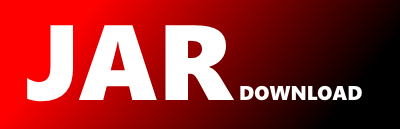
java.fedora.server.validation.DOValidatorXMLSchema Maven / Gradle / Ivy
/*
* -----------------------------------------------------------------------------
*
* License and Copyright: The contents of this file are subject to the
* Apache License, Version 2.0 (the "License"); you may not use
* this file except in compliance with the License. You may obtain a copy of
* the License at
* http://www.fedora-commons.org/licenses.
*
* Software distributed under the License is distributed on an "AS IS" basis,
* WITHOUT WARRANTY OF ANY KIND, either express or implied. See the License for
* the specific language governing rights and limitations under the License.
*
* The entire file consists of original code.
* Copyright © 2008 Fedora Commons, Inc.
*
Copyright © 2002-2007 The Rector and Visitors of the University of
* Virginia and Cornell University
* All rights reserved.
*
* -----------------------------------------------------------------------------
*/
package fedora.server.validation;
// JAXP imports
import javax.xml.parsers.*;
import org.xml.sax.*;
import java.io.File;
import java.io.InputStream;
import java.io.FileInputStream;
import java.io.IOException;
import java.net.URI;
import org.apache.log4j.Logger;
import fedora.server.errors.ObjectValidityException;
import fedora.server.errors.GeneralException;
/**
* XML Schema validation for Digital Objects.
*
* @author [email protected]
* @version $Id: DOValidatorXMLSchema.java 5218 2006-11-20 05:10:11Z cwilper $
*/
public class DOValidatorXMLSchema implements EntityResolver
{
/** Logger for this class. */
private static final Logger LOG = Logger.getLogger(
DOValidatorXMLSchema.class.getName());
/** Constants used for JAXP 1.2 */
private static final String JAXP_SCHEMA_LANGUAGE =
"http://java.sun.com/xml/jaxp/properties/schemaLanguage";
private static final String W3C_XML_SCHEMA =
"http://www.w3.org/2001/XMLSchema";
private static final String JAXP_SCHEMA_SOURCE =
"http://java.sun.com/xml/jaxp/properties/schemaSource";
private URI schemaURI = null;
public DOValidatorXMLSchema(String schemaPath) throws GeneralException
{
try
{
schemaURI = (new File(schemaPath)).toURI();
}
catch (Exception e)
{
LOG.error("Error constructing validator", e);
throw new GeneralException(e.getMessage());
}
}
public void validate(File objectAsFile)
throws ObjectValidityException, GeneralException
{
try
{
validate(new InputSource(new FileInputStream(objectAsFile)));
}
catch (IOException e)
{
String msg = "DOValidatorXMLSchema returned error.\n"
+ "The underlying exception was a " + e.getClass().getName() + ".\n"
+ "The message was " + "\"" + e.getMessage() + "\"";
throw new GeneralException(msg);
}
}
public void validate(InputStream objectAsStream)
throws ObjectValidityException, GeneralException
{
validate(new InputSource(objectAsStream));
}
private void validate(InputSource objectAsSource)
throws ObjectValidityException, GeneralException
{
InputSource doXML = objectAsSource;
try
{
// XMLSchema validation via SAX parser
SAXParserFactory spf = SAXParserFactory.newInstance();
spf.setNamespaceAware(true);
spf.setValidating(true);
SAXParser sp = spf.newSAXParser();
sp.setProperty(JAXP_SCHEMA_LANGUAGE, W3C_XML_SCHEMA);
// JAXP property for schema location
sp.setProperty(
"http://java.sun.com/xml/jaxp/properties/schemaSource",
schemaURI.toString());
XMLReader xmlreader = sp.getXMLReader();
xmlreader.setErrorHandler(new DOValidatorXMLErrorHandler());
xmlreader.setEntityResolver(this);
xmlreader.parse(doXML);
}
catch (ParserConfigurationException e)
{
String msg = "DOValidatorXMLSchema returned parser error.\n"
+ "The underlying exception was a " + e.getClass().getName() + ".\n"
+ "The message was " + "\"" + e.getMessage() + "\"";
throw new GeneralException(msg, e);
}
catch (SAXException e)
{
String msg = "DOValidatorXMLSchema returned validation exception.\n"
+ "The underlying exception was a " + e.getClass().getName() + ".\n"
+ "The message was " + "\"" + e.getMessage() + "\"";
throw new ObjectValidityException(msg, e);
}
catch (Exception e)
{
String msg = "DOValidatorXMLSchema returned error.\n"
+ "The underlying error was a " + e.getClass().getName() + ".\n"
+ "The message was " + "\"" + e.getMessage() + "\"";
throw new GeneralException(msg, e);
}
}
/**
* Resolve the entity if it's referring to a local schema.
* Otherwise, return an empty InputSource.
*
* This behavior is required in order to ensure that Xerces never
* attempts to load external schemas specified with xsi:schemaLocation.
* It is not enough that we specify processContents="skip" in our own
* schema.
*/
public InputSource resolveEntity(String publicId, String systemId) {
if (systemId != null && systemId.startsWith("file:")) {
return null;
} else {
return new InputSource();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy