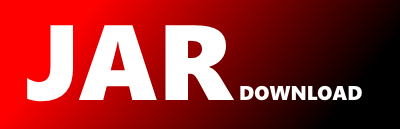
java.fedora.swing.jtable.JSortTable Maven / Gradle / Ivy
Show all versions of fcrepo-client Show documentation
/*
* -----------------------------------------------------------------------------
*
* License and Copyright: The contents of this file are subject to the
* Apache License, Version 2.0 (the "License"); you may not use
* this file except in compliance with the License. You may obtain a copy of
* the License at
* http://www.fedora-commons.org/licenses.
*
* Software distributed under the License is distributed on an "AS IS" basis,
* WITHOUT WARRANTY OF ANY KIND, either express or implied. See the License for
* the specific language governing rights and limitations under the License.
*
* The entire file consists of original code.
* Copyright © 2008 Fedora Commons, Inc.
*
Copyright © 2002-2007 The Rector and Visitors of the University of
* Virginia and Cornell University
* All rights reserved.
*
* -----------------------------------------------------------------------------
*/
package fedora.swing.jtable;
import java.awt.event.*;
import java.util.*;
import javax.swing.*;
import javax.swing.table.*;
/**
*
* Title: JSortTable.java
*
*
* Description:
*
*
* -----------------------------------------------------------------------------
*
* Portions created by Claude Duguay are Copyright © Claude Duguay,
* originally made available at
* http://www.fawcette.com/javapro/2002_08/magazine/columns/visualcomponents/
*
*
* -----------------------------------------------------------------------------
*
* @author Claude Duguay, [email protected]
* @version $Id: JSortTable.java 5162 2006-10-25 00:49:06Z eddie $
*/
public class JSortTable extends JTable implements MouseListener {
private static final long serialVersionUID = 1L;
protected int sortedColumnIndex = -1;
protected boolean sortedColumnAscending = true;
public JSortTable() {
this(new DefaultSortTableModel());
}
public JSortTable(int rows, int cols) {
this(new DefaultSortTableModel(rows, cols));
}
public JSortTable(Object[][] data, Object[] names) {
this(new DefaultSortTableModel(data, names));
}
public JSortTable(Vector data, Vector names) {
this(new DefaultSortTableModel(data, names));
}
public JSortTable(SortTableModel model) {
super(model);
initSortHeader();
}
public JSortTable(SortTableModel model, TableColumnModel colModel) {
super(model, colModel);
initSortHeader();
}
public JSortTable(SortTableModel model, TableColumnModel colModel,
ListSelectionModel selModel) {
super(model, colModel, selModel);
initSortHeader();
}
protected void initSortHeader() {
JTableHeader header = getTableHeader();
header.setDefaultRenderer(new SortHeaderRenderer());
header.addMouseListener(this);
}
public int getSortedColumnIndex() {
return sortedColumnIndex;
}
public boolean isSortedColumnAscending() {
return sortedColumnAscending;
}
public void mouseReleased(MouseEvent event) {
TableColumnModel colModel = getColumnModel();
int index = colModel.getColumnIndexAtX(event.getX());
int modelIndex = colModel.getColumn(index).getModelIndex();
SortTableModel model = (SortTableModel) getModel();
if (model.isSortable(modelIndex)) {
// toggle ascension, if already sorted
if (sortedColumnIndex == index) {
sortedColumnAscending = !sortedColumnAscending;
}
sortedColumnIndex = index;
model.sortColumn(modelIndex, sortedColumnAscending);
}
}
public void mousePressed(MouseEvent event) {
}
public void mouseClicked(MouseEvent event) {
}
public void mouseEntered(MouseEvent event) {
}
public void mouseExited(MouseEvent event) {
}
}