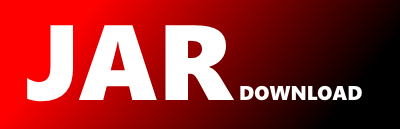
java.fedora.server.storage.translation.DOTranslatorImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fcrepo-client Show documentation
Show all versions of fcrepo-client Show documentation
The Fedora Client is a Java Library that allows API access to a Fedora Repository. The client is typically one part of a full Fedora installation.
The newest version!
/*
* -----------------------------------------------------------------------------
*
* License and Copyright: The contents of this file are subject to the
* Apache License, Version 2.0 (the "License"); you may not use
* this file except in compliance with the License. You may obtain a copy of
* the License at
* http://www.fedora-commons.org/licenses.
*
* Software distributed under the License is distributed on an "AS IS" basis,
* WITHOUT WARRANTY OF ANY KIND, either express or implied. See the License for
* the specific language governing rights and limitations under the License.
*
* The entire file consists of original code.
* Copyright © 2008 Fedora Commons, Inc.
*
Copyright © 2002-2007 The Rector and Visitors of the University of
* Virginia and Cornell University
* All rights reserved.
*
* -----------------------------------------------------------------------------
*/
package fedora.server.storage.translation;
import java.io.InputStream;
import java.io.OutputStream;
import java.io.UnsupportedEncodingException;
import java.util.Map;
import org.apache.log4j.Logger;
import fedora.server.errors.ObjectIntegrityException;
import fedora.server.errors.ServerException;
import fedora.server.errors.StreamIOException;
import fedora.server.errors.UnsupportedTranslationException;
import fedora.server.storage.types.DigitalObject;
/**
* DOTranslation implementation.
*
* @author [email protected]
* @version $Id: DOTranslatorImpl.java 5220 2006-11-20 13:52:20Z cwilper $
*/
public class DOTranslatorImpl
implements DOTranslator {
/** Logger for this class. */
private static final Logger LOG = Logger.getLogger(
DOTranslatorImpl.class.getName());
private Map m_serializers;
private Map m_deserializers;
public DOTranslatorImpl(Map serializers, Map deserializers) {
m_serializers=serializers;
m_deserializers=deserializers;
}
public void deserialize(InputStream in, DigitalObject out,
String format, String encoding, int transContext)
throws ObjectIntegrityException, StreamIOException,
UnsupportedTranslationException, ServerException {
try {
LOG.debug("Grabbing deserializer for: " + format);
DODeserializer des=(DODeserializer) m_deserializers.get(format);
if (des==null) {
throw new UnsupportedTranslationException("No deserializer exists "
+ "for format: " + format);
}
DODeserializer newDes=des.getInstance();
newDes.deserialize(in, out, encoding, transContext);
} catch (UnsupportedEncodingException uee) {
throw new UnsupportedTranslationException("Deserializer for format: "
+ format + " does not support encoding: " + encoding);
}
}
public void serialize(DigitalObject in, OutputStream out,
String format, String encoding, int transContext)
throws ObjectIntegrityException, StreamIOException,
UnsupportedTranslationException, ServerException {
try {
LOG.debug("Grabbing serializer for: " + format);
DOSerializer ser=(DOSerializer) m_serializers.get(format);
if (ser==null) {
throw new UnsupportedTranslationException(
"No serializer exists for format: " + format);
}
DOSerializer newSer=ser.getInstance();
newSer.serialize(in, out, encoding, transContext);
} catch (UnsupportedEncodingException uee) {
throw new UnsupportedTranslationException("Serializer for format: "
+ format + " does not support encoding: " + encoding);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy