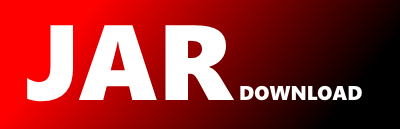
com.googlecode.sarasvati.xml.XmlProcessDefinition Maven / Gradle / Ivy
The newest version!
/*
This file is part of Sarasvati.
Sarasvati is free software: you can redistribute it and/or modify
it under the terms of the GNU Lesser General Public License as
published by the Free Software Foundation, either version 3 of the
License, or (at your option) any later version.
Sarasvati is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU Lesser General Public License for more details.
You should have received a copy of the GNU Lesser General Public
License along with Sarasvati. If not, see .
Copyright 2008 Paul Lorenz
*/
package com.googlecode.sarasvati.xml;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.TreeMap;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlTransient;
import com.googlecode.sarasvati.SarasvatiException;
import com.googlecode.sarasvati.load.SarasvatiLoadException;
import com.googlecode.sarasvati.load.definition.ExternalDefinition;
import com.googlecode.sarasvati.load.definition.NodeDefinition;
import com.googlecode.sarasvati.load.definition.ProcessDefinition;
import com.googlecode.sarasvati.load.properties.DOMToObjectLoadHelper;
import com.googlecode.sarasvati.util.SvUtil;
@XmlRootElement(name = "process-definition")
@XmlAccessorType(XmlAccessType.FIELD)
public class XmlProcessDefinition implements ProcessDefinition
{
@XmlAttribute(name = "name", required = true)
protected String name;
@XmlElement(name = "node")
protected List nodes = new ArrayList();
@XmlElement(name = "external")
protected List externals = new ArrayList();
@XmlElement(name="custom")
protected XmlCustom custom;
@XmlTransient
protected String messageDigest = null;
@Override
public String getName ()
{
return name;
}
public void setName (final String name)
{
this.name = name;
}
@Override
public List getNodes ()
{
return nodes;
}
public void setNodes (final List nodes)
{
this.nodes = nodes;
}
@Override
public List getExternals ()
{
return externals;
}
public void setExternals (final List externals)
{
this.externals = externals;
}
@Override
public List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy