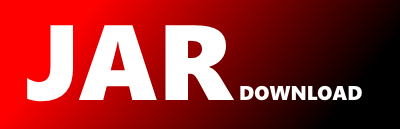
java.fedora.localservices.fop.FOPServlet Maven / Gradle / Ivy
Show all versions of fcrepo-client Show documentation
/*
* -----------------------------------------------------------------------------
*
* License and Copyright: The contents of this file are subject to the
* Apache License, Version 2.0 (the "License"); you may not use
* this file except in compliance with the License. You may obtain a copy of
* the License at
* http://www.fedora-commons.org/licenses.
*
* Software distributed under the License is distributed on an "AS IS" basis,
* WITHOUT WARRANTY OF ANY KIND, either express or implied. See the License for
* the specific language governing rights and limitations under the License.
*
* The entire file consists of original code.
* Copyright © 2008 Fedora Commons, Inc.
*
Copyright © 2002-2007 The Rector and Visitors of the University of
* Virginia and Cornell University
* All rights reserved.
*
* -----------------------------------------------------------------------------
*/
package fedora.localservices.fop;
import java.io.*;
import javax.servlet.*;
import javax.servlet.http.*;
import org.xml.sax.InputSource;
import org.apache.fop.apps.Driver;
import org.apache.fop.messaging.MessageHandler;
import org.apache.avalon.framework.logger.ConsoleLogger;
import org.apache.avalon.framework.logger.Logger;
/**
*
* Title: FOPServlet.java
* Description: Servlet for generating and serving a PDF, given the
* URL to an XSL-FO file.
* Servlet param is:
*
* - source: the path to a formatting object file to render
*
*
* @author [email protected]
* @version $Id: FOPServlet.java 5162 2006-10-25 00:49:06Z eddie $
*/
public class FOPServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
public static final String FO_REQUEST_PARAM = "source";
Logger log = null;
public void doGet(HttpServletRequest request,
HttpServletResponse response) throws ServletException {
if (log == null) {
log = new ConsoleLogger(ConsoleLogger.LEVEL_WARN);
MessageHandler.setScreenLogger(log);
}
try {
String foParam = request.getParameter(FO_REQUEST_PARAM);
if (foParam != null) {
renderFO(new InputSource(foParam), response);
} else {
PrintWriter out = response.getWriter();
out.println("Error \n"+
"FOPServlet Error
No 'source' "+
"request param given.");
}
} catch (ServletException ex) {
throw ex;
}
catch (Exception ex) {
throw new ServletException(ex);
}
}
public void renderFO(InputSource foFile,
HttpServletResponse response) throws ServletException {
try {
ByteArrayOutputStream out = new ByteArrayOutputStream();
response.setContentType("application/pdf");
Driver driver = new Driver(foFile, out);
driver.setLogger(log);
driver.setRenderer(Driver.RENDER_PDF);
driver.run();
byte[] content = out.toByteArray();
response.setContentLength(content.length);
response.getOutputStream().write(content);
response.getOutputStream().flush();
} catch (Exception ex) {
throw new ServletException(ex);
}
}
}