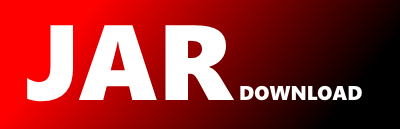
com.gradle.develocity.agent.maven.api.DevelocityApi Maven / Gradle / Ivy
Show all versions of develocity-maven-extension Show documentation
package com.gradle.develocity.agent.maven.api;
import com.gradle.develocity.agent.maven.api.cache.BuildCacheApi;
import com.gradle.develocity.agent.maven.api.scan.BuildScanApi;
import javax.annotation.Nullable;
import java.net.URI;
import java.nio.file.Path;
/**
* Allows to interact with the Develocity Maven extension.
*
* @since 1.21
*/
public interface DevelocityApi {
/**
* Whether the Develocity Maven extension is enabled.
*
* @return {@code true} if the Develocity Maven extension is enabled, {@code false} otherwise
*/
boolean isEnabled();
/**
* Sets whether to enable the Develocity Maven extension.
*
* Configuration via the {@code develocity.enabled} system property will always take precedence.
*
* @param enabled whether to enable the Develocity Maven extension
*/
void setEnabled(boolean enabled);
/**
* Returns the project identifier.
*
* @return the project identifier or {@code null}
*/
@Nullable
String getProjectId();
/**
* Sets the project identifier. The value must not be empty or exceed 256 characters.
*
* @param projectId the project identifier or {@code null}
*/
void setProjectId(@Nullable String projectId);
/**
* Returns the Develocity Maven extension storage directory.
*
* @return the Develocity Maven extension storage directory.
* @see Develocity Maven extension documentation.
*/
Path getStorageDirectory();
/**
* Sets the Develocity Maven extension storage directory to the specified path.
*
* Configuration via the {@code develocity.storage.directory} system property will always take precedence.
*
* @param path The new storage directory
* @see Develocity Maven extension documentation.
*/
void setStorageDirectory(Path path);
/**
* Returns the URL of the Develocity server.
*
* @return the Develocity server or {@code null}
*/
@Nullable
String getServer();
/**
* Sets the URL of the Develocity server.
*
* Configuration via the {@code develocity.url} system property will always take precedence.
*
* @param url the server URL or {@code null}
*/
default void setServer(@Nullable String url) {
setServer(url == null ? null : URI.create(url));
}
/**
* Sets the URL of the Develocity server.
*
* Configuration via the {@code develocity.url} system property will always take precedence.
*
* @param url the server URL or {@code null}
*/
void setServer(@Nullable URI url);
/**
* Whether it is acceptable to communicate with a Develocity server with an untrusted SSL certificate.
*
* @return true
if it is acceptable to communicate with a Develocity server with an untrusted SSL certificate
**/
boolean getAllowUntrustedServer();
/**
* Specifies whether it is acceptable to communicate with a Develocity server using an untrusted SSL certificate.
*
* The default (public) Develocity server uses SSL certificates that are trusted by default by standard modern Java environments.
* If you are using a different Develocity server, it may use an untrusted certificate.
* This may be due to the use of an internally provisioned or self-signed certificate.
*
* In such a scenario, you can either configure the build JVM environment to trust the certificate,
* or call this method with {@code true} to disable verification of the server's identity.
* Alternatively, you may disable SSL completely for Develocity installation but this is not recommended.
*
* Allowing communication with untrusted servers keeps data encrypted during transmission,
* but makes it easy for a man-in-the-middle to impersonate the intended server and capture data.
*
* This value has no effect if a server is specified using the HTTP protocol (i.e. has SSL disabled).
*
* Configuration via the {@code develocity.allowUntrustedServer} system property will always take precedence.
*
* @param allow whether to allow communication with an HTTPS server with an untrusted certificate
*/
void setAllowUntrustedServer(boolean allow);
/**
* Returns the access key for authenticating with the Develocity server.
*
* Only the value of the explicitly configured access key (via {@link #setAccessKey(String)} or {@code develocity.xml}) is returned but
* not the value of an access key configured via the {@code DEVELOCITY_ACCESS_KEY} environment variable or access key file entry.
*
* @return the configured Develocity server access key, if available; otherwise, {@code null}
*/
@Nullable
String getAccessKey();
/**
* Sets the access key for authenticating with the Develocity server.
*
* An access key configured this way will take precedence over the {@code DEVELOCITY_ACCESS_KEY}
* environment variable or access key file entry associated with the server.
*
* @param accessKey a Develocity server access key without any hostname prefix
*/
void setAccessKey(String accessKey);
/**
* The build scan API.
*
* @return the build scan API
*/
BuildScanApi getBuildScan();
/**
* The build cache API.
*
* @return the build cache API
*/
BuildCacheApi getBuildCache();
}