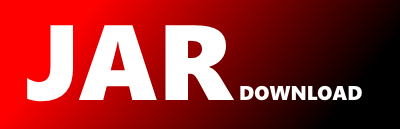
com.gradle.develocity.agent.maven.api.cache.Server Maven / Gradle / Ivy
Show all versions of develocity-maven-extension Show documentation
package com.gradle.develocity.agent.maven.api.cache;
import com.gradle.develocity.agent.maven.api.DevelocityApi;
import javax.annotation.Nullable;
import java.net.URI;
/**
* Configures the remote build cache node server.
*
* The specified values will take precedence over the values defined in the {@code develocity.xml}.
*
* @since 1.21
*/
public interface Server {
/**
* Gets the remote build cache server ID.
*
* @return the remote build cache server ID or {@code null}
*/
@Nullable
String getServerId();
/**
* Sets the remote build cache server ID.
*
* Configuration via the {@code develocity.cache.remote.serverId} system property will always take precedence.
*
* @param serverId the remote build cache server ID or {@code null}
*/
void setServerId(@Nullable String serverId);
/**
* Gets the remote build cache node URL.
*
* @return the remote build cache node URL or {@code null}
*/
@Nullable
URI getUrl();
/**
* Sets the remote build cache node URL.
*
* Configuration via the {@code develocity.cache.remote.url} system property will always take precedence.
*
* @param url the remote build cache node URL or {@code null}
*/
default void setUrl(@Nullable String url) {
setUrl(url == null ? null : URI.create(url));
}
/**
* Sets the remote build cache node URL.
*
* Configuration via the {@code develocity.cache.remote.url} system property will always take precedence.
*
* @param url the remote build cache node URL or {@code null}
*/
void setUrl(@Nullable URI url);
/**
* Gets whether it is allowed to communicate with the remote build cache node with an untrusted SSL certificate.
*
* @return {@code true} if it is allowed to communicate with the remote build cache node with an untrusted SSL certificate, {@code false} otherwise
*/
boolean isAllowUntrusted();
/**
* Sets whether it is allowed to communicate with the remote build cache node with an untrusted SSL certificate.
*
* The default (built-in) remote build cache node uses SSL certificates that are trusted by default by standard modern Java environments.
* If you are using a different remote build cache node, it may use an untrusted certificate.
* This may be due to the use of an internally provisioned or self-signed certificate.
*
* In such a scenario, you can either configure the build JVM environment to trust the certificate,
* or call this method with {@code true} to disable verification of the remote build cache node identity.
*
* Allowing communication with untrusted servers keeps data encrypted during transmission,
* but makes it easy for a man-in-the-middle to impersonate the intended server and capture data.
*
*
* Setting this property to {@code true} is a convenient workaround during the initial evaluation, but it is a serious
* security issue and should not be used in production.
*
*
* This value has no effect if the remote build cache node URL is specified using the HTTP protocol (i.e. has SSL disabled).
*
* Configuration via the {@code develocity.cache.remote.allowUntrustedServer} system property will always take precedence.
*
* @param allowUntrusted whether to allow communication with an HTTPS server with an untrusted certificate
*/
void setAllowUntrusted(boolean allowUntrusted);
/**
* Gets whether it is allowed to communicate with the remote build cache node over an insecure HTTP connection.
*
* @return {@code true} if communication over an insecure HTTP connection is allowed, {@code false} otherwise
*/
boolean isAllowInsecureProtocol();
/**
* Sets whether it is allowed to communicate with the remote build cache node over an insecure HTTP connection.
*
* For security purposes this intentionally requires a user to opt in to using insecure protocols on case by case basis.
*
* Allowing communication over insecure protocols allows for a man-in-the-middle to impersonate the intended server,
* and gives an attacker the ability to serve malicious executable code onto the system.
*
*
* Setting this property to {@code true} is a convenient workaround during the initial evaluation, but it is a serious
* security issue and should not be used in production.
*
*
* Configuration via the {@code develocity.cache.remote.allowInsecureProtocol} system property will always take precedence.
*
* @param allowInsecureProtocol whether to allow communication over an insecure HTTP connection
*/
void setAllowInsecureProtocol(boolean allowInsecureProtocol);
/**
* Gets whether the client should use HTTP Expect-Continue when storing data on the server.
*
* @return {@code true} if the client uses HTTP Expect-Continue, {@code false} otherwise
*/
boolean isUseExpectContinue();
/**
* Sets whether the client should use HTTP Expect-Continue when storing data on the server.
*
* Configuration via the {@code develocity.cache.remote.useExpectContinue} system property will always take precedence.
*
* @param useExpectContinue whether the client should use HTTP Expect-Continue
*/
void setUseExpectContinue(boolean useExpectContinue);
/**
* Gets the credentials configuration.
*
* If not specified and an access key is available for the {@link DevelocityApi#getServer()} value, it will be used to authenticate with the build cache
* (regardless of the build cache server address set with {@link #setUrl(String)} or {@link #setUrl(URI)}).
*
* @return the credentials configuration
*/
Credentials getCredentials();
}