd.doubleValue();
}
private static void usage() {
System.out.println("Runs a set of performance tests over a running Fedora repository.");
System.out.println("USAGE: ant performance-tests " +
"-Dhost=HOST " +
"-Dport=PORT " +
"-Dusername=USERNAME " +
"-Dpassword=PASSWORD " +
"-Diterations=NUM-ITERATIONS " +
"-Dthreads=NUM-THREADS " +
"-Dfile=OUTPUT-FILE " +
"-Dname=TEST-NAME");
System.out.println("Where:");
System.out.println(" HOST = Host on which Fedora server is running.");
System.out.println(" PORT = Port on which the Fedora server APIs can be accessed.");
System.out.println(" USERNAME = A fedora user with administrative privileges.");
System.out.println(" PASSWORD = The fedora user's password.");
System.out.println(" NUM-ITERATIONS = The number of times to perform each operation.");
System.out.println(" NUM-THREADS = The number of threads to use in the thread pool");
System.out.println(" when running threaded tests.");
System.out.println(" OUTPUT-FILE = The file to which the test results will be written.");
System.out.println(" If the file does not exist, it will be created, if the");
System.out.println(" file does exist the new results will be appended.");
System.out.println(" TEST-NAME = A name for this test run.");
System.out.println("Example:");
System.out.println("ant performance-tests " +
"-Dhost=localhost " +
"-Dport=8080 " +
"-Dusername=fedoraAdmin " +
"-Dpassword=fedoraAdmin " +
"-Diterations=100 " +
"-Dthreads=10 " +
"-Dfile=C:\\temp\\performance_testing_output.txt " +
"-Dname=\"Test 1\"");
System.exit(1);
}
public static void main(String[] args) throws Exception {
if(args.length != 8) {
usage();
}
String host = args[0];
String port = args[1];
String username = args[2];
String password = args[3];
String itr = args[4];
String thrds = args[5];
String output = args[6];
String name = args[7];
if(host == null || host.startsWith("$") ||
port == null || port.startsWith("$") ||
username == null || username.startsWith("$") ||
password == null || password.startsWith("$") ||
itr == null || itr.startsWith("$") ||
thrds == null || thrds.startsWith("$") ||
output == null || output.startsWith("$") ||
name == null || name.startsWith("$")) {
usage();
}
name = name.replaceAll(",", ";");
iterations = Integer.parseInt(itr);
threads = Integer.parseInt(thrds);
boolean newFile = true;
File outputFile = new File(output);
File tempFile = null;
BufferedReader reader = null;
String line = "";
if(outputFile.exists()) {
newFile = false;
// Create a copy of the file to read from
tempFile = File.createTempFile("performance-test", "tmp");
BufferedReader input = new BufferedReader(new FileReader(outputFile));
PrintStream tempOut = new PrintStream(tempFile);
while ((line = input.readLine()) != null) {
tempOut.println(line);
}
input.close();
tempOut.close();
reader = new BufferedReader(new FileReader(tempFile));
}
PrintStream out = new PrintStream(outputFile);
if(newFile) {
out.println("--------------------------------------------------------------" +
" Performance Test Results " +
"--------------------------------------------------------------");
}
PerformanceTests tests = new PerformanceTests();
tests.init(host, port, username, password);
System.out.println("Running Ingest Round-Trip Test...");
long ingestResults = tests.runIngestTest();
System.out.println("Running AddDatastream Round-Trip Test...");
long addDsResults = tests.runAddDatastreamTest();
System.out.println("Running ModifyDatastreamByReference Round-Trip Test...");
long modifyRefResults = tests.runModifyDatastreamByRefTest();
System.out.println("Running ModifyDatastreamByValue Round-Trip Test...");
long modifyValResults = tests.runModifyDatastreamByValueTest();
System.out.println("Running PurgeDatastream Round-Trip Test...");
long purgeDsResults = tests.runPurgeDatastreamTest();
System.out.println("Running PurgeObject Round-Trip Test...");
long purgeObjectResults = tests.runPurgeObjectTest();
System.out.println("Running GetDatastream Round-Trip Test...");
long getDatastreamResults = tests.runGetDatastreamTest();
System.out.println("Running GetDatastreamREST Round-Trip Test...");
long getDatastreamRestResults = tests.runGetDatastreamRestTest();
System.out.println("Running Throughput Tests...");
long[] tpResults = tests.runThroughputTests();
System.out.println("Running Threaded Throughput Tests...");
long[] tptResults = tests.runThreadedThroughputTests();
if(newFile) {
out.println("1. Test performing each operation in isolation. Time (in ms) is the average required to perform each operation.");
out.println("test name, ingest, addDatastream, modifyDatastreamByReference, modifyDatastreamByValue, purgeDatastream, purgeObject, getDatastream, getDatastreamREST");
} else {
line = reader.readLine();
while (line != null && line.length() > 2) {
out.println(line);
line = reader.readLine();
}
}
out.println(name + ", " +
ingestResults + ", " +
addDsResults + ", " +
modifyRefResults + ", " +
modifyValResults + ", " +
purgeDsResults + ", " +
purgeObjectResults + ", " +
getDatastreamResults/iterations + ", " +
getDatastreamRestResults/iterations);
out.println();
if(newFile) {
out.println("2. Operations-Per-Second based on results listed in item 1.");
out.println("test name, ingest, addDatastream, modifyDatastreamByReference, modifyDatastreamByValue, purgeDatastream, purgeObject, getDatastream, getDatastreamREST");
} else {
line = reader.readLine();
while (line != null && line.length() > 2) {
out.println(line);
line = reader.readLine();
}
}
double ingestPerSecond = 1000/(double)ingestResults;
double addDsPerSecond = 1000/(double)addDsResults;
double modifyRefPerSecond = 1000/(double)modifyRefResults;
double modifyValPerSecond = 1000/(double)modifyValResults;
double purgeDsPerSecond = 1000/(double)purgeDsResults;
double purgeObjPerSecond = 1000/(double)purgeObjectResults;
double getDatastreamPerSecond = 1000/((double)getDatastreamResults/iterations);
double getDatastreamRestPerSecond = 1000/((double)getDatastreamRestResults/iterations);
out.println(name + ", " +
round(ingestPerSecond) + ", " +
round(addDsPerSecond) + ", " +
round(modifyRefPerSecond) + ", " +
round(modifyValPerSecond) + ", " +
round(purgeDsPerSecond) + ", " +
round(purgeObjPerSecond) + ", " +
round(getDatastreamPerSecond) + ", " +
round(getDatastreamRestPerSecond));
out.println();
if(newFile) {
out.println("3. Test performing operations back-to-back. Time (in ms) is that required to perform all iterations.");
out.println("test name, ingest, addDatastream, modifyDatastreamByReference, modifyDatastreamByValue, purgeDatastream, purgeObject, getDatastream, getDatastreamREST");
} else {
line = reader.readLine();
while (line != null && line.length() > 2) {
out.println(line);
line = reader.readLine();
}
}
out.println(name + ", " +
tpResults[0] + ", " +
tpResults[1] + ", " +
tpResults[2] + ", " +
tpResults[3] + ", " +
tpResults[4] + ", " +
tpResults[5] + ", " +
getDatastreamResults + ", " +
getDatastreamRestResults);
out.println();
if(newFile) {
out.println("4. Operations-Per-Second based on results listed in item 3.");
out.println("test name, ingest, addDatastream, modifyDatastreamByReference, modifyDatastreamByValue, purgeDatastream, purgeObject, getDatastream, getDatastreamREST");
} else {
line = reader.readLine();
while (line != null && line.length() > 2) {
out.println(line);
line = reader.readLine();
}
}
double ingestItPerSecond = (double)(iterations * 1000)/tpResults[0];
double addDsItPerSecond = (double)(iterations * 1000)/tpResults[1];
double modifyRefItPerSecond = (double)(iterations * 1000)/tpResults[2];
double modifyValItPerSecond = (double)(iterations * 1000)/tpResults[3];
double purgeDsItPerSecond = (double)(iterations * 1000)/tpResults[4];
double purgeObjItPerSecond = (double)(iterations * 1000)/tpResults[5];
double getDsItPerSecond = (double)(iterations * 1000)/getDatastreamResults;
double getDsRestItPerSecond = (double)(iterations * 1000)/getDatastreamRestResults;
out.println(name + ", " +
round(ingestItPerSecond) + ", " +
round(addDsItPerSecond) + ", " +
round(modifyRefItPerSecond) + ", " +
round(modifyValItPerSecond) + ", " +
round(purgeDsItPerSecond) + ", " +
round(purgeObjItPerSecond) + ", " +
round(getDsItPerSecond) + ", " +
round(getDsRestItPerSecond));
out.println();
if(newFile) {
out.println("5. Test performing operations using a thread pool. Time (in ms) is that required to perform all iterations.");
out.println("test name, ingest, addDatastream, modifyDatastreamByReference, modifyDatastreamByValue, purgeDatastream, purgeObject, getDatastream, getDatastreamREST");
} else {
line = reader.readLine();
while (line != null && line.length() > 2) {
out.println(line);
line = reader.readLine();
}
}
out.println(name + ", " +
tptResults[0] + ", " +
tptResults[1] + ", " +
tptResults[2] + ", " +
tptResults[3] + ", " +
tptResults[4] + ", " +
tptResults[5] + ", " +
tptResults[6] + ", " +
tptResults[7]);
out.println();
if(newFile) {
out.println("6. Operations-Per-Second based on results listed in item 5.");
out.println("test name, ingest, addDatastream, modifyDatastreamByReference, modifyDatastreamByValue, purgeDatastream, purgeObject, getDatastream, getDatastreamREST");
} else {
line = reader.readLine();
while (line != null && line.length() > 2) {
out.println(line);
line = reader.readLine();
}
}
double thrdIngestItPerSecond = (double)(iterations * 1000)/tptResults[0];
double thrdAddDsItPerSecond = (double)(iterations * 1000)/tptResults[1];
double thrdModifyRefItPerSecond = (double)(iterations * 1000)/tptResults[2];
double thrdModifyValItPerSecond = (double)(iterations * 1000)/tptResults[3];
double thrdPurgeDsItPerSecond = (double)(iterations * 1000)/tptResults[4];
double thrdPurgeObjItPerSecond = (double)(iterations * 1000)/tptResults[5];
double thrdGetDsItPerSecond = (double)(iterations * 1000)/tptResults[6];
double thrdGetDsRestItPerSecond = (double)(iterations * 1000)/tptResults[7];
out.println(name + ", " +
round(thrdIngestItPerSecond) + ", " +
round(thrdAddDsItPerSecond) + ", " +
round(thrdModifyRefItPerSecond) + ", " +
round(thrdModifyValItPerSecond) + ", " +
round(thrdPurgeDsItPerSecond) + ", " +
round(thrdPurgeObjItPerSecond) + ", " +
round(thrdGetDsItPerSecond) + ", " +
round(thrdGetDsRestItPerSecond));
if(!newFile){
reader.close();
tempFile.delete();
}
out.close();
System.out.println("Performance Tests Complete.");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy