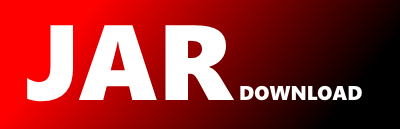
org.springframework.contributions.ioc.util.CollectionFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spring-contributions Show documentation
Show all versions of spring-contributions Show documentation
This project adds a so called contribution mechanism (like known from Tapestry IOC or Eclipse Plugins) for configuration and extension of services to the Spring project.
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package org.springframework.contributions.ioc.util;
import java.util.*;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentMap;
import java.util.concurrent.CopyOnWriteArrayList;
/**
* Modified Copy of {@link org.apache.tapestry5.ioc.internal.util.CollectionFactory} from Tapestry in version 5.2.5
*
* Static factory methods to ease the creation of new collection types (when using generics). Most of these method
* leverage the compiler's ability to match generic types by return value. Typical usage (with a static import):
*
*
* Map<Foo, Bar> map = newMap();
*
*
*
* This is a replacement for:
*
*
* Map<Foo, Bar> map = new HashMap<Foo, Bar>();
*
*/
public final class CollectionFactory
{
/**
* Constructs and returns a generic {@link HashMap} instance.
*/
public static Map newMap()
{
return new HashMap();
}
/**
* Constructs and returns a generic {@link java.util.HashSet} instance.
*/
public static Set newSet()
{
return new HashSet();
}
/**
* Contructs a new {@link HashSet} and initializes it using the provided collection.
*/
public static Set newSet(Collection values)
{
return new HashSet(values);
}
public static Set newSet(V... values)
{
// Was a call to newSet(), but Sun JDK can't handle that. Fucking generics.
return new HashSet(Arrays.asList(values));
}
/**
* Constructs a new {@link java.util.HashMap} instance by copying an existing Map instance.
*/
public static Map newMap(Map extends K, ? extends V> map)
{
return new HashMap(map);
}
/**
* Constructs a new concurrent map, which is safe to access via multiple threads.
*/
public static ConcurrentMap newConcurrentMap()
{
return new ConcurrentHashMap();
}
/**
* Contructs and returns a new generic {@link java.util.ArrayList} instance.
*/
public static List newList()
{
return new ArrayList();
}
/**
* Creates a new, fully modifiable list from an initial set of elements.
*/
public static List newList(V... elements)
{
// Was call to newList(), but Sun JDK can't handle that.
return new ArrayList(Arrays.asList(elements));
}
/**
* Useful for queues.
*/
public static LinkedList newLinkedList()
{
return new LinkedList();
}
/**
* Constructs and returns a new {@link java.util.ArrayList} as a copy of the provided collection.
*/
public static List newList(Collection list)
{
return new ArrayList(list);
}
/**
* Constructs and returns a new {@link java.util.concurrent.CopyOnWriteArrayList}.
*/
public static List newThreadSafeList()
{
return new CopyOnWriteArrayList();
}
public static Stack newStack()
{
return new Stack();
}
public static Stack newStack(int initialSize)
{
return new Stack(initialSize);
}
public static Map newCaseInsensitiveMap()
{
return new CaseInsensitiveMap();
}
public static Map newCaseInsensitiveMap(Map map)
{
return new CaseInsensitiveMap(map);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy