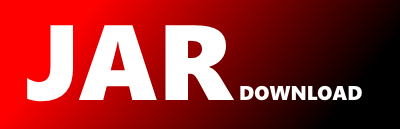
java.fedora.utilities.BuildAxisStubWrapper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fcrepo-client Show documentation
Show all versions of fcrepo-client Show documentation
The Fedora Client is a Java Library that allows API access to a Fedora Repository. The client is typically one part of a full Fedora installation.
The newest version!
/*
* -----------------------------------------------------------------------------
*
* License and Copyright: The contents of this file are subject to the
* Apache License, Version 2.0 (the "License"); you may not use
* this file except in compliance with the License. You may obtain a copy of
* the License at
* http://www.fedora-commons.org/licenses.
*
* Software distributed under the License is distributed on an "AS IS" basis,
* WITHOUT WARRANTY OF ANY KIND, either express or implied. See the License for
* the specific language governing rights and limitations under the License.
*
* The entire file consists of original code.
* Copyright © 2008 Fedora Commons, Inc.
*
Copyright © 2002-2007 The Rector and Visitors of the University of
* Virginia and Cornell University
* All rights reserved.
*
* -----------------------------------------------------------------------------
*/
package fedora.utilities;
import java.io.*;
/**
* Builds a wrapper around a generated Axis client stub, which includes
* imports for the class and code that should run before and after each
* method invocation.
*
* This is a cheap way of writing specialized API-A and API-M client classes
* that need to do something extra (the same thing) for each method call.
* Like, for instance, run it in a SwingWorker thread because it's being called
* from a GUI. This is cleaner than writing the SwingWorker code for each
* piece in the code where API-A or API-M methods need to be called.
*
* @author [email protected]
* @version $Id: BuildAxisStubWrapper.java 3966 2005-04-21 13:33:01Z rlw $
*/
public class BuildAxisStubWrapper {
private BufferedWriter m_writer;
private static String N=System.getProperty("line.separator");
public BuildAxisStubWrapper(File stubFile, File templateFile,
String wrapperPackage, String wrapperClass,
File wrapperFile)
throws Exception {
System.out.println("Dynamically generating sourcecode for " + wrapperPackage + "." + wrapperClass);
// first read the template, splitting by ##SPLITTER##, into 3 buffers
StringBuffer importBuf=new StringBuffer();
StringBuffer tsBuf=new StringBuffer();
StringBuffer tfBuf=new StringBuffer();
BufferedReader reader=new BufferedReader(
new InputStreamReader(new FileInputStream(templateFile)));
boolean sawSplitter=false;
String line="first";
int i;
boolean sawImports=false;
while (line!=null) {
if (sawImports && line.equals("##SPLITTER##")) {
sawSplitter=true;
} else {
if (!line.equals("first")) {
if (!sawImports) {
int x=0;
while (!line.equals("##SPLITTER##")) {
importBuf.append(line + N);
line=reader.readLine();
x++;
if (x==2000) {
throw new IOException("Template file must contain two ##SPLITTER## lines.");
}
}
sawImports=true;
} else {
if (sawSplitter) {
tfBuf.append(" " + line + N);
} else {
tsBuf.append(" " + line + N);
}
}
}
}
line=reader.readLine();
}
reader.close();
String methodStart=tsBuf.toString();
String methodFinish=tfBuf.toString();
if (!sawSplitter) throw new IOException(
"Bad template... does not contain ##SPLITTER## line.");
// then start writing the output..
m_writer=new BufferedWriter(
new FileWriter(wrapperFile));
println("package " + wrapperPackage + ";" + N + N + "import java.util.HashMap; // needed by generated code" + N);
println(importBuf.toString());
println("public class " + wrapperClass);
// do the rest as we scan the stub file
reader=new BufferedReader(
new InputStreamReader(new FileInputStream(stubFile)));
line="";
while (line!=null) {
i=line.indexOf("implements");
if (i!=-1) {
String endPart=line.substring(i+11);
String interfaceClassName=endPart.substring(0, endPart.indexOf(" "));
println(" implements " + interfaceClassName + " {");
println("");
println(" /** The wrapped instance */");
println(" private " + interfaceClassName + " m_instance;");
println("");
println(" public " + wrapperClass + "(" + interfaceClassName
+ " instance) {");
println(" m_instance=instance;");
println(" }");
} else {
if ( (line.indexOf("public")!=-1)
&& (line.indexOf("throws java.rmi.RemoteException")!=-1) ) {
println("");
println(line);
i=line.indexOf("(");
String beforeParams=line.substring(0, i);
int j=beforeParams.lastIndexOf(" ");
String methodName=beforeParams.substring(j+1);
println(" String METHOD_NAME=\"" + methodName + "\";");
println(" HashMap PARMS=new HashMap();");
String thisMethodStart;
String thisMethodFinish;
if (line.indexOf(" void ")==-1) {
String afterPublic=line.substring(line.indexOf("public")+7);
String returnType=afterPublic.substring(0, afterPublic.indexOf(" "));
thisMethodStart=methodStart.replaceAll("##RETURN_TYPE##", returnType);
thisMethodFinish=methodFinish.replaceAll("##RETURN_TYPE##", returnType);
thisMethodStart=thisMethodStart.replaceAll("##RETURN##", "return ");
thisMethodFinish=thisMethodFinish.replaceAll("##RETURN##", "return ");
} else {
thisMethodStart=methodStart.replaceAll("##RETURN_TYPE##", "Object");
thisMethodFinish=methodFinish.replaceAll("##RETURN_TYPE##", "Object");
thisMethodStart=thisMethodStart.replaceAll("##RETURN##", "// ");
thisMethodFinish=thisMethodFinish.replaceAll("##RETURN##", "// ");
}
StringBuffer callBuf=new StringBuffer();
if (line.indexOf(" void ")==-1) {
callBuf.append("return ");
}
callBuf.append("m_instance." + methodName + "(");
// get the names of the parameters
String parmsAndEnd=line.substring(i+1);
String justParms=parmsAndEnd.substring(0, parmsAndEnd.indexOf(")"));
String[] sigs=justParms.split(" ");
if (sigs.length>1) {
// at least one parm
if (sigs.length==2) {
// one parm
doParm(sigs[0], sigs[1], callBuf);
} else {
// multiple parms
for (int z=0; z
© 2015 - 2025 Weber Informatics LLC | Privacy Policy