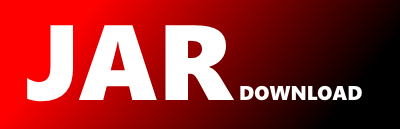
com.googlecode.sarasvati.impl.TimerBasedDelayedTokenScheduler Maven / Gradle / Ivy
The newest version!
/*
This file is part of Sarasvati.
Sarasvati is free software: you can redistribute it and/or modify
it under the terms of the GNU Lesser General Public License as
published by the Free Software Foundation, either version 3 of the
License, or (at your option) any later version.
Sarasvati is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU Lesser General Public License for more details.
You should have received a copy of the GNU Lesser General Public
License along with Sarasvati. If not, see .
Copyright 2012 Paul Lorenz
*/
package com.googlecode.sarasvati.impl;
import java.util.Timer;
import java.util.TimerTask;
import com.googlecode.sarasvati.DelayedTokenScheduler;
import com.googlecode.sarasvati.Engine;
import com.googlecode.sarasvati.EngineFactory;
import com.googlecode.sarasvati.NodeToken;
public enum TimerBasedDelayedTokenScheduler
{
INSTANCE;
// Make sure this isn't initialized until first use
private static enum TimerContainer
{
TIMER_SINGLETON;
public final Timer timer = new Timer(getClass().getName() + "TimerThread", true);
}
private static Timer getTimer()
{
return TimerContainer.TIMER_SINGLETON.timer;
}
public static DelayedTokenScheduler newDelayedTokenScheduler(final EngineFactory engineFactory)
{
return new DelayedTokenScheduler()
{
@Override
public void scheduleDelayedToken(final NodeToken token)
{
TimerBasedDelayedTokenScheduler.INSTANCE.scheduleDelayedToken(token, engineFactory);
}
};
}
public void scheduleDelayedToken(final NodeToken token, final EngineFactory engineFactory)
{
getTimer().schedule(new TokenReevaluateTimerTask(engineFactory, token), token.getDelayUntilTime());
}
public static void shutdown()
{
getTimer().cancel();
}
private class TokenReevaluateTimerTask extends TimerTask
{
private final EngineFactory engineFactory;
private final NodeToken token;
public TokenReevaluateTimerTask (final EngineFactory engineFactory, final NodeToken token)
{
this.engineFactory = engineFactory;
this.token = token;
}
/**
* @see java.util.TimerTask#run()
*/
@Override
public void run()
{
final T engine = engineFactory.getEngine();
try
{
engine.reevaluateDelayedToken(token);
engineFactory.dispose(engine);
}
catch(final Throwable t)
{
engineFactory.dispose(engine, t);
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy