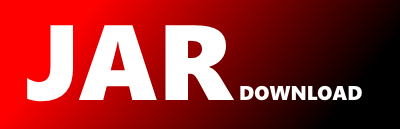
java.fedora.server.storage.DOManager Maven / Gradle / Ivy
/*
* -----------------------------------------------------------------------------
*
* License and Copyright: The contents of this file are subject to the
* Apache License, Version 2.0 (the "License"); you may not use
* this file except in compliance with the License. You may obtain a copy of
* the License at
* http://www.fedora-commons.org/licenses.
*
* Software distributed under the License is distributed on an "AS IS" basis,
* WITHOUT WARRANTY OF ANY KIND, either express or implied. See the License for
* the specific language governing rights and limitations under the License.
*
* The entire file consists of original code.
* Copyright © 2008 Fedora Commons, Inc.
*
Copyright © 2002-2007 The Rector and Visitors of the University of
* Virginia and Cornell University
* All rights reserved.
*
* -----------------------------------------------------------------------------
*/
package fedora.server.storage;
import java.io.InputStream;
import fedora.server.Context;
import fedora.server.errors.ServerException;
import fedora.server.search.FieldSearchQuery;
import fedora.server.search.FieldSearchResult;
/**
*
* Title: DOManager.java
* Description: A RepositoryReader that provides facilities for creating
* and modifying objects within the repository, as well as
* a query facility.
*
* @author [email protected]
* @version $Id: DOManager.java 5016 2006-09-01 20:54:17Z cwilper $
*/
public interface DOManager
extends RepositoryReader {
/**
* Relinquishes control of a DOWriter back to the DOManager.
*
* When a DOManager provides a DOWriter, it creates a session lock.
* This is used to guarantee that there will never be concurrent changes
* to the same object. To release the session lock, a DOWriter user
* calls this method.
*
* @param writer an instance of a digital object writer.
* @throws ServerException if an error occurs in obtaining a writer.
*/
public abstract void releaseWriter(DOWriter writer)
throws ServerException;
/**
* Gets a DOWriter for an existing digital object.
*
* @param context The context of this request.
* @param pid The PID of the object.
* @return A writer, or null if the pid didn't point to an accessible object.
* @throws ServerException If anything went wrong.
*/
public abstract DOWriter getWriter(boolean cachedObjectRequired, Context context, String pid)
throws ServerException;
/**
* Creates a digital object with a newly-allocated pid, and returns
* a DOWriter on it.
*
* @param context The context of this request.
* @return A writer.
* @throws ServerException If anything went wrong.
*/
/*
public abstract DOWriter getIngestWriter(Context context)
throws ServerException;
*/
/**
* Creates a copy of the digital object given by the InputStream,
* with either a new PID or the PID indicated by the InputStream.
*
* @param context The context of this request.
* @param in A serialization of the digital object.
* @param format The format of the serialization.
* @param encoding The character encoding.
* @param newPid Whether a new PID should be generated or the one indicated
* by the InputStream should be used.
* @return a writer.
* @throws ServerException If anything went wrong.
*/
public abstract DOWriter getIngestWriter(boolean cachedObjectRequired, Context context, InputStream in, String format, String encoding, boolean newPid)
throws ServerException;
public FieldSearchResult findObjects(Context context,
String[] resultFields, int maxResults, FieldSearchQuery query)
throws ServerException;
public FieldSearchResult resumeFindObjects(Context context,
String sessionToken)
throws ServerException;
public String[] getNextPID(int numPIDs, String namespace) throws ServerException;
/**
* Reserve a series of PIDs so that they are never used for
* subsequent PID generations.
*/
public void reservePIDs(String[] pidList) throws ServerException;
/**
* Get a "hash" of the repository.
*
* This value can be compared to a previous value to determine whether
* the content of the repository has changed. It is not necessary for
* this value to precisely reflect the state of the repository, but
* if the repository hasn't changed, subsequent calls should return the
* same value.
*/
public String getRepositoryHash() throws ServerException;
}