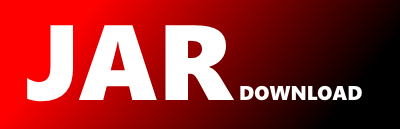
java.fedora.server.storage.SimpleDOWriter Maven / Gradle / Ivy
Show all versions of fcrepo-client Show documentation
/* * ----------------------------------------------------------------------------- * *
, this is taken to be the smallest possible * value. * @param end The end date (inclusive) of versions to remove. If *License and Copyright: The contents of this file are subject to the * Apache License, Version 2.0 (the "License"); you may not use * this file except in compliance with the License. You may obtain a copy of * the License at * http://www.fedora-commons.org/licenses.
* *Software distributed under the License is distributed on an "AS IS" basis, * WITHOUT WARRANTY OF ANY KIND, either express or implied. See the License for * the specific language governing rights and limitations under the License.
* *The entire file consists of original code.
*Copyright © 2008 Fedora Commons, Inc.
*Copyright © 2002-2007 The Rector and Visitors of the University of * Virginia and Cornell University
* * ----------------------------------------------------------------------------- */ package fedora.server.storage; import java.util.ArrayList; import java.util.Date; import java.util.Iterator; import java.util.List; import fedora.server.Context; import fedora.server.Server; import fedora.server.errors.ServerException; import fedora.server.errors.ObjectIntegrityException; import fedora.server.storage.DefaultDOManager; import fedora.server.storage.translation.DOTranslator; import fedora.server.storage.types.AuditRecord; import fedora.server.storage.types.Datastream; import fedora.server.storage.types.DigitalObject; import fedora.server.storage.types.Disseminator; /** * A DigitalObject-backed DOWriter. * *
* All rights reserved.This interface supports transaction behavior with the commit(String) and * rollBack() methods. When a DOWriter is instantiated, there is an implicit * transaction. Write methods may be called, but they won't affect the * the underlying data store until commit(String) is invoked. This also has * the effect of creating another implicit transaction. If temporary * changes are no longer wanted, rollBack() may be called to return the object * to it's original form. rollBack() is only valid for the current transaction.
* *The read methods of DOWriter reflect on the composition of the object in * the context of the current transaction.
* * @author [email protected] * @version $Id: SimpleDOWriter.java 5351 2006-12-07 13:04:02Z rlw $ */ public class SimpleDOWriter extends SimpleDOReader implements DOWriter { private static ObjectIntegrityException ERROR_PENDING_REMOVAL = new ObjectIntegrityException("That can't be done because you said " + "I should remove the object and i assume that's what you " + "want unless you call rollback()"); private static ObjectIntegrityException ERROR_INVALIDATED = new ObjectIntegrityException("The handle is no longer valid " + "... this object has already been committed or explicitly" + " invalidated."); private DigitalObject m_obj; private Context m_context; private DefaultDOManager m_mgr; private boolean m_pendingRemoval=false; private boolean m_invalidated=false; private boolean m_committed=false; public SimpleDOWriter(Context context, DefaultDOManager mgr, DOTranslator translator, String exportFormat, String encoding, DigitalObject obj) { super(context, mgr, translator, exportFormat, encoding, obj); m_context=context; m_obj=obj; m_mgr=mgr; } public void setState(String state) throws ObjectIntegrityException { assertNotInvalidated(); assertNotPendingRemoval(); m_obj.setState(state); } public void setOwnerId(String ownerId) throws ObjectIntegrityException { assertNotInvalidated(); assertNotPendingRemoval(); m_obj.setOwnerId(ownerId); } public void setDatastreamState(String datastreamID, String dsState) throws ServerException { assertNotInvalidated(); assertNotPendingRemoval(); List allVersions = m_obj.datastreams(datastreamID); Iterator dsIter = allVersions.iterator(); // Set all versions of this datastreamID to the specified state while (dsIter.hasNext()) { Datastream ds = (Datastream) dsIter.next(); ds.DSState=dsState; } } public void setDatastreamVersionable(String datastreamID, boolean versionable) throws ServerException { assertNotInvalidated(); assertNotPendingRemoval(); List allVersions = m_obj.datastreams(datastreamID); Iterator dsIter = allVersions.iterator(); // Set all versions of this datastreamID to the specified versionable status while (dsIter.hasNext()) { Datastream ds = (Datastream) dsIter.next(); ds.DSVersionable = versionable; } } public void setDisseminatorState(String disseminatorID, String dissState) throws ServerException { assertNotInvalidated(); assertNotPendingRemoval(); List allVersions = m_obj.disseminators(disseminatorID); Iterator dissIter = allVersions.iterator(); // Set all versions of this disseminatorID to the specified state while (dissIter.hasNext()) { Disseminator diss = (Disseminator) dissIter.next(); diss.dissState=dissState; } } public void setLabel(String label) throws ObjectIntegrityException { assertNotInvalidated(); assertNotPendingRemoval(); m_obj.setLabel(label); } /** * Removes the entire digital object. * * @throws ServerException If any type of error occurred fulfilling the * request. */ public void remove() throws ObjectIntegrityException { assertNotInvalidated(); assertNotPendingRemoval(); m_pendingRemoval=true; } /** * Adds a datastream to the object. * * @param datastream The datastream. * @throws ServerException If any type of error occurred fulfilling the * request. */ public void addDatastream(Datastream datastream, boolean addNewVersion) throws ServerException { assertNotInvalidated(); assertNotPendingRemoval(); // use this call to handle versionable m_obj.addDatastreamVersion(datastream, addNewVersion); } /** * Adds a disseminator to the object. * * @param disseminator The disseminator. * @throws ServerException If any type of error occurred fulfilling the * request. */ public void addDisseminator(Disseminator disseminator) throws ServerException { assertNotInvalidated(); assertNotPendingRemoval(); m_obj.disseminators(disseminator.dissID).add(disseminator); } /** * Removes a datastream from the object. * * @param id The id of the datastream. * @param start The start date (inclusive) of versions to remove. If *null
, this is taken to be the smallest possible * value. * @param end The end date (inclusive) of versions to remove. If *null
, this is taken to be the greatest possible * value. * @throws ServerException If any type of error occurred fulfilling the * request. */ public Date[] removeDatastream(String id, Date start, Date end) throws ServerException { assertNotInvalidated(); assertNotPendingRemoval(); List versions=m_obj.datastreams(id); ArrayList removeList=new ArrayList(); for (int i=0; i=0) && (ds.DSCreateDT.compareTo(end)<=0) ) { doRemove=true; } } else { if (ds.DSCreateDT.compareTo(start)>=0) { doRemove=true; } } } else { if (end!=null) { if (ds.DSCreateDT.compareTo(end)<=0) { doRemove=true; } } else { doRemove=true; } } if (doRemove) { // Note: We don't remove old audit records by design. // add this datastream to the datastream to-be-removed list. removeList.add(ds); } } versions.removeAll(removeList); // finally, return the dates of each deleted item Date[] deletedDates=new Date[removeList.size()]; for (int i=0; i null null
, this is taken to be the greatest possible * value. * @throws ServerException If any type of error occurred fulfilling the * request. */ public Date[] removeDisseminator(String id, Date start, Date end) throws ServerException { assertNotInvalidated(); assertNotPendingRemoval(); List versions=m_obj.disseminators(id); ArrayList removeList=new ArrayList(); for (int i=0; i=0) && (diss.dissCreateDT.compareTo(end)<=0) ) { doRemove=true; } } else { if (diss.dissCreateDT.compareTo(start)>=0) { doRemove=true; } } } else { if (end!=null) { if (diss.dissCreateDT.compareTo(end)<=0) { doRemove=true; } } else { doRemove=true; } } if (doRemove) { // Note: We don't remove old audit records by design. // add this disseminator to the disseminator to-be-removed list. removeList.add(diss); } } versions.removeAll(removeList); // finally, return the dates of each deleted item Date[] deletedDates=new Date[removeList.size()]; for (int i=0; i