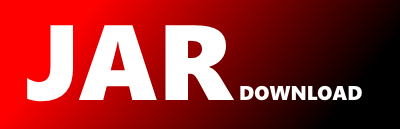
java.fedora.server.security.BackendPolicies Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fcrepo-client Show documentation
Show all versions of fcrepo-client Show documentation
The Fedora Client is a Java Library that allows API access to a Fedora Repository. The client is typically one part of a full Fedora installation.
The newest version!
/*
* -----------------------------------------------------------------------------
*
* License and Copyright: The contents of this file are subject to the
* Apache License, Version 2.0 (the "License"); you may not use
* this file except in compliance with the License. You may obtain a copy of
* the License at
* http://www.fedora-commons.org/licenses.
*
* Software distributed under the License is distributed on an "AS IS" basis,
* WITHOUT WARRANTY OF ANY KIND, either express or implied. See the License for
* the specific language governing rights and limitations under the License.
*
* The entire file consists of original code.
* Copyright © 2008 Fedora Commons, Inc.
*
Copyright © 2002-2007 The Rector and Visitors of the University of
* Virginia and Cornell University
* All rights reserved.
*
* -----------------------------------------------------------------------------
*/
/*
* Created on May 4, 2005
*
*/
package fedora.server.security;
import java.io.File;
import java.io.FileOutputStream;
import java.io.PrintStream;
import java.util.Hashtable;
import java.util.Iterator;
import java.util.Set;
import org.apache.log4j.Logger;
import fedora.common.PID;
/**
* @author [email protected]
*/
public class BackendPolicies {
/** Logger for this class. */
private static final Logger LOG = Logger.getLogger(
BackendPolicies.class.getName());
public static final String FEDORA_INTERNAL_CALL = "fedoraInternalCall-1";
public static final String BACKEND_SERVICE_CALL_UNSECURE = "fedoraInternalCall-2";
private String inFilePath = null;
private String outFilePath = null;
private BackendSecuritySpec backendSecuritySpec = null;
public BackendPolicies(String inFilePath, String outFilePath) {
this.inFilePath = inFilePath;
this.outFilePath = outFilePath;
}
public BackendPolicies(String inFilePath) {
this(inFilePath, null);
}
public Hashtable generateBackendPolicies() throws Exception {
LOG.debug("in BackendPolicies.generateBackendPolicies() 1");
Hashtable tempfiles = null;
if (inFilePath.endsWith(".xml")) { // replacing code for .properties
LOG.debug("in BackendPolicies.generateBackendPolicies() .xml 1");
BackendSecurityDeserializer bds = new BackendSecurityDeserializer("UTF-8", false);
LOG.debug("in BackendPolicies.generateBackendPolicies() .xml 2");
backendSecuritySpec = bds.deserialize(inFilePath);
LOG.debug("in BackendPolicies.generateBackendPolicies() .xml 3");
tempfiles = writePolicies();
LOG.debug("in BackendPolicies.generateBackendPolicies() .xml 4");
}
return tempfiles;
}
private static final String[] parseForSlash(String key) throws Exception {
int lastSlash = key.lastIndexOf("/");
if (lastSlash+1 == key.length()) {
throw new Exception("BackendPolicies.newWritePolicies() " + "can't handle key ending with '/'");
}
if (lastSlash != key.indexOf("/")) {
throw new Exception("BackendPolicies.newWritePolicies() " + "can't handle key containing multiple instances of '/'");
}
String[] parts = null;
if ((-1 < lastSlash) && (lastSlash < key.length())) {
parts = key.split("/");
} else {
parts = new String[] {key};
}
return parts;
}
private static final String getExcludedRolesText(String key, Set roles) {
StringBuffer excludedRolesText = new StringBuffer();
if ("default".equals(key) && (roles.size() > 1)) {
excludedRolesText.append("\t\t\n");
Iterator excludedRoleIterator = roles.iterator();
while (excludedRoleIterator.hasNext()) {
LOG.debug("in BackendPolicies.newWritePolicies() another inner it");
String excludedRole = (String) excludedRoleIterator.next();
if ("default".equals(excludedRole)) {
continue;
}
LOG.debug("in BackendPolicies.newWritePolicies() excludedRole=" + excludedRole);
excludedRolesText.append("\t\t\t");
excludedRolesText.append(excludedRole);
excludedRolesText.append(" \n");
}
excludedRolesText.append("\t\t \n");
}
return excludedRolesText.toString();
}
private static final String writeRules(String callbackBasicAuth, String callbackSsl, String iplist, String role, Set roles) throws Exception {
StringBuffer temp = new StringBuffer();
temp.append("\t\n");
temp.append(getExcludedRolesText(role, roles));
if ("true".equals(callbackBasicAuth)) {
temp.append("\t\t \n");
}
if ("true".equals(callbackSsl)) {
temp.append("\t\t \n");
}
LOG.debug("DEBUGGING IPREGEX0 [" + iplist + "]");
String[] ipRegexes = new String[0];
if ((iplist != null) && ! "".equals(iplist.trim())) {
ipRegexes = iplist.trim().split("\\s");
}
/*
if (ipRegexes.length == 1) { //fixup
ipRegexes[0] = ipRegexes[0].trim();
}
*/
LOG.debug("DEBUGGING IPREGEX1 [" + iplist.trim() + "]");
if (ipRegexes.length != 0) {
temp.append("\t\t\n");
for (int i = 0; i < ipRegexes.length; i++) {
LOG.debug("DEBUGGING IPREGEX2 " + ipRegexes[i]);
temp.append("\t\t\t");
temp.append(ipRegexes[i]);
temp.append(" \n");
}
temp.append("\t\t \n");
}
temp.append("\t \n");
if (("true".equals(callbackBasicAuth))
|| ("true".equals(callbackSsl))
|| (ipRegexes.length != 0)) {
temp.append("\t\n");
temp.append(getExcludedRolesText(role, roles));
temp.append("\t \n");
}
return temp.toString();
}
private Hashtable writePolicies() throws Exception {
LOG.debug("in BackendPolicies.newWritePolicies() 1");
StringBuffer sb = null;
Hashtable tempfiles = new Hashtable();
Iterator coarseIterator = backendSecuritySpec.listRoleKeys().iterator();
while (coarseIterator.hasNext()) {
String key = (String) coarseIterator.next();
String[] parts = parseForSlash(key);
String filename1 = "";
String filename2 = "";
switch (parts.length) {
case 2:
filename2 = "-method-" + parts[1];
//break purposely absent: fall through
case 1:
if (-1 == parts[0].indexOf(":")) {
filename1 = "callback-by:" + parts[0];
} else {
filename1 = "callback-by-bmech-" + parts[0];
}
if ("".equals(filename2)) {
if (! "default".equals(parts[0])) {
filename2 = "-other-methods";
}
}
break;
default:
//bad value
throw new Exception("BackendPolicies.newWritePolicies() " + "didn't correctly parse key " + key);
}
sb = new StringBuffer();
LOG.debug("in BackendPolicies.newWritePolicies() another outer it, key=" + key);
Hashtable properties = backendSecuritySpec.getSecuritySpec(key);
LOG.debug("in BackendPolicies.newWritePolicies() properties.size()=" + properties.size());
LOG.debug("in BackendPolicies.newWritePolicies() properties.get(BackendSecurityDeserializer.ROLE)=" + properties.get(BackendSecurityDeserializer.ROLE));
String callbackBasicAuth = (String) properties.get(BackendSecurityDeserializer.CALLBACK_BASIC_AUTH);
if (callbackBasicAuth == null) {
callbackBasicAuth = "false";
}
LOG.debug("in BackendPolicies.newWritePolicies() CallbackBasicAuth=" + callbackBasicAuth);
String callbackSsl = (String) properties.get(BackendSecurityDeserializer.CALLBACK_SSL);
if (callbackSsl == null) {
callbackSsl = "false";
}
String iplist = (String) properties.get(BackendSecurityDeserializer.IPLIST);
if (iplist == null) {
iplist = "";
}
LOG.debug("in BackendPolicies.newWritePolicies() coarseIplist=" + iplist);
String id = "generated_for_" + key.replace(':','-');
LOG.debug("in BackendPolicies.newWritePolicies() id=" + id);
LOG.debug("in BackendPolicies.newWritePolicies() " + filename1 + " " + filename2);
String filename = filename1 + filename2; //was id.replace(':','-');
LOG.debug("in BackendPolicies.newWritePolicies() " + filename);
PID tempPid = new PID(filename);
LOG.debug("in BackendPolicies.newWritePolicies() got PID " + tempPid);
filename = tempPid.toFilename();
LOG.debug("in BackendPolicies.newWritePolicies() filename=" + filename);
sb.append("\n");
sb.append("\tthis policy is machine-generated at each Fedora server startup. edit beSecurity.xml to change this policy. \n");
sb.append("\t\n");
sb.append("\t\t\n");
if ("default".equals(key)) {
sb.append("\t\t\t \n");
} else {
sb.append("\t\t\t\n");
sb.append("\t\t\t\t\n");
sb.append("\t\t\t\t\t" + key + " \n");
sb.append("\t\t\t\t \n");
sb.append("\t\t\t \n");
}
sb.append("\t\t \n");
sb.append("\t \n");
String temp = writeRules(callbackBasicAuth, callbackSsl, iplist, key, backendSecuritySpec.listRoleKeys());
sb.append(temp);
sb.append(" \n");
LOG.debug("\ndumping policy\n" + sb + "\n");
File outfile = null;
if (outFilePath == null) {
outfile = File.createTempFile(filename,".xml");
} else {
outfile = new File(outFilePath + File.separator + filename + ".xml");
}
tempfiles.put(filename + ".xml", outfile.getAbsolutePath());
PrintStream pos = new PrintStream(new FileOutputStream(outfile));
pos.println(sb);
pos.close();
}
LOG.debug("finished writing temp files");
return tempfiles;
}
public static void main(String[] args) throws Exception {
BackendPolicies backendPolicies = new BackendPolicies(args[0], args[1]);
backendPolicies.generateBackendPolicies();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy