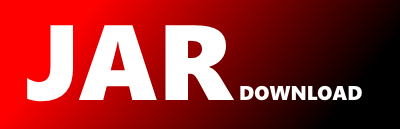
java.fedora.server.storage.ConnectionPoolManagerImpl Maven / Gradle / Ivy
Show all versions of fcrepo-client Show documentation
/*
* -----------------------------------------------------------------------------
*
* License and Copyright: The contents of this file are subject to the
* Apache License, Version 2.0 (the "License"); you may not use
* this file except in compliance with the License. You may obtain a copy of
* the License at
* http://www.fedora-commons.org/licenses.
*
* Software distributed under the License is distributed on an "AS IS" basis,
* WITHOUT WARRANTY OF ANY KIND, either express or implied. See the License for
* the specific language governing rights and limitations under the License.
*
* The entire file consists of original code.
* Copyright © 2008 Fedora Commons, Inc.
*
Copyright © 2002-2007 The Rector and Visitors of the University of
* Virginia and Cornell University
* All rights reserved.
*
* -----------------------------------------------------------------------------
*/
package fedora.server.storage;
import java.sql.SQLException;
import java.util.HashMap;
import java.util.Hashtable;
import java.util.Map;
import java.util.Set;
import org.apache.log4j.Logger;
import fedora.server.errors.ConnectionPoolNotFoundException;
import fedora.server.errors.ModuleInitializationException;
import fedora.server.DatastoreConfig;
import fedora.server.Module;
import fedora.server.Server;
import fedora.server.utilities.DDLConverter;
/**
* Implements ConnectionPoolManager
to facilitate obtaining
* database connection pools.
*
* This class initializes the connection pools specified by parameters in
* the Fedora fedora.fcfg
configuration file. The Fedora server
* must be instantiated in order for this class to function properly.
*
* @author [email protected]
* @version $Id: ConnectionPoolManagerImpl.java 5304 2006-12-05 11:47:52Z cwilper $
*/
public class ConnectionPoolManagerImpl extends Module
implements ConnectionPoolManager
{
/** Logger for this class. */
private static final Logger LOG = Logger.getLogger(
ConnectionPoolManagerImpl.class.getName());
private static Hashtable h_ConnectionPools = new Hashtable();
private static String defaultPoolName = null;
private String jdbcDriverClass = null;
private String dbUsername = null;
private String dbPassword = null;
private String jdbcURL = null;
private int maxActive = 0;
private int maxIdle = 0;
private long maxWait = 0;
private long minEvictableIdleTimeMillis =0;
private int minIdle = 0;
private int numTestsPerEvictionRun = 0;
private long softMinEvictableIdleTimeMillis = 0;
private boolean testOnBorrow = false;
private boolean testOnReturn = false;
private boolean testWhileIdle = false;
private long timeBetweenEvictionRunsMillis = 0;
private byte whenExhaustedAction = 0;
/**
* Constructs a new ConnectionPoolManagerImpl
*
* @param moduleParameters The name/value pair map of module parameters.
* @param server The server instance.
* @param role The module role name.
* @throws ModuleInitializationException If initialization values are
* invalid or initialization fails for some other reason.
*/
public ConnectionPoolManagerImpl(Map moduleParameters,
Server server, String role)
throws ModuleInitializationException
{
super(moduleParameters, server, role);
}
/**
* Initializes the Module based on configuration parameters. The
* implementation of this method is dependent on the schema used to define
* the parameter names for the role of
* fedora.server.storage.ConnectionPoolManager
.
*
* @throws ModuleInitializationException If initialization values are
* invalid or initialization fails for some other reason.
*/
public void initModule() throws ModuleInitializationException
{
try
{
Server s_server = this.getServer();
defaultPoolName = this.getParameter("defaultPoolName");
if (defaultPoolName == null || defaultPoolName.equalsIgnoreCase(""))
{
throw new ModuleInitializationException("Default Connection Pool "
+ "Name Not Specified", getRole());
}
LOG.debug("DefaultPoolName: "+defaultPoolName);
String poolList = this.getParameter("poolNames");
// Pool names should be comma delimited
String[] poolNames = poolList.split(",");
// Initialize each connection pool
for (int i=0; i cProps = new HashMap();
for (String name : (Set) config.getParameters().keySet()) {
if (name.startsWith("connection.")) {
String realName = name.substring(11);
LOG.debug("Connection property " + realName + " = "
+ config.getParameter(name));
cProps.put(realName, config.getParameter(name));
}
}
// If a ddlConverter has been specified for the pool,
// try to instantiate it so the ConnectionPool can use
// it when it provides a TableCreatingConnection.
// If a ddlConverter has been specified, it is assumed
// that a failure to initialize (construct) it should
// trigger a ModuleInitializationException (a fatal startup error).
DDLConverter ddlConverter=null;
String ddlConverterClassName=getServer().
getDatastoreConfig(poolNames[i]).
getParameter("ddlConverter");
if (ddlConverterClassName!=null)
{
try
{
ddlConverter=(DDLConverter)
Class.forName(ddlConverterClassName).newInstance();
} catch (Throwable th) {
throw new ModuleInitializationException("A DDLConverter was "
+ "specified for the pool \"" + poolNames[i]
+ "\", but it couldn't be instantiated.", getRole(), th);
}
}
// Create connection pool
try
{
ConnectionPool connectionPool = new ConnectionPool(jdbcDriverClass,
jdbcURL,
dbUsername,
dbPassword,
ddlConverter,
maxActive,
maxIdle,
maxWait,
minIdle,
minEvictableIdleTimeMillis,
numTestsPerEvictionRun,
timeBetweenEvictionRunsMillis,
testOnBorrow,
testOnReturn,
testWhileIdle,
whenExhaustedAction);
connectionPool.setConnectionProperties(cProps);
LOG.debug("Initialized Pool: "+connectionPool);
h_ConnectionPools.put(poolNames[i],connectionPool);
LOG.debug("putPoolInHash: "+h_ConnectionPools.size());
} catch (SQLException sqle)
{
LOG.error("Unable to initialize connection pool: "
+ poolNames[i] + ": " + sqle.getMessage());
}
}
} catch (Throwable th)
{
th.printStackTrace();
throw new ModuleInitializationException("A connection pool could "
+ "not be instantiated. The underlying error was a "
+ th.getClass().getName() + "The message was \""
+ th.getMessage() + "\".", getRole());
}
}
/**
* Gets a named connection pool.
*
* @param poolName The name of the connection pool.
* @return The named connection pool.
* @throws ConnectionPoolNotFoundException If the specified connection pool
* cannot be found.
*/
public ConnectionPool getPool(String poolName)
throws ConnectionPoolNotFoundException
{
ConnectionPool connectionPool = null;
try
{
if (h_ConnectionPools.containsKey(poolName))
{
connectionPool = (ConnectionPool)h_ConnectionPools.get(poolName);
} else
{
// Error: pool was never initialized or name could not be found
throw new ConnectionPoolNotFoundException("Connection pool "
+ "not found: " + poolName);
}
} catch (Throwable th) {
throw new ConnectionPoolNotFoundException("The specified connection "
+ "pool \"" + poolName + "\" could not be found. The underlying "
+ "error was a " + th.getClass().getName()
+ "The message was \"" + th.getMessage() + "\".");
}
return connectionPool;
}
/**
* Gets the default Connection Pool. This method overrides
* getPool(String poolName)
.
*
* @return The default connection pool.
* @throws ConnectionPoolNotFoundException If the default connection pool
* cannot be found.
*/
public ConnectionPool getPool()
throws ConnectionPoolNotFoundException
{
ConnectionPool connectionPool = null;
try
{
if (h_ConnectionPools.containsKey(defaultPoolName))
{
connectionPool = (ConnectionPool)h_ConnectionPools.get(defaultPoolName);
} else
{
// Error: default pool was never initialized or could not be found
throw new ConnectionPoolNotFoundException("Default connection pool " +
"not found: " + defaultPoolName);
}
} catch (Throwable th) {
throw new ConnectionPoolNotFoundException("The default connection "
+ "pool \"" + defaultPoolName + "\" could not be found. The "
+ "underlying error was a " + th.getClass().getName()
+ "The message was \"" + th.getMessage() + "\".");
}
return connectionPool;
}
}