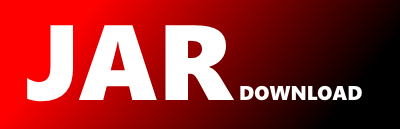
java.fedora.server.storage.service.DSInputSpecParser Maven / Gradle / Ivy
Show all versions of fcrepo-client Show documentation
/*
* -----------------------------------------------------------------------------
*
* License and Copyright: The contents of this file are subject to the
* Apache License, Version 2.0 (the "License"); you may not use
* this file except in compliance with the License. You may obtain a copy of
* the License at
* http://www.fedora-commons.org/licenses.
*
* Software distributed under the License is distributed on an "AS IS" basis,
* WITHOUT WARRANTY OF ANY KIND, either express or implied. See the License for
* the specific language governing rights and limitations under the License.
*
* The entire file consists of original code.
* Copyright © 2008 Fedora Commons, Inc.
*
Copyright © 2002-2007 The Rector and Visitors of the University of
* Virginia and Cornell University
* All rights reserved.
*
* -----------------------------------------------------------------------------
*/
package fedora.server.storage.service;
import fedora.server.errors.RepositoryConfigurationException;
import fedora.server.errors.ObjectIntegrityException;
import fedora.server.storage.types.BMechDSBindSpec;
import fedora.server.storage.types.BMechDSBindRule;
import java.io.InputStream;
import java.util.Vector;
import java.util.StringTokenizer;
import java.util.HashMap;
import javax.xml.parsers.SAXParser;
import javax.xml.parsers.SAXParserFactory;
import org.xml.sax.*;
import org.xml.sax.helpers.DefaultHandler;
/**
*
* Title: DSInputSpecParser.java
* Description: A class for parsing the special XML format in Fedora
* for a Datastream Input Specification (DSInputSpec). A DSInputSpec exists
* within a Behavior Mechanism Object (bmech), and is used to define the
* "data contract" between the service represented by the bmech and a data
* object.
*
* @author [email protected]
* @version $Id: DSInputSpecParser.java 5210 2006-11-16 05:55:44Z eddie $
*/
public class DSInputSpecParser extends DefaultHandler
{
/** The namespaces we know we will encounter */
private final static String FBS = "http://fedora.comm.nsdlib.org/service/bindspec";
/**
* URI-to-namespace prefix mapping info from SAX2 startPrefixMapping events.
*/
private HashMap nsPrefixMap;
// Variables for keeping state during SAX parse.
private boolean inDSInputSpec = false;
private boolean inDSInput = false;
private boolean inDSInputLabel = false;
private boolean inDSInputInstructions = false;
private boolean inDSInputMIME = false;
// Fedora Datastream Binding Spec objects
private BMechDSBindSpec dsInputSpec;
private BMechDSBindRule dsInputRule;
private String BMechPID;
// Working variables...
private Vector tmp_InputRules;
/**
* Constructor to enable another class to initiate the parsing
*/
public DSInputSpecParser(String parentPID)
{
BMechPID = parentPID;
}
/**
* Constructor allows this class to initiate the parsing
*/
public DSInputSpecParser(String parentPID, InputStream in)
throws RepositoryConfigurationException, ObjectIntegrityException
{
BMechPID = parentPID;
XMLReader xmlReader = null;
try
{
SAXParserFactory saxfactory=SAXParserFactory.newInstance();
saxfactory.setValidating(false);
SAXParser parser=saxfactory.newSAXParser();
xmlReader=parser.getXMLReader();
xmlReader.setContentHandler(this);
xmlReader.setFeature("http://xml.org/sax/features/namespaces", false);
xmlReader.setFeature("http://xml.org/sax/features/namespace-prefixes", false);
}
catch (Exception e)
{
throw new RepositoryConfigurationException("Internal SAX error while "
+ "preparing for DSInputSpec datastream deserialization: "
+ e.getMessage());
}
try
{
xmlReader.parse(new InputSource(in));
}
catch (Exception e)
{
throw new ObjectIntegrityException("Error parsing DSInputSpec datastream" +
e.getClass().getName() + ": " + e.getMessage());
}
}
public BMechDSBindSpec getServiceDSInputSpec() {
return dsInputSpec;
}
public void startDocument() throws SAXException
{
nsPrefixMap = new HashMap();
tmp_InputRules = new Vector();
dsInputSpec = new BMechDSBindSpec();
}
public void endDocument() throws SAXException
{
dsInputSpec.dsBindRules = (BMechDSBindRule[]) tmp_InputRules.toArray(new BMechDSBindRule[0]);
tmp_InputRules = null;
nsPrefixMap = null;
}
public void startPrefixMapping(String prefix, String uri) throws SAXException
{
nsPrefixMap.put(uri, prefix);
}
public void skippedEntity(String name) throws SAXException
{
StringBuffer sb = new StringBuffer();
sb.append('&');
sb.append(name);
sb.append(';');
char[] text = new char[sb.length()];
sb.getChars(0, sb.length(), text, 0);
this.characters(text, 0, text.length);
}
public void characters(char ch[], int start, int length) throws SAXException
{
if (inDSInputLabel)
{
dsInputRule.bindingLabel = new String(ch, start, length);
}
else if (inDSInputInstructions)
{
dsInputRule.bindingInstruction = new String(ch, start, length);
}
else if (inDSInputMIME)
{
StringTokenizer st = new StringTokenizer(new String(ch, start, length), " ");
String[] MIMETypes = new String[st.countTokens()];
for (int i = 0; i