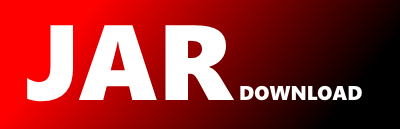
com.googlecode.sarasvati.impl.AbstractGraph Maven / Gradle / Ivy
The newest version!
/*
This file is part of Sarasvati.
Sarasvati is free software: you can redistribute it and/or modify
it under the terms of the GNU Lesser General Public License as
published by the Free Software Foundation, either version 3 of the
License, or (at your option) any later version.
Sarasvati is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU Lesser General Public License for more details.
You should have received a copy of the GNU Lesser General Public
License along with Sarasvati. If not, see .
Copyright 2008 Paul Lorenz
*/
package com.googlecode.sarasvati.impl;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import com.googlecode.sarasvati.Arc;
import com.googlecode.sarasvati.Graph;
import com.googlecode.sarasvati.Node;
import com.googlecode.sarasvati.util.SvUtil;
/**
* Provides some base logic for getting input and output arcs
* for a given node. Works by iterating over the arcs
* and building the set of associated inputs and outputs. This
* happens once, the first time a set of input or output arcs
* are requested.
*
* @author Paul Lorenz
*/
public abstract class AbstractGraph implements Graph
{
protected Map> inputMap;
protected Map> outputMap;
/**
* @see Graph#getInputArcs(Node)
*/
@Override
public List getInputArcs (final Node node)
{
if ( inputMap == null )
{
initialize();
}
List result = inputMap.get( node );
if ( result == null )
{
return Collections.emptyList();
}
return result;
}
/**
* @see Graph#getInputArcs(Node, String)
*/
@Override
public List getInputArcs (final Node node, final String arcName)
{
List arcList = getInputArcs( node );
if ( arcList.isEmpty() )
{
return arcList;
}
List result = new ArrayList( arcList.size() );
for ( Arc arc : arcList )
{
if ( SvUtil.equals( arcName, arc.getName() ) )
{
result.add( arc );
}
}
return result;
}
/**
* @see Graph#getOutputArcs(Node)
*/
@Override
public List getOutputArcs (final Node node)
{
if (outputMap == null)
{
initialize();
}
List result = outputMap.get( node );
if ( result == null )
{
return Collections.emptyList();
}
return result;
}
/**
* @see Graph#getOutputArcs(Node, String)
*/
@Override
public List getOutputArcs (final Node node, final String arcName)
{
List arcList = getOutputArcs( node );
if ( arcList.isEmpty() )
{
return arcList;
}
List result = new ArrayList( arcList.size() );
for ( Arc arc : arcList )
{
if ( SvUtil.equals( arcName, arc.getName() ) )
{
result.add( arc );
}
}
return result;
}
/**
* @see Graph#getOutputArcs(Node, String)
*/
@Override
public List getOutputArcs (final Node node, final String...arcNames)
{
if (arcNames == null || arcNames.length == 0)
{
return Collections.emptyList();
}
final List arcList = getOutputArcs( node );
if ( arcList.isEmpty() )
{
return arcList;
}
final List result = new ArrayList( arcList.size() );
for (final Arc arc : arcList)
{
for (final String arcName : arcNames)
{
if ( SvUtil.equals( arcName, arc.getName() ) )
{
result.add( arc );
}
}
}
return result;
}
private void initialize ()
{
inputMap = new HashMap>();
outputMap = new HashMap>();
for ( Arc arc : getArcs() )
{
Node node = arc.getStartNode();
List list = outputMap.get( node );
if ( list == null )
{
list = new LinkedList();
outputMap.put( node, list );
}
list.add( arc );
node = arc.getEndNode();
list = inputMap.get( node );
if ( list == null )
{
list = new LinkedList();
inputMap.put( node, list );
}
list.add( arc );
}
}
/**
* @see Graph#getStartNodes()
*/
@Override
public List getStartNodes ()
{
List startNodes = new LinkedList();
for ( Node node : getNodes() )
{
if ( node.isStart() )
{
startNodes.add( node );
}
}
return startNodes;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy