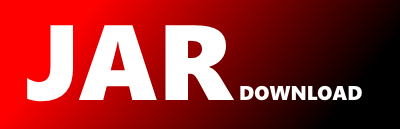
java.fedora.swing.mdi.MDIDesktopPane Maven / Gradle / Ivy
Show all versions of fcrepo-client Show documentation
/*
* -----------------------------------------------------------------------------
*
* License and Copyright: The contents of this file are subject to the
* Apache License, Version 2.0 (the "License"); you may not use
* this file except in compliance with the License. You may obtain a copy of
* the License at
* http://www.fedora-commons.org/licenses.
*
* Software distributed under the License is distributed on an "AS IS" basis,
* WITHOUT WARRANTY OF ANY KIND, either express or implied. See the License for
* the specific language governing rights and limitations under the License.
*
* The entire file consists of original code.
* Copyright © 2008 Fedora Commons, Inc.
*
Copyright © 2002-2007 The Rector and Visitors of the University of
* Virginia and Cornell University
* All rights reserved.
*
* -----------------------------------------------------------------------------
*/
package fedora.swing.mdi;
import javax.swing.*;
import java.awt.*;
import java.beans.*;
/**
* Title: SortHeaderRenderer.java
* Description:
* An extension of WDesktopPane that supports often used MDI functionality. This
* class also handles setting scroll bars for when windows move too far to the left or
* bottom, providing the MDIDesktopPane is in a ScrollPane.
*
*
* -----------------------------------------------------------------------------
*
* Portions created by Gerald Nunn are Copyright ©
* Gerald Nunn, originally made available at
* http://www.javaworld.com/javaworld/jw-05-2001/jw-0525-mdi.html
*
* -----------------------------------------------------------------------------
*
* @author Gerald Nunn, [email protected]
* @version $Id: MDIDesktopPane.java 5162 2006-10-25 00:49:06Z eddie $
*/
public class MDIDesktopPane extends JDesktopPane {
private static final long serialVersionUID = 1L;
private static int FRAME_OFFSET=20;
private MDIDesktopManager manager;
public MDIDesktopPane() {
manager=new MDIDesktopManager(this);
setDesktopManager(manager);
// setDragMode(JDesktopPane.OUTLINE_DRAG_MODE);
setDragMode(JDesktopPane.LIVE_DRAG_MODE);
}
public void setBounds(int x, int y, int w, int h) {
super.setBounds(x,y,w,h);
checkDesktopSize();
}
public Component add(JInternalFrame frame) {
JInternalFrame[] array = getAllFrames();
Point p;
int w;
int h;
Component retval=super.add(frame);
checkDesktopSize();
if (array.length > 0) {
p = array[0].getLocation();
p.x = p.x + FRAME_OFFSET;
p.y = p.y + FRAME_OFFSET;
}
else {
p = new Point(0, 0);
}
frame.setLocation(p.x, p.y);
moveToFront(frame);
frame.setVisible(true);
try {
frame.setSelected(true);
} catch (PropertyVetoException e) {
frame.toBack();
}
return retval;
}
public void remove(Component c) {
super.remove(c);
checkDesktopSize();
}
/**
* Cascade all internal frames, un-iconfying any minimized first
*/
public void cascadeFrames() {
restoreFrames();
int x = 0;
int y = 0;
JInternalFrame allFrames[] = getAllFrames();
manager.setNormalSize();
int frameHeight = (getBounds().height - 5) - allFrames.length * FRAME_OFFSET;
int frameWidth = (getBounds().width - 5) - allFrames.length * FRAME_OFFSET;
for (int i = allFrames.length - 1; i >= 0; i--) {
allFrames[i].setSize(frameWidth,frameHeight);
allFrames[i].setLocation(x,y);
x = x + FRAME_OFFSET;
y = y + FRAME_OFFSET;
}
}
/**
* Tile all internal frames, un-iconifying any minimized first
*/
public void tileFrames() {
restoreFrames();
java.awt.Component allFrames[] = getAllFrames();
manager.setNormalSize();
int frameHeight = getBounds().height/allFrames.length;
int y = 0;
for (int i = 0; i < allFrames.length; i++) {
allFrames[i].setSize(getBounds().width,frameHeight);
allFrames[i].setLocation(0,y);
y = y + frameHeight;
}
}
public void minimizeFrames() {
JInternalFrame[] array = getAllFrames();
for (int i=0; ix) {
x = allFrames[i].getX() + allFrames[i].getWidth();
}
if (allFrames[i].getY()+allFrames[i].getHeight()>y) {
y = allFrames[i].getY() + allFrames[i].getHeight();
}
}
Dimension d=scrollPane.getVisibleRect().getSize();
if (scrollPane.getBorder() != null) {
d.setSize(d.getWidth() - scrollInsets.left - scrollInsets.right,
d.getHeight() - scrollInsets.top - scrollInsets.bottom);
}
if (x <= d.getWidth()) x = ((int)d.getWidth()) - 20;
if (y <= d.getHeight()) y = ((int)d.getHeight()) - 20;
desktop.setAllSize(x,y);
scrollPane.invalidate();
scrollPane.validate();
}
}
}