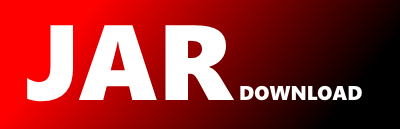
java.fedora.utilities.NamespaceContextImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fcrepo-client Show documentation
Show all versions of fcrepo-client Show documentation
The Fedora Client is a Java Library that allows API access to a Fedora Repository. The client is typically one part of a full Fedora installation.
The newest version!
/*
* -----------------------------------------------------------------------------
*
* License and Copyright: The contents of this file are subject to the
* Apache License, Version 2.0 (the "License"); you may not use
* this file except in compliance with the License. You may obtain a copy of
* the License at
* http://www.fedora-commons.org/licenses.
*
* Software distributed under the License is distributed on an "AS IS" basis,
* WITHOUT WARRANTY OF ANY KIND, either express or implied. See the License for
* the specific language governing rights and limitations under the License.
*
* The entire file consists of original code.
* Copyright © 2008 Fedora Commons, Inc.
*
Copyright © 2002-2007 The Rector and Visitors of the University of
* Virginia and Cornell University
* All rights reserved.
*
* -----------------------------------------------------------------------------
*/
/* The contents of this file are subject to the license and copyright terms
* detailed in the license directory at the root of the source tree (also
* available online at http://www.fedora.info/license/).
*/
package fedora.utilities;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
import javax.xml.XMLConstants;
import javax.xml.namespace.NamespaceContext;
/**
* An implementation of {@link NamespaceContext NamespaceContext} that provides
* an addNamespace method.
*
* @author Edwin Shin
* @version $Id: NamespaceContextImpl.java 7438 2008-07-08 06:41:33Z pangloss $
*/
public class NamespaceContextImpl
implements NamespaceContext {
/**
* Namespace URI to use to represent that there is no Namespace.
* Defined by the Namespace specification to be "".
*/
private static final String NULL_NS_URI = "";
private Map prefix2ns = new ConcurrentHashMap();
public NamespaceContextImpl() {
prefix2ns.put(XMLConstants.XML_NS_PREFIX, XMLConstants.XML_NS_URI);
prefix2ns.put(XMLConstants.XMLNS_ATTRIBUTE, XMLConstants.XMLNS_ATTRIBUTE_NS_URI);
}
public NamespaceContextImpl(String prefix, String namespaceURI) {
this();
this.addNamespace(prefix, namespaceURI);
}
/**
* Constructor that takes a Map of prefix to namespaces.
*
* @param prefix2ns a mapping of prefixes to namespaces.
* @throws IllegalArgumentException if prefix2ns contains
* {@value XMLConstants.XML_NS_URI} or {@value XMLConstants.XMLNS_ATTRIBUTE_NS_URI}
*/
public NamespaceContextImpl(Map prefix2ns) {
this();
for (String prefix : prefix2ns.keySet()) {
addNamespace(prefix, prefix2ns.get(prefix));
}
}
/**
* {@inheritDoc}
*/
public String getNamespaceURI(String prefix) {
if (prefix == null) {
throw new IllegalArgumentException("null prefix not allowed.");
}
if (prefix2ns.containsKey(prefix)) {
return prefix2ns.get(prefix);
}
return NULL_NS_URI;
}
/**
* {@inheritDoc}
*/
public String getPrefix(String namespaceURI) {
if (namespaceURI == null) {
throw new IllegalArgumentException("null namespaceURI not allowed.");
}
for (String prefix : prefix2ns.keySet()) {
if (prefix2ns.get(prefix).equals(namespaceURI)) {
return prefix;
}
}
return null;
}
/**
* {@inheritDoc}
*/
public Iterator getPrefixes(String namespaceURI) {
List prefixes = new ArrayList();
for (String prefix : prefix2ns.keySet()) {
if (prefix2ns.containsKey(prefix) &&
prefix2ns.get(prefix).equals(namespaceURI)) {
prefixes.add(prefix);
}
}
return Collections.unmodifiableList(prefixes).iterator();
}
/**
* Add a prefix to namespace mapping.
*
* @param prefix
* @param namespaceURI
* @throws IllegalArgumentException if namespaceURI is one of
* {@value XMLConstants.XML_NS_URI} or {@value XMLConstants.XMLNS_ATTRIBUTE_NS_URI}
*/
public void addNamespace(String prefix, String namespaceURI) {
if (prefix == null || namespaceURI == null) {
throw new IllegalArgumentException("null arguments not allowed.");
}
if (namespaceURI.equals(XMLConstants.XML_NS_URI)) {
throw new IllegalArgumentException("Adding a new namespace for " +
XMLConstants.XML_NS_URI + "not allowed.");
} else if (namespaceURI.equals(XMLConstants.XMLNS_ATTRIBUTE_NS_URI)) {
throw new IllegalArgumentException("Adding a new namespace for " +
XMLConstants.XMLNS_ATTRIBUTE_NS_URI + "not allowed.");
}
prefix2ns.put(prefix, namespaceURI);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy