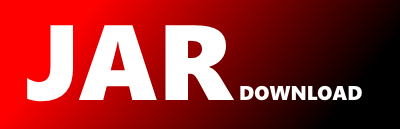
javazoom.spi.mpeg.sampled.file.MpegAudioFileFormat Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mp3spi Show documentation
Show all versions of mp3spi Show documentation
Maven artifact for MP3SPI library. http://www.javazoom.net/mp3spi/mp3spi.html
The newest version!
/*
* MpegAudioFileFormat.
*
* JavaZOOM : [email protected]
* http://www.javazoom.net
*
*-----------------------------------------------------------------------
* This program is free software; you can redistribute it and/or modify
* it under the terms of the GNU Library General Public License as published
* by the Free Software Foundation; either version 2 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Library General Public License for more details.
*
* You should have received a copy of the GNU Library General Public
* License along with this program; if not, write to the Free Software
* Foundation, Inc., 675 Mass Ave, Cambridge, MA 02139, USA.
*----------------------------------------------------------------------
*/
package javazoom.spi.mpeg.sampled.file;
import java.util.Map;
import javax.sound.sampled.AudioFormat;
import org.tritonus.share.sampled.file.TAudioFileFormat;
/**
* @author JavaZOOM
*/
public class MpegAudioFileFormat extends TAudioFileFormat
{
/**
* Contructor.
* @param type
* @param audioFormat
* @param nLengthInFrames
* @param nLengthInBytes
*/
public MpegAudioFileFormat(Type type, AudioFormat audioFormat, int nLengthInFrames, int nLengthInBytes, Map properties)
{
super(type, audioFormat, nLengthInFrames, nLengthInBytes, properties);
}
/**
* MP3 audio file format parameters.
* Some parameters might be unavailable. So availability test is required before reading any parameter.
*
*
AudioFileFormat parameters.
*
* - duration [Long], duration in microseconds.
*
- title [String], Title of the stream.
*
- author [String], Name of the artist of the stream.
*
- album [String], Name of the album of the stream.
*
- date [String], The date (year) of the recording or release of the stream.
*
- copyright [String], Copyright message of the stream.
*
- comment [String], Comment of the stream.
*
*
MP3 parameters.
*
* - mp3.version.mpeg [String], mpeg version : 1,2 or 2.5
*
- mp3.version.layer [String], layer version 1, 2 or 3
*
- mp3.version.encoding [String], mpeg encoding : MPEG1, MPEG2-LSF, MPEG2.5-LSF
*
- mp3.channels [Integer], number of channels 1 : mono, 2 : stereo.
*
- mp3.frequency.hz [Integer], sampling rate in hz.
*
- mp3.bitrate.nominal.bps [Integer], nominal bitrate in bps.
*
- mp3.length.bytes [Integer], length in bytes.
*
- mp3.length.frames [Integer], length in frames.
*
- mp3.framesize.bytes [Integer], framesize of the first frame. framesize is not constant for VBR streams.
*
- mp3.framerate.fps [Float], framerate in frames per seconds.
*
- mp3.header.pos [Integer], position of first audio header (or ID3v2 size).
*
- mp3.vbr [Boolean], vbr flag.
*
- mp3.vbr.scale [Integer], vbr scale.
*
- mp3.crc [Boolean], crc flag.
*
- mp3.original [Boolean], original flag.
*
- mp3.copyright [Boolean], copyright flag.
*
- mp3.padding [Boolean], padding flag.
*
- mp3.mode [Integer], mode 0:STEREO 1:JOINT_STEREO 2:DUAL_CHANNEL 3:SINGLE_CHANNEL
*
- mp3.id3tag.genre [String], ID3 tag (v1 or v2) genre.
*
- mp3.id3tag.track [String], ID3 tag (v1 or v2) track info.
*
- mp3.id3tag.encoded [String], ID3 tag v2 encoded by info.
*
- mp3.id3tag.composer [String], ID3 tag v2 composer info.
*
- mp3.id3tag.grouping [String], ID3 tag v2 grouping info.
*
- mp3.id3tag.disc [String], ID3 tag v2 track info.
*
- mp3.id3tag.publisher [String], ID3 tag v2 publisher info.
*
- mp3.id3tag.orchestra [String], ID3 tag v2 orchestra info.
*
- mp3.id3tag.length [String], ID3 tag v2 file length in seconds.
*
- mp3.id3tag.v2 [InputStream], ID3v2 frames.
*
- mp3.id3tag.v2.version [String], ID3v2 major version (2=v2.2.0, 3=v2.3.0, 4=v2.4.0).
*
- mp3.shoutcast.metadata.key [String], Shoutcast meta key with matching value.
*
For instance :
*
mp3.shoutcast.metadata.icy-irc=#shoutcast
*
mp3.shoutcast.metadata.icy-metaint=8192
*
mp3.shoutcast.metadata.icy-genre=Trance Techno Dance
*
mp3.shoutcast.metadata.icy-url=http://www.di.fm
*
and so on ...
*
*/
public Map properties()
{
return super.properties();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy