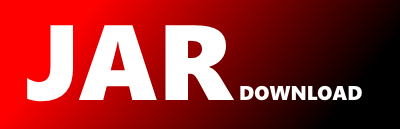
java.fedora.server.config.webxml.WebXML Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fcrepo-client Show documentation
Show all versions of fcrepo-client Show documentation
The Fedora Client is a Java Library that allows API access to a Fedora Repository. The client is typically one part of a full Fedora installation.
The newest version!
/*
* -----------------------------------------------------------------------------
*
* License and Copyright: The contents of this file are subject to the
* Apache License, Version 2.0 (the "License"); you may not use
* this file except in compliance with the License. You may obtain a copy of
* the License at
* http://www.fedora-commons.org/licenses.
*
* Software distributed under the License is distributed on an "AS IS" basis,
* WITHOUT WARRANTY OF ANY KIND, either express or implied. See the License for
* the specific language governing rights and limitations under the License.
*
* The entire file consists of original code.
* Copyright © 2008 Fedora Commons, Inc.
*
Copyright © 2002-2007 The Rector and Visitors of the University of
* Virginia and Cornell University
* All rights reserved.
*
* -----------------------------------------------------------------------------
*/
package fedora.server.config.webxml;
import java.beans.IntrospectionException;
import java.io.File;
import java.io.IOException;
import java.io.Serializable;
import java.io.Writer;
import java.util.ArrayList;
import java.util.List;
import org.apache.commons.betwixt.io.BeanReader;
import org.apache.commons.betwixt.io.BeanWriter;
import org.apache.commons.betwixt.strategy.NamespacePrefixMapper;
import org.xml.sax.InputSource;
import org.xml.sax.SAXException;
public class WebXML
implements Serializable {
private static final long serialVersionUID = 1L;
private static final String BETWIXT_MAPPING =
"/fedora/server/config/webxml/webxml-mapping.xml";
private String id;
private String version;
private String displayName;
private final List servlets;
private final List servletMappings;
private final List filters;
private final List filterMappings;
private final List securityConstraints;
private WelcomeFileList welcomeFileList;
private final List errorPages;
private LoginConfig loginConfig;
private final List securityRoles;
public WebXML() {
servlets = new ArrayList();
servletMappings = new ArrayList();
filters = new ArrayList();
filterMappings = new ArrayList();
securityConstraints = new ArrayList();
errorPages = new ArrayList();
securityRoles = new ArrayList();
}
public static WebXML getInstance() {
return new WebXML();
}
/**
* Create an instance of WebXML from the specified file.
*
* @param webxml
* Path to web.xml file.
* @return instance of WebXML
*/
public static WebXML getInstance(String webxml) {
WebXML wx = null;
BeanReader reader = new BeanReader();
reader.getXMLIntrospector().getConfiguration()
.setAttributesForPrimitives(false);
reader.getBindingConfiguration().setMapIDs(false);
try {
reader.registerMultiMapping(getBetwixtMapping());
wx = (WebXML) reader.parse(new File(webxml).toURI().toString());
} catch (IntrospectionException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (SAXException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return wx;
}
public String getDisplayName() {
return displayName;
}
public void setDisplayName(String displayName) {
this.displayName = displayName;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getVersion() {
return version;
}
public void setVersion(String version) {
this.version = version;
}
public List getServlets() {
return servlets;
}
public void addServlet(Servlet servlet) {
servlets.add(servlet);
}
public List getServletMappings() {
return servletMappings;
}
public void addServletMapping(ServletMapping servletMapping) {
servletMappings.add(servletMapping);
}
public List getFilters() {
return filters;
}
public void addFilter(Filter filter) {
filters.add(filter);
}
public List getFilterMappings() {
return filterMappings;
}
public void addFilterMapping(FilterMapping filterMapping) {
filterMappings.add(filterMapping);
}
public void removeFilterMapping(FilterMapping filterMapping) {
filterMappings.remove(filterMapping);
}
public List getSecurityConstraints() {
return securityConstraints;
}
public void addSecurityConstraint(SecurityConstraint securityConstraint) {
securityConstraints.add(securityConstraint);
}
public void removeSecurityConstraint(SecurityConstraint securityConstraint) {
securityConstraints.remove(securityConstraint);
}
public WelcomeFileList getWelcomeFileList() {
return welcomeFileList;
}
public void setWelcomeFileList(WelcomeFileList welcomeFileList) {
this.welcomeFileList = welcomeFileList;
}
public List getErrorPages() {
return errorPages;
}
public void addErrorPage(ErrorPage errorPage) {
errorPages.add(errorPage);
}
public LoginConfig getLoginConfig() {
return loginConfig;
}
public void setLoginConfig(LoginConfig loginConfig) {
this.loginConfig = loginConfig;
}
public List getSecurityRoles() {
return securityRoles;
}
public void addSecurityRole(SecurityRole securityRole) {
securityRoles.add(securityRole);
}
public void write(Writer outputWriter) throws IOException {
//
NamespacePrefixMapper nspm = new NamespacePrefixMapper();
nspm.setPrefix("http://www.w3.org/2001/XMLSchema-instance", "xsi");
nspm.setPrefix("http://java.sun.com/xml/ns/j2ee", "xmlns");
//
outputWriter.write("\n");
BeanWriter beanWriter = new BeanWriter(outputWriter);
beanWriter.getBindingConfiguration().setMapIDs(false);
beanWriter.setWriteEmptyElements(false);
beanWriter.enablePrettyPrint();
try {
beanWriter.getXMLIntrospector().register(getBetwixtMapping());
beanWriter.getXMLIntrospector().getConfiguration()
.setPrefixMapper(nspm);
beanWriter.write("web-app", this);
} catch (IntrospectionException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (SAXException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
beanWriter.flush();
beanWriter.close();
}
private static InputSource getBetwixtMapping() {
return new InputSource(WebXML.class
.getResourceAsStream(BETWIXT_MAPPING));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy