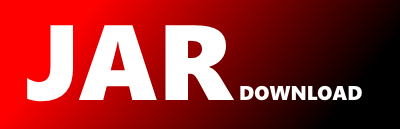
java.fedora.server.management.BasicPIDGenerator Maven / Gradle / Ivy
/*
* -----------------------------------------------------------------------------
*
* License and Copyright: The contents of this file are subject to the
* Apache License, Version 2.0 (the "License"); you may not use
* this file except in compliance with the License. You may obtain a copy of
* the License at
* http://www.fedora-commons.org/licenses.
*
* Software distributed under the License is distributed on an "AS IS" basis,
* WITHOUT WARRANTY OF ANY KIND, either express or implied. See the License for
* the specific language governing rights and limitations under the License.
*
* The entire file consists of original code.
* Copyright © 2008 Fedora Commons, Inc.
*
Copyright © 2002-2007 The Rector and Visitors of the University of
* Virginia and Cornell University
* All rights reserved.
*
* -----------------------------------------------------------------------------
*/
package fedora.server.management;
import java.io.File;
import java.io.IOException;
import java.util.Map;
import org.apache.log4j.Logger;
import fedora.common.PID;
import fedora.server.Module;
import fedora.server.Server;
import fedora.server.errors.ConnectionPoolNotFoundException;
import fedora.server.errors.ModuleInitializationException;
import fedora.server.storage.ConnectionPoolManager;
/**
* A wrapper around the DBPIDGenerator class that casts it as a Module.
*
* @author [email protected]
* @version $Id: BasicPIDGenerator.java 5227 2006-11-25 18:48:50Z cwilper $
*/
public class BasicPIDGenerator
extends Module implements PIDGenerator {
/** Logger for this class. */
private static final Logger LOG = Logger.getLogger(
BasicPIDGenerator.class.getName());
private DBPIDGenerator m_pidGenerator;
private File m_oldPidGenDir;
/**
* Constructs a BasicPIDGenerator.
*
* @param moduleParameters A pre-loaded Map of name-value pairs comprising
* the intended configuration of this Module.
* @param server The Server
instance.
* @param role The role this module fulfills, a java class name.
* @throws ModuleInitializationException If initilization values are
* invalid or initialization fails for some other reason.
*/
public BasicPIDGenerator(Map moduleParameters, Server server, String role)
throws ModuleInitializationException {
super(moduleParameters, server, role);
}
public void initModule() {
// this parameter is no longer required; but if it's specified,
// we can automatically upgrade from a pre-1.2 version of Fedora by
// making sure the old "last pid generated" value is respected later.
String dir=getParameter("pidgen_log_dir");
if (dir!=null && !dir.equals("")) {
if (dir.startsWith("/")
|| dir.startsWith("\\")
|| dir.substring(1).startsWith(":\\")) {
m_oldPidGenDir=new File(dir);
} else {
m_oldPidGenDir=new File(getServer().getHomeDir(), dir);
}
}
}
/**
* Get a reference to the ConnectionPoolManager so we can give the
* instance constructor a ConnectionPool later in initializeIfNeeded().
*/
public void postInitModule()
throws ModuleInitializationException {
ConnectionPoolManager mgr = (ConnectionPoolManager) getServer()
.getModule("fedora.server.storage.ConnectionPoolManager");
if (mgr==null) {
throw new ModuleInitializationException(
"ConnectionPoolManager module not loaded.", getRole());
}
try {
m_pidGenerator=new DBPIDGenerator(mgr.getPool(), m_oldPidGenDir);
} catch (Exception e) {
String msg = "Can't get default connection pool";
LOG.fatal(msg, e);
throw new ModuleInitializationException(msg, getRole());
}
}
public PID generatePID(String namespaceID)
throws IOException {
return m_pidGenerator.generatePID(namespaceID);
}
public PID getLastPID()
throws IOException {
return m_pidGenerator.getLastPID();
}
public void neverGeneratePID(String pid)
throws IOException {
m_pidGenerator.neverGeneratePID(pid);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy