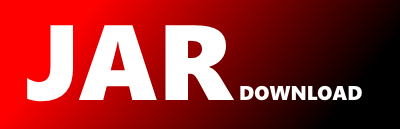
java.fedora.server.storage.replication.DbmsConnection Maven / Gradle / Ivy
/*
* -----------------------------------------------------------------------------
*
* License and Copyright: The contents of this file are subject to the
* Apache License, Version 2.0 (the "License"); you may not use
* this file except in compliance with the License. You may obtain a copy of
* the License at
* http://www.fedora-commons.org/licenses.
*
* Software distributed under the License is distributed on an "AS IS" basis,
* WITHOUT WARRANTY OF ANY KIND, either express or implied. See the License for
* the specific language governing rights and limitations under the License.
*
* The entire file consists of original code.
* Copyright © 2008 Fedora Commons, Inc.
*
Copyright © 2002-2007 The Rector and Visitors of the University of
* Virginia and Cornell University
* All rights reserved.
*
* -----------------------------------------------------------------------------
*/
package fedora.server.storage.replication;
import java.sql.*;
import java.io.*;
import org.apache.log4j.Logger;
import fedora.common.Constants;
import fedora.server.storage.*;
import fedora.server.Server;
import fedora.server.errors.ConnectionPoolNotFoundException;
import fedora.server.errors.InitializationException;
/**
* @author Paul Charlton
* @version $Id: DbmsConnection.java 5313 2006-12-06 12:14:27Z cwilper $
*/
public class DbmsConnection {
/** Logger for this class. */
private static final Logger LOG = Logger.getLogger(
DbmsConnection.class.getName());
private static ConnectionPool connectionPool = null;
private static final String dbPropsFile = "db.properties";
private static boolean debug = true;
public DbmsConnection() throws Exception {
initDB();
LOG.debug("DbmsConnection constructor: using connectionPool: " + connectionPool);
}
public Connection getConnection() throws Exception {
Connection connection = null;
connection = connectionPool.getConnection();
return connection;
}
public void freeConnection(Connection connection) {
if (connection!=null) connectionPool.free(connection);
}
/**
* Initializes the relational database connection.
*
* @throws Exception if unable to establish database connection
*/
public static void initDB() throws Exception
{
try
{
ConnectionPoolManager cpmgr=(ConnectionPoolManager) s_server.getModule(
"fedora.server.storage.ConnectionPoolManager");
if (cpmgr==null) {
throw new SQLException( "Server module not loaded: "
+ "fedora.server.storage.ConnectionPoolManager");
} else {
try {
connectionPool=cpmgr.getPool();
} catch (ConnectionPoolNotFoundException cpnfe) {
throw new SQLException("Can't get default pool from cpmgr.");
}
}
} catch (SQLException sqle)
{
// Problem with connection pool and/or database
LOG.error("Unable to create connection pool", sqle);
ConnectionPool connectionPool = null;
connectionPool = null;
// FIXME!! - Decide on Exception handling
Exception e = new Exception("SQLException in DbmsConnection: " + sqle.getMessage());
e.initCause(sqle);
throw e;
}
}
private static Server s_server;
static
{
try
{
s_server=Server.getInstance(new File(Constants.FEDORA_HOME),
false);
} catch (InitializationException ie)
{
LOG.error("Error getting server instance", ie);
}
}
/**
*
* Used for unit testing and demonstration purposes.
*
* @param args program arguments
*
* @exception Exception exceptions that are thrown from called methods
*/
public static void main(String[] args) throws Exception {
Connection connection;
DbmsConnection db = new DbmsConnection();
connection = db.getConnection();
db.freeConnection(connection);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy