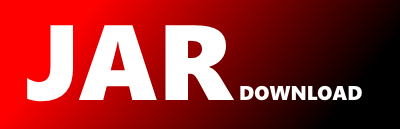
java.fedora.oai.sample.SampleOAIProvider Maven / Gradle / Ivy
/*
* -----------------------------------------------------------------------------
*
* License and Copyright: The contents of this file are subject to the
* Apache License, Version 2.0 (the "License"); you may not use
* this file except in compliance with the License. You may obtain a copy of
* the License at
* http://www.fedora-commons.org/licenses.
*
* Software distributed under the License is distributed on an "AS IS" basis,
* WITHOUT WARRANTY OF ANY KIND, either express or implied. See the License for
* the specific language governing rights and limitations under the License.
*
* The entire file consists of original code.
* Copyright © 2008 Fedora Commons, Inc.
*
Copyright © 2002-2007 The Rector and Visitors of the University of
* Virginia and Cornell University
* All rights reserved.
*
* -----------------------------------------------------------------------------
*/
package fedora.oai.sample;
import java.util.ArrayList;
import java.util.Date;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import fedora.oai.BadResumptionTokenException;
import fedora.oai.DateGranularitySupport;
import fedora.oai.DeletedRecordSupport;
import fedora.oai.IDDoesNotExistException;
import fedora.oai.OAIProvider;
import fedora.oai.Record;
import fedora.oai.SimpleHeader;
import fedora.oai.SimpleMetadataFormat;
import fedora.oai.SimpleRecord;
import fedora.oai.SimpleSetInfo;
/**
*
* Title: SampleOAIProvider.java
* Description: A sample implementation of OAIProvider for testing and demonstration
* purposes.
*
* @author [email protected]
* @version $Id: SampleOAIProvider.java 5166 2006-10-25 11:05:45Z eddie $
*/
public class SampleOAIProvider
implements OAIProvider {
private static String s_rec1_identifier="sample:1";
private static String s_rec1_metadata=" \n"
+ " Using Structural Metadata to Localize Experience of \n"
+ " Digital Content \n"
+ " Dushay, Naomi \n"
+ " Digital Libraries \n"
+ " With the increasing technical sophistication of\n"
+ " both information consumers and providers, there is\n"
+ " increasing demand for more meaningful experiences of digital\n"
+ " information. We present a framework that separates digital\n"
+ " object experience, or rendering, from digital object storage\n"
+ " and manipulation, so the rendering can be tailored to\n"
+ " particular communities of users.\n"
+ " \n"
+ " Comment: 23 pages including 2 appendices,\n"
+ " 8 figures \n"
+ " 2001-12-14 \n"
+ " ";
private static String s_rec1_about=" \n"
+ " \n"
+ " http://some.oa.org \n"
+ " oai:r2.org:klik001 \n"
+ " 2001-01-01 \n"
+ " http://www.openarchives.org/OAI/2.0/oai_dc/ \n"
+ " \n"
+ " ";
private SimpleHeader m_head1;
private SimpleRecord m_rec1;
public SampleOAIProvider() {
HashSet s=new HashSet();
s.add("cs");
s.add("cornell");
m_head1=new SimpleHeader(s_rec1_identifier, new Date(), s, true);
HashSet a=new HashSet();
a.add(s_rec1_about);
m_rec1=new SimpleRecord(m_head1, s_rec1_metadata, a);
}
public String getRepositoryName() {
return "My Repository";
}
public String getBaseURL(String protocol, String port) {
return protocol + "://localhost:" + port + "/path/to/servlet";
}
public String getProtocolVersion() {
return "2.0";
}
public Date getEarliestDatestamp() {
return new Date();
}
public DeletedRecordSupport getDeletedRecordSupport() {
return DeletedRecordSupport.NO;
}
public DateGranularitySupport getDateGranularitySupport() {
return DateGranularitySupport.SECONDS;
}
public Set getAdminEmails() {
HashSet s=new HashSet();
s.add("[email protected]");
return s;
}
public Set getSupportedCompressionEncodings() {
return new HashSet();
}
public Set getDescriptions() {
return new HashSet();
}
public Record getRecord(String identifier, String metadataPrefix)
throws IDDoesNotExistException {
// throws CannotDisseminateFormatException, IDDoesNotExistException;
if (identifier.equals("sample:1")) {
return m_rec1;
} else {
throw new IDDoesNotExistException("An item with that id was not found.");
}
}
public List getRecords(Date from, Date until, String metadataPrefix,
String set) {
// throws CannotDisseminateFormatException,
// NoRecordsMatchException, NoSetHierarchyException;
ArrayList a=new ArrayList();
a.add(m_rec1);
return a;
}
public List getRecords(String resumptionToken)
throws BadResumptionTokenException {
throw new BadResumptionTokenException("Sample doesn't support resumptionTokens.");
}
public List getHeaders(Date from, Date until, String metadataPrefix,
String set) {
ArrayList a=new ArrayList();
a.add(m_head1);
return a;
}
public List getHeaders(String resumptionToken)
throws BadResumptionTokenException {
throw new BadResumptionTokenException("Sample doesn't support resumptionTokens.");
}
public List getSets() {
ArrayList a=new ArrayList();
a.add(new SimpleSetInfo("Computer Science", "cs", new HashSet()));
a.add(new SimpleSetInfo("Cornell University", "cornell", new HashSet()));
return a;
}
public List getSets(String resumptionToken)
throws BadResumptionTokenException {
throw new BadResumptionTokenException("Sample doesn't support resumptionTokens.");
}
public Set getMetadataFormats(String id) {
HashSet s=new HashSet();
s.add(new SimpleMetadataFormat("oai_dc",
"http://www.openarchives.org/OAI/2.0/oai_dc.xsd",
"http://www.openarchives.org/OAI/2.0/oai_dc/"));
return s;
}
public long getMaxSets() {
return 10;
}
public long getMaxRecords() {
return 10;
}
public long getMaxHeaders() {
return 10;
}
}