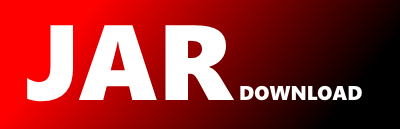
test.junit.fedora.server.storage.MockRepositoryReader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fcrepo-client Show documentation
Show all versions of fcrepo-client Show documentation
The Fedora Client is a Java Library that allows API access to a Fedora Repository. The client is typically one part of a full Fedora installation.
The newest version!
package fedora.server.storage;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
import fedora.server.Context;
import fedora.server.errors.GeneralException;
import fedora.server.errors.ObjectNotFoundException;
import fedora.server.errors.ServerException;
import fedora.server.storage.types.DigitalObject;
/**
* Mock implementation of RepositoryReader
for testing.
*
* This works by simply keeping a map of DigitalObject
* instances in memory.
*
* @author [email protected]
*/
public class MockRepositoryReader implements RepositoryReader {
/**
* The DigitalObject
s in the "repository", keyed by PID.
*/
private Map _objects = new HashMap();
/**
* Construct with an empty "repository".
*/
public MockRepositoryReader() {
}
/**
* Adds/replaces the object into the "repository".
*/
public synchronized void putObject(DigitalObject obj) {
_objects.put(obj.getPid(), obj);
}
/**
* Removes the object from the "repository" and returns it (or null
* if it didn't exist in the first place).
*/
public synchronized DigitalObject deleteObject(String pid) {
return (DigitalObject) _objects.remove(pid);
}
/**
* Get a DigitalObject
if it's in the "repository".
*
* @throws ObjectNotFoundException if it's not in the "repository".
*/
public synchronized DigitalObject getObject(String pid)
throws ObjectNotFoundException {
DigitalObject obj = (DigitalObject) _objects.get(pid);
if (obj == null) {
throw new ObjectNotFoundException("No such object: " + pid);
} else {
return obj;
}
}
// Mock methods from RepositoryReader interface.
/**
* {@inheritDoc}
*/
public synchronized DOReader getReader(boolean cachedObjectRequired,
Context context,
String pid)
throws ServerException {
DigitalObject obj = getObject(pid);
if (obj.getFedoraObjectType() != DigitalObject.FEDORA_OBJECT) {
throw new GeneralException("Not a data object: " + pid);
} else {
return new SimpleDOReader(null, this, null, null, null, obj);
}
}
/**
* {@inheritDoc}
*/
public synchronized BMechReader getBMechReader(boolean cachedObjectRequired,
Context context,
String pid)
throws ServerException {
DigitalObject obj = getObject(pid);
if (obj.getFedoraObjectType() != DigitalObject.FEDORA_BMECH_OBJECT) {
throw new GeneralException("Not a bmech object: " + pid);
} else {
return new SimpleBMechReader(null, this, null, null, null, obj);
}
}
/**
* {@inheritDoc}
*/
public synchronized BDefReader getBDefReader(boolean cachedObjectRequired,
Context context,
String pid)
throws ServerException {
DigitalObject obj = getObject(pid);
if (obj.getFedoraObjectType() != DigitalObject.FEDORA_BDEF_OBJECT) {
throw new GeneralException("Not a bdef object: " + pid);
} else {
return new SimpleBDefReader(null, this, null, null, null, obj);
}
}
/**
* {@inheritDoc}
*/
public synchronized String[] listObjectPIDs(Context context)
throws ServerException {
String[] pids = new String[_objects.keySet().size()];
Iterator iter = _objects.keySet().iterator();
int i = 0;
while (iter.hasNext()) {
pids[i++] = (String) iter.next();
}
return pids;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy