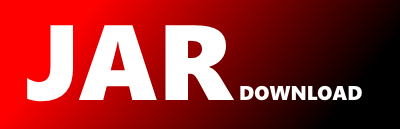
com.googlecode.sarasvati.mem.MemGraphRepository Maven / Gradle / Ivy
The newest version!
/*
This file is part of Sarasvati.
Sarasvati is free software: you can redistribute it and/or modify
it under the terms of the GNU Lesser General Public License as
published by the Free Software Foundation, either version 3 of the
License, or (at your option) any later version.
Sarasvati is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU Lesser General Public License for more details.
You should have received a copy of the GNU Lesser General Public
License along with Sarasvati. If not, see .
Copyright 2008 Paul Lorenz
*/
package com.googlecode.sarasvati.mem;
import java.util.ArrayList;
import java.util.Collections;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
import com.googlecode.sarasvati.GraphProcess;
import com.googlecode.sarasvati.NodeToken;
import com.googlecode.sarasvati.load.GraphRepository;
/**
* A Graph Repository which can either store graphs in a global/static or local/per-repository cache.
*
* @author Paul Lorenz
*/
public class MemGraphRepository implements GraphRepository
{
private static final Map globalCache = new ConcurrentHashMap();
public static final MemGraphRepository INSTANCE = new MemGraphRepository();
private final Map cache;
public MemGraphRepository()
{
this(true);
}
public MemGraphRepository(final boolean useGlobalCache)
{
this.cache = useGlobalCache ? globalCache : new ConcurrentHashMap();
}
@Override
public void addGraph (final MemGraph graph)
{
cache.put( graph.getName(), graph );
}
@Override
public List getGraphs(final String name)
{
final MemGraph graph = cache.get( name );
if ( graph == null )
{
return Collections.emptyList();
}
return Collections.singletonList( graph );
}
@Override
public List getGraphs()
{
final ArrayList graphs = new ArrayList( cache.size() );
graphs.addAll( cache.values() );
return graphs;
}
@Override
public MemGraph getLatestGraph(final String name)
{
return cache.get( name );
}
/* (non-Javadoc)
* @see com.googlecode.sarasvati.load.GraphRepository#getActiveNestedProcesses(com.googlecode.sarasvati.GraphProcess)
*/
@Override
public List getActiveNestedProcesses(final GraphProcess process)
{
List processes = new LinkedList();
for (final NodeToken t : process.getActiveNodeTokens())
{
MemNodeToken token = (MemNodeToken) t;
if (token.getNestedProcess() != null && !token.getNestedProcess().isComplete() && !token.getNestedProcess().isCanceled())
{
processes.add(token.getNestedProcess());
}
}
return processes;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy