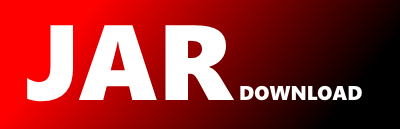
com.google.code.yanf4j.util.SimpleQueue Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xmemcached Show documentation
Show all versions of xmemcached Show documentation
Extreme performance modern memcached client for java
package com.google.code.yanf4j.util;
import java.util.Iterator;
import java.util.LinkedList;
/**
* Simple queue. All methods are thread-safe.
*
* @author dennis zhuang
*/
public class SimpleQueue extends java.util.AbstractQueue {
protected final LinkedList list;
public SimpleQueue(int initializeCapacity) {
this.list = new LinkedList();
}
public SimpleQueue() {
this(100);
}
public synchronized boolean offer(T e) {
return this.list.add(e);
}
public synchronized T peek() {
return this.list.peek();
}
public synchronized T poll() {
return this.list.poll();
}
@Override
public Iterator iterator() {
return this.list.iterator();
}
@Override
public int size() {
return this.list.size();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy