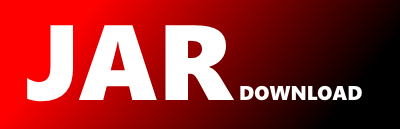
java.fedora.client.utility.ingest.IngestLogger Maven / Gradle / Ivy
Show all versions of fcrepo-client Show documentation
/*
* -----------------------------------------------------------------------------
*
* License and Copyright: The contents of this file are subject to the
* Apache License, Version 2.0 (the "License"); you may not use
* this file except in compliance with the License. You may obtain a copy of
* the License at
* http://www.fedora-commons.org/licenses.
*
* Software distributed under the License is distributed on an "AS IS" basis,
* WITHOUT WARRANTY OF ANY KIND, either express or implied. See the License for
* the specific language governing rights and limitations under the License.
*
* The entire file consists of original code.
* Copyright © 2008 Fedora Commons, Inc.
*
Copyright © 2002-2007 The Rector and Visitors of the University of
* Virginia and Cornell University
* All rights reserved.
*
* -----------------------------------------------------------------------------
*/
package fedora.client.utility.ingest;
import java.io.*;
import fedora.common.Constants;
import fedora.server.utilities.StreamUtility;
/**
* Title: IngestLogger.java
* Description: Methods to create and update a log of ingest activity.
*
*/
public class IngestLogger {
public static File newLogFile(String logRootName){
String fileName=logRootName + "-" + System.currentTimeMillis() + ".xml";
File outFile;
String fedoraHome = Constants.FEDORA_HOME;
if (fedoraHome == null) {
// to current dir
outFile=new File(fileName);
} else {
// to client/log
File logDir=new File(new File(new File(fedoraHome), "client"), "logs");
if (!logDir.exists()) {
logDir.mkdir();
}
outFile=new File(logDir, fileName);
}
return outFile;
}
public static void openLog(PrintStream log, String rootName) throws Exception {
log.println("");
log.println("<" + rootName + ">");
}
public static void logFromFile(PrintStream log, File f, String fType, String pid)
throws Exception {
log.println(" ");
}
public static void logFromFile(PrintStream log, File f, char fType, String pid)
throws Exception {
log.println(" ");
}
public static void logFailedFromFile(PrintStream log, File f, String fType, Exception e)
throws Exception {
String message=e.getMessage();
if (message==null) message=e.getClass().getName();
log.println(" ");
log.println(" " + StreamUtility.enc(message));
log.println(" ");
}
public static void logFailedFromFile(PrintStream log, File f, char fType, Exception e)
throws Exception {
String message=e.getMessage();
if (message==null) message=e.getClass().getName();
log.println(" ");
log.println(" " + StreamUtility.enc(message));
log.println(" ");
}
public static void logFromRepos(PrintStream log, String sourcePID, String fType, String targetPID)
throws Exception {
log.println(" ");
}
public static void logFromRepos(PrintStream log, String sourcePID, char fType, String targetPID)
throws Exception {
log.println(" ");
}
public static void logFailedFromRepos(PrintStream log, String sourcePID, String fType, Exception e)
throws Exception {
String message=e.getMessage();
if (message==null) message=e.getClass().getName();
log.println(" ");
log.println(" " + StreamUtility.enc(message));
log.println(" ");
}
public static void logFailedFromRepos(PrintStream log, String sourcePID, char fType, Exception e)
throws Exception {
String message=e.getMessage();
if (message==null) message=e.getClass().getName();
log.println(" ");
log.println(" " + StreamUtility.enc(message));
log.println(" ");
}
public static void closeLog(PrintStream log, String rootName) throws Exception {
log.println("" + rootName + ">");
log.close();
}
}