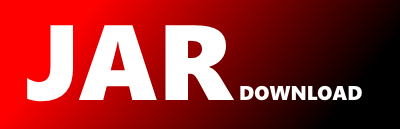
test.junit.fedora.server.resourceIndex.MockMethodInfoStore Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fcrepo-client Show documentation
Show all versions of fcrepo-client Show documentation
The Fedora Client is a Java Library that allows API access to a Fedora Repository. The client is typically one part of a full Fedora installation.
The newest version!
package fedora.server.resourceIndex;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
import fedora.server.errors.ResourceIndexException;
import fedora.server.storage.BDefReader;
import fedora.server.storage.BMechReader;
import fedora.server.storage.types.BMechDSBindSpec;
import fedora.server.storage.types.MethodDef;
import fedora.server.storage.types.MethodDefOperationBind;
import fedora.server.storage.types.MethodParmDef;
public class MockMethodInfoStore implements MethodInfoStore {
/**
* Whether permutation information should be stored.
*/
private boolean _storePermutations;
/**
* Key: bDef pid, Value: Set of method names it defines.
*/
private Map> _bDefMethods;
/**
* Key: bDef pid/methodName, Value: Set of permutations.
*/
private Map> _bDefMethodPermutations;
/**
* Key: bMech pid, Value: Associated bDef pid.
*/
private Map _bMechBDef;
/**
* Key: bMech pid, Value: Set of method names it implements.
*/
private Map> _bMechMethods;
/**
* Key: bMech pid/methodName, Value: Set of binding keys for method.
*/
private Map> _bMechMethodBindingKeys;
/**
* Key: bMech pid/methodName, Value: Set of return MIME types for method.
*/
private Map> _bMechMethodReturnTypes;
/**
* Get an empty instance.
*/
public MockMethodInfoStore(boolean storePermutations) {
_storePermutations = storePermutations;
_bDefMethods = new HashMap>();
_bDefMethodPermutations = new HashMap>();
_bMechBDef = new HashMap();
_bMechMethods = new HashMap>();
_bMechMethodBindingKeys = new HashMap>();
_bMechMethodReturnTypes = new HashMap>();
}
/**
* {@inheritDoc}
*/
public synchronized void putBDefInfo(BDefReader reader)
throws ResourceIndexException {
try {
String pid = reader.GetObjectPID();
deleteBDefInfo(pid);
MethodDef[] methodDefs = reader.getAbstractMethods(null);
Set methods = new HashSet();
for (int i = 0; i < methodDefs.length; i++) {
MethodDef def = methodDefs[i];
methods.add(def.methodName);
Set perms;
if (_storePermutations) {
perms = new ParamDomainMap(def.methodName,
def.methodParms, true).getPermutations();
} else {
perms = new HashSet();
// NOTE: This seems counterintuitive, but it mimicks the
// original implementation. If we knew it wouldn't
// cause problems, we could remove it.
perms.add(def.methodName);
}
_bDefMethodPermutations.put(pid + "/" + def.methodName, perms);
}
_bDefMethods.put(pid, methods);
} catch (Exception e) {
throw new ResourceIndexException("Error putting BDef info", e);
}
}
/**
* {@inheritDoc}
*/
public synchronized void putBMechInfo(BMechReader reader)
throws ResourceIndexException {
try {
String pid = reader.GetObjectPID();
deleteBMechInfo(pid);
BMechDSBindSpec inputSpec = reader.getServiceDSInputSpec(null);
_bMechBDef.put(pid, inputSpec.bDefPID);
MethodDefOperationBind[] methodBindings =
reader.getServiceMethodBindings(null);
Set methods = new HashSet();
for (int i = 0; i < methodBindings.length; i++) {
MethodDefOperationBind methodBinding = methodBindings[i];
methods.add(methodBinding.methodName);
String key = pid + "/" + methodBinding.methodName;
_bMechMethodBindingKeys.put(key,
getStringSet(methodBinding.dsBindingKeys));
_bMechMethodReturnTypes.put(key,
getStringSet(methodBinding.outputMIMETypes));
}
_bMechMethods.put(pid, methods);
} catch (Exception e) {
throw new ResourceIndexException("Error putting BMech info", e);
}
}
/**
* Get a set of strings for the given array of strings.
*/
private static Set getStringSet(String[] values) {
Set set = new HashSet(values.length);
for (String value : values) {
set.add(value);
}
return set;
}
/**
* {@inheritDoc}
*/
public synchronized void deleteBDefInfo(String bDefPID) {
Set methods = _bDefMethods.get(bDefPID);
if (methods != null) {
for (String method : methods) {
_bDefMethodPermutations.remove(bDefPID + "/" + method);
}
_bDefMethods.remove(bDefPID);
}
}
/**
* {@inheritDoc}
*/
public synchronized void deleteBMechInfo(String bMechPID)
throws ResourceIndexException {
_bMechBDef.remove(bMechPID);
Set methods = _bMechMethods.get(bMechPID);
if (methods != null) {
for (String method : methods) {
_bMechMethodBindingKeys.remove(bMechPID + "/" + method);
_bMechMethodReturnTypes.remove(bMechPID + "/" + method);
}
_bMechMethods.remove(bMechPID);
}
}
/**
* {@inheritDoc}
*/
public synchronized Set getMethodInfo(String bMechPID)
throws ResourceIndexException {
Set methods = _bMechMethods.get(bMechPID);
if (methods != null) {
Set methodInfo =
new HashSet(methods.size());
for (String method : methods) {
String bDefPID = _bMechBDef.get(bMechPID);
Set perms = _bDefMethodPermutations.get(bDefPID + "/" + method);
if (perms == null) {
throw new ResourceIndexException("BDef (" + bDefPID + ") "
+ "for BMech (" + bMechPID + ") is missing; "
+ "cannot determine method permutations");
}
MethodInfo info = new MethodInfo(method,
_bMechMethodBindingKeys.get(bMechPID + "/" + method),
_bMechMethodReturnTypes.get(bMechPID + "/" + method),
perms);
methodInfo.add(info);
}
return methodInfo;
} else {
throw new ResourceIndexException("No such bMech: " + bMechPID);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy